
Shows how to send and receive SMS messages using a Vodafone USB dongle.
Dependencies: VodafoneUSBModem mbed-rtos mbed
main.cpp
00001 // stuff to use DBG debug output 00002 #define __DEBUG__ 4 //Maximum verbosity 00003 #ifndef __MODULE__ 00004 #define __MODULE__ "main.cpp" 00005 #endif 00006 00007 // relevant headers 00008 #include "mbed.h" 00009 #include "VodafoneUSBModem.h" 00010 00011 #define TEST_NUMBER "0000" 00012 #define MAX_SMS_LEN 256 00013 00014 int main() { 00015 // setup debug macro 00016 DBG_INIT(); 00017 DBG_SET_SPEED(115200); 00018 DBG_SET_NEWLINE("\r\n"); 00019 00020 // construct modem object 00021 VodafoneUSBModem modem; 00022 00023 // locals 00024 size_t smCount = 0; 00025 char numBuffer[32], msgBuffer[256]; 00026 00027 // send a wake-up SMS 00028 DBG("Sending test SMS to %s",TEST_NUMBER); 00029 if(modem.sendSM(TEST_NUMBER,"Hello!")!=0) { 00030 DBG("Error sending test SMS!"); 00031 } 00032 00033 // loop forever printing received SMSs 00034 while(1) { 00035 00036 // get SM count 00037 if(modem.getSMCount(&smCount)!=0) { 00038 DBG("Error receiving SMS count!"); 00039 continue; 00040 } 00041 00042 // if SMS in mailbox 00043 if(smCount>0) { 00044 00045 // get SMS and sender 00046 if(modem.getSM(numBuffer,msgBuffer,MAX_SMS_LEN)!=0) { 00047 DBG("Error retrieving SMS from mailbox!"); 00048 continue; 00049 } 00050 00051 // print SMS and sender 00052 DBG("SMS: \"%s\", From: \"%s\"",msgBuffer,numBuffer); 00053 00054 } 00055 00056 // wait 1 second 00057 Thread::wait(1000); 00058 } 00059 }
Generated on Wed Jul 13 2022 23:39:28 by
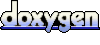