cyassl re-port with cellular comms, PSK test
Dependencies: VodafoneUSBModem_bleedingedge2 mbed-rtos mbed-src
camellia.h
00001 /* camellia.h ver 1.2.0 00002 * 00003 * Copyright (c) 2006,2007 00004 * NTT (Nippon Telegraph and Telephone Corporation) . All rights reserved. 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 1. Redistributions of source code must retain the above copyright 00010 * notice, this list of conditions and the following disclaimer as 00011 * the first lines of this file unmodified. 00012 * 2. Redistributions in binary form must reproduce the above copyright 00013 * notice, this list of conditions and the following disclaimer in the 00014 * documentation and/or other materials provided with the distribution. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY NTT ``AS IS'' AND ANY EXPRESS OR 00017 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES 00018 * OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00019 * IN NO EVENT SHALL NTT BE LIABLE FOR ANY DIRECT, INDIRECT, 00020 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 00021 * NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00022 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00023 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF 00025 * THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 /* camellia.h 00029 * 00030 * Copyright (C) 2006-2013 Sawtooth Consulting Ltd. 00031 * 00032 * This file is part of CyaSSL. 00033 * 00034 * CyaSSL is free software; you can redistribute it and/or modify 00035 * it under the terms of the GNU General Public License as published by 00036 * the Free Software Foundation; either version 2 of the License, or 00037 * (at your option) any later version. 00038 * 00039 * CyaSSL is distributed in the hope that it will be useful, 00040 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00041 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00042 * GNU General Public License for more details. 00043 * 00044 * You should have received a copy of the GNU General Public License 00045 * along with this program; if not, write to the Free Software 00046 * Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA 00047 */ 00048 00049 #ifdef HAVE_CAMELLIA 00050 00051 #ifndef CTAO_CRYPT_CAMELLIA_H 00052 #define CTAO_CRYPT_CAMELLIA_H 00053 00054 00055 #include <cyassl/ctaocrypt/types.h> 00056 00057 #ifdef __cplusplus 00058 extern "C" { 00059 #endif 00060 00061 00062 enum { 00063 CAMELLIA_BLOCK_SIZE = 16 00064 }; 00065 00066 #define CAMELLIA_TABLE_BYTE_LEN 272 00067 #define CAMELLIA_TABLE_WORD_LEN (CAMELLIA_TABLE_BYTE_LEN / sizeof(word32)) 00068 00069 typedef word32 KEY_TABLE_TYPE[CAMELLIA_TABLE_WORD_LEN]; 00070 00071 typedef struct Camellia { 00072 word32 keySz; 00073 KEY_TABLE_TYPE key; 00074 word32 reg[CAMELLIA_BLOCK_SIZE / sizeof(word32)]; /* for CBC mode */ 00075 word32 tmp[CAMELLIA_BLOCK_SIZE / sizeof(word32)]; /* for CBC mode */ 00076 } Camellia; 00077 00078 00079 CYASSL_API int CamelliaSetKey(Camellia* cam, 00080 const byte* key, word32 len, const byte* iv); 00081 CYASSL_API int CamelliaSetIV(Camellia* cam, const byte* iv); 00082 CYASSL_API void CamelliaEncryptDirect(Camellia* cam, byte* out, const byte* in); 00083 CYASSL_API void CamelliaDecryptDirect(Camellia* cam, byte* out, const byte* in); 00084 CYASSL_API void CamelliaCbcEncrypt(Camellia* cam, 00085 byte* out, const byte* in, word32 sz); 00086 CYASSL_API void CamelliaCbcDecrypt(Camellia* cam, 00087 byte* out, const byte* in, word32 sz); 00088 00089 00090 #ifdef __cplusplus 00091 } /* extern "C" */ 00092 #endif 00093 00094 #endif /* CTAO_CRYPT_AES_H */ 00095 #endif /* HAVE_CAMELLIA */ 00096
Generated on Thu Jul 14 2022 00:25:23 by
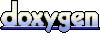