cyassl re-port with cellular comms, PSK test
Dependencies: VodafoneUSBModem_bleedingedge2 mbed-rtos mbed-src
sha.c
00001 /* sha.c 00002 * 00003 * Copyright (C) 2006-2012 Sawtooth Consulting Ltd. 00004 * 00005 * This file is part of CyaSSL. 00006 * 00007 * CyaSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * CyaSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA 00020 */ 00021 00022 #ifdef HAVE_CONFIG_H 00023 #include <config.h> 00024 #endif 00025 00026 #include <cyassl/ctaocrypt/sha.h> 00027 #ifdef NO_INLINE 00028 #include <cyassl/ctaocrypt/misc.h> 00029 #else 00030 #include <ctaocrypt/src/misc.c> 00031 #endif 00032 00033 00034 #ifdef STM32F2_CRYPTO 00035 /* 00036 * STM32F2 hardware SHA1 support through the STM32F2 standard peripheral 00037 * library. Documentation located in STM32F2xx Standard Peripheral Library 00038 * document (See note in README). 00039 */ 00040 #include "stm32f2xx.h" 00041 00042 void InitSha(Sha* sha) 00043 { 00044 /* STM32F2 struct notes: 00045 * sha->buffer = first 4 bytes used to hold partial block if needed 00046 * sha->buffLen = num bytes currently stored in sha->buffer 00047 * sha->loLen = num bytes that have been written to STM32 FIFO 00048 */ 00049 XMEMSET(sha->buffer, 0, SHA_REG_SIZE); 00050 sha->buffLen = 0; 00051 sha->loLen = 0; 00052 00053 /* initialize HASH peripheral */ 00054 HASH_DeInit(); 00055 00056 /* configure algo used, algo mode, datatype */ 00057 HASH->CR &= ~ (HASH_CR_ALGO | HASH_CR_DATATYPE | HASH_CR_MODE); 00058 HASH->CR |= (HASH_AlgoSelection_SHA1 | HASH_AlgoMode_HASH 00059 | HASH_DataType_8b); 00060 00061 /* reset HASH processor */ 00062 HASH->CR |= HASH_CR_INIT; 00063 } 00064 00065 void ShaUpdate(Sha* sha, const byte* data, word32 len) 00066 { 00067 word32 i = 0; 00068 word32 fill = 0; 00069 word32 diff = 0; 00070 00071 /* if saved partial block is available */ 00072 if (sha->buffLen) { 00073 fill = 4 - sha->buffLen; 00074 00075 /* if enough data to fill, fill and push to FIFO */ 00076 if (fill <= len) { 00077 XMEMCPY((byte*)sha->buffer + sha->buffLen, data, fill); 00078 HASH_DataIn(*(uint32_t*)sha->buffer); 00079 00080 data += fill; 00081 len -= fill; 00082 sha->loLen += 4; 00083 sha->buffLen = 0; 00084 } else { 00085 /* append partial to existing stored block */ 00086 XMEMCPY((byte*)sha->buffer + sha->buffLen, data, len); 00087 sha->buffLen += len; 00088 return; 00089 } 00090 } 00091 00092 /* write input block in the IN FIFO */ 00093 for(i = 0; i < len; i += 4) 00094 { 00095 diff = len - i; 00096 if ( diff < 4) { 00097 /* store incomplete last block, not yet in FIFO */ 00098 XMEMSET(sha->buffer, 0, SHA_REG_SIZE); 00099 XMEMCPY((byte*)sha->buffer, data, diff); 00100 sha->buffLen = diff; 00101 } else { 00102 HASH_DataIn(*(uint32_t*)data); 00103 data+=4; 00104 } 00105 } 00106 00107 /* keep track of total data length thus far */ 00108 sha->loLen += (len - sha->buffLen); 00109 } 00110 00111 void ShaFinal(Sha* sha, byte* hash) 00112 { 00113 __IO uint16_t nbvalidbitsdata = 0; 00114 00115 /* finish reading any trailing bytes into FIFO */ 00116 if (sha->buffLen) { 00117 HASH_DataIn(*(uint32_t*)sha->buffer); 00118 sha->loLen += sha->buffLen; 00119 } 00120 00121 /* calculate number of valid bits in last word of input data */ 00122 nbvalidbitsdata = 8 * (sha->loLen % SHA_REG_SIZE); 00123 00124 /* configure number of valid bits in last word of the data */ 00125 HASH_SetLastWordValidBitsNbr(nbvalidbitsdata); 00126 00127 /* start HASH processor */ 00128 HASH_StartDigest(); 00129 00130 /* wait until Busy flag == RESET */ 00131 while (HASH_GetFlagStatus(HASH_FLAG_BUSY) != RESET) {} 00132 00133 /* read message digest */ 00134 sha->digest[0] = HASH->HR[0]; 00135 sha->digest[1] = HASH->HR[1]; 00136 sha->digest[2] = HASH->HR[2]; 00137 sha->digest[3] = HASH->HR[3]; 00138 sha->digest[4] = HASH->HR[4]; 00139 00140 ByteReverseWords(sha->digest, sha->digest, SHA_DIGEST_SIZE); 00141 00142 XMEMCPY(hash, sha->digest, SHA_DIGEST_SIZE); 00143 00144 InitSha(sha); /* reset state */ 00145 } 00146 00147 #else /* CTaoCrypt software implementation */ 00148 00149 #ifndef min 00150 00151 static INLINE word32 min(word32 a, word32 b) 00152 { 00153 return a > b ? b : a; 00154 } 00155 00156 #endif /* min */ 00157 00158 00159 void InitSha(Sha* sha) 00160 { 00161 sha->digest[0] = 0x67452301L; 00162 sha->digest[1] = 0xEFCDAB89L; 00163 sha->digest[2] = 0x98BADCFEL; 00164 sha->digest[3] = 0x10325476L; 00165 sha->digest[4] = 0xC3D2E1F0L; 00166 00167 sha->buffLen = 0; 00168 sha->loLen = 0; 00169 sha->hiLen = 0; 00170 } 00171 00172 #define blk0(i) (W[i] = sha->buffer[i]) 00173 #define blk1(i) (W[i&15] = \ 00174 rotlFixed(W[(i+13)&15]^W[(i+8)&15]^W[(i+2)&15]^W[i&15],1)) 00175 00176 #define f1(x,y,z) (z^(x &(y^z))) 00177 #define f2(x,y,z) (x^y^z) 00178 #define f3(x,y,z) ((x&y)|(z&(x|y))) 00179 #define f4(x,y,z) (x^y^z) 00180 00181 /* (R0+R1), R2, R3, R4 are the different operations used in SHA1 */ 00182 #define R0(v,w,x,y,z,i) z+= f1(w,x,y) + blk0(i) + 0x5A827999+ \ 00183 rotlFixed(v,5); w = rotlFixed(w,30); 00184 #define R1(v,w,x,y,z,i) z+= f1(w,x,y) + blk1(i) + 0x5A827999+ \ 00185 rotlFixed(v,5); w = rotlFixed(w,30); 00186 #define R2(v,w,x,y,z,i) z+= f2(w,x,y) + blk1(i) + 0x6ED9EBA1+ \ 00187 rotlFixed(v,5); w = rotlFixed(w,30); 00188 #define R3(v,w,x,y,z,i) z+= f3(w,x,y) + blk1(i) + 0x8F1BBCDC+ \ 00189 rotlFixed(v,5); w = rotlFixed(w,30); 00190 #define R4(v,w,x,y,z,i) z+= f4(w,x,y) + blk1(i) + 0xCA62C1D6+ \ 00191 rotlFixed(v,5); w = rotlFixed(w,30); 00192 00193 00194 static void Transform(Sha* sha) 00195 { 00196 word32 W[SHA_BLOCK_SIZE / sizeof(word32)]; 00197 00198 /* Copy context->state[] to working vars */ 00199 word32 a = sha->digest[0]; 00200 word32 b = sha->digest[1]; 00201 word32 c = sha->digest[2]; 00202 word32 d = sha->digest[3]; 00203 word32 e = sha->digest[4]; 00204 00205 #ifdef USE_SLOW_SHA 00206 word32 t, i; 00207 00208 for (i = 0; i < 16; i++) { 00209 R0(a, b, c, d, e, i); 00210 t = e; e = d; d = c; c = b; b = a; a = t; 00211 } 00212 00213 for (; i < 20; i++) { 00214 R1(a, b, c, d, e, i); 00215 t = e; e = d; d = c; c = b; b = a; a = t; 00216 } 00217 00218 for (; i < 40; i++) { 00219 R2(a, b, c, d, e, i); 00220 t = e; e = d; d = c; c = b; b = a; a = t; 00221 } 00222 00223 for (; i < 60; i++) { 00224 R3(a, b, c, d, e, i); 00225 t = e; e = d; d = c; c = b; b = a; a = t; 00226 } 00227 00228 for (; i < 80; i++) { 00229 R4(a, b, c, d, e, i); 00230 t = e; e = d; d = c; c = b; b = a; a = t; 00231 } 00232 #else 00233 /* nearly 1 K bigger in code size but 25% faster */ 00234 /* 4 rounds of 20 operations each. Loop unrolled. */ 00235 R0(a,b,c,d,e, 0); R0(e,a,b,c,d, 1); R0(d,e,a,b,c, 2); R0(c,d,e,a,b, 3); 00236 R0(b,c,d,e,a, 4); R0(a,b,c,d,e, 5); R0(e,a,b,c,d, 6); R0(d,e,a,b,c, 7); 00237 R0(c,d,e,a,b, 8); R0(b,c,d,e,a, 9); R0(a,b,c,d,e,10); R0(e,a,b,c,d,11); 00238 R0(d,e,a,b,c,12); R0(c,d,e,a,b,13); R0(b,c,d,e,a,14); R0(a,b,c,d,e,15); 00239 00240 R1(e,a,b,c,d,16); R1(d,e,a,b,c,17); R1(c,d,e,a,b,18); R1(b,c,d,e,a,19); 00241 00242 R2(a,b,c,d,e,20); R2(e,a,b,c,d,21); R2(d,e,a,b,c,22); R2(c,d,e,a,b,23); 00243 R2(b,c,d,e,a,24); R2(a,b,c,d,e,25); R2(e,a,b,c,d,26); R2(d,e,a,b,c,27); 00244 R2(c,d,e,a,b,28); R2(b,c,d,e,a,29); R2(a,b,c,d,e,30); R2(e,a,b,c,d,31); 00245 R2(d,e,a,b,c,32); R2(c,d,e,a,b,33); R2(b,c,d,e,a,34); R2(a,b,c,d,e,35); 00246 R2(e,a,b,c,d,36); R2(d,e,a,b,c,37); R2(c,d,e,a,b,38); R2(b,c,d,e,a,39); 00247 00248 R3(a,b,c,d,e,40); R3(e,a,b,c,d,41); R3(d,e,a,b,c,42); R3(c,d,e,a,b,43); 00249 R3(b,c,d,e,a,44); R3(a,b,c,d,e,45); R3(e,a,b,c,d,46); R3(d,e,a,b,c,47); 00250 R3(c,d,e,a,b,48); R3(b,c,d,e,a,49); R3(a,b,c,d,e,50); R3(e,a,b,c,d,51); 00251 R3(d,e,a,b,c,52); R3(c,d,e,a,b,53); R3(b,c,d,e,a,54); R3(a,b,c,d,e,55); 00252 R3(e,a,b,c,d,56); R3(d,e,a,b,c,57); R3(c,d,e,a,b,58); R3(b,c,d,e,a,59); 00253 00254 R4(a,b,c,d,e,60); R4(e,a,b,c,d,61); R4(d,e,a,b,c,62); R4(c,d,e,a,b,63); 00255 R4(b,c,d,e,a,64); R4(a,b,c,d,e,65); R4(e,a,b,c,d,66); R4(d,e,a,b,c,67); 00256 R4(c,d,e,a,b,68); R4(b,c,d,e,a,69); R4(a,b,c,d,e,70); R4(e,a,b,c,d,71); 00257 R4(d,e,a,b,c,72); R4(c,d,e,a,b,73); R4(b,c,d,e,a,74); R4(a,b,c,d,e,75); 00258 R4(e,a,b,c,d,76); R4(d,e,a,b,c,77); R4(c,d,e,a,b,78); R4(b,c,d,e,a,79); 00259 #endif 00260 00261 /* Add the working vars back into digest state[] */ 00262 sha->digest[0] += a; 00263 sha->digest[1] += b; 00264 sha->digest[2] += c; 00265 sha->digest[3] += d; 00266 sha->digest[4] += e; 00267 } 00268 00269 00270 static INLINE void AddLength(Sha* sha, word32 len) 00271 { 00272 word32 tmp = sha->loLen; 00273 if ( (sha->loLen += len) < tmp) 00274 sha->hiLen++; /* carry low to high */ 00275 } 00276 00277 00278 void ShaUpdate(Sha* sha, const byte* data, word32 len) 00279 { 00280 /* do block size increments */ 00281 byte* local = (byte*)sha->buffer; 00282 00283 while (len) { 00284 word32 add = min(len, SHA_BLOCK_SIZE - sha->buffLen); 00285 XMEMCPY(&local[sha->buffLen], data, add); 00286 00287 sha->buffLen += add; 00288 data += add; 00289 len -= add; 00290 00291 if (sha->buffLen == SHA_BLOCK_SIZE) { 00292 #ifdef LITTLE_ENDIAN_ORDER 00293 ByteReverseBytes(local, local, SHA_BLOCK_SIZE); 00294 #endif 00295 Transform(sha); 00296 AddLength(sha, SHA_BLOCK_SIZE); 00297 sha->buffLen = 0; 00298 } 00299 } 00300 } 00301 00302 00303 void ShaFinal(Sha* sha, byte* hash) 00304 { 00305 byte* local = (byte*)sha->buffer; 00306 00307 AddLength(sha, sha->buffLen); /* before adding pads */ 00308 00309 local[sha->buffLen++] = 0x80; /* add 1 */ 00310 00311 /* pad with zeros */ 00312 if (sha->buffLen > SHA_PAD_SIZE) { 00313 XMEMSET(&local[sha->buffLen], 0, SHA_BLOCK_SIZE - sha->buffLen); 00314 sha->buffLen += SHA_BLOCK_SIZE - sha->buffLen; 00315 00316 #ifdef LITTLE_ENDIAN_ORDER 00317 ByteReverseBytes(local, local, SHA_BLOCK_SIZE); 00318 #endif 00319 Transform(sha); 00320 sha->buffLen = 0; 00321 } 00322 XMEMSET(&local[sha->buffLen], 0, SHA_PAD_SIZE - sha->buffLen); 00323 00324 /* put lengths in bits */ 00325 sha->hiLen = (sha->loLen >> (8*sizeof(sha->loLen) - 3)) + 00326 (sha->hiLen << 3); 00327 sha->loLen = sha->loLen << 3; 00328 00329 /* store lengths */ 00330 #ifdef LITTLE_ENDIAN_ORDER 00331 ByteReverseBytes(local, local, SHA_BLOCK_SIZE); 00332 #endif 00333 /* ! length ordering dependent on digest endian type ! */ 00334 XMEMCPY(&local[SHA_PAD_SIZE], &sha->hiLen, sizeof(word32)); 00335 XMEMCPY(&local[SHA_PAD_SIZE + sizeof(word32)], &sha->loLen, sizeof(word32)); 00336 00337 Transform(sha); 00338 #ifdef LITTLE_ENDIAN_ORDER 00339 ByteReverseWords(sha->digest, sha->digest, SHA_DIGEST_SIZE); 00340 #endif 00341 XMEMCPY(hash, sha->digest, SHA_DIGEST_SIZE); 00342 00343 InitSha(sha); /* reset state */ 00344 } 00345 00346 #endif /* STM32F2_CRYPTO */ 00347
Generated on Thu Jul 14 2022 00:25:23 by
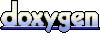