mbed port of tinydtls
Embed:
(wiki syntax)
Show/hide line numbers
peer.c
00001 /* dtls -- a very basic DTLS implementation 00002 * 00003 * Copyright (C) 2011--2013 Olaf Bergmann <bergmann@tzi.org> 00004 * 00005 * Permission is hereby granted, free of charge, to any person 00006 * obtaining a copy of this software and associated documentation 00007 * files (the "Software"), to deal in the Software without 00008 * restriction, including without limitation the rights to use, copy, 00009 * modify, merge, publish, distribute, sublicense, and/or sell copies 00010 * of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be 00014 * included in all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00017 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00018 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00019 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS 00020 * BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN 00021 * ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN 00022 * CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00023 * SOFTWARE. 00024 */ 00025 00026 #define __DEBUG__ 4 00027 00028 #ifndef __MODULE__ 00029 #define __MODULE__ "peer.c" 00030 #endif 00031 00032 #include "peer.h" 00033 #include "debug.h" 00034 #include "dbg.h" 00035 00036 #ifndef NDEBUG 00037 #include <stdio.h> 00038 00039 extern size_t dsrv_print_addr(const session_t *addr, unsigned char *buf, 00040 size_t len); 00041 #endif /* NDEBUG */ 00042 00043 #ifndef WITH_CONTIKI 00044 void peer_init() 00045 { 00046 } 00047 00048 static inline dtls_peer_t * 00049 dtls_malloc_peer() { 00050 return (dtls_peer_t *)malloc(sizeof(dtls_peer_t)); 00051 } 00052 00053 void 00054 dtls_free_peer(dtls_peer_t *peer) { 00055 free(peer); 00056 } 00057 #else /* WITH_CONTIKI */ 00058 PROCESS(dtls_retransmit_process, "DTLS retransmit process"); 00059 00060 #include "memb.h" 00061 MEMB(peer_storage, dtls_peer_t, DTLS_PEER_MAX); 00062 00063 void 00064 peer_init() { 00065 memb_init(&peer_storage); 00066 } 00067 00068 static inline dtls_peer_t * 00069 dtls_malloc_peer() { 00070 return memb_alloc(&peer_storage); 00071 } 00072 00073 void 00074 dtls_free_peer(dtls_peer_t *peer) { 00075 memb_free(&peer_storage, peer); 00076 } 00077 #endif /* WITH_CONTIKI */ 00078 00079 dtls_peer_t * 00080 dtls_new_peer(const session_t *session) { 00081 DBG("Entered dtls_new_peer"); 00082 dtls_peer_t *peer; 00083 00084 peer = dtls_malloc_peer(); 00085 if (peer) { 00086 memset(peer, 0, sizeof(dtls_peer_t)); 00087 memcpy(&peer->session, session, sizeof(session_t)); 00088 00089 #ifndef NDEBUG 00090 if (dtls_get_log_level() >= LOG_DEBUG) { 00091 unsigned char addrbuf[72]; 00092 dsrv_print_addr(session, addrbuf, sizeof(addrbuf)); 00093 DBG("Created peer for: \"%s\"", addrbuf); 00094 } 00095 #endif 00096 /* initially allow the NULL cipher */ 00097 CURRENT_CONFIG(peer)->cipher = TLS_NULL_WITH_NULL_NULL; 00098 00099 /* initialize the handshake hash wrt. the hard-coded DTLS version */ 00100 DBG("DTLSv12: initialize HASH_SHA256"); 00101 /* TLS 1.2: PRF(secret, label, seed) = P_<hash>(secret, label + seed) */ 00102 /* FIXME: we use the default SHA256 here, might need to support other 00103 hash functions as well */ 00104 dtls_hash_init(&peer->hs_state.hs_hash); 00105 } 00106 DBG("New peer created @ %u",peer); 00107 00108 return peer; 00109 } 00110
Generated on Thu Jul 14 2022 20:00:56 by
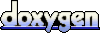