cyassl re-port with cellular comms, PSK test
Dependencies: VodafoneUSBModem_bleedingedge2 mbed-rtos mbed-src
rsa.h
00001 /* rsa.h for openSSL */ 00002 00003 00004 #ifndef CYASSL_RSA_H_ 00005 #define CYASSL_RSA_H_ 00006 00007 #include <cyassl/openssl/ssl.h> 00008 #include <cyassl/openssl/bn.h> 00009 00010 00011 #ifdef __cplusplus 00012 extern "C" { 00013 #endif 00014 00015 00016 enum { 00017 RSA_PKCS1_PADDING = 1 00018 }; 00019 00020 struct CYASSL_RSA { 00021 CYASSL_BIGNUM* n; 00022 CYASSL_BIGNUM* e; 00023 CYASSL_BIGNUM* d; 00024 CYASSL_BIGNUM* p; 00025 CYASSL_BIGNUM* q; 00026 CYASSL_BIGNUM* dmp1; /* dP */ 00027 CYASSL_BIGNUM* dmq1; /* dQ */ 00028 CYASSL_BIGNUM* iqmp; /* u */ 00029 void* internal; /* our RSA */ 00030 char inSet; /* internal set from external ? */ 00031 char exSet; /* external set from internal ? */ 00032 }; 00033 00034 00035 CYASSL_API CYASSL_RSA* CyaSSL_RSA_new(void); 00036 CYASSL_API void CyaSSL_RSA_free(CYASSL_RSA*); 00037 00038 CYASSL_API int CyaSSL_RSA_generate_key_ex(CYASSL_RSA*, int bits, CYASSL_BIGNUM*, 00039 void* cb); 00040 00041 CYASSL_API int CyaSSL_RSA_blinding_on(CYASSL_RSA*, CYASSL_BN_CTX*); 00042 CYASSL_API int CyaSSL_RSA_public_encrypt(int len, unsigned char* fr, 00043 unsigned char* to, CYASSL_RSA*, int padding); 00044 CYASSL_API int CyaSSL_RSA_private_decrypt(int len, unsigned char* fr, 00045 unsigned char* to, CYASSL_RSA*, int padding); 00046 00047 CYASSL_API int CyaSSL_RSA_size(const CYASSL_RSA*); 00048 CYASSL_API int CyaSSL_RSA_sign(int type, const unsigned char* m, 00049 unsigned int mLen, unsigned char* sigRet, 00050 unsigned int* sigLen, CYASSL_RSA*); 00051 CYASSL_API int CyaSSL_RSA_public_decrypt(int flen, unsigned char* from, 00052 unsigned char* to, CYASSL_RSA*, int padding); 00053 CYASSL_API int CyaSSL_RSA_GenAdd(CYASSL_RSA*); 00054 CYASSL_API int CyaSSL_RSA_LoadDer(CYASSL_RSA*, const unsigned char*, int sz); 00055 00056 00057 #define RSA_new CyaSSL_RSA_new 00058 #define RSA_free CyaSSL_RSA_free 00059 00060 #define RSA_generate_key_ex CyaSSL_RSA_generate_key_ex 00061 00062 #define RSA_blinding_on CyaSSL_RSA_blinding_on 00063 #define RSA_public_encrypt CyaSSL_RSA_public_encrypt 00064 #define RSA_private_decrypt CyaSSL_RSA_private_decrypt 00065 00066 #define RSA_size CyaSSL_RSA_size 00067 #define RSA_sign CyaSSL_RSA_sign 00068 #define RSA_public_decrypt CyaSSL_RSA_public_decrypt 00069 00070 00071 #ifdef __cplusplus 00072 } /* extern "C" */ 00073 #endif 00074 00075 #endif /* header */
Generated on Thu Jul 14 2022 00:25:23 by
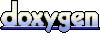