mbed port of tinydtls
Embed:
(wiki syntax)
Show/hide line numbers
hmac.h
00001 /* dtls -- a very basic DTLS implementation 00002 * 00003 * Copyright (C) 2011--2012 Olaf Bergmann <bergmann@tzi.org> 00004 * 00005 * Permission is hereby granted, free of charge, to any person 00006 * obtaining a copy of this software and associated documentation 00007 * files (the "Software"), to deal in the Software without 00008 * restriction, including without limitation the rights to use, copy, 00009 * modify, merge, publish, distribute, sublicense, and/or sell copies 00010 * of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be 00014 * included in all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00017 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00018 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00019 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS 00020 * BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN 00021 * ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN 00022 * CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00023 * SOFTWARE. 00024 */ 00025 00026 #ifndef _HMAC_H_ 00027 #define _HMAC_H_ 00028 00029 #ifdef HAVE_SYS_TYPES_H 00030 #include <sys/types.h> 00031 #endif 00032 00033 #include "global.h" 00034 00035 #ifdef WITH_SHA256 00036 /** Aaron D. Gifford's implementation of SHA256 00037 * see http://www.aarongifford.com/ */ 00038 #include "sha2/sha2.h" 00039 00040 typedef SHA256_CTX dtls_hash_ctx; 00041 typedef dtls_hash_ctx *dtls_hash_t; 00042 #define DTLS_HASH_CTX_SIZE sizeof(SHA256_CTX) 00043 00044 static inline void 00045 dtls_hash_init(dtls_hash_t ctx) { 00046 SHA256_Init((SHA256_CTX *)ctx); 00047 } 00048 00049 static inline void 00050 dtls_hash_update(dtls_hash_t ctx, const unsigned char *input, size_t len) { 00051 SHA256_Update((SHA256_CTX *)ctx, input, len); 00052 } 00053 00054 static inline size_t 00055 dtls_hash_finalize(unsigned char *buf, dtls_hash_t ctx) { 00056 SHA256_Final(buf, (SHA256_CTX *)ctx); 00057 return SHA256_DIGEST_LENGTH; 00058 } 00059 #endif /* WITH_SHA256 */ 00060 00061 /** 00062 * \defgroup HMAC Keyed-Hash Message Authentication Code (HMAC) 00063 * NIST Standard FIPS 198 describes the Keyed-Hash Message Authentication 00064 * Code (HMAC) which is used as hash function for the DTLS PRF. 00065 * @{ 00066 */ 00067 00068 #define DTLS_HMAC_BLOCKSIZE 64 /**< size of hmac blocks */ 00069 #define DTLS_HMAC_DIGEST_SIZE 32 /**< digest size (for SHA-256) */ 00070 #define DTLS_HMAC_MAX 64 /**< max number of bytes in digest */ 00071 00072 /** 00073 * List of known hash functions for use in dtls_hmac_init(). The 00074 * identifiers are the same as the HashAlgorithm defined in 00075 * <a href="http://tools.ietf.org/html/rfc5246#section-7.4.1.4.1" 00076 * >Section 7.4.1.4.1 of RFC 5246</a>. 00077 */ 00078 typedef enum { 00079 HASH_NONE=0, HASH_MD5=1, HASH_SHA1=2, HASH_SHA224=3, 00080 HASH_SHA256=4, HASH_SHA384=5, HASH_SHA512=6 00081 } dtls_hashfunc_t; 00082 00083 /** 00084 * Context for HMAC generation. This object is initialized with 00085 * dtls_hmac_init() and must be passed to dtls_hmac_update() and 00086 * dtls_hmac_finalize(). Once, finalized, the component \c H is 00087 * invalid and must be initialized again with dtls_hmac_init() before 00088 * the structure can be used again. 00089 */ 00090 typedef struct { 00091 unsigned char pad[DTLS_HMAC_BLOCKSIZE]; /**< ipad and opad storage */ 00092 dtls_hash_ctx data; /**< context for hash function */ 00093 } dtls_hmac_context_t; 00094 00095 /** 00096 * Initializes an existing HMAC context. 00097 * 00098 * @param ctx The HMAC context to initialize. 00099 * @param key The secret key. 00100 * @param klen The length of @p key. 00101 */ 00102 void dtls_hmac_init(dtls_hmac_context_t *ctx, const unsigned char *key, size_t klen); 00103 00104 /** 00105 * Allocates a new HMAC context \p ctx with the given secret key. 00106 * This function returns \c 1 if \c ctx has been set correctly, or \c 00107 * 0 or \c -1 otherwise. Note that this function allocates new storage 00108 * that must be released by dtls_hmac_free(). 00109 * 00110 * \param key The secret key. 00111 * \param klen The length of \p key. 00112 * \return A new dtls_hmac_context_t object or @c NULL on error 00113 */ 00114 dtls_hmac_context_t *dtls_hmac_new(const unsigned char *key, size_t klen); 00115 00116 /** 00117 * Releases the storage for @p ctx that has been allocated by 00118 * dtls_hmac_new(). 00119 * 00120 * @param ctx The dtls_hmac_context_t to free. 00121 */ 00122 void dtls_hmac_free(dtls_hmac_context_t *ctx); 00123 00124 /** 00125 * Updates the HMAC context with data from \p input. 00126 * 00127 * \param ctx The HMAC context. 00128 * \param input The input data. 00129 * \param ilen Size of \p input. 00130 */ 00131 void dtls_hmac_update(dtls_hmac_context_t *ctx, 00132 const unsigned char *input, size_t ilen); 00133 00134 /** 00135 * Completes the HMAC generation and writes the result to the given 00136 * output parameter \c result. The buffer must be large enough to hold 00137 * the message digest created by the actual hash function. If in 00138 * doubt, use \c DTLS_HMAC_MAX. The function returns the number of 00139 * bytes written to \c result. 00140 * 00141 * \param ctx The HMAC context. 00142 * \param result Output parameter where the MAC is written to. 00143 * \return Length of the MAC written to \p result. 00144 */ 00145 int dtls_hmac_finalize(dtls_hmac_context_t *ctx, unsigned char *result); 00146 00147 /**@}*/ 00148 00149 #endif /* _HMAC_H_ */
Generated on Thu Jul 14 2022 20:00:56 by
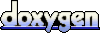