mbed port of tinydtls
Embed:
(wiki syntax)
Show/hide line numbers
prng.h
Go to the documentation of this file.
00001 /* prng.h -- Pseudo Random Numbers 00002 * 00003 * Copyright (C) 2010--2012 Olaf Bergmann <bergmann@tzi.org> 00004 * 00005 * This file is part of the library tinydtls. Please see 00006 * README for terms of use. 00007 */ 00008 00009 /** 00010 * @file prng.h 00011 * @brief Pseudo Random Numbers 00012 */ 00013 00014 #ifndef _DTLS_PRNG_H_ 00015 #define _DTLS_PRNG_H_ 00016 00017 #include "config.h" 00018 00019 /** 00020 * @defgroup prng Pseudo Random Numbers 00021 * @{ 00022 */ 00023 00024 #ifndef WITH_CONTIKI 00025 #include <stdlib.h> 00026 00027 /** 00028 * Fills \p buf with \p len random bytes. This is the default 00029 * implementation for prng(). You might want to change prng() to use 00030 * a better PRNG on your specific platform. 00031 */ 00032 static inline int 00033 dtls_prng_impl(unsigned char *buf, size_t len) { 00034 while (len--) 00035 *buf++ = rand() & 0xFF; 00036 return 1; 00037 } 00038 #else /* WITH_CONTIKI */ 00039 #include <string.h> 00040 00041 #ifdef HAVE_PRNG 00042 extern int contiki_prng_impl(unsigned char *buf, size_t len); 00043 #else 00044 /** 00045 * Fills \p buf with \p len random bytes. This is the default 00046 * implementation for prng(). You might want to change prng() to use 00047 * a better PRNG on your specific platform. 00048 */ 00049 static inline int 00050 contiki_prng_impl(unsigned char *buf, size_t len) { 00051 unsigned short v = random_rand(); 00052 while (len > sizeof(v)) { 00053 memcpy(buf, &v, sizeof(v)); 00054 len -= sizeof(v); 00055 buf += sizeof(v); 00056 v = random_rand(); 00057 } 00058 00059 memcpy(buf, &v, len); 00060 return 1; 00061 } 00062 #endif /* HAVE_PRNG */ 00063 00064 #define prng(Buf,Length) contiki_prng_impl((Buf), (Length)) 00065 #define prng_init(Value) random_init((unsigned short)(Value)) 00066 #endif /* WITH_CONTIKI */ 00067 00068 #ifndef prng 00069 /** 00070 * Fills \p Buf with \p Length bytes of random data. 00071 * 00072 * @hideinitializer 00073 */ 00074 #define prng(Buf,Length) dtls_prng_impl((Buf), (Length)) 00075 #endif 00076 00077 #ifndef prng_init 00078 /** 00079 * Called to set the PRNG seed. You may want to re-define this to 00080 * allow for a better PRNG. 00081 * 00082 * @hideinitializer 00083 */ 00084 #define prng_init(Value) srand((unsigned long)(Value)) 00085 #endif 00086 00087 /** @} */ 00088 00089 #endif /* _DTLS_PRNG_H_ */
Generated on Thu Jul 14 2022 20:00:56 by
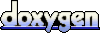