mn
Embed:
(wiki syntax)
Show/hide line numbers
Thermistor.cpp
00001 /* 00002 * Thermistor Temperature library 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS, 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 */ 00015 #include "mbed.h" 00016 #include "Thermistor.h" 00017 00018 00019 Thermistor::Thermistor(PinName pin) : _pin(pin) { // _pin(pin) means pass pin to the AnalogIn constructor 00020 init(); 00021 } 00022 00023 void Thermistor::init() { 00024 ThermistorNominal = THERMISTORNOMINAL; 00025 TemperatureNominal = TEMPERATURENOMINAL; 00026 BCoefficient = BCOEFFICIENT; 00027 SeriesResistor = SERIESRESISTOR; 00028 } 00029 00030 // This is the workhorse routine that calculates the temperature 00031 // using the Steinhart-Hart equation for thermistors 00032 // https://en.wikipedia.org/wiki/Steinhart%E2%80%93Hart_equation 00033 float Thermistor::get_temperature() 00034 { 00035 double temperature =0, resistance =0; 00036 double steinhart =0; 00037 double a=0; 00038 int smooth = 5; // Number of samples to smooth 00039 00040 for(int i=0;i<smooth;i++) { 00041 a += _pin.read_u16(); // Read 16bit Analog value 00042 } 00043 a = a/smooth; // Get average of samples 00044 // pc.printf("Raw Analog Value for Thermistor = %d\r\n",a); 00045 00046 /* Calculate the resistance of the thermistor from analog votage read. */ 00047 resistance = (float) SeriesResistor / ((65536.0 / a) - 1); 00048 // pc.printf("Resistance for Thermistor = %f\r\n",resistance); 00049 00050 steinhart = resistance / ThermistorNominal; // (R/Ro) 00051 steinhart = log(steinhart); // ln(R/Ro) 00052 steinhart /= BCoefficient; // 1/B * ln(R/Ro) 00053 steinhart += 1.0 / (TemperatureNominal + 273.15); // + (1/To) 00054 steinhart = 1.0 / steinhart; // Invert 00055 temperature = steinhart - 273.15; // convert to C 00056 00057 return temperature; 00058 } 00059 00060 void Thermistor::set_ThermistorNominal(float thermnom) { 00061 ThermistorNominal = thermnom; 00062 } 00063 void Thermistor::set_TemperatureNominal(float tempnom){ 00064 TemperatureNominal = tempnom; 00065 } 00066 void Thermistor::set_BCoefficient(float bcoefficient){ 00067 BCoefficient = bcoefficient; 00068 } 00069 void Thermistor::set_SeriesResistor(float resistor){ 00070 SeriesResistor = resistor; 00071 } 00072 00073 00074 00075
Generated on Thu Jul 28 2022 14:08:26 by
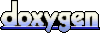