ajout module_mouvement
Dependencies: mbed xbee_lib ADXL345_I2C IMUfilter ITG3200 Motor RangeFinder Servo mbos PID
Fork of Labo_TRSE_Drone by
Acc_Giro.h
00001 #ifndef Acc_Giro_H 00002 #define Acc_Giro_H 00003 00004 /** 00005 * Includes 00006 */ 00007 #include "mbed.h" 00008 #include "ADXL345_I2C.h" 00009 #include "ITG3200.h" 00010 #include "IMUfilter.h" 00011 00012 /** 00013 * Defines 00014 */ 00015 //Gravity at Earth's surface in m/s/s 00016 #define g0 9.812865328 00017 //Number of samples to average. 00018 #define SAMPLES 4 00019 //Number of samples to be averaged for a null bias calculation 00020 //during calibration. 00021 #define CALIBRATION_SAMPLES 128 00022 //Convert from radians to degrees. 00023 #define toDegrees(x) (x * 57.2957795) 00024 //Convert from degrees to radians. 00025 #define toRadians(x) (x * 0.01745329252) 00026 //ITG-3200 sensitivity is 14.375 LSB/(degrees/sec). 00027 #define GYROSCOPE_GAIN (1 / 14.375) 00028 //Full scale resolution on the ADXL345 is 4mg/LSB. 00029 #define ACCELEROMETER_GAIN (0.004 * g0) 00030 //Sampling gyroscope at 200Hz. 00031 #define GYRO_RATE 0.002 00032 //Sampling accelerometer at 200Hz. 00033 #define ACC_RATE 0.002 00034 //Updating filter at 100Hz. 00035 #define FILTER_RATE 0.01 00036 #define CORRECTION_MAGNITUDE 50 00037 00038 class Acc_Giro { 00039 00040 public: 00041 00042 //Constructor. 00043 Acc_Giro(); 00044 //destructeur 00045 // ~Acc_Giro(); 00046 00047 //Set up the ADXL345 appropriately. 00048 void initializeAccelerometer(void); 00049 //Calculate the null bias. 00050 void calibrateAccelerometer(void); 00051 //Take a set of samples and average them. 00052 void sampleAccelerometer(void); 00053 //Set up the ITG3200 appropriately. 00054 void initializeGyroscope(void); 00055 //Calculate the null bias. 00056 void calibrateGyroscope(void); 00057 //Take a set of samples and average them. 00058 void sampleGyroscope(void); 00059 //Update the filter and calculate the Euler angles. 00060 void filter(void); 00061 void GetI2CAddress(); 00062 void dataSender(void); 00063 00064 //membre classe 00065 IMUfilter *imuFilter; 00066 ADXL345_I2C *accelerometer; 00067 I2C *i2c; 00068 ITG3200 *gyroscope; 00069 Ticker accelerometerTicker; 00070 Ticker gyroscopeTicker; 00071 Ticker filterTicker; 00072 Ticker dataTicker; 00073 Ticker algorithmTicker; 00074 00075 00076 00077 int calibrate; 00078 int calibrated; 00079 int start; 00080 int started; 00081 int alg_enable; 00082 00083 char data[128]; 00084 //Offsets for the gyroscope. 00085 //The readings we take when the gyroscope is stationary won't be 0, so we'll 00086 //average a set of readings we do get when the gyroscope is stationary and 00087 //take those away from subsequent readings to ensure the gyroscope is offset 00088 //or "biased" to 0. 00089 double w_xBias; 00090 double w_yBias; 00091 double w_zBias; 00092 00093 //Offsets for the accelerometer. 00094 //Same as with the gyroscope. 00095 double a_xBias; 00096 double a_yBias; 00097 double a_zBias; 00098 00099 //Accumulators used for oversampling and then averaging. 00100 volatile double a_xAccumulator; 00101 volatile double a_yAccumulator; 00102 volatile double a_zAccumulator; 00103 volatile double w_xAccumulator; 00104 volatile double w_yAccumulator; 00105 volatile double w_zAccumulator; 00106 00107 //Accelerometer and gyroscope readings for x, y, z axes. 00108 volatile double a_x; 00109 volatile double a_y; 00110 volatile double a_z; 00111 volatile double w_x; 00112 volatile double w_y; 00113 volatile double w_z; 00114 00115 //Buffer for accelerometer readings. 00116 int readings[3]; 00117 //Number of accelerometer samples we're on. 00118 int accelerometerSamples; 00119 //Number of gyroscope samples we're on. 00120 int gyroscopeSamples; 00121 00122 }; 00123 00124 00125 00126 #endif /* PID_H */
Generated on Wed Jul 13 2022 00:16:44 by
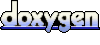