cyassl re-port with cellular comms, PSK test
Dependencies: VodafoneUSBModem_bleedingedge2 mbed-rtos mbed-src
test.h
00001 /* test.h */ 00002 00003 #ifndef CyaSSL_TEST_H 00004 #define CyaSSL_TEST_H 00005 00006 #include <stdio.h> 00007 #include <stdlib.h> 00008 #include <assert.h> 00009 #include <ctype.h> 00010 #include <cyassl/ssl.h> 00011 #include <cyassl/ctaocrypt/types.h> 00012 00013 #ifdef USE_WINDOWS_API 00014 #include <winsock2.h> 00015 #include <process.h> 00016 #ifdef TEST_IPV6 /* don't require newer SDK for IPV4 */ 00017 #include <ws2tcpip.h> 00018 #include <wspiapi.h> 00019 #endif 00020 #define SOCKET_T unsigned int 00021 #else 00022 #include <string.h> 00023 #include <unistd.h> 00024 #include <netdb.h> 00025 #include <netinet/in.h> 00026 #include <netinet/tcp.h> 00027 #include <arpa/inet.h> 00028 #include <sys/ioctl.h> 00029 #include <sys/time.h> 00030 #include <sys/types.h> 00031 #include <sys/socket.h> 00032 #include <pthread.h> 00033 #include <fcntl.h> 00034 #ifdef TEST_IPV6 00035 #include <netdb.h> 00036 #endif 00037 #define SOCKET_T int 00038 #ifndef SO_NOSIGPIPE 00039 #include <signal.h> /* ignore SIGPIPE */ 00040 #endif 00041 #endif /* USE_WINDOWS_API */ 00042 00043 #ifdef HAVE_CAVIUM 00044 #include "cavium_sysdep.h" 00045 #include "cavium_common.h" 00046 #include "cavium_ioctl.h" 00047 #endif 00048 00049 #ifdef _MSC_VER 00050 /* disable conversion warning */ 00051 /* 4996 warning to use MS extensions e.g., strcpy_s instead of strncpy */ 00052 #pragma warning(disable:4244 4996) 00053 #endif 00054 00055 00056 #if defined(__MACH__) || defined(USE_WINDOWS_API) 00057 #ifndef _SOCKLEN_T 00058 typedef int socklen_t; 00059 #endif 00060 #endif 00061 00062 00063 /* HPUX doesn't use socklent_t for third parameter to accept */ 00064 #if !defined(__hpux__) 00065 typedef socklen_t* ACCEPT_THIRD_T; 00066 #else 00067 typedef int* ACCEPT_THIRD_T; 00068 #endif 00069 00070 00071 #ifdef USE_WINDOWS_API 00072 #define CloseSocket(s) closesocket(s) 00073 #define StartTCP() { WSADATA wsd; WSAStartup(0x0002, &wsd); } 00074 #else 00075 #define CloseSocket(s) close(s) 00076 #define StartTCP() 00077 #endif 00078 00079 00080 #ifdef SINGLE_THREADED 00081 typedef unsigned int THREAD_RETURN; 00082 typedef void* THREAD_TYPE; 00083 #define CYASSL_THREAD 00084 #else 00085 #ifdef _POSIX_THREADS 00086 typedef void* THREAD_RETURN; 00087 typedef pthread_t THREAD_TYPE; 00088 #define CYASSL_THREAD 00089 #define INFINITE -1 00090 #define WAIT_OBJECT_0 0L 00091 #else 00092 typedef unsigned int THREAD_RETURN; 00093 typedef HANDLE THREAD_TYPE; 00094 #define CYASSL_THREAD __stdcall 00095 #endif 00096 #endif 00097 00098 00099 #ifdef TEST_IPV6 00100 typedef struct sockaddr_in6 SOCKADDR_IN_T; 00101 #define AF_INET_V AF_INET6 00102 #else 00103 typedef struct sockaddr_in SOCKADDR_IN_T; 00104 #define AF_INET_V AF_INET 00105 #endif 00106 00107 00108 #define SERVER_DEFAULT_VERSION 3 00109 #define CLIENT_DEFAULT_VERSION 3 00110 00111 /* all certs relative to CyaSSL home directory now */ 00112 #define caCert "./certs/ca-cert.pem" 00113 #define eccCert "./certs/server-ecc.pem" 00114 #define eccKey "./certs/ecc-key.pem" 00115 #define svrCert "./certs/server-cert.pem" 00116 #define svrKey "./certs/server-key.pem" 00117 #define cliCert "./certs/client-cert.pem" 00118 #define cliKey "./certs/client-key.pem" 00119 #define ntruCert "./certs/ntru-cert.pem" 00120 #define ntruKey "./certs/ntru-key.raw" 00121 #define dhParam "./certs/dh2048.pem" 00122 #define cliEccKey "./certs/ecc-client-key.pem" 00123 #define cliEccCert "./certs/client-ecc-cert.pem" 00124 #define crlPemDir "./certs/crl" 00125 00126 typedef struct tcp_ready { 00127 int ready; /* predicate */ 00128 #ifdef _POSIX_THREADS 00129 pthread_mutex_t mutex; 00130 pthread_cond_t cond; 00131 #endif 00132 } tcp_ready; 00133 00134 00135 void InitTcpReady(tcp_ready*); 00136 void FreeTcpReady(tcp_ready*); 00137 00138 00139 typedef struct func_args { 00140 int argc; 00141 char** argv; 00142 int return_code; 00143 tcp_ready* signal; 00144 } func_args; 00145 00146 void wait_tcp_ready(func_args*); 00147 00148 typedef THREAD_RETURN CYASSL_THREAD THREAD_FUNC(void*); 00149 00150 void start_thread(THREAD_FUNC, func_args*, THREAD_TYPE*); 00151 void join_thread(THREAD_TYPE); 00152 00153 /* yaSSL */ 00154 static const char* const yasslIP = "127.0.0.1"; 00155 static const word16 yasslPort = 11111; 00156 00157 00158 static INLINE void err_sys(const char* msg) 00159 { 00160 printf("yassl error: %s\n", msg); 00161 if (msg) 00162 exit(EXIT_FAILURE); 00163 } 00164 00165 00166 #define MY_EX_USAGE 2 00167 00168 extern int myoptind; 00169 extern char* myoptarg; 00170 00171 static INLINE int mygetopt(int argc, char** argv, const char* optstring) 00172 { 00173 static char* next = NULL; 00174 00175 char c; 00176 char* cp; 00177 00178 if (myoptind == 0) 00179 next = NULL; /* we're starting new/over */ 00180 00181 if (next == NULL || *next == '\0') { 00182 if (myoptind == 0) 00183 myoptind++; 00184 00185 if (myoptind >= argc || argv[myoptind][0] != '-' || 00186 argv[myoptind][1] == '\0') { 00187 myoptarg = NULL; 00188 if (myoptind < argc) 00189 myoptarg = argv[myoptind]; 00190 00191 return -1; 00192 } 00193 00194 if (strcmp(argv[myoptind], "--") == 0) { 00195 myoptind++; 00196 myoptarg = NULL; 00197 00198 if (myoptind < argc) 00199 myoptarg = argv[myoptind]; 00200 00201 return -1; 00202 } 00203 00204 next = argv[myoptind]; 00205 next++; /* skip - */ 00206 myoptind++; 00207 } 00208 00209 c = *next++; 00210 /* The C++ strchr can return a different value */ 00211 cp = (char*)strchr(optstring, c); 00212 00213 if (cp == NULL || c == ':') 00214 return '?'; 00215 00216 cp++; 00217 00218 if (*cp == ':') { 00219 if (*next != '\0') { 00220 myoptarg = next; 00221 next = NULL; 00222 } 00223 else if (myoptind < argc) { 00224 myoptarg = argv[myoptind]; 00225 myoptind++; 00226 } 00227 else 00228 return '?'; 00229 } 00230 00231 return c; 00232 } 00233 00234 00235 #ifdef OPENSSL_EXTRA 00236 00237 static INLINE int PasswordCallBack(char* passwd, int sz, int rw, void* userdata) 00238 { 00239 (void)rw; 00240 (void)userdata; 00241 strncpy(passwd, "yassl123", sz); 00242 return 8; 00243 } 00244 00245 #endif 00246 00247 00248 static INLINE void showPeer(CYASSL* ssl) 00249 { 00250 #ifdef OPENSSL_EXTRA 00251 00252 CYASSL_CIPHER* cipher; 00253 CYASSL_X509* peer = CyaSSL_get_peer_certificate(ssl); 00254 if (peer) { 00255 char* altName; 00256 char* issuer = CyaSSL_X509_NAME_oneline( 00257 CyaSSL_X509_get_issuer_name(peer), 0, 0); 00258 char* subject = CyaSSL_X509_NAME_oneline( 00259 CyaSSL_X509_get_subject_name(peer), 0, 0); 00260 byte serial[32]; 00261 int ret; 00262 int sz = sizeof(serial); 00263 00264 printf("peer's cert info:\n issuer : %s\n subject: %s\n", issuer, 00265 subject); 00266 00267 while ( (altName = CyaSSL_X509_get_next_altname(peer)) ) 00268 printf(" altname = %s\n", altName); 00269 00270 ret = CyaSSL_X509_get_serial_number(peer, serial, &sz); 00271 if (ret == 0) { 00272 int i; 00273 int strLen; 00274 char serialMsg[80]; 00275 00276 /* testsuite has multiple threads writing to stdout, get output 00277 message ready to write once */ 00278 strLen = sprintf(serialMsg, " serial number"); 00279 for (i = 0; i < sz; i++) 00280 sprintf(serialMsg + strLen + (i*3), ":%02x ", serial[i]); 00281 printf("%s\n", serialMsg); 00282 } 00283 00284 XFREE(subject, 0, DYNAMIC_TYPE_OPENSSL); 00285 XFREE(issuer, 0, DYNAMIC_TYPE_OPENSSL); 00286 } 00287 else 00288 printf("peer has no cert!\n"); 00289 printf("SSL version is %s\n", CyaSSL_get_version(ssl)); 00290 00291 cipher = CyaSSL_get_current_cipher(ssl); 00292 printf("SSL cipher suite is %s\n", CyaSSL_CIPHER_get_name(cipher)); 00293 #endif 00294 00295 #if defined(SESSION_CERTS) && defined(SHOW_CERTS) 00296 { 00297 CYASSL_X509_CHAIN* chain = CyaSSL_get_peer_chain(ssl); 00298 int count = CyaSSL_get_chain_count(chain); 00299 int i; 00300 00301 for (i = 0; i < count; i++) { 00302 int length; 00303 unsigned char buffer[3072]; 00304 00305 CyaSSL_get_chain_cert_pem(chain,i,buffer, sizeof(buffer), &length); 00306 buffer[length] = 0; 00307 printf("cert %d has length %d data = \n%s\n", i, length, buffer); 00308 } 00309 } 00310 #endif 00311 (void)ssl; 00312 } 00313 00314 00315 static INLINE void build_addr(SOCKADDR_IN_T* addr, 00316 const char* peer, word16 port) 00317 { 00318 #ifndef TEST_IPV6 00319 const char* host = peer; 00320 00321 /* peer could be in human readable form */ 00322 if (peer != INADDR_ANY && isalpha(peer[0])) { 00323 struct hostent* entry = gethostbyname(peer); 00324 00325 if (entry) { 00326 struct sockaddr_in tmp; 00327 memset(&tmp, 0, sizeof(struct sockaddr_in)); 00328 memcpy(&tmp.sin_addr.s_addr, entry->h_addr_list[0], 00329 entry->h_length); 00330 host = inet_ntoa(tmp.sin_addr); 00331 } 00332 else 00333 err_sys("no entry for host"); 00334 } 00335 #endif 00336 00337 memset(addr, 0, sizeof(SOCKADDR_IN_T)); 00338 00339 #ifndef TEST_IPV6 00340 addr->sin_family = AF_INET_V; 00341 addr->sin_port = htons(port); 00342 if (host == INADDR_ANY) 00343 addr->sin_addr.s_addr = INADDR_ANY; 00344 else 00345 addr->sin_addr.s_addr = inet_addr(host); 00346 #else 00347 (void)peer; 00348 addr->sin6_family = AF_INET_V; 00349 addr->sin6_port = htons(port); 00350 addr->sin6_addr = in6addr_loopback; 00351 #endif 00352 } 00353 00354 00355 static INLINE void tcp_socket(SOCKET_T* sockfd, int udp) 00356 { 00357 if (udp) 00358 *sockfd = socket(AF_INET_V, SOCK_DGRAM, 0); 00359 else 00360 *sockfd = socket(AF_INET_V, SOCK_STREAM, 0); 00361 00362 #ifndef USE_WINDOWS_API 00363 #ifdef SO_NOSIGPIPE 00364 { 00365 int on = 1; 00366 socklen_t len = sizeof(on); 00367 int res = setsockopt(*sockfd, SOL_SOCKET, SO_NOSIGPIPE, &on, len); 00368 if (res < 0) 00369 err_sys("setsockopt SO_NOSIGPIPE failed\n"); 00370 } 00371 #else /* no S_NOSIGPIPE */ 00372 signal(SIGPIPE, SIG_IGN); 00373 #endif /* S_NOSIGPIPE */ 00374 00375 #if defined(TCP_NODELAY) 00376 if (!udp) 00377 { 00378 int on = 1; 00379 socklen_t len = sizeof(on); 00380 int res = setsockopt(*sockfd, IPPROTO_TCP, TCP_NODELAY, &on, len); 00381 if (res < 0) 00382 err_sys("setsockopt TCP_NODELAY failed\n"); 00383 } 00384 #endif 00385 #endif /* USE_WINDOWS_API */ 00386 } 00387 00388 00389 static INLINE void tcp_connect(SOCKET_T* sockfd, const char* ip, word16 port, 00390 int udp) 00391 { 00392 SOCKADDR_IN_T addr; 00393 build_addr(&addr, ip, port); 00394 tcp_socket(sockfd, udp); 00395 00396 if (!udp) { 00397 if (connect(*sockfd, (const struct sockaddr*)&addr, sizeof(addr)) != 0) 00398 err_sys("tcp connect failed"); 00399 } 00400 } 00401 00402 00403 static INLINE void udp_connect(SOCKET_T* sockfd, void* addr, int addrSz) 00404 { 00405 if (connect(*sockfd, (const struct sockaddr*)addr, addrSz) != 0) 00406 err_sys("tcp connect failed"); 00407 } 00408 00409 00410 enum { 00411 TEST_SELECT_FAIL, 00412 TEST_TIMEOUT, 00413 TEST_RECV_READY, 00414 TEST_ERROR_READY 00415 }; 00416 00417 static INLINE int tcp_select(SOCKET_T socketfd, unsigned int to_sec) 00418 { 00419 fd_set recvfds, errfds; 00420 SOCKET_T nfds = socketfd + 1; 00421 struct timeval timeout = {to_sec, 0}; 00422 int result; 00423 00424 FD_ZERO(&recvfds); 00425 FD_SET(socketfd, &recvfds); 00426 FD_ZERO(&errfds); 00427 FD_SET(socketfd, &errfds); 00428 00429 result = select(nfds, &recvfds, NULL, &errfds, &timeout); 00430 00431 if (result == 0) 00432 return TEST_TIMEOUT; 00433 else if (result > 0) { 00434 if (FD_ISSET(socketfd, &recvfds)) 00435 return TEST_RECV_READY; 00436 else if(FD_ISSET(socketfd, &errfds)) 00437 return TEST_ERROR_READY; 00438 } 00439 00440 return TEST_SELECT_FAIL; 00441 } 00442 00443 00444 static INLINE void tcp_listen(SOCKET_T* sockfd, int port, int useAnyAddr, 00445 int udp) 00446 { 00447 SOCKADDR_IN_T addr; 00448 00449 /* don't use INADDR_ANY by default, firewall may block, make user switch 00450 on */ 00451 build_addr(&addr, (useAnyAddr ? INADDR_ANY : yasslIP), port); 00452 tcp_socket(sockfd, udp); 00453 00454 #ifndef USE_WINDOWS_API 00455 { 00456 int on = 1; 00457 socklen_t len = sizeof(on); 00458 setsockopt(*sockfd, SOL_SOCKET, SO_REUSEADDR, &on, len); 00459 } 00460 #endif 00461 00462 if (bind(*sockfd, (const struct sockaddr*)&addr, sizeof(addr)) != 0) 00463 err_sys("tcp bind failed"); 00464 if (!udp) { 00465 if (listen(*sockfd, 5) != 0) 00466 err_sys("tcp listen failed"); 00467 } 00468 } 00469 00470 00471 static INLINE int udp_read_connect(SOCKET_T sockfd) 00472 { 00473 SOCKADDR_IN_T cliaddr; 00474 byte b[1500]; 00475 int n; 00476 socklen_t len = sizeof(cliaddr); 00477 00478 n = (int)recvfrom(sockfd, (char*)b, sizeof(b), MSG_PEEK, 00479 (struct sockaddr*)&cliaddr, &len); 00480 if (n > 0) { 00481 if (connect(sockfd, (const struct sockaddr*)&cliaddr, 00482 sizeof(cliaddr)) != 0) 00483 err_sys("udp connect failed"); 00484 } 00485 else 00486 err_sys("recvfrom failed"); 00487 00488 return sockfd; 00489 } 00490 00491 static INLINE void udp_accept(SOCKET_T* sockfd, int* clientfd, int useAnyAddr, 00492 func_args* args) 00493 { 00494 SOCKADDR_IN_T addr; 00495 00496 (void)args; 00497 build_addr(&addr, (useAnyAddr ? INADDR_ANY : yasslIP), yasslPort); 00498 tcp_socket(sockfd, 1); 00499 00500 00501 #ifndef USE_WINDOWS_API 00502 { 00503 int on = 1; 00504 socklen_t len = sizeof(on); 00505 setsockopt(*sockfd, SOL_SOCKET, SO_REUSEADDR, &on, len); 00506 } 00507 #endif 00508 00509 if (bind(*sockfd, (const struct sockaddr*)&addr, sizeof(addr)) != 0) 00510 err_sys("tcp bind failed"); 00511 00512 #if defined(_POSIX_THREADS) && defined(NO_MAIN_DRIVER) 00513 /* signal ready to accept data */ 00514 { 00515 tcp_ready* ready = args->signal; 00516 pthread_mutex_lock(&ready->mutex); 00517 ready->ready = 1; 00518 pthread_cond_signal(&ready->cond); 00519 pthread_mutex_unlock(&ready->mutex); 00520 } 00521 #endif 00522 00523 *clientfd = udp_read_connect(*sockfd); 00524 } 00525 00526 static INLINE void tcp_accept(SOCKET_T* sockfd, int* clientfd, func_args* args, 00527 int port, int useAnyAddr, int udp) 00528 { 00529 SOCKADDR_IN_T client; 00530 socklen_t client_len = sizeof(client); 00531 00532 if (udp) { 00533 udp_accept(sockfd, clientfd, useAnyAddr, args); 00534 return; 00535 } 00536 00537 tcp_listen(sockfd, port, useAnyAddr, udp); 00538 00539 #if defined(_POSIX_THREADS) && defined(NO_MAIN_DRIVER) 00540 /* signal ready to tcp_accept */ 00541 { 00542 tcp_ready* ready = args->signal; 00543 pthread_mutex_lock(&ready->mutex); 00544 ready->ready = 1; 00545 pthread_cond_signal(&ready->cond); 00546 pthread_mutex_unlock(&ready->mutex); 00547 } 00548 #endif 00549 00550 *clientfd = accept(*sockfd, (struct sockaddr*)&client, 00551 (ACCEPT_THIRD_T)&client_len); 00552 if (*clientfd == -1) 00553 err_sys("tcp accept failed"); 00554 } 00555 00556 00557 static INLINE void tcp_set_nonblocking(SOCKET_T* sockfd) 00558 { 00559 #ifdef USE_WINDOWS_API 00560 unsigned long blocking = 1; 00561 int ret = ioctlsocket(*sockfd, FIONBIO, &blocking); 00562 #else 00563 int flags = fcntl(*sockfd, F_GETFL, 0); 00564 fcntl(*sockfd, F_SETFL, flags | O_NONBLOCK); 00565 #endif 00566 } 00567 00568 00569 #ifndef NO_PSK 00570 00571 static INLINE unsigned int my_psk_client_cb(CYASSL* ssl, const char* hint, 00572 char* identity, unsigned int id_max_len, unsigned char* key, 00573 unsigned int key_max_len) 00574 { 00575 (void)ssl; 00576 (void)hint; 00577 (void)key_max_len; 00578 00579 /* identity is OpenSSL testing default for openssl s_client, keep same */ 00580 strncpy(identity, "Client_identity", id_max_len); 00581 00582 00583 /* test key in hex is 0x1a2b3c4d , in decimal 439,041,101 , we're using 00584 unsigned binary */ 00585 key[0] = 26; 00586 key[1] = 43; 00587 key[2] = 60; 00588 key[3] = 77; 00589 00590 return 4; /* length of key in octets or 0 for error */ 00591 } 00592 00593 00594 static INLINE unsigned int my_psk_server_cb(CYASSL* ssl, const char* identity, 00595 unsigned char* key, unsigned int key_max_len) 00596 { 00597 (void)ssl; 00598 (void)key_max_len; 00599 00600 /* identity is OpenSSL testing default for openssl s_client, keep same */ 00601 if (strncmp(identity, "Client_identity", 15) != 0) 00602 return 0; 00603 00604 /* test key in hex is 0x1a2b3c4d , in decimal 439,041,101 , we're using 00605 unsigned binary */ 00606 key[0] = 26; 00607 key[1] = 43; 00608 key[2] = 60; 00609 key[3] = 77; 00610 00611 return 4; /* length of key in octets or 0 for error */ 00612 } 00613 00614 #endif /* NO_PSK */ 00615 00616 00617 #ifdef USE_WINDOWS_API 00618 00619 #define WIN32_LEAN_AND_MEAN 00620 #include <windows.h> 00621 00622 static INLINE double current_time() 00623 { 00624 static int init = 0; 00625 static LARGE_INTEGER freq; 00626 00627 LARGE_INTEGER count; 00628 00629 if (!init) { 00630 QueryPerformanceFrequency(&freq); 00631 init = 1; 00632 } 00633 00634 QueryPerformanceCounter(&count); 00635 00636 return (double)count.QuadPart / freq.QuadPart; 00637 } 00638 00639 #else 00640 00641 #include <sys/time.h> 00642 00643 static INLINE double current_time(void) 00644 { 00645 struct timeval tv; 00646 gettimeofday(&tv, 0); 00647 00648 return (double)tv.tv_sec + (double)tv.tv_usec / 1000000; 00649 } 00650 00651 #endif /* USE_WINDOWS_API */ 00652 00653 00654 #if defined(NO_FILESYSTEM) && !defined(NO_CERTS) 00655 00656 enum { 00657 CYASSL_CA = 1, 00658 CYASSL_CERT = 2, 00659 CYASSL_KEY = 3 00660 }; 00661 00662 static INLINE void load_buffer(CYASSL_CTX* ctx, const char* fname, int type) 00663 { 00664 /* test buffer load */ 00665 long sz = 0; 00666 byte buff[10000]; 00667 FILE* file = fopen(fname, "rb"); 00668 00669 if (!file) 00670 err_sys("can't open file for buffer load " 00671 "Please run from CyaSSL home directory if not"); 00672 fseek(file, 0, SEEK_END); 00673 sz = ftell(file); 00674 rewind(file); 00675 fread(buff, sizeof(buff), 1, file); 00676 00677 if (type == CYASSL_CA) { 00678 if (CyaSSL_CTX_load_verify_buffer(ctx, buff, sz, SSL_FILETYPE_PEM) 00679 != SSL_SUCCESS) 00680 err_sys("can't load buffer ca file"); 00681 } 00682 else if (type == CYASSL_CERT) { 00683 if (CyaSSL_CTX_use_certificate_buffer(ctx, buff, sz, 00684 SSL_FILETYPE_PEM) != SSL_SUCCESS) 00685 err_sys("can't load buffer cert file"); 00686 } 00687 else if (type == CYASSL_KEY) { 00688 if (CyaSSL_CTX_use_PrivateKey_buffer(ctx, buff, sz, 00689 SSL_FILETYPE_PEM) != SSL_SUCCESS) 00690 err_sys("can't load buffer key file"); 00691 } 00692 } 00693 00694 #endif /* NO_FILESYSTEM */ 00695 00696 #ifdef VERIFY_CALLBACK 00697 00698 static INLINE int myVerify(int preverify, CYASSL_X509_STORE_CTX* store) 00699 { 00700 char buffer[80]; 00701 00702 #ifdef OPENSSL_EXTRA 00703 CYASSL_X509* peer; 00704 #endif 00705 00706 printf("In verification callback, error = %d, %s\n", store->error, 00707 CyaSSL_ERR_error_string(store->error, buffer)); 00708 #ifdef OPENSSL_EXTRA 00709 peer = store->current_cert; 00710 if (peer) { 00711 char* issuer = CyaSSL_X509_NAME_oneline( 00712 CyaSSL_X509_get_issuer_name(peer), 0, 0); 00713 char* subject = CyaSSL_X509_NAME_oneline( 00714 CyaSSL_X509_get_subject_name(peer), 0, 0); 00715 printf("peer's cert info:\n issuer : %s\n subject: %s\n", issuer, 00716 subject); 00717 XFREE(subject, 0, DYNAMIC_TYPE_OPENSSL); 00718 XFREE(issuer, 0, DYNAMIC_TYPE_OPENSSL); 00719 } 00720 else 00721 printf("peer has no cert!\n"); 00722 #endif 00723 printf("Subject's domain name is %s\n", store->domain); 00724 00725 printf("Allowing to continue anyway (shouldn't do this, EVER!!!)\n"); 00726 return 1; 00727 } 00728 00729 #endif /* VERIFY_CALLBACK */ 00730 00731 00732 #ifdef HAVE_CRL 00733 00734 static INLINE void CRL_CallBack(const char* url) 00735 { 00736 printf("CRL callback url = %s\n", url); 00737 } 00738 00739 #endif 00740 00741 00742 #ifndef NO_CERTS 00743 00744 static INLINE void CaCb(unsigned char* der, int sz, int type) 00745 { 00746 (void)der; 00747 printf("Got CA cache add callback, derSz = %d, type = %d\n", sz, type); 00748 } 00749 00750 00751 static INLINE void SetDH(CYASSL* ssl) 00752 { 00753 /* dh1024 p */ 00754 static unsigned char p[] = 00755 { 00756 0xE6, 0x96, 0x9D, 0x3D, 0x49, 0x5B, 0xE3, 0x2C, 0x7C, 0xF1, 0x80, 0xC3, 00757 0xBD, 0xD4, 0x79, 0x8E, 0x91, 0xB7, 0x81, 0x82, 0x51, 0xBB, 0x05, 0x5E, 00758 0x2A, 0x20, 0x64, 0x90, 0x4A, 0x79, 0xA7, 0x70, 0xFA, 0x15, 0xA2, 0x59, 00759 0xCB, 0xD5, 0x23, 0xA6, 0xA6, 0xEF, 0x09, 0xC4, 0x30, 0x48, 0xD5, 0xA2, 00760 0x2F, 0x97, 0x1F, 0x3C, 0x20, 0x12, 0x9B, 0x48, 0x00, 0x0E, 0x6E, 0xDD, 00761 0x06, 0x1C, 0xBC, 0x05, 0x3E, 0x37, 0x1D, 0x79, 0x4E, 0x53, 0x27, 0xDF, 00762 0x61, 0x1E, 0xBB, 0xBE, 0x1B, 0xAC, 0x9B, 0x5C, 0x60, 0x44, 0xCF, 0x02, 00763 0x3D, 0x76, 0xE0, 0x5E, 0xEA, 0x9B, 0xAD, 0x99, 0x1B, 0x13, 0xA6, 0x3C, 00764 0x97, 0x4E, 0x9E, 0xF1, 0x83, 0x9E, 0xB5, 0xDB, 0x12, 0x51, 0x36, 0xF7, 00765 0x26, 0x2E, 0x56, 0xA8, 0x87, 0x15, 0x38, 0xDF, 0xD8, 0x23, 0xC6, 0x50, 00766 0x50, 0x85, 0xE2, 0x1F, 0x0D, 0xD5, 0xC8, 0x6B, 00767 }; 00768 00769 /* dh1024 g */ 00770 static unsigned char g[] = 00771 { 00772 0x02, 00773 }; 00774 00775 CyaSSL_SetTmpDH(ssl, p, sizeof(p), g, sizeof(g)); 00776 } 00777 00778 static INLINE void SetDHCtx(CYASSL_CTX* ctx) 00779 { 00780 /* dh1024 p */ 00781 static unsigned char p[] = 00782 { 00783 0xE6, 0x96, 0x9D, 0x3D, 0x49, 0x5B, 0xE3, 0x2C, 0x7C, 0xF1, 0x80, 0xC3, 00784 0xBD, 0xD4, 0x79, 0x8E, 0x91, 0xB7, 0x81, 0x82, 0x51, 0xBB, 0x05, 0x5E, 00785 0x2A, 0x20, 0x64, 0x90, 0x4A, 0x79, 0xA7, 0x70, 0xFA, 0x15, 0xA2, 0x59, 00786 0xCB, 0xD5, 0x23, 0xA6, 0xA6, 0xEF, 0x09, 0xC4, 0x30, 0x48, 0xD5, 0xA2, 00787 0x2F, 0x97, 0x1F, 0x3C, 0x20, 0x12, 0x9B, 0x48, 0x00, 0x0E, 0x6E, 0xDD, 00788 0x06, 0x1C, 0xBC, 0x05, 0x3E, 0x37, 0x1D, 0x79, 0x4E, 0x53, 0x27, 0xDF, 00789 0x61, 0x1E, 0xBB, 0xBE, 0x1B, 0xAC, 0x9B, 0x5C, 0x60, 0x44, 0xCF, 0x02, 00790 0x3D, 0x76, 0xE0, 0x5E, 0xEA, 0x9B, 0xAD, 0x99, 0x1B, 0x13, 0xA6, 0x3C, 00791 0x97, 0x4E, 0x9E, 0xF1, 0x83, 0x9E, 0xB5, 0xDB, 0x12, 0x51, 0x36, 0xF7, 00792 0x26, 0x2E, 0x56, 0xA8, 0x87, 0x15, 0x38, 0xDF, 0xD8, 0x23, 0xC6, 0x50, 00793 0x50, 0x85, 0xE2, 0x1F, 0x0D, 0xD5, 0xC8, 0x6B, 00794 }; 00795 00796 /* dh1024 g */ 00797 static unsigned char g[] = 00798 { 00799 0x02, 00800 }; 00801 00802 CyaSSL_CTX_SetTmpDH(ctx, p, sizeof(p), g, sizeof(g)); 00803 } 00804 00805 #endif /* !NO_CERTS */ 00806 00807 #ifdef HAVE_CAVIUM 00808 00809 static INLINE int OpenNitroxDevice(int dma_mode,int dev_id) 00810 { 00811 Csp1CoreAssignment core_assign; 00812 Uint32 device; 00813 00814 if (CspInitialize(CAVIUM_DIRECT,CAVIUM_DEV_ID)) 00815 return -1; 00816 if (Csp1GetDevType(&device)) 00817 return -1; 00818 if (device != NPX_DEVICE) { 00819 if (ioctl(gpkpdev_hdlr[CAVIUM_DEV_ID], IOCTL_CSP1_GET_CORE_ASSIGNMENT, 00820 (Uint32 *)&core_assign)!= 0) 00821 return -1; 00822 } 00823 CspShutdown(CAVIUM_DEV_ID); 00824 00825 return CspInitialize(dma_mode, dev_id); 00826 } 00827 00828 #endif /* HAVE_CAVIUM */ 00829 00830 00831 #ifdef USE_WINDOWS_API 00832 00833 /* do back x number of directories */ 00834 static INLINE void ChangeDirBack(int x) 00835 { 00836 char path[MAX_PATH]; 00837 00838 if (x == 1) 00839 strncpy(path, "..\\", MAX_PATH); 00840 else if (x == 2) 00841 strncpy(path, "..\\..\\", MAX_PATH); 00842 else if (x == 3) 00843 strncpy(path, "..\\..\\..\\", MAX_PATH); 00844 else if (x == 4) 00845 strncpy(path, "..\\..\\..\\..\\", MAX_PATH); 00846 else 00847 strncpy(path, ".\\", MAX_PATH); 00848 00849 SetCurrentDirectoryA(path); 00850 } 00851 00852 /* does current dir contain str */ 00853 static INLINE int CurrentDir(const char* str) 00854 { 00855 char path[MAX_PATH]; 00856 char* baseName; 00857 00858 GetCurrentDirectoryA(sizeof(path), path); 00859 00860 baseName = strrchr(path, '\\'); 00861 if (baseName) 00862 baseName++; 00863 else 00864 baseName = path; 00865 00866 if (strstr(baseName, str)) 00867 return 1; 00868 00869 return 0; 00870 } 00871 00872 #else 00873 00874 #ifndef MAX_PATH 00875 #define MAX_PATH 256 00876 #endif 00877 00878 /* do back x number of directories */ 00879 static INLINE void ChangeDirBack(int x) 00880 { 00881 char path[MAX_PATH]; 00882 00883 if (x == 1) 00884 strncpy(path, "../", MAX_PATH); 00885 else if (x == 2) 00886 strncpy(path, "../../", MAX_PATH); 00887 else if (x == 3) 00888 strncpy(path, "../../../", MAX_PATH); 00889 else if (x == 4) 00890 strncpy(path, "../../../../", MAX_PATH); 00891 else 00892 strncpy(path, "./", MAX_PATH); 00893 00894 if (chdir(path) < 0) 00895 printf("chdir to %s failed\n", path); 00896 } 00897 00898 /* does current dir contain str */ 00899 static INLINE int CurrentDir(const char* str) 00900 { 00901 char path[MAX_PATH]; 00902 char* baseName; 00903 00904 if (getcwd(path, sizeof(path)) == NULL) { 00905 printf("no current dir?\n"); 00906 return 0; 00907 } 00908 00909 baseName = strrchr(path, '/'); 00910 if (baseName) 00911 baseName++; 00912 else 00913 baseName = path; 00914 00915 if (strstr(baseName, str)) 00916 return 1; 00917 00918 return 0; 00919 } 00920 00921 #endif /* USE_WINDOWS_API */ 00922 00923 #endif /* CyaSSL_TEST_H */ 00924
Generated on Thu Jul 14 2022 00:25:24 by
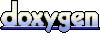