mbed port of tinydtls
Embed:
(wiki syntax)
Show/hide line numbers
netq.h
00001 /* netq.h -- Simple packet queue 00002 * 00003 * Copyright (C) 2010--2012 Olaf Bergmann <bergmann@tzi.org> 00004 * 00005 * This file is part of the library tinyDTLS. Please see the file 00006 * LICENSE for terms of use. 00007 */ 00008 00009 #ifndef _NETQ_H_ 00010 #define _NETQ_H_ 00011 00012 #include "config.h" 00013 #include "global.h" 00014 //#include "dtls.h" 00015 #include "time.h" 00016 #include "peer.h" 00017 00018 /** 00019 * \defgroup netq Network Packet Queue 00020 * The netq utility functions implement an ordered queue of data packets 00021 * to send over the network and can also be used to queue received packets 00022 * from the network. 00023 * @{ 00024 */ 00025 00026 #ifndef NETQ_MAXCNT 00027 #define NETQ_MAXCNT 4 /**< maximum number of elements in netq structure */ 00028 #endif 00029 00030 /** 00031 * Datagrams in the netq_t structure have a fixed maximum size of 00032 * DTLS_MAX_BUF to simplify memory management on constrained nodes. */ 00033 typedef unsigned char netq_packet_t[DTLS_MAX_BUF]; 00034 00035 typedef struct netq_t { 00036 struct netq_t *next; 00037 00038 clock_time_t t; /**< when to send PDU for the next time */ 00039 unsigned char retransmit_cnt; /**< retransmission counter, will be removed when zero */ 00040 unsigned int timeout; /**< randomized timeout value */ 00041 00042 dtls_peer_t *peer; /**< remote address */ 00043 00044 size_t length; /**< actual length of data */ 00045 netq_packet_t data; /**< the datagram to send */ 00046 } netq_t; 00047 00048 /** 00049 * Adds a node to the given queue, ordered by their time-stamp t. 00050 * This function returns @c 0 on error, or non-zero if @p node has 00051 * been added successfully. 00052 * 00053 * @param queue A pointer to the queue head where @p node will be added. 00054 * @param node The new item to add. 00055 * @return @c 0 on error, or non-zero if the new item was added. 00056 */ 00057 int netq_insert_node(netq_t **queue, netq_t *node); 00058 00059 /** Destroys specified node and releases any memory that was allocated 00060 * for the associated datagram. */ 00061 void netq_node_free(netq_t *node); 00062 00063 /** Removes all items from given queue and frees the allocated storage */ 00064 void netq_delete_all(netq_t *queue); 00065 00066 /** Creates a new node suitable for adding to a netq_t queue. */ 00067 netq_t *netq_node_new(); 00068 00069 /** 00070 * Returns a pointer to the first item in given queue or NULL if 00071 * empty. 00072 */ 00073 netq_t *netq_head(netq_t **queue); 00074 00075 /** 00076 * Removes the first item in given queue and returns a pointer to the 00077 * removed element. If queue is empty when netq_pop_first() is called, 00078 * this function returns NULL. 00079 */ 00080 netq_t *netq_pop_first(netq_t **queue); 00081 00082 /**@}*/ 00083 00084 #endif /* _NETQ_H_ */
Generated on Thu Jul 14 2022 20:00:56 by
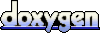