mbed port of tinydtls
Embed:
(wiki syntax)
Show/hide line numbers
state.h
Go to the documentation of this file.
00001 /* dtls -- a very basic DTLS implementation 00002 * 00003 * Copyright (C) 2011--2013 Olaf Bergmann <bergmann@tzi.org> 00004 * 00005 * Permission is hereby granted, free of charge, to any person 00006 * obtaining a copy of this software and associated documentation 00007 * files (the "Software"), to deal in the Software without 00008 * restriction, including without limitation the rights to use, copy, 00009 * modify, merge, publish, distribute, sublicense, and/or sell copies 00010 * of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be 00014 * included in all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00017 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00018 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00019 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS 00020 * BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN 00021 * ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN 00022 * CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00023 * SOFTWARE. 00024 */ 00025 00026 /** 00027 * @file state.h 00028 * @brief state information for DTLS FSM 00029 */ 00030 00031 #ifndef _STATE_H_ 00032 #define _STATE_H_ 00033 00034 #include "config.h" 00035 #include "global.h" 00036 #include "hmac.h" 00037 00038 typedef enum { 00039 DTLS_STATE_INIT = 0, DTLS_STATE_SERVERHELLO, DTLS_STATE_KEYEXCHANGE, 00040 DTLS_STATE_WAIT_FINISHED, DTLS_STATE_FINISHED, 00041 /* client states */ 00042 DTLS_STATE_CLIENTHELLO, DTLS_STATE_WAIT_SERVERHELLODONE, 00043 DTLS_STATE_WAIT_SERVERFINISHED, 00044 00045 DTLS_STATE_CONNECTED, 00046 DTLS_STATE_CLOSING, 00047 DTLS_STATE_CLOSED, 00048 } dtls_state_t; 00049 00050 typedef struct { 00051 uint24 mseq; /**< handshake message sequence number counter */ 00052 00053 /** pending config that is updated during handshake */ 00054 /* FIXME: dtls_security_parameters_t pending_config; */ 00055 00056 /* temporary storage for the final handshake hash */ 00057 dtls_hash_ctx hs_hash; 00058 } dtls_hs_state_t; 00059 00060 #endif /* _STATE_H_ */
Generated on Thu Jul 14 2022 20:00:56 by
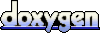