Library for initializing, configuring, and acquiring data from the Atmel QT2100 device. The QT2100 is a capacitive touch controller with 10 configurable QTouch® channels, supporting up to seven buttons, and either one slider or one wheel.
QT2100.h
00001 00002 /* 00003 Copyright (c) 2015 John Magyar 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef QT2100_H 00025 #define QT2100_H 00026 00027 #include "mbed.h" 00028 00029 /* 00030 00031 Header file for the Atmel QT2100 Capacative Touch sensor device Mbed library. 00032 00033 The QT2100 is a capacitive touch controller device with 10 configurable QTouch channels, 00034 supporting up to seven touch buttons, and either a single slider (or wheel). 00035 00036 Datasheet: http://www.atmel.com/images/at42qt2100_e_level0%2013ww04.pdf 00037 00038 */ 00039 00040 class QT2100 00041 { 00042 public: 00043 QT2100(PinName mosi, 00044 PinName miso, 00045 PinName sck, 00046 PinName cs, 00047 PinName tx, 00048 PinName rx); 00049 ~QT2100(); 00050 00051 public: 00052 void init(); 00053 void terminalDisplay(); 00054 void xfer3bytes(int byte0, int byte1, int byte2); 00055 void verifyChannels(); 00056 void devId(); 00057 int8_t keys(); 00058 int8_t slider(); 00059 int8_t wheel(); 00060 00061 private: 00062 SPI _spi; 00063 DigitalOut _cs; 00064 Serial _pc; 00065 }; 00066 00067 #endif // QT2100_H
Generated on Wed Jul 13 2022 09:56:57 by
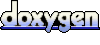