Embed:
(wiki syntax)
Show/hide line numbers
touch_tft.cpp
00001 #include "touch_tft.h" 00002 #include "mbed.h" 00003 #include "Arial12x12.h" 00004 00005 #define threshold 10000 00006 00007 touch_tft::touch_tft(PinName xp, PinName xm, PinName yp, PinName ym, PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset, PinName bk, const char *name) 00008 :_xp(xp),_xm(xm),_yp(yp),_ym(ym),_ax(xp),_ay(yp), SPI_TFT(mosi,miso,sclk,cs,reset,bk,name) { 00009 xa = xp; 00010 ya = yp; 00011 00012 } 00013 00014 /* Esta clase trae el valor de p, siendo "p" un puntero 00015 * 00016 */ 00017 point touch_tft::get_touch() { //puntero a touch clase get_touch 00018 unsigned short x1 = 0,x2 = 0, y1 = 0, y2 = 0; 00019 unsigned int s1 = 0,s2 = 0,d1 = 0 , d2 = 0; 00020 point p; 00021 00022 do { 00023 /******************************************************************************************/ 00024 // lee el voltaje en Y 00025 _xp.output(); //define salidas para x+ y x- 00026 _xm.output(); 00027 switch (orientation) { 00028 case(0): //En casos de orientacion 0 y 3 00029 case(3): //pone en alto el x+ 00030 _xp = 1; //pone en bajo el x- 00031 _xm = 0; 00032 break; 00033 case(1): //sino lo contrario 00034 case(2): 00035 _xp = 0; 00036 _xm = 1; 00037 break; 00038 } 00039 _ym.input(); // define y- como entrada para q sea "pasivo" 00040 AnalogIn Ay(ya); // define constructor analogico como Ay para el pin y+ 00041 // wait_us(10); 00042 y1 = Ay.read_u16(); // trae el voltaje en y1 desde y+ en el rango de 0 a 65535 00043 d1 = (y1 > y2)? (y1-y2) : (y2-y1); // //condiciona si y1 es mayor q y2 entonces opera d1=y1-y2 y si no es correcto entonces opera d1=y2-y1 00044 if (((y1 < 8000) && (d1 < 2000)) || ((y1 > 8000) && (d1 < 150))) s1 ++; // si (y1<8000 Y d1<2000) O (y1>8000 Y d1<150) 00045 // 32768 8192 32768 336 00046 else { 00047 if (s1 > 0) s1=0; //entonces s1 = s1+1, sino pregunta si s1>0 entonces s1 = s1-1 00048 } 00049 y2 = y1; //y2 es ahora igual a y1 00050 //debug 00051 //locate(1,7); 00052 //printf("d: %4d y: %5d s1: %4d",d1,y1,s1); 00053 /******************************************************************************************/ 00054 // // lee el voltaje en X 00055 _yp.output(); //define salidas para y+ y y- 00056 _ym.output(); 00057 switch (orientation) { 00058 case(0): //En casos de orientacion 0 y 3 00059 case(1): //pone en alto el x+ 00060 _yp = 1; //pone en bajo el x- 00061 _ym = 0; 00062 break; 00063 case(2): 00064 case(3): 00065 _yp = 0; //sino lo contrario 00066 _ym = 1; 00067 break; 00068 } 00069 _xm.input(); // define x- como entrada para q sea "pasivo" 00070 AnalogIn Ax(xa); // define constructor analogico como Ax para el pin x+ 00071 // wait_us(0); 00072 x1 = Ax.read_u16(); // trae el voltaje en x1 desde x+ en el rango de 0 a 65535 00073 d2 = (x1 > x2)? (x1-x2) : (x2-x1); //condiciona si x1 es mayor q x2 entonces opera d2=x1-x2 y si no es correcto entonces opera d2=x2-x1 00074 if (((x1 < 8000) && (d2 < 2000)) || ((x1 > 8000) && (d2 < 150))) s2 ++; // si (x1<8000 Y d2<2000) O (x1>8000 Y d2<150) //si(1+1)-> s2++ 00075 // 32768 8192 32768 336 00076 else { 00077 if (s2 > 0) s2=0; //entonces s2 = s2+1, sino pregunta si s2>0 entonces s2 = s2-1 00078 } 00079 x2 = x1; //y2 es ahora igual a y1 00080 // debug 00081 //locate(1,18); 00082 //printf("d: %4d x: %5d s2: %4d",d2,x1,s2); 00083 00084 00085 //wait_us(20); 00086 00087 } while (s1 < 5 || s2 < 5); // read until we have three samples close together 00088 00089 00090 00091 00092 switch (orientation) { 00093 case(0): 00094 case(2): 00095 p.y = (x1+x2) / 2; // promedio de dos muestras 00096 p.x = (y1+y2) / 2; 00097 break; 00098 case(1): 00099 case(3): 00100 p.x = (x1+x2) / 2; // promedio de dos muestras 00101 p.y = (y1+y2) / 2; 00102 break; 00103 } 00104 00105 return(p); 00106 } 00107 00108 void touch_tft::calibrate(void) { 00109 int i; 00110 int a = 0,b = 0,c = 0, d = 0; 00111 00112 //desde aki ocultar despues 00113 /* 00114 int pos_x, pos_y; 00115 point p; 00116 00117 set_font((unsigned char*)Arial12x12); // select the font 00118 // get the center of the screen 00119 pos_x = (columns() / 2) + 2; 00120 pos_x = pos_x * font[1]; 00121 pos_y = (rows() / 2) - 1; 00122 pos_y = pos_y * font[2]; 00123 00124 00125 ////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00126 00127 //calcula primera posicion en extremo superior derecho 00128 cls(); 00129 line(0,3,3,3,Orange); 00130 line(3,0,3,3,Orange); 00131 locate(pos_x- font[2]*3,pos_y); 00132 printf(" top "); 00133 locate(pos_x - font[2]*3 ,pos_y + font[2]); 00134 printf("right"); 00135 00136 for (i=0; i<5; i++) { 00137 while (p.y < threshold | p.x < threshold) // wait for TRUE touch 8192 00138 { 00139 p = get_touch(); 00140 } 00141 a += p.x; 00142 b += p.y; 00143 } 00144 00145 a = a/5;//55025;//a / 5; 00146 b = b/5;//9566;//b / 5; 00147 locate(1,36); 00148 printf("a: %4d b: %5d p.x: %4d p.y: %4d",a,b,p.x,p.y);//55405 9202 54669 9394 55069 9602 54957 10066 promedio a:55025 00149 wait(10); 00150 cls(); 00151 locate(pos_x - font[2]*2,pos_y); 00152 printf("Good!"); 00153 wait(0.5); 00154 00155 while (p.y > 10000 | p.x > 10000) // wait for no touch 00156 { 00157 p = get_touch(); 00158 locate(1,36); 00159 printf("p.x: %4d p.y: %4d",p.x,p.y);//55405 9202 54669 9394 55069 9602 54957 10066 promedio a:55025 00160 } 00161 00162 ////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00163 //calcula segunda posicion en extremo inferior izquierdo 00164 cls(); 00165 line(317,237,320,237,Orange); // paint cross 00166 line(317,237,317,240,Orange); 00167 locate(pos_x- font[2]*3,pos_y); 00168 printf("booton"); 00169 locate(pos_x- font[2]*3,pos_y + font[2]); 00170 printf(" left "); 00171 for (i=0; i<5; i++) { 00172 while (p.y < threshold | p.x < threshold) // wait for TRUE touch 00173 { 00174 p = get_touch(); 00175 } 00176 c+= p.x; 00177 d+= p.y; 00178 } 00179 c = c/5;//9786;// c / 5; 00180 d = d/5;//55933;// d / 5; 00181 locate(1,66); 00182 printf("c: %4d d: %5d p.x: %4d p.y: %4d",c,d,p.x,p.y);// 9842 55965 9122 55901 9730 56541 00183 wait(10); 00184 cls(); 00185 locate(pos_x - font[2]*2,pos_y); 00186 printf("Good!"); 00187 wait(0.5); 00188 00189 while (p.y > 10000 | p.x > 10000) // wait for no touch 00190 { 00191 p = get_touch(); 00192 locate(1,36); 00193 printf("p.x: %4d p.y: %4d",p.x,p.y);//55405 9202 54669 9394 55069 9602 54957 10066 promedio a:55025 00194 } 00195 00196 */ 00197 ///////////////////////////////////////////////////HASTA AKI LAS OPERACIONES//////////////////////////////////////////////////////// 00198 //Constantes para orientaciones 1 y 3: 00199 /* 00200 a = 9850; 00201 b = 9314;//11650 00202 c = 55250; 00203 d = 54821;//55313 00204 */ 00205 //Constantes para orientaciones 0 y 2: 00206 00207 a = 9750; 00208 b = 8550;//11650 00209 c = 55250; 00210 d = 54521;//55313 00211 00212 00213 x_off = a; 00214 y_off = b; 00215 00216 i = c-a; // delta x pp_tx = (c-a)/(w-6) = (54450-11600)/(320-6) = 42850/(314) = 136.46 00217 pp_tx = abs(i) / (width() - 6); 00218 00219 i = d-b; // delta y 00220 pp_ty = abs(i) / (height() - 4); 00221 //cls(); 00222 } 00223 00224 00225 point touch_tft::to_pixel(point a_point) { 00226 point p; 00227 00228 00229 p.x = ((a_point.x - x_off) / pp_tx)+4; 00230 if (p.x > width()) p.x = width(); 00231 p.y = (a_point.y - y_off) / pp_ty; 00232 if (p.y > height()) p.y = height(); 00233 return (p); 00234 } 00235 00236 bool touch_tft::is_touched(point a) { 00237 if (a.x > threshold & a.y > threshold) return(true); 00238 else return(false); 00239 } 00240 00241 point touch_tft::shared_pointer(unsigned int xo,unsigned int xf, unsigned int speed, unsigned repetitions) { 00242 int Delta_X; 00243 point p; 00244 Delta_X = (xf-xo); 00245 windows (xo,0,xf,240); 00246 p = get_touch(); 00247 if (is_touched(p)) { 00248 p = to_pixel(p); 00249 if (p.x <= xf && p.x >= xo){ 00250 //if (p.x <= Delta_X) 00251 sharepoint(xo,320-xf,Delta_X,abs(Delta_X-p.x-1),speed,repetitions); 00252 } 00253 wait(0.125); 00254 } 00255 return (p); 00256 00257 }
Generated on Wed Jul 20 2022 01:51:28 by
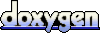