ajout module_mouvement
Dependencies: mbed xbee_lib ADXL345_I2C IMUfilter ITG3200 Motor RangeFinder Servo mbos PID
Fork of Labo_TRSE_Drone by
Buffer_Trame.cpp
00001 /* Copyright (c) 2012 - 2013 AUTEUR 00002 * 00003 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR "AS IS" AND ANY EXPRESS OR IMPLIED 00004 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00005 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00006 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00007 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00008 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00009 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, 00010 * STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY 00011 * OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00012 */ 00013 00014 /* 00015 * Description : Cette classe contient les fonctionnalités d'un buffer de trame. 00016 * Input 00017 * Output 00018 */ 00019 00020 #include "Buffer_Trame.h" 00021 00022 /* CONSRTRUCTEUR(S) */ 00023 C_FrameBuffer::C_FrameBuffer() 00024 { 00025 m_frameBuffer = new frame[default_size]; 00026 m_maxSize = default_size; 00027 m_currentReadIndex = 0; 00028 m_currentWriteIndex = 0; 00029 m_numberMessage = 0; 00030 } 00031 00032 C_FrameBuffer::C_FrameBuffer(unsigned int size) 00033 { 00034 m_maxSize = size; 00035 m_frameBuffer = new frame[m_maxSize]; 00036 m_currentReadIndex = 0; 00037 m_currentWriteIndex = 0; 00038 m_numberMessage = 0; 00039 } 00040 00041 /* DESTRUCTEUR */ 00042 C_FrameBuffer::~C_FrameBuffer() 00043 { 00044 delete [] m_frameBuffer; 00045 m_maxSize = 0; 00046 m_currentReadIndex = 0; 00047 m_currentWriteIndex = 0; 00048 m_numberMessage = 0; 00049 } 00050 00051 /* Propriétés */ 00052 void C_FrameBuffer::frameBuffer(frame newFrame) 00053 { 00054 m_frameBuffer[m_currentWriteIndex] = newFrame; 00055 m_currentWriteIndex++; 00056 m_numberMessage++; 00057 00058 if(m_currentWriteIndex >= m_maxSize) m_currentWriteIndex = 0; 00059 } 00060 00061 frame C_FrameBuffer::frameBuffer(void) 00062 { 00063 frame newFrame = m_frameBuffer[m_currentReadIndex]; 00064 m_currentReadIndex++; 00065 m_numberMessage--; 00066 00067 if(m_currentReadIndex >= m_maxSize) m_currentReadIndex = 0; 00068 00069 return newFrame; 00070 } 00071 00072 unsigned int C_FrameBuffer::maxSize(void) 00073 { 00074 return m_maxSize; 00075 } 00076 00077 unsigned int C_FrameBuffer::currentReadIndex(void) 00078 { 00079 return m_currentReadIndex; 00080 } 00081 00082 unsigned int C_FrameBuffer::currentWriteIndex(void) 00083 { 00084 return m_currentWriteIndex; 00085 } 00086 00087 unsigned int C_FrameBuffer::numberMessage(void) 00088 { 00089 return m_numberMessage; 00090 }
Generated on Wed Jul 13 2022 00:16:44 by
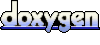