cyassl re-port with cellular comms, PSK test
Dependencies: VodafoneUSBModem_bleedingedge2 mbed-rtos mbed-src
callbacks.h
00001 /* cyassl_callbacks.h 00002 * 00003 * Copyright (C) 2012 Sawtooth Consulting Ltd. 00004 * 00005 * This file is part of CyaSSL. 00006 * 00007 * CyaSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * CyaSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA 00020 */ 00021 00022 00023 #ifndef CYASSL_CALLBACKS_H 00024 #define CYASSL_CALLBACKS_H 00025 00026 #include <sys/time.h> 00027 00028 #ifdef __cplusplus 00029 extern "C" { 00030 #endif 00031 00032 00033 enum { /* CALLBACK CONTSTANTS */ 00034 MAX_PACKETNAME_SZ = 24, 00035 MAX_CIPHERNAME_SZ = 24, 00036 MAX_TIMEOUT_NAME_SZ = 24, 00037 MAX_PACKETS_HANDSHAKE = 14, /* 12 for client auth plus 2 alerts */ 00038 MAX_VALUE_SZ = 128, /* all handshake packets but Cert should 00039 fit here */ 00040 }; 00041 00042 00043 typedef struct handShakeInfo_st { 00044 char cipherName[MAX_CIPHERNAME_SZ + 1]; /* negotiated cipher */ 00045 char packetNames[MAX_PACKETS_HANDSHAKE][MAX_PACKETNAME_SZ + 1]; 00046 /* SSL packet names */ 00047 int numberPackets; /* actual # of packets */ 00048 int negotiationError; /* cipher/parameter err */ 00049 } HandShakeInfo; 00050 00051 00052 typedef struct timeval Timeval; 00053 00054 00055 typedef struct packetInfo_st { 00056 char packetName[MAX_PACKETNAME_SZ + 1]; /* SSL packet name */ 00057 Timeval timestamp; /* when it occured */ 00058 unsigned char value[MAX_VALUE_SZ]; /* if fits, it's here */ 00059 unsigned char* bufferValue; /* otherwise here (non 0) */ 00060 int valueSz; /* sz of value or buffer */ 00061 } PacketInfo; 00062 00063 00064 typedef struct timeoutInfo_st { 00065 char timeoutName[MAX_TIMEOUT_NAME_SZ + 1]; /* timeout Name */ 00066 int flags; /* for future use */ 00067 int numberPackets; /* actual # of packets */ 00068 PacketInfo packets[MAX_PACKETS_HANDSHAKE]; /* list of all packets */ 00069 Timeval timeoutValue; /* timer that caused it */ 00070 } TimeoutInfo; 00071 00072 00073 00074 #ifdef __cplusplus 00075 } /* extern "C" */ 00076 #endif 00077 00078 00079 #endif /* CyaSSL_CALLBACKS_H */ 00080
Generated on Thu Jul 14 2022 00:25:23 by
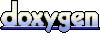