Melexis MLX90614 library
Dependents: IR_temperature IR_temperature IR_temperature UserIntefaceLCD ... more
mlx90614.cpp
00001 00002 #include "mlx90614.h" 00003 00004 00005 00006 00007 MLX90614::MLX90614(I2C* i2c,int addr){ 00008 00009 this->i2caddress = addr; 00010 this->i2c = i2c; 00011 00012 } 00013 00014 00015 bool MLX90614::getTemp(float* temp_val){ 00016 00017 char p1,p2,p3; 00018 float temp_thermo; 00019 bool ch; 00020 00021 i2c->stop(); //stop i2c if not ack 00022 wait(0.01); 00023 i2c->start(); //start I2C 00024 ch=i2c->write(i2caddress); //device address with write condition 00025 00026 if(!ch)return false; //No Ack, return False 00027 00028 ch=i2c->write(0x07); //device ram address where Tobj value is present 00029 00030 if(!ch)return false; //No Ack, return False 00031 00032 00033 i2c->start(); //repeat start 00034 ch=i2c->write(i2caddress|0x01); //device address with read condition 00035 if(!ch)return false; //No Ack, return False 00036 00037 p1=i2c->read(1); //Tobj low byte 00038 p2=i2c->read(1); //Tobj heigh byte 00039 p3=i2c->read(0); //PEC 00040 00041 i2c->stop(); //stop condition 00042 00043 00044 temp_thermo=((((p2&0x007f)<<8)+p1)*0.02)-0.01; //degree centigrate conversion 00045 *temp_val=temp_thermo-273; //Convert kelvin to degree Celsius 00046 00047 return true; //load data successfully, return true 00048 }
Generated on Sun Jul 17 2022 03:44:29 by
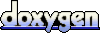