cyassl re-port with cellular comms, PSK test
Dependencies: VodafoneUSBModem_bleedingedge2 mbed-rtos mbed-src
ecc.h
00001 /* ecc.h 00002 * 00003 * Copyright (C) 2006-2012 Sawtooth Consulting Ltd. 00004 * 00005 * This file is part of CyaSSL. 00006 * 00007 * CyaSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * CyaSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA 00020 */ 00021 00022 #ifdef HAVE_ECC 00023 00024 #ifndef CTAO_CRYPT_ECC_H 00025 #define CTAO_CRYPT_ECC_H 00026 00027 #include <cyassl/ctaocrypt/types.h> 00028 #include <cyassl/ctaocrypt/integer.h> 00029 #include <cyassl/ctaocrypt/random.h> 00030 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif 00034 00035 00036 enum { 00037 ECC_PUBLICKEY = 1, 00038 ECC_PRIVATEKEY = 2, 00039 ECC_MAXNAME = 16, /* MAX CURVE NAME LENGTH */ 00040 SIG_HEADER_SZ = 6, /* ECC signature header size */ 00041 ECC_BUFSIZE = 256, /* for exported keys temp buffer */ 00042 ECC_MINSIZE = 20, /* MIN Private Key size */ 00043 ECC_MAXSIZE = 66 /* MAX Private Key size */ 00044 }; 00045 00046 00047 /* ECC set type defined a NIST GF(p) curve */ 00048 typedef struct { 00049 int size; /* The size of the curve in octets */ 00050 const char* name; /* name of this curve */ 00051 const char* prime; /* prime that defines the field, curve is in (hex) */ 00052 const char* B; /* fields B param (hex) */ 00053 const char* order; /* order of the curve (hex) */ 00054 const char* Gx; /* x coordinate of the base point on curve (hex) */ 00055 const char* Gy; /* y coordinate of the base point on curve (hex) */ 00056 } ecc_set_type; 00057 00058 00059 /* A point on an ECC curve, stored in Jacbobian format such that (x,y,z) => 00060 (x/z^2, y/z^3, 1) when interpreted as affine */ 00061 typedef struct { 00062 mp_int x; /* The x coordinate */ 00063 mp_int y; /* The y coordinate */ 00064 mp_int z; /* The z coordinate */ 00065 } ecc_point; 00066 00067 00068 /* An ECC Key */ 00069 typedef struct { 00070 int type; /* Public or Private */ 00071 int idx; /* Index into the ecc_sets[] for the parameters of 00072 this curve if -1, this key is using user supplied 00073 curve in dp */ 00074 const ecc_set_type* dp; /* domain parameters, either points to NIST 00075 curves (idx >= 0) or user supplied */ 00076 ecc_point pubkey; /* public key */ 00077 mp_int k; /* private key */ 00078 } ecc_key; 00079 00080 00081 /* ECC predefined curve sets */ 00082 extern const ecc_set_type ecc_sets[]; 00083 00084 00085 CYASSL_API 00086 int ecc_make_key(RNG* rng, int keysize, ecc_key* key); 00087 CYASSL_API 00088 int ecc_shared_secret(ecc_key* private_key, ecc_key* public_key, byte* out, 00089 word32* outlen); 00090 CYASSL_API 00091 int ecc_sign_hash(const byte* in, word32 inlen, byte* out, word32 *outlen, 00092 RNG* rng, ecc_key* key); 00093 CYASSL_API 00094 int ecc_verify_hash(const byte* sig, word32 siglen, byte* hash, word32 hashlen, 00095 int* stat, ecc_key* key); 00096 CYASSL_API 00097 void ecc_init(ecc_key* key); 00098 CYASSL_API 00099 void ecc_free(ecc_key* key); 00100 00101 00102 /* ASN key helpers */ 00103 CYASSL_API 00104 int ecc_export_x963(ecc_key*, byte* out, word32* outLen); 00105 CYASSL_API 00106 int ecc_import_x963(const byte* in, word32 inLen, ecc_key* key); 00107 CYASSL_API 00108 int ecc_import_private_key(const byte* priv, word32 privSz, const byte* pub, 00109 word32 pubSz, ecc_key* key); 00110 00111 /* size helper */ 00112 CYASSL_API 00113 int ecc_size(ecc_key* key); 00114 CYASSL_API 00115 int ecc_sig_size(ecc_key* key); 00116 00117 /* TODO: fix mutex types */ 00118 #define MUTEX_GLOBAL(x) int (x); 00119 #define MUTEX_LOCK(x) 00120 #define MUTEX_UNLOCK(x) 00121 00122 00123 00124 #ifdef __cplusplus 00125 } /* extern "C" */ 00126 #endif 00127 00128 #endif /* CTAO_CRYPT_ECC_H */ 00129 #endif /* HAVE_ECC */
Generated on Thu Jul 14 2022 00:25:23 by
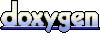