
debug
Dependencies: mbed mbed-rtos PinDetect X_NUCLEO_53L0A1
LSM9DS1_Types.h
00001 /****************************************************************************** 00002 LSM9DS1_Types.h 00003 SFE_LSM9DS1 Library - LSM9DS1 Types and Enumerations 00004 Jim Lindblom @ SparkFun Electronics 00005 Original Creation Date: April 21, 2015 00006 https://github.com/sparkfun/LSM9DS1_Breakout 00007 00008 This file defines all types and enumerations used by the LSM9DS1 class. 00009 00010 Development environment specifics: 00011 IDE: Arduino 1.6.0 00012 Hardware Platform: Arduino Uno 00013 LSM9DS1 Breakout Version: 1.0 00014 00015 This code is beerware; if you see me (or any other SparkFun employee) at the 00016 local, and you've found our code helpful, please buy us a round! 00017 00018 Distributed as-is; no warranty is given. 00019 ******************************************************************************/ 00020 00021 #ifndef __LSM9DS1_Types_H__ 00022 #define __LSM9DS1_Types_H__ 00023 00024 #include "LSM9DS1_Registers.h" 00025 00026 // The LSM9DS1 functions over both I2C or SPI. This library supports both. 00027 // But the interface mode used must be sent to the LSM9DS1 constructor. Use 00028 // one of these two as the first parameter of the constructor. 00029 enum interface_mode 00030 { 00031 IMU_MODE_SPI, 00032 IMU_MODE_I2C, 00033 }; 00034 00035 // accel_scale defines all possible FSR's of the accelerometer: 00036 enum accel_scale 00037 { 00038 A_SCALE_2G, // 00: 2g 00039 A_SCALE_16G,// 01: 16g 00040 A_SCALE_4G, // 10: 4g 00041 A_SCALE_8G // 11: 8g 00042 }; 00043 00044 // gyro_scale defines the possible full-scale ranges of the gyroscope: 00045 enum gyro_scale 00046 { 00047 G_SCALE_245DPS, // 00: 245 degrees per second 00048 G_SCALE_500DPS, // 01: 500 dps 00049 G_SCALE_2000DPS, // 11: 2000 dps 00050 }; 00051 00052 // mag_scale defines all possible FSR's of the magnetometer: 00053 enum mag_scale 00054 { 00055 M_SCALE_4GS, // 00: 4Gs 00056 M_SCALE_8GS, // 01: 8Gs 00057 M_SCALE_12GS, // 10: 12Gs 00058 M_SCALE_16GS, // 11: 16Gs 00059 }; 00060 00061 // gyro_odr defines all possible data rate/bandwidth combos of the gyro: 00062 enum gyro_odr 00063 { 00064 //! TODO 00065 G_ODR_PD, // Power down (0) 00066 G_ODR_149, // 14.9 Hz (1) 00067 G_ODR_595, // 59.5 Hz (2) 00068 G_ODR_119, // 119 Hz (3) 00069 G_ODR_238, // 238 Hz (4) 00070 G_ODR_476, // 476 Hz (5) 00071 G_ODR_952 // 952 Hz (6) 00072 }; 00073 // accel_oder defines all possible output data rates of the accelerometer: 00074 enum accel_odr 00075 { 00076 XL_POWER_DOWN, // Power-down mode (0x0) 00077 XL_ODR_10, // 10 Hz (0x1) 00078 XL_ODR_50, // 50 Hz (0x02) 00079 XL_ODR_119, // 119 Hz (0x3) 00080 XL_ODR_238, // 238 Hz (0x4) 00081 XL_ODR_476, // 476 Hz (0x5) 00082 XL_ODR_952 // 952 Hz (0x6) 00083 }; 00084 00085 // accel_abw defines all possible anti-aliasing filter rates of the accelerometer: 00086 enum accel_abw 00087 { 00088 A_ABW_408, // 408 Hz (0x0) 00089 A_ABW_211, // 211 Hz (0x1) 00090 A_ABW_105, // 105 Hz (0x2) 00091 A_ABW_50, // 50 Hz (0x3) 00092 }; 00093 00094 00095 // mag_odr defines all possible output data rates of the magnetometer: 00096 enum mag_odr 00097 { 00098 M_ODR_0625, // 0.625 Hz (0) 00099 M_ODR_125, // 1.25 Hz (1) 00100 M_ODR_250, // 2.5 Hz (2) 00101 M_ODR_5, // 5 Hz (3) 00102 M_ODR_10, // 10 Hz (4) 00103 M_ODR_20, // 20 Hz (5) 00104 M_ODR_40, // 40 Hz (6) 00105 M_ODR_80 // 80 Hz (7) 00106 }; 00107 00108 enum interrupt_select 00109 { 00110 XG_INT1 = INT1_CTRL, 00111 XG_INT2 = INT2_CTRL 00112 }; 00113 00114 enum interrupt_generators 00115 { 00116 INT_DRDY_XL = (1<<0), // Accelerometer data ready (INT1 & INT2) 00117 INT_DRDY_G = (1<<1), // Gyroscope data ready (INT1 & INT2) 00118 INT1_BOOT = (1<<2), // Boot status (INT1) 00119 INT2_DRDY_TEMP = (1<<2),// Temp data ready (INT2) 00120 INT_FTH = (1<<3), // FIFO threshold interrupt (INT1 & INT2) 00121 INT_OVR = (1<<4), // Overrun interrupt (INT1 & INT2) 00122 INT_FSS5 = (1<<5), // FSS5 interrupt (INT1 & INT2) 00123 INT_IG_XL = (1<<6), // Accel interrupt generator (INT1) 00124 INT1_IG_G = (1<<7), // Gyro interrupt enable (INT1) 00125 INT2_INACT = (1<<7), // Inactivity interrupt output (INT2) 00126 }; 00127 00128 enum accel_interrupt_generator 00129 { 00130 XLIE_XL = (1<<0), 00131 XHIE_XL = (1<<1), 00132 YLIE_XL = (1<<2), 00133 YHIE_XL = (1<<3), 00134 ZLIE_XL = (1<<4), 00135 ZHIE_XL = (1<<5), 00136 GEN_6D = (1<<6) 00137 }; 00138 00139 enum gyro_interrupt_generator 00140 { 00141 XLIE_G = (1<<0), 00142 XHIE_G = (1<<1), 00143 YLIE_G = (1<<2), 00144 YHIE_G = (1<<3), 00145 ZLIE_G = (1<<4), 00146 ZHIE_G = (1<<5) 00147 }; 00148 00149 enum mag_interrupt_generator 00150 { 00151 ZIEN = (1<<5), 00152 YIEN = (1<<6), 00153 XIEN = (1<<7) 00154 }; 00155 00156 enum h_lactive 00157 { 00158 INT_ACTIVE_HIGH, 00159 INT_ACTIVE_LOW 00160 }; 00161 00162 enum pp_od 00163 { 00164 INT_PUSH_PULL, 00165 INT_OPEN_DRAIN 00166 }; 00167 00168 enum fifoMode_type 00169 { 00170 FIFO_OFF = 0, 00171 FIFO_THS = 1, 00172 FIFO_CONT_TRIGGER = 3, 00173 FIFO_OFF_TRIGGER = 4, 00174 FIFO_CONT = 5 00175 }; 00176 00177 struct gyroSettings 00178 { 00179 // Gyroscope settings: 00180 uint8_t enabled; 00181 uint16_t scale; // Changed this to 16-bit 00182 uint8_t sampleRate; 00183 // New gyro stuff: 00184 uint8_t bandwidth; 00185 uint8_t lowPowerEnable; 00186 uint8_t HPFEnable; 00187 uint8_t HPFCutoff; 00188 uint8_t flipX; 00189 uint8_t flipY; 00190 uint8_t flipZ; 00191 uint8_t orientation; 00192 uint8_t enableX; 00193 uint8_t enableY; 00194 uint8_t enableZ; 00195 uint8_t latchInterrupt; 00196 }; 00197 00198 struct deviceSettings 00199 { 00200 uint8_t commInterface; // Can be I2C, SPI 4-wire or SPI 3-wire 00201 uint8_t agAddress; // I2C address or SPI CS pin 00202 uint8_t mAddress; // I2C address or SPI CS pin 00203 }; 00204 00205 struct accelSettings 00206 { 00207 // Accelerometer settings: 00208 uint8_t enabled; 00209 uint8_t scale; 00210 uint8_t sampleRate; 00211 // New accel stuff: 00212 uint8_t enableX; 00213 uint8_t enableY; 00214 uint8_t enableZ; 00215 int8_t bandwidth; 00216 uint8_t highResEnable; 00217 uint8_t highResBandwidth; 00218 }; 00219 00220 struct magSettings 00221 { 00222 // Magnetometer settings: 00223 uint8_t enabled; 00224 uint8_t scale; 00225 uint8_t sampleRate; 00226 // New mag stuff: 00227 uint8_t tempCompensationEnable; 00228 uint8_t XYPerformance; 00229 uint8_t ZPerformance; 00230 uint8_t lowPowerEnable; 00231 uint8_t operatingMode; 00232 }; 00233 00234 struct temperatureSettings 00235 { 00236 // Temperature settings 00237 uint8_t enabled; 00238 }; 00239 00240 struct IMUSettings 00241 { 00242 deviceSettings device; 00243 00244 gyroSettings gyro; 00245 accelSettings accel; 00246 magSettings mag; 00247 00248 temperatureSettings temp; 00249 }; 00250 00251 #endif
Generated on Sun Jul 24 2022 15:36:09 by
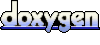