
Example which shows HTTP client use with the USB modem.
Dependencies: HTTPClient VodafoneUSBModem mbed-rtos mbed
Fork of VodafoneUSBModemHTTPClientTest by
main.cpp
00001 // stuff to use DBG debug output 00002 #define __DEBUG__ 4 //Maximum verbosity 00003 #ifndef __MODULE__ 00004 #define __MODULE__ "main.cpp" 00005 #endif 00006 00007 #include "mbed.h" 00008 #include "VodafoneUSBModem.h" 00009 #include "HTTPClient.h" 00010 #include "socket.h" 00011 00012 // connect to socket at ipAddress and port 00013 bool connectToSocket(char *ipAddress, int port, int *sockfd) { 00014 *sockfd = -1; 00015 00016 // create the socket 00017 if((*sockfd=socket(AF_INET,SOCK_STREAM,0))<0) { 00018 INFO("Error opening socket"); 00019 return false; 00020 } 00021 00022 // create the socket address 00023 sockaddr_in serverAddress; 00024 std::memset(&serverAddress, 0, sizeof(struct sockaddr_in)); 00025 serverAddress.sin_addr.s_addr = inet_addr(ipAddress); 00026 serverAddress.sin_family = AF_INET; 00027 serverAddress.sin_port = htons(port); 00028 00029 // do socket connect 00030 if(connect(*sockfd, (const struct sockaddr *)&serverAddress, sizeof(serverAddress))<0) { 00031 close(*sockfd); 00032 INFO("Could not connect"); 00033 return false; 00034 } 00035 return true; 00036 } 00037 00038 int main() { 00039 // setup debug macro 00040 DBG_INIT(); 00041 DBG_SET_SPEED(115200); 00042 DBG_SET_NEWLINE("\r\n"); 00043 INFO("Begin"); 00044 00045 // construct modem object 00046 VodafoneUSBModem modem; 00047 00048 // construc http client object 00049 HTTPClient http; 00050 00051 // locals 00052 char str[512]; 00053 str[0] = 0x00; 00054 int ret = 1; 00055 00056 // connect to the mobile network (get an IP) 00057 ret = modem.connect("internet","web","web"); 00058 if(ret!=0) { 00059 DBG("Error connecting to the Vodafone network."); 00060 return; 00061 } 00062 00063 int sockfd = 0; 00064 if(connectToSocket("109.74.199.96",80,&sockfd)) { 00065 INFO("Connected to socket OK"); 00066 close(sockfd); 00067 } 00068 00069 // do an HTTP GET 00070 INFO("Trying to fetch page..."); 00071 ret = http.get("http://m2mthings.com/test.txt", str, 128,3000); 00072 if(ret==0) { 00073 INFO("Page fetched successfully - read %d characters", strlen(str)); 00074 INFO("Result: \"%s\"", str); 00075 } else { 00076 INFO("Error - ret = %d - HTTP return code = %d", ret, http.getHTTPResponseCode()); 00077 } 00078 00079 // do an HTTP POST 00080 HTTPMap map; 00081 HTTPText text(str, 512); 00082 map.put("Hello", "World"); 00083 map.put("test", "1234"); 00084 INFO("Trying to post data..."); 00085 ret = http.post("http://httpbin.org/post", map, &text); 00086 if(ret==0) { 00087 INFO("Executed POST successfully - read %d characters", strlen(str)); 00088 INFO("Result: %s\n", str); 00089 } else { 00090 INFO("Error - ret = %d - HTTP return code = %d", ret, http.getHTTPResponseCode()); 00091 } 00092 00093 // disconnect from the mobile network 00094 modem.disconnect(); 00095 00096 // flash led 1 forever 00097 DigitalOut l1(LED1); 00098 while(1) { 00099 l1 = !l1; 00100 Thread::wait(1000); 00101 } 00102 }
Generated on Fri Jul 15 2022 12:01:20 by
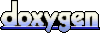