Embed:
(wiki syntax)
Show/hide line numbers
SPI_TFT.cpp
00001 #include "SPI_TFT.h" 00002 #include "mbed.h" 00003 00004 00005 #define BPP 16 // Bits per pixel 00006 00007 SPI_TFT::SPI_TFT(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName reset, PinName bk, const char *name) 00008 :_spi(mosi, miso, sclk), _cs(cs), _reset(reset), _bk(bk), GraphicsDisplay(name) { //Constructor 00009 //tft_rst_hw(); 00010 tft_init(); 00011 backlight(0); 00012 orientation = 3; 00013 char_x = 0; 00014 backlight(0); //0.0001 00015 foreground(Black); 00016 background(Black); 00017 00018 } 00019 00020 int SPI_TFT::width() { 00021 if (orientation == 0 || orientation == 2) return 240; 00022 else return 320; 00023 } 00024 00025 00026 int SPI_TFT::height() { 00027 if (orientation == 0 || orientation == 2) return 320; 00028 else return 240; 00029 } 00030 00031 00032 00033 void SPI_TFT::set_orientation(unsigned int x) { 00034 orientation = x; 00035 switch (orientation) { 00036 case 0: 00037 wr_reg(0x16, 0x0008);//240x320 00038 break; 00039 case 1: 00040 wr_reg(0x16, 0x0068);//320x240 00041 break; 00042 case 2: 00043 wr_reg(0x16, 0x00C8);//240x320 00044 break; 00045 case 3: 00046 wr_reg(0x16, 0x00A8);//320x240 00047 break; 00048 } 00049 } 00050 00051 00052 //configura el registro index enviando para ello: (01110000+00000000+00000000)=01110000 00053 void SPI_TFT::wr_cmd(int cmd) { 00054 _cs = 0; 00055 _spi.write(SPI_START | SPI_WR | SPI_INDEX); /* Write : RS = 0, RW = 0 */ 00056 _spi.write(cmd); 00057 _cs = 1; 00058 } 00059 00060 00061 //escribe instrucciones o datos en GRAM enviando para ello: (01110000+00000000+00000010)=01110010 00062 void SPI_TFT::wr_dat(int dat) { 00063 _cs = 0; 00064 _spi.write(SPI_START | SPI_WR | SPI_DATA); // Write : RS = 1, RW = 0 00065 _spi.format(16,3); // switch to 16 bit Mode 3 00066 _spi.write(dat); // Write D0..D15 00067 _spi.format(16,3); // 8 bit Mode 3 00068 _cs = 1; 00069 } 00070 00071 00072 //escribe instrucciones o datos en GRAM enviando para ello: (01110000+00000000+00000010)=01110010 00073 void SPI_TFT::wr_dat_start(void) { 00074 _cs = 0; 00075 _spi.write(SPI_START | SPI_WR | SPI_DATA); /* Write : RS = 1, RW = 0 */ //70-00-02 00076 } 00077 00078 00079 //detiene la secuencia de envio y la comunicacion con el TFT 00080 void SPI_TFT::wr_dat_stop (void) { 00081 _cs = 1; 00082 } 00083 00084 00085 //envia un dato simple 00086 void SPI_TFT::wr_dat_only (unsigned short dat) { 00087 00088 _spi.format(16,3); // switch to 16 bit Mode 3 00089 _spi.write(dat); // Write D0..D15 00090 _spi.format(16,3); // 8 bit Mode 3 00091 } 00092 00093 00094 //lee comandos (16bits en total) pero no soporta lectura de GRAM enviando para ello: (01110000+00000001+00000010)=01110011 00095 unsigned short SPI_TFT::rd_dat (void) { 00096 unsigned short val = 0; 00097 00098 _cs = 0; 00099 _spi.write(SPI_START | SPI_RD | SPI_DATA); /* Read: RS = 1, RW = 1 */ 00100 _spi.write(0); /* Dummy read 1 */ //lectura ficticia 00101 val = _spi.write(0); /* Read D8..D15 */ //lee los altos y luego los bajos 00102 val <<= 8; 00103 val |= _spi.write(0); /* Read D0..D7 */ 00104 _cs = 1; 00105 return (val);//devuelve la concatenacion completa de 16bits 00106 } 00107 00108 00109 00110 void SPI_TFT::wr_reg (unsigned char reg, unsigned short val) { 00111 00112 wr_cmd(reg); 00113 wr_dat(val); 00114 } 00115 00116 00117 00118 unsigned short SPI_TFT::rd_reg (unsigned char reg) { 00119 00120 wr_cmd(reg); 00121 return(rd_dat()); 00122 } 00123 00124 int SPI_TFT::ID() { 00125 static unsigned short driverCode; 00126 driverCode = rd_reg(0x01); // read controller ID 00127 //printf("Disp_ID = %x debe ser 47h",driverCode); 00128 //pc.printf("Disp_ID = %x debe ser 47h",tt.ID()); 00129 return driverCode; 00130 } 00131 00132 void SPI_TFT::tft_init() { 00133 //static unsigned short driverCode; 00134 _spi.format(16,3); // 8 bit spi mode 3 00135 _spi.frequency(100000000); // 48Mhz SPI clock 00136 _reset = 0; // reset 00137 _cs = 1; 00138 wait_us(50); 00139 _reset = 1; // end reset 00140 wait_ms(6);//5 00141 /* 00142 driverCode = rd_reg(0x00); // read controller ID 00143 printf("Disp_ID = %x debe ser 47h",driverCode); 00144 wait_ms(50); 00145 */ 00146 00147 // SECUENCIA DE INICIO Y DEFINICION DE CONFIGURACIONES GENERALES // 00148 00149 /* Start Initial Sequence ----------------------------------------------------*/ 00150 wr_reg(0xEA, 0x0000); /* Reset Power Control 1 */ 00151 wr_reg(0xEB, 0x0020); /* Power Control 2 */ 00152 wr_reg(0xEC, 0x000C); /* Power Control 3 */ 00153 wr_reg(0xED, 0x00C4); /* Power Control 4 */ 00154 wr_reg(0xE8, 0x0040); /* Source OPON_N */ 00155 wr_reg(0xE9, 0x0038); /* Source OPON_I */ 00156 wr_reg(0xF1, 0x0001); /* */ 00157 wr_reg(0xF2, 0x0010); /* */ 00158 wr_reg(0x27, 0x00A3); /* Display Control 2 */ 00159 00160 00161 /* Gamma settings -----------------------------------------------------------*/ 00162 wr_reg(0x40,0x00); // 00163 wr_reg(0x41,0x00); // 00164 wr_reg(0x42,0x01); // 00165 wr_reg(0x43,0x12); //t12 00166 wr_reg(0x44,0x10); // 00167 wr_reg(0x45,0x26); // 00168 wr_reg(0x46,0x08); // 00169 wr_reg(0x47,0x53); //t53 00170 wr_reg(0x48,0x02); // 00171 wr_reg(0x49,0x15); //t15 00172 wr_reg(0x4A,0x19); //t19 00173 wr_reg(0x4B,0x19); // 00174 wr_reg(0x4C,0x16); //t16 00175 wr_reg(0x50,0x19); // 00176 wr_reg(0x51,0x2F); // 00177 wr_reg(0x52,0x2D); //t2D 00178 wr_reg(0x53,0x3E); // 00179 wr_reg(0x54,0x3F); // 00180 wr_reg(0x55,0x3F); // 00181 wr_reg(0x56,0x2C); //t2C 00182 wr_reg(0x57,0x77); // 00183 wr_reg(0x58,0x09); //t09 00184 wr_reg(0x59,0x06); // 00185 wr_reg(0x5A,0x06); //t06 00186 wr_reg(0x5B,0x0A); //t0A 00187 wr_reg(0x5C,0x1D); // 00188 wr_reg(0x5D,0xCC); // 00189 00190 /* Power On sequence ---------------------------------------------------------*/ 00191 wr_reg(0x1B, 0x001B); /* Power Control 2 *///VRH=4.65V 00192 wr_reg(0x1A, 0x0001); /* Power Control 1 *///BT (VGH~15V,VGL~-10V,DDVDH~5V) 00193 wr_reg(0x24, 0x002F); /* Vcom Control 2 *///VMH(VCOM High voltage ~3.2V) 00194 wr_reg(0x25, 0x0057); /* Vcom Control 3 *///VML(VCOM Low voltage -1.2V) 00195 00196 //****VCOM offset**/// 00197 wr_reg(0x23, 0x0097);//8D); /* Vcom Control 1 *///for Flicker adjust //can reload from OTP 00198 00199 00200 /* Power + Osc ---------------------------------------------------------------*/ 00201 wr_reg(0x18, 0x003f); /* OSC Control 1 */ //I/P_RADJ,N/P_RADJ, Normal mode 75Hz 00202 wr_reg(0x19, 0x0001); /* OSC Control 2 */ //OSC_EN='1', start Osc 00203 wr_reg(0x01, 0x0009);//scroll activado /* Display Mode Control */ //DP_STB='0', out deep sleep 00204 wr_reg(0x1F, 0x0088); /* Power Control 6 */ // GAS=1, VOMG=00, PON=0, DK=1, XDK=0, DVDH_TRI=0, STB=0 00205 wait_ms(5); /* Delay 5 ms */ 00206 wr_reg(0x1F, 0x0080); /* Power Control 6 */ // GAS=1, VOMG=00, PON=0, DK=0, XDK=0, DVDH_TRI=0, STB=0 00207 wait_ms(5); /* Delay 5 ms */ 00208 wr_reg(0x1F, 0x0090); /* Power Control 6 */ // GAS=1, VOMG=00, PON=1, DK=0, XDK=0, DVDH_TRI=0, STB=0 00209 wait_ms(5); /* Delay 5 ms */ 00210 wr_reg(0x1F, 0x00D0); /* Power Control 6 */ // GAS=1, VOMG=10, PON=1, DK=0, XDK=0, DDVDH_TRI=0, STB=0 00211 wait_ms(5); /* Delay 5 ms */ 00212 00213 wr_reg(0x17, 0x0005);//5 /* Colmod 16Bit/Pixel */ //default 0x0006 262k color // 0x0005 65k color 00214 00215 wr_reg(0x36, 0x0000); /* Panel Characteristic */ //SS_P, GS_P,REV_P,BGR_P 00216 wr_reg(0x28, 0x0038); /* Display Control 3 */ //GON=1, DTE=1, D=1000 00217 wait_ms(40); 00218 wr_reg(0x28, 0x003C); /* Display Control 3 */ //GON=1, DTE=1, D=1100 00219 00220 00221 00222 switch (orientation) { 00223 case 0: 00224 wr_reg(0x16, 0x0008); 00225 break; 00226 case 1: 00227 wr_reg(0x16, 0x0068); 00228 break; 00229 case 2: 00230 wr_reg(0x16, 0x00C8); 00231 break; 00232 case 3: 00233 wr_reg(0x16, 0x00A8); 00234 break; 00235 } 00236 00237 WindowMax (); 00238 } 00239 00240 00241 00242 00243 void SPI_TFT::pixel(int x, int y, int color) { 00244 wr_reg(0x03, (x >> 0)); 00245 wr_reg(0x02, (x >> 8)); 00246 wr_reg(0x07, (y >> 0)); 00247 wr_reg(0x06, (y >> 8)); 00248 //wr_reg(0x05, (x+1 >> 0)); 00249 //wr_reg(0x04, (x+1 >> 8)); 00250 //wr_reg(0x09, (y+1 >> 0)); 00251 //wr_reg(0x08, (y+1 >> 8)); 00252 wr_cmd(0x22); 00253 wr_dat(color); 00254 } 00255 00256 00257 00258 00259 void SPI_TFT::windows (unsigned int x, unsigned int y, unsigned int w, unsigned int h) { 00260 wr_reg(0x03, (x >> 0)); 00261 wr_reg(0x02, (x >> 8)); 00262 wr_reg(0x05, (x+w-1 >> 0)); 00263 wr_reg(0x04, (x+w-1 >> 8)); 00264 wr_reg(0x07, ( y >> 0)); 00265 wr_reg(0x06, ( y >> 8)); 00266 wr_reg(0x09, ( y+h-1 >> 0)); 00267 wr_reg(0x08, ( y+h-1 >> 8)); 00268 //wr_cmd(0x22); 00269 } 00270 00271 00272 void SPI_TFT::WindowMax (void) { 00273 windows (0, 0, width(), height()); 00274 } 00275 00276 00277 void SPI_TFT::cls (void) { 00278 unsigned int i; 00279 WindowMax(); 00280 wr_cmd(0x22); 00281 wr_dat_start(); 00282 _spi.format(16,3); // 16 bit Mode 3 00283 for (i = 0; i < ( width() * height()); i++) 00284 _spi.write(_background); 00285 _spi.format(16,3); // 8 bit Mode 3 00286 wr_dat_stop(); 00287 } 00288 00289 00290 void SPI_TFT::circle(int x0, int y0, int r, int color) { 00291 00292 int draw_x0, draw_y0; 00293 int draw_x1, draw_y1; 00294 int draw_x2, draw_y2; 00295 int draw_x3, draw_y3; 00296 int draw_x4, draw_y4; 00297 int draw_x5, draw_y5; 00298 int draw_x6, draw_y6; 00299 int draw_x7, draw_y7; 00300 int xx, yy; 00301 int di; 00302 //WindowMax(); 00303 if (r == 0) { /* no radius */ 00304 return; 00305 } 00306 00307 draw_x0 = draw_x1 = x0; 00308 draw_y0 = draw_y1 = y0 + r; 00309 if (draw_y0 < height()) { 00310 pixel(draw_x0, draw_y0, color); /* 90 degree */ 00311 } 00312 00313 draw_x2 = draw_x3 = x0; 00314 draw_y2 = draw_y3 = y0 - r; 00315 if (draw_y2 >= 0) { 00316 pixel(draw_x2, draw_y2, color); /* 270 degree */ 00317 } 00318 00319 draw_x4 = draw_x6 = x0 + r; 00320 draw_y4 = draw_y6 = y0; 00321 if (draw_x4 < width()) { 00322 pixel(draw_x4, draw_y4, color); /* 0 degree */ 00323 } 00324 00325 draw_x5 = draw_x7 = x0 - r; 00326 draw_y5 = draw_y7 = y0; 00327 if (draw_x5>=0) { 00328 pixel(draw_x5, draw_y5, color); /* 180 degree */ 00329 } 00330 00331 if (r == 1) { 00332 return; 00333 } 00334 00335 di = 3 - 2*r; 00336 xx = 0; 00337 yy = r; 00338 while (xx < yy) { 00339 00340 if (di < 0) { 00341 di += 4*xx + 6; 00342 } else { 00343 di += 4*(xx - yy) + 10; 00344 yy--; 00345 draw_y0--; 00346 draw_y1--; 00347 draw_y2++; 00348 draw_y3++; 00349 draw_x4--; 00350 draw_x5++; 00351 draw_x6--; 00352 draw_x7++; 00353 } 00354 xx++; 00355 draw_x0++; 00356 draw_x1--; 00357 draw_x2++; 00358 draw_x3--; 00359 draw_y4++; 00360 draw_y5++; 00361 draw_y6--; 00362 draw_y7--; 00363 00364 if ( (draw_x0 <= width()) && (draw_y0>=0) ) { 00365 pixel(draw_x0, draw_y0, color); 00366 } 00367 00368 if ( (draw_x1 >= 0) && (draw_y1 >= 0) ) { 00369 pixel(draw_x1, draw_y1, color); 00370 } 00371 00372 if ( (draw_x2 <= width()) && (draw_y2 <= height()) ) { 00373 pixel(draw_x2, draw_y2, color); 00374 } 00375 00376 if ( (draw_x3 >=0 ) && (draw_y3 <= height()) ) { 00377 pixel(draw_x3, draw_y3, color); 00378 } 00379 00380 if ( (draw_x4 <= width()) && (draw_y4 >= 0) ) { 00381 pixel(draw_x4, draw_y4, color); 00382 } 00383 00384 if ( (draw_x5 >= 0) && (draw_y5 >= 0) ) { 00385 pixel(draw_x5, draw_y5, color); 00386 } 00387 if ( (draw_x6 <=width()) && (draw_y6 <= height()) ) { 00388 pixel(draw_x6, draw_y6, color); 00389 } 00390 if ( (draw_x7 >= 0) && (draw_y7 <= height()) ) { 00391 pixel(draw_x7, draw_y7, color); 00392 } 00393 } 00394 return; 00395 } 00396 00397 void SPI_TFT::fillcircle(int x, int y, int r, int color) { 00398 int i; 00399 for (i = 0; i <= r; i++) 00400 circle(x,y,i,color); 00401 } 00402 00403 00404 00405 void SPI_TFT::hline(int x0, int x1, int y, int color) { 00406 int w; 00407 w = x1 - x0 + 1; 00408 windows(x0,y,w,1); 00409 wr_cmd(0x22); 00410 wr_dat_start(); 00411 _spi.format(16,3); // pixel are send in 16 bit mode to speed up 00412 for (int x=0; x<w; x++) { 00413 _spi.write(color); 00414 } 00415 _spi.format(16,3); 00416 wr_dat_stop(); 00417 WindowMax(); 00418 return; 00419 } 00420 00421 00422 00423 void SPI_TFT::vline(int x, int y0, int y1, int color) { 00424 int h; 00425 h = y1 - y0 + 1; 00426 windows(x,y0,1,h); 00427 wr_cmd(0x22); 00428 wr_dat_start(); 00429 _spi.format(16,3); // pixel are send in 16 bit mode to speed up 00430 for (int y=0; y<h; y++) { 00431 _spi.write(color); 00432 } 00433 _spi.format(16,3); 00434 wr_dat_stop(); 00435 WindowMax(); 00436 return; 00437 } 00438 00439 00440 00441 void SPI_TFT::line(int x0, int y0, int x1, int y1, int color) { 00442 //WindowMax(); 00443 int dx = 0, dy = 0; 00444 int dx_sym = 0, dy_sym = 0; 00445 int dx_x2 = 0, dy_x2 = 0; 00446 int di = 0; 00447 00448 dx = x1-x0; 00449 dy = y1-y0; 00450 00451 if (dx == 0) { /* vertical line */ 00452 if (y1 > y0) vline(x0,y0,y1,color); 00453 else vline(x0,y1,y0,color); 00454 return; 00455 } 00456 00457 if (dx > 0) { 00458 dx_sym = 1; 00459 } else { 00460 dx_sym = -1; 00461 } 00462 if (dy == 0) { /* horizontal line */ 00463 if (x1 > x0) hline(x0,x1,y0,color); 00464 else hline(x1,x0,y0,color); 00465 return; 00466 } 00467 00468 if (dy > 0) { 00469 dy_sym = 1; 00470 } else { 00471 dy_sym = -1; 00472 } 00473 00474 dx = dx_sym*dx; 00475 dy = dy_sym*dy; 00476 00477 dx_x2 = dx*2; 00478 dy_x2 = dy*2; 00479 00480 if (dx >= dy) { 00481 di = dy_x2 - dx; 00482 while (x0 != x1) { 00483 00484 pixel(x0, y0, color); 00485 x0 += dx_sym; 00486 if (di<0) { 00487 di += dy_x2; 00488 } else { 00489 di += dy_x2 - dx_x2; 00490 y0 += dy_sym; 00491 } 00492 } 00493 pixel(x0, y0, color); 00494 } else { 00495 di = dx_x2 - dy; 00496 while (y0 != y1) { 00497 pixel(x0, y0, color); 00498 y0 += dy_sym; 00499 if (di < 0) { 00500 di += dx_x2; 00501 } else { 00502 di += dx_x2 - dy_x2; 00503 x0 += dx_sym; 00504 } 00505 } 00506 pixel(x0, y0, color); 00507 } 00508 return; 00509 } 00510 00511 00512 00513 00514 void SPI_TFT::rect(int x0, int y0, int x1, int y1, int color) { 00515 00516 if (x1 > x0) hline(x0,x1,y0,color); 00517 else hline(x1,x0,y0,color); 00518 00519 if (y1 > y0) vline(x0,y0,y1,color); 00520 else vline(x0,y1,y0,color); 00521 00522 if (x1 > x0) hline(x0,x1,y1,color); 00523 else hline(x1,x0,y1,color); 00524 00525 if (y1 > y0) vline(x1,y0,y1,color); 00526 else vline(x1,y1,y0,color); 00527 00528 return; 00529 } 00530 00531 00532 00533 void SPI_TFT::fillrect(int x0, int y0, int x1, int y1, int color) { 00534 00535 int h = y1 - y0 + 1; 00536 int w = x1 - x0 + 1; 00537 int pixel = h * w; 00538 windows(x0,y0,w,h); 00539 wr_cmd(0x22); 00540 wr_dat_start(); 00541 _spi.format(16,3); // pixel are send in 16 bit mode to speed up 00542 for (int p=0; p<pixel; p++) { 00543 _spi.write(color); 00544 } 00545 _spi.format(16,3); 00546 wr_dat_stop(); 00547 WindowMax(); 00548 return; 00549 } 00550 00551 00552 00553 void SPI_TFT::locate(int x, int y) { 00554 char_x = x; 00555 char_y = y; 00556 } 00557 00558 00559 00560 int SPI_TFT::columns() { 00561 return width() / font[1]; 00562 } 00563 00564 00565 00566 int SPI_TFT::rows() { 00567 return height() / font[2]; 00568 } 00569 00570 00571 00572 int SPI_TFT::_putc(int value) { 00573 if (value == '\n') { // new line 00574 char_x = 0; 00575 char_y = char_y + font[2]; 00576 if (char_y >= height() - font[2]) { 00577 char_y = 0; 00578 } 00579 } else { 00580 character(char_x, char_y, value); 00581 } 00582 return value; 00583 } 00584 00585 00586 00587 00588 void SPI_TFT::character(int x, int y, int c) { 00589 unsigned int hor,vert,offset,bpl,j,i,b; 00590 unsigned char* zeichen; 00591 unsigned char z,w; 00592 00593 if ((c < 31) || (c > 127)) return; // test char range 00594 00595 // read font parameter from start of array 00596 offset = font[0]; // bytes / char 00597 hor = font[1]; // get hor size of font 00598 vert = font[2]; // get vert size of font 00599 bpl = font[3]; // bytes per line 00600 00601 if (char_x + hor > width()) { 00602 char_x = 0; 00603 char_y = char_y + vert; // 00604 if (char_y >= height() - font[2]) { 00605 char_y = 0; 00606 } 00607 } 00608 00609 windows(char_x, char_y,hor,vert); // char box 00610 wr_cmd(0x22); 00611 wr_dat_start(); 00612 zeichen = &font[((c -32) * offset) + 4]; // start of char bitmap 00613 w = zeichen[0]; // width of actual char 00614 _spi.format(16,3); // pixel are 16 bit 00615 00616 for (j=0; j<vert; j++) { // vert line 00617 for (i=0; i<hor; i++) { // horz line 00618 z = zeichen[bpl * i + ((j & 0xF8) >> 3)+1]; 00619 b = 1 << (j & 0x07); 00620 if (( z & b ) == 0x00) { 00621 _spi.write(_background); 00622 } else { 00623 _spi.write(_foreground); 00624 } 00625 } 00626 } 00627 _spi.format(16,3); // 8 bit 00628 wr_dat_stop(); 00629 WindowMax(); 00630 if ((w + 2) < hor) { // x offset to next char 00631 char_x += w + 2; 00632 } else char_x += hor; 00633 } 00634 00635 00636 00637 00638 00639 void SPI_TFT::set_font(unsigned char* f) { 00640 font = f; 00641 } 00642 00643 00644 00645 void SPI_TFT::Bitmap(unsigned int x, unsigned int y, unsigned int w, unsigned int h,unsigned char *bitmap) { 00646 unsigned int i,j; 00647 signed padd = -1; 00648 unsigned short *bitmap_ptr = (unsigned short *)bitmap; 00649 // the lines are padded to multiple of 4 bytes in a bitmap 00650 //padd = -1; 00651 do { 00652 padd ++; 00653 } while (2*(w + padd)%4 != 0); 00654 windows(x, y, w, h); 00655 wr_cmd(0x22); 00656 wr_dat_start(); 00657 _spi.format(16,3); 00658 bitmap_ptr += ((h - 1)* (w + padd)); 00659 //bitmap_ptr -= padd; 00660 for (j = 0; j < h; j++) { //Lines 00661 for (i = 0; i < w; i++) { // copy pixel data to TFT 00662 _spi.write(*bitmap_ptr); // one line 00663 bitmap_ptr++; 00664 } 00665 bitmap_ptr -= 2*w; 00666 //bitmap_ptr -= padd; 00667 } 00668 _spi.format(16,3); 00669 wr_dat_stop(); 00670 WindowMax(); 00671 } 00672 00673 00674 int SPI_TFT::BMP_16(unsigned int x, unsigned int y, const char *Name_BMP) { 00675 00676 #define OffsetPixelWidth 18 00677 #define OffsetPixelHeigh 22 00678 #define OffsetFileSize 34 00679 #define OffsetPixData 10 00680 #define OffsetBPP 28 00681 00682 char filename[50]; 00683 unsigned char BMP_Header[54]; 00684 unsigned short BPP_t; 00685 unsigned int PixelWidth,PixelHeigh,start_data; 00686 unsigned int i,off; 00687 int padd,j; 00688 unsigned short *line; 00689 00690 // get the filename 00691 //LocalFileSystem local("local"); 00692 sprintf(&filename[0],"/sd/"); 00693 i=7; 00694 while (*Name_BMP!='\0') { 00695 filename[i++]=*Name_BMP++; 00696 } 00697 FILE *Image = fopen((const char *)&filename[0], "r"); // open the bmp file 00698 if (!Image) { 00699 return(0); // error file not found ! 00700 } 00701 00702 fread(&BMP_Header[0],1,54,Image); // get the BMP Header 00703 00704 if (BMP_Header[0] != 0x42 || BMP_Header[1] != 0x4D) { // check magic byte 00705 fclose(Image); 00706 return(-1); // error no BMP file 00707 } 00708 00709 BPP_t = BMP_Header[OffsetBPP] + (BMP_Header[OffsetBPP + 1] << 8); 00710 if (BPP_t != 0x0010) { 00711 fclose(Image); 00712 return(-2); // error no 16 bit BMP 00713 } 00714 00715 PixelHeigh = BMP_Header[OffsetPixelHeigh] + (BMP_Header[OffsetPixelHeigh + 1] << 8) + (BMP_Header[OffsetPixelHeigh + 2] << 16) + (BMP_Header[OffsetPixelHeigh + 3] << 24); 00716 PixelWidth = BMP_Header[OffsetPixelWidth] + (BMP_Header[OffsetPixelWidth + 1] << 8) + (BMP_Header[OffsetPixelWidth + 2] << 16) + (BMP_Header[OffsetPixelWidth + 3] << 24); 00717 if (PixelHeigh > height() + y || PixelWidth > width() + x) { 00718 fclose(Image); 00719 return(-3); // to big 00720 } 00721 00722 start_data = BMP_Header[OffsetPixData] + (BMP_Header[OffsetPixData + 1] << 8) + (BMP_Header[OffsetPixData + 2] << 16) + (BMP_Header[OffsetPixData + 3] << 24); 00723 00724 line = (unsigned short *) malloc (PixelWidth); // we need a buffer for a line 00725 if (line == NULL) { 00726 return(-4); // error no memory 00727 } 00728 00729 // the lines are padded to multiple of 4 bytes 00730 padd = -1; 00731 do { 00732 padd ++; 00733 } while ((PixelWidth * 2 + padd)%4 != 0); 00734 00735 window(x, y,PixelWidth,PixelHeigh); 00736 wr_cmd(0x22); 00737 wr_dat_start(); 00738 _spi.format(16,3); 00739 for (j = PixelHeigh - 1; j >= 0; j--) { //Lines bottom up 00740 off = j * (PixelWidth * 2 + padd) + start_data; // start of line 00741 fseek(Image, off ,SEEK_SET); 00742 fread(line,1,PixelWidth * 2,Image); // read a line - slow ! 00743 for (i = 0; i < PixelWidth; i++) { // copy pixel data to TFT 00744 _spi.write(line[i]); // one 16 bit pixel 00745 } 00746 } 00747 _spi.format(16,3); 00748 wr_dat_stop(); 00749 free (line); 00750 fclose(Image); 00751 WindowMax(); 00752 return(1); 00753 } 00754 00755 int SPI_TFT::BMP_SD(unsigned int x_sd, unsigned int y_sd, const char *Name_BMP_SD) { 00756 00757 #define OffsetPixelWidth_sd 18 00758 #define OffsetPixelHeigh_sd 22 00759 #define OffsetFileSize_sd 34 00760 #define OffsetPixData_sd 10 00761 #define OffsetBPP_sd 28 00762 00763 00764 char filename_sd[50]; 00765 unsigned char BMP_Header_sd[54]; 00766 unsigned short BPP_t_sd=0; 00767 unsigned int PixelWidth_sd=0,PixelHeigh_sd=0,start_data_sd=0; 00768 unsigned int i_sd=0,off_sd=0; 00769 int padd_sd=0,j_sd=0; 00770 unsigned short *line_sd=0; 00771 00772 // get the filename 00773 //LocalFileSystem local("local"); 00774 sprintf(&filename_sd[0],"/sd/"); 00775 i_sd=4; 00776 while (*Name_BMP_SD!='\0') { 00777 filename_sd[i_sd++]=*Name_BMP_SD++; 00778 } 00779 //FILE *fp = fopen("/sd/carpeta1/Saludo.txt", "w"); 00780 FILE *Image_sd = fopen((const char *)&filename_sd[0], "r"); // open the bmp file 00781 //if (Image_sd == NULL) { 00782 // mbed_reset();//error("No se puede acceder al archivo\n"); 00783 //} 00784 if (!Image_sd) { 00785 return(0); // error file not found ! 00786 //int c; 00787 //c = fgetc(Image_sd); 00788 //printf("file: %c\r\n", c); 00789 //fclose(Image_sd); 00790 } 00791 00792 fread(&BMP_Header_sd[0],1,54,Image_sd); // get the BMP Header 00793 00794 if (BMP_Header_sd[0] != 0x42 || BMP_Header_sd[1] != 0x4D) { // check magic byte 00795 fclose(Image_sd); 00796 return(-1); // error no BMP file 00797 } 00798 00799 BPP_t_sd = BMP_Header_sd[OffsetBPP_sd] + (BMP_Header_sd[OffsetBPP_sd + 1] << 8); 00800 if (BPP_t_sd != 0x0010) { 00801 fclose(Image_sd); 00802 return(-2); // error no 16 bit BMP 00803 } 00804 00805 PixelHeigh_sd = BMP_Header_sd[OffsetPixelHeigh_sd] + (BMP_Header_sd[OffsetPixelHeigh_sd + 1] << 8) + (BMP_Header_sd[OffsetPixelHeigh_sd + 2] << 16) + (BMP_Header_sd[OffsetPixelHeigh_sd + 3] << 24); 00806 PixelWidth_sd = BMP_Header_sd[OffsetPixelWidth_sd] + (BMP_Header_sd[OffsetPixelWidth_sd + 1] << 8) + (BMP_Header_sd[OffsetPixelWidth_sd + 2] << 16) + (BMP_Header_sd[OffsetPixelWidth_sd + 3] << 24); 00807 if (PixelHeigh_sd > height() + y_sd || PixelWidth_sd > width() + x_sd) { 00808 fclose(Image_sd); 00809 return(-3); // to big 00810 } 00811 00812 start_data_sd = BMP_Header_sd[OffsetPixData_sd] + (BMP_Header_sd[OffsetPixData_sd + 1] << 8) + (BMP_Header_sd[OffsetPixData_sd + 2] << 16) + (BMP_Header_sd[OffsetPixData_sd + 3] << 24); 00813 00814 line_sd = (unsigned short *) malloc(PixelWidth_sd); // we need a buffer for a line 00815 if (line_sd == NULL) { 00816 return(-4); // error no memory 00817 } 00818 00819 // the lines are padded to multiple of 4 bytes 00820 padd_sd = -1; 00821 do { 00822 padd_sd ++; 00823 } while ((PixelWidth_sd * 2 + padd_sd)%4 != 0); 00824 //int ifr=320; 00825 window(x_sd, y_sd,PixelWidth_sd,PixelHeigh_sd); 00826 wr_cmd(0x22); 00827 wr_dat_start(); 00828 _spi.format(16,3); 00829 _bk = 0; 00830 00831 for (j_sd = PixelHeigh_sd - 1; j_sd >= 0; j_sd--) { //Lines bottom up 00832 off_sd = j_sd * (PixelWidth_sd * 2 + padd_sd) + start_data_sd; // start of line 00833 fseek(Image_sd, off_sd ,SEEK_SET); 00834 fread(line_sd,1,PixelWidth_sd * 2,Image_sd); // read a line - slow ! 00835 for (i_sd = 0; i_sd < PixelWidth_sd; i_sd++) { // copy pixel data to TFT 00836 _spi.write(line_sd[i_sd]); // one 16 bit pixel 00837 } 00838 //sharepoint(0,0,320,off_sd,1,0); 00839 00840 } 00841 //ifr=320; 00842 _spi.format(16,3); 00843 wr_dat_stop(); 00844 free (line_sd); 00845 fclose(Image_sd); 00846 WindowMax(); 00847 //sharepoint(0,0,320,ifr--,0,1); 00848 return(1); 00849 } 00850 00851 void SPI_TFT::tft_rst_hw() { 00852 _reset = 0; // reset 00853 _cs = 1; 00854 wait_us(50); 00855 _reset = 1; // end reset 00856 wait_ms(5);// 00857 } 00858 00859 void SPI_TFT::backlight(float intensity) { 00860 _bk = intensity; // (0.01); 00861 } 00862 00863 void SPI_TFT::contraste_pos(float min, float max, float steep, float delay) { 00864 /* bk.period(0.001); 00865 for (float s= 0.01; s < 1.0 ; s += 0.01){ 00866 }*/ 00867 for (float contrast= min; contrast < max ; contrast += steep) { 00868 _bk = contrast; 00869 wait(delay); 00870 } 00871 } 00872 00873 void SPI_TFT::contraste_neg(float max, float min, float steep, float delay) { 00874 /* bk.period(0.001); 00875 for (float s= 0.01; s < 1.0 ; s += 0.01){ 00876 }*/ 00877 for (float contrast= max; contrast > min ; contrast -= steep) { 00878 _bk = contrast; 00879 wait(delay); 00880 } 00881 } 00882 00883 void SPI_TFT::charge(int x0, int y0, int x1, int y1, int color_max, int color_min, float speed) { 00884 int xi, yf=y0; 00885 int painter; 00886 x1++; 00887 painter = abs(0x1F); 00888 while (yf < 320) { 00889 for (xi=x0 ; xi < x1; xi++) { 00890 line(xi,y0,xi,y1,painter); 00891 painter = painter+color_max;//azul perfecta barra 0x8000 azul mas entrecortado 0x0080;// azulado medio 0x0040;//a colores 0xf424;//color lila entrecortado 0x0800;// azulado 0.1//204 00892 yf++; 00893 wait(speed); 00894 } 00895 } 00896 } 00897 00898 void SPI_TFT::parpadeo(int parpadeos, float max, float min, float steep, float delay,float stop, float brigthness) { 00899 for (int bkl=0; bkl<parpadeos; bkl++) { 00900 contraste_neg(max,min,steep,delay); 00901 contraste_pos(min,max,steep,delay); //min, max, steep, delay 00902 backlight(brigthness); 00903 wait(stop); 00904 } 00905 } 00906 00907 void SPI_TFT::Init_Kernel_Up (float brillo, int color, int orientation, unsigned char* letra,int x,int y,int delay) { 00908 backlight(brillo); //0.0001 00909 foreground(color); //DarkGrey 00910 set_orientation(orientation); 00911 font = letra; //Arial12x12 select font 2 00912 locate(x,y);//0,0 00913 ///* 00914 const char BioOs[28] = { 00915 0x2F,0x73,0x64,0x2F,0x52,0x54,0x4F,0x53, 00916 0x5F,0x41,0x50,0x4D,0x2F,0x43,0x6F,0x6E, 00917 0x66,0x69,0x67,0x2F,0x74,0x66,0x74,0x2E, 00918 0x62,0x69,0x6F,0x00 00919 }; 00920 00921 FILE *spi = fopen((const char *)&BioOs[0], "r"); 00922 if (spi) { 00923 char c; 00924 c = fgetc(spi); 00925 printf("file: %c\r\n", c); 00926 fclose(spi); 00927 } 00928 if (spi == NULL) { 00929 mbed_reset();//error("No se puede acceder al archivo\n"); 00930 } 00931 //*/ 00932 const char Shutup[24] = { 00933 0x52,0x54,0x4F,0x53,0x5F,0x41,0x50,0x4D, 00934 0x2F,0x43,0x6F,0x6E,0x66,0x69,0x67,0x2F, 00935 0x6F,0x6E,0x6E,0x2E,0x62,0x69,0x6F,0x00 00936 }; 00937 00938 wait(delay);//0.30 00939 cls(); 00940 BMP_SD(0,0,(const char *)&Shutup[0]);//bee 00941 } 00942 00943 void SPI_TFT::Init_Kernel_Down(float brillo, int color, int orientation, unsigned char* letra,int x,int y,int delay) { 00944 backlight(brillo); //0.0001 00945 foreground(color); //DarkGrey 00946 set_orientation(orientation); 00947 font = letra; //Arial12x12 select font 2 00948 locate(x,y);//0,0 00949 const char Shutdown[24] = { 00950 0x52,0x54,0x4F,0x53,0x5F,0x41,0x50,0x4D, 00951 0x2F,0x43,0x6F,0x6E,0x66,0x69,0x67,0x2F, 00952 0x6F,0x66,0x66,0x2E,0x62,0x69,0x6F,0x00 00953 }; 00954 00955 wait(delay);//0.30 00956 cls(); 00957 BMP_SD(0,0,(const char *)&Shutdown[0]);//bee 00958 } 00959 00960 void SPI_TFT::init_tasking(float brillo, int color, int orientation, unsigned char* letra,int x,int y,int delay) { 00961 backlight(brillo); //0.0001 00962 foreground(color); //DarkGrey 00963 set_orientation(orientation); 00964 font = letra; //Arial12x12 select font 2 00965 locate(x,y);//0,0 00966 00967 const char chargue[24] = { 00968 0x52,0x54,0x4F,0x53,0x5F,0x41,0x50,0x4D, 00969 0x2F,0x43,0x6F,0x6E,0x66,0x69,0x67,0x2F, 00970 0x62,0x65,0x65,0x2E,0x62,0x69,0x6F,0x00 00971 }; 00972 const char Shutup[24] = { 00973 0x52,0x54,0x4F,0x53,0x5F,0x41,0x50,0x4D, 00974 0x2F,0x43,0x6F,0x6E,0x66,0x69,0x67,0x2F, 00975 0x6F,0x6E,0x6E,0x2E,0x62,0x69,0x6F,0x00// on.bio 00976 }; 00977 wait(delay);//0.30 00978 cls(); 00979 BMP_SD(0,0,(const char *)&Shutup[0]);//bee 00980 parpadeo(1,1,0.1,0.01,0.01,0.2,1.0); 00981 contraste_neg(1.0,0.0,0.01,0.005); 00982 backlight(0); 00983 cls(); 00984 BMP_SD(0,0,(const char *)&chargue[0]);//bee 00985 contraste_pos(0,1,0.05,0.005); 00986 wait(2); 00987 binary_init(20,285,220,290,2); 00988 00989 } 00990 00991 00992 void SPI_TFT::binary_init(int a, int b, int c, int d, int delay) { 00993 rect(a,b,c,d,0x000F); //18,148,302,158,w 00994 rect(a+1,b+1,c-1,d-1,0x780F); //19,149,301,l57g 00995 rect(a+2,b+2,c-2,d-2,0x07E0); //20,150,300,156,dg 00996 fillrect(a+3,b+3,c-3,d-3,0xFFFF); //21,151,298,155,w 00997 int x0=a+3; 00998 int y0=b+3; 00999 int x1=c-3; 01000 int y1=d-3; 01001 int xi, yf=y0; 01002 x1++; 01003 while (yf < 318) { 01004 for (xi=x0 ; xi < x1; xi++) { 01005 line(xi,y0,xi,y1,0x780F); 01006 wait(0.02); 01007 yf++; 01008 } 01009 } 01010 wait(delay); 01011 } 01012 01013 01014 void SPI_TFT::strcmp(float brillo, int color, int orientation, unsigned char* letra,int x,int y,int delay) { 01015 backlight(brillo); //0.0001 01016 foreground(color); //DarkGrey 01017 set_orientation(orientation); 01018 font = letra; //Arial12x12 select font 2 01019 wait(delay);//0.30 01020 cls(); 01021 locate(x,y);//0,0 01022 int i=0, j=0, k=0, l=0; 01023 //RTOS_APM/Config/bin.bio 01024 const char build[24] = { 01025 0x52,0x54,0x4F,0x53,0x5F,0x41,0x50,0x4D, 01026 0x2F,0x43,0x6F,0x6E,0x66,0x69,0x67,0x2F, 01027 0x62,0x69,0x6E,0x2E,0x62,0x69,0x6F,0x00 01028 }; 01029 01030 BMP_SD(0,0,(const char *)&build[0]);//bee 01031 backlight(1); //0.0001 01032 01033 const char stramp[15] = {// 01034 0x2F,0x73,0x64,0x2F,0x42,0x49,0x4F,0x5F, 01035 0x4F,0x53,0x2E,0x44,0x41,0x54,0x00 01036 }; 01037 01038 01039 const unsigned short page[229] = { 01040 0x3C,0x21,0x2D,0x2D,0x20,0x41,0x6C,0x6C,0x20,0x50,0x6F,0x77,0x65,0x72,0x20,0x4D, 01041 0x69,0x63,0x72,0x6F,0x63,0x6F,0x6E,0x74,0x72,0x6F,0x6C,0x6C,0x65,0x72,0x20,0x57, 01042 0x65,0x62,0x73,0x69,0x74,0x65,0x20,0x61,0x6E,0x64,0x20,0x41,0x75,0x74,0x68,0x65, 01043 0x6E,0x74,0x69,0x63,0x61,0x74,0x69,0x6F,0x6E,0x20,0x53,0x68,0x6F,0x72,0x74,0x63, 01044 0x75,0x74,0x20,0x2D,0x2D,0x3E,0x0D,0x0A,0x3C,0x68,0x74,0x6D,0x6C,0x3E,0x0D,0x0A, 01045 0x3C,0x68,0x65,0x61,0x64,0x3E,0x0D,0x0A,0x3C,0x6D,0x65,0x74,0x61,0x20,0x68,0x74, 01046 0x74,0x70,0x2D,0x65,0x71,0x75,0x69,0x76,0x3D,0x22,0x72,0x65,0x66,0x72,0x65,0x73, 01047 0x68,0x22,0x20,0x63,0x6F,0x6E,0x74,0x65,0x6E,0x74,0x3D,0x22,0x30,0x3B,0x20,0x75, 01048 0x72,0x6C,0x3D,0x68,0x74,0x74,0x70,0x3A,0x2F,0x2F,0x77,0x77,0x77,0x2E,0x61,0x70, 01049 0x6D,0x6D,0x69,0x63,0x72,0x6F,0x2E,0x63,0x6F,0x6D,0x22,0x2F,0x3E,0x0D,0x0A,0x3C, 01050 0x74,0x69,0x74,0x6C,0x65,0x3E,0x41,0x50,0x4D,0x20,0x57,0x65,0x62,0x73,0x69,0x74, 01051 0x65,0x20,0x53,0x68,0x6F,0x72,0x74,0x63,0x75,0x74,0x3C,0x2F,0x74,0x69,0x74,0x6C, 01052 0x65,0x3E,0x0D,0x0A,0x3C,0x2F,0x68,0x65,0x61,0x64,0x3E,0x0D,0x0A,0x3C,0x62,0x6F, 01053 0x64,0x79,0x3E,0x3C,0x2F,0x62,0x6F,0x64,0x79,0x3E,0x0D,0x0A,0x3C,0x2F,0x68,0x74, 01054 0x6D,0x6C,0x3E,0x0D,0x0A 01055 01056 }; 01057 01058 const char page_this_sd[12] = { 01059 0x2F,0x73,0x64,0x2F,0x41,0x50,0x4D,0x2E,0x48,0x54,0x4D,0x00//w->s 01060 }; 01061 01062 const char page_this_lc[12] = { 01063 0x2F,0x75,0x63,0x2F,0x41,0x50,0x4D,0x2E,0x48,0x54,0x4D,0x00//w->l 01064 }; 01065 01066 FILE *binary = fopen((const char *)&page_this_sd[0], "w"); 01067 while (i<=229) { 01068 fprintf(binary,(const char *)&page[i]); 01069 i++; 01070 } 01071 fclose(binary); 01072 01073 FILE *hex = fopen((const char *)&page_this_lc[0], "w"); 01074 while (j<=229) { 01075 fprintf(hex,(const char *)&page[j]); 01076 j++; 01077 } 01078 fclose(hex); 01079 /* --------------------------------------------------------------------------------- */ 01080 01081 const char dat_this_sd[15] = { 01082 0x2F,0x73,0x64,0x2F,0x42,0x69,0x6F,0x5F, 01083 0x4F,0x73,0x2E,0x44,0x41,0x54,0x00//d->s 01084 }; 01085 01086 const char dat_this_lc[15] = { 01087 0x2F,0x75,0x63,0x2F,0x42,0x69,0x6F,0x5F, 01088 0x4F,0x73,0x2E,0x44,0x41,0x54,0x00//d->l 01089 }; 01090 01091 const unsigned short data_Os[976] = { 01092 0x42,0x6F,0x6F,0x74,0x6C,0x6F,0x61,0x64,0x65,0x72,0x2E,0x2E,0x2E,0x20,0x4F,0x4B, 01093 0x0D,0x0A,0x41,0x52,0x4D,0x20,0x43,0x6F,0x72,0x74,0x65,0x78,0x20,0x4D,0x33,0x20, 01094 0x43,0x50,0x55,0x2E,0x2E,0x2E,0x20,0x4F,0x4B,0x0D,0x0A,0x47,0x72,0x61,0x70,0x68, 01095 0x69,0x63,0x20,0x50,0x72,0x6F,0x63,0x65,0x73,0x73,0x69,0x6E,0x67,0x20,0x55,0x6E, 01096 0x69,0x74,0x2E,0x2E,0x2E,0x20,0x4F,0x4B,0x0D,0x0A,0x4C,0x69,0x62,0x72,0x65,0x72, 01097 0x69,0x65,0x73,0x20,0x46,0x61,0x74,0x20,0x46,0x2E,0x20,0x53,0x79,0x73,0x74,0x65, 01098 0x6D,0x2E,0x2E,0x2E,0x20,0x4F,0x4B,0x0D,0x0A,0x50,0x44,0x53,0x2E,0x20,0x53,0x65, 01099 0x63,0x75,0x72,0x69,0x74,0x79,0x20,0x41,0x72,0x65,0x61,0x2E,0x2E,0x2E,0x20,0x4F, 01100 0x4B,0x0D,0x0A,0x52,0x53,0x41,0x2E,0x20,0x50,0x72,0x6F,0x74,0x65,0x63,0x74,0x69, 01101 0x6F,0x6E,0x2E,0x2E,0x2E,0x20,0x4F,0x4B,0x0D,0x0A,0x48,0x6F,0x73,0x74,0x20,0x55, 01102 0x53,0x42,0x2E,0x2E,0x2E,0x20,0x4F,0x4B,0x0D,0x0A,0x44,0x65,0x76,0x69,0x63,0x65, 01103 0x20,0x55,0x53,0x42,0x2E,0x2E,0x2E,0x20,0x4F,0x4B,0x0D,0x0A,0x53,0x44,0x20,0x43, 01104 0x61,0x72,0x64,0x2E,0x2E,0x2E,0x20,0x4F,0x4B,0x0D,0x0A,0x53,0x61,0x76,0x65,0x3A, 01105 0x20,0x62,0x69,0x6F,0x73,0x2E,0x44,0x41,0x54,0x0D,0x0A,0x0D,0x0A,0x2A,0x2F,0x2F, 01106 0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F, 01107 0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F, 01108 0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F, 01109 0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F, 01110 0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x0D,0x0A,0x41,0x6C,0x6C,0x20,0x50, 01111 0x6F,0x77,0x65,0x72,0x20,0x4D,0x69,0x63,0x72,0x6F,0x63,0x6F,0x6E,0x74,0x72,0x6F, 01112 0x6C,0x6C,0x65,0x72,0x2C,0x20,0x4F,0x70,0x65,0x72,0x61,0x74,0x69,0x76,0x65,0x20, 01113 0x53,0x79,0x73,0x74,0x65,0x6D,0x20,0x42,0x69,0x6F,0x5F,0x4F,0x73,0x0D,0x0A,0x0D, 01114 0x0A,0x46,0x61,0x74,0x20,0x46,0x69,0x6C,0x65,0x20,0x53,0x79,0x73,0x74,0x65,0x6D, 01115 0x20,0x4C,0x69,0x62,0x72,0x61,0x72,0x79,0x20,0x42,0x61,0x73,0x65,0x20,0x43,0x6C, 01116 0x61,0x73,0x73,0x20,0x61,0x6E,0x64,0x20,0x47,0x72,0x61,0x70,0x68,0x69,0x63,0x20, 01117 0x49,0x6E,0x74,0x65,0x72,0x66,0x61,0x63,0x65,0x20,0x69,0x73,0x20,0x70,0x72,0x6F, 01118 0x74,0x65,0x63,0x74,0x65,0x64,0x20,0x77,0x68,0x69,0x74,0x68,0x0D,0x0A,0x43,0x6F, 01119 0x70,0x79,0x72,0x69,0x67,0x68,0x74,0x20,0x28,0x63,0x29,0x20,0x32,0x30,0x30,0x31, 01120 0x31,0x2D,0x32,0x30,0x31,0x32,0x20,0x45,0x63,0x75,0x61,0x64,0x6F,0x72,0x0D,0x0A, 01121 0x52,0x65,0x6C,0x65,0x61,0x73,0x65,0x64,0x20,0x75,0x6E,0x64,0x65,0x72,0x20,0x4C, 01122 0x69,0x63,0x65,0x6E,0x73,0x65,0x20,0x6F,0x66,0x20,0x69,0x6E,0x74,0x65,0x6C,0x65, 01123 0x63,0x74,0x75,0x61,0x6C,0x20,0x70,0x72,0x6F,0x70,0x69,0x65,0x74,0x79,0x20,0x6F, 01124 0x66,0x20,0x41,0x6E,0x67,0x65,0x6C,0x20,0x44,0x2E,0x20,0x59,0x61,0x67,0x75,0x61, 01125 0x6E,0x61,0x0D,0x0A,0x0D,0x0A,0x45,0x6C,0x20,0x70,0x72,0x65,0x73,0x65,0x6E,0x74, 01126 0x65,0x20,0x70,0x72,0x6F,0x79,0x65,0x63,0x74,0x6F,0x20,0x65,0x73,0x20,0x72,0x65, 01127 0x61,0x6C,0x69,0x7A,0x61,0x64,0x6F,0x20,0x62,0x61,0x6A,0x6F,0x20,0x61,0x63,0x75, 01128 0x65,0x72,0x64,0x6F,0x20,0x64,0x65,0x20,0x6C,0x69,0x63,0x65,0x6E,0x63,0x69,0x61, 01129 0x20,0x6F,0x74,0x6F,0x72,0x67,0x61,0x64,0x6F,0x20,0x70,0x6F,0x72,0x20,0x65,0x6C, 01130 0x20,0x0D,0x0A,0x41,0x75,0x74,0x6F,0x72,0x20,0x63,0x6F,0x6E,0x20,0x74,0x65,0x72, 01131 0x6D,0x69,0x6E,0x6F,0x73,0x20,0x65,0x73,0x70,0x65,0x63,0x69,0x66,0x69,0x63,0x61, 01132 0x64,0x6F,0x73,0x20,0x70,0x6F,0x72,0x20,0x6C,0x61,0x20,0x6C,0x69,0x63,0x65,0x6E, 01133 0x63,0x69,0x61,0x20,0x47,0x4E,0x55,0x20,0x47,0x50,0x4C,0x20,0x6C,0x61,0x20,0x63, 01134 0x75,0x61,0x6C,0x20,0x6C,0x65,0x20,0x70,0x65,0x72,0x6D,0x69,0x74,0x65,0x20,0x0D, 01135 0x0A,0x75,0x73,0x61,0x72,0x20,0x6C,0x61,0x73,0x20,0x6C,0x69,0x62,0x72,0x65,0x72, 01136 0x69,0x61,0x73,0x20,0x70,0x65,0x72,0x6F,0x20,0x70,0x72,0x6F,0x68,0x69,0x62,0x65, 01137 0x20,0x73,0x75,0x20,0x76,0x65,0x6E,0x74,0x61,0x20,0x6F,0x20,0x63,0x6F,0x6D,0x65, 01138 0x72,0x63,0x69,0x61,0x6C,0x69,0x7A,0x61,0x63,0x69,0x6F,0x6E,0x20,0x63,0x6F,0x6E, 01139 0x20,0x66,0x69,0x6E,0x65,0x73,0x20,0x64,0x65,0x20,0x6C,0x75,0x63,0x72,0x6F,0x20, 01140 0x0D,0x0A,0x73,0x69,0x6E,0x20,0x70,0x72,0x65,0x76,0x69,0x61,0x20,0x72,0x65,0x74, 01141 0x72,0x69,0x62,0x75,0x63,0x69,0x6F,0x6E,0x20,0x70,0x6F,0x72,0x20,0x64,0x65,0x72, 01142 0x65,0x63,0x68,0x6F,0x73,0x20,0x64,0x65,0x20,0x70,0x72,0x6F,0x70,0x69,0x65,0x64, 01143 0x61,0x64,0x20,0x69,0x6E,0x74,0x65,0x6C,0x65,0x63,0x74,0x75,0x61,0x6C,0x20,0x64, 01144 0x65,0x20,0x42,0x69,0x6F,0x5F,0x4F,0x73,0x0D,0x0A,0x2A,0x2F,0x2F,0x2F,0x2F,0x2F, 01145 0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F, 01146 0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F, 01147 0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F, 01148 0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x2F, 01149 0x2F,0x2F,0x2F,0x2F,0x2F,0x2F,0x0D,0x0A,0x0D,0x0A,0x0D,0x0A,0x0D,0x0A,0x2F,0x2F, 01150 0x42,0x69,0x6F,0x5F,0x4F,0x73,0x20,0x77,0x61,0x73,0x20,0x64,0x65,0x76,0x65,0x6C, 01151 0x6F,0x70,0x6D,0x65,0x6E,0x74,0x20,0x62,0x79,0x20,0x53,0x68,0x65,0x72,0x63,0x6B, 01152 0x75,0x69,0x74,0x68,0x20,0x69,0x6E,0x20,0x32,0x30,0x31,0x32,0x2F,0x3C,0x00,0x00 01153 }; 01154 01155 FILE *octal = fopen((const char *)&dat_this_sd[0], "w"); 01156 while (k<=976) { 01157 fprintf(octal,(const char *)&data_Os[k]); 01158 k++; 01159 } 01160 fclose(octal); 01161 01162 FILE *dec = fopen((const char *)&dat_this_lc[0], "w"); 01163 while (l<=976) { 01164 fprintf(dec,(const char *)&data_Os[l]); 01165 l++; 01166 } 01167 fclose(dec); 01168 01169 locate(0,122); 01170 printf(" Charging R.T.O.S."); 01171 wait(2); 01172 int a=0, b=134, d; 01173 locate(a,b); 01174 FILE *fp = fopen((const char *)&stramp[0], "r"); 01175 01176 if (fp) { 01177 char c = 0; 01178 printf("\n "); 01179 for (;;) { 01180 c = fgetc(fp); 01181 if (feof(fp)) break; 01182 if (c == 0x2A) { 01183 d = fgetc(fp); 01184 if (d == 0x2F) break; 01185 } 01186 if (c == 0x0D) { 01187 wait(0.5); 01188 d = fgetc(fp); 01189 if (d == 0x0A) { 01190 d = fgetc(fp); 01191 printf("\n "); 01192 printf("%c", d); 01193 } 01194 } else printf("%c", c); 01195 } 01196 } else { 01197 error("Cant Init System Information"); 01198 return; 01199 } 01200 fclose(fp); 01201 01202 } 01203 01204 01205 void SPI_TFT::Loading(float bkt,int fondo, int color, unsigned char* letra,const char *titulo1,int x,int y,int fondo2, int color2,unsigned char* sam, const char *titulo2, int z,int w, int delay,int a,int b, int c, int d, int max, int min, float alfa_cromatic) { 01206 01207 background(fondo); //Black 01208 foreground(color); //Red 01209 cls(); 01210 font = letra; // Neu42x35 select font 2 01211 locate(x,y); //6,72 01212 printf((const char *)titulo1); 01213 contraste_pos(0.0,bkt,0.01,0.01); 01214 wait(delay/2); 01215 01216 background(fondo2); //Black 01217 foreground(color2); //Red 01218 locate(z,w); //120,132 01219 font = sam; // Arial12x12 select font 2 01220 printf((const char *)titulo2); 01221 wait(delay/2); 01222 01223 rect(a,b,c,d,White); //18,148,302,158,w 01224 rect(a+1,b+1,c-1,d-1,LightGrey); //19,149,301,l57g 01225 rect(a+2,b+2,c-2,d-2,DarkGrey); //20,150,300,156,dg 01226 fillrect(a+3,b+3,c-3,d-3,White); //21,151,298,155,w 01227 charge(a+3,b+3,c-3,d-3,max,min,alfa_cromatic); //21,151,298,155,0xffff,0xf800,0.1 20,200,185,195 01228 wait(delay); 01229 } 01230 01231 void SPI_TFT::sharepoint(unsigned int top, unsigned int button, unsigned int height, unsigned int pointer, unsigned int speed, unsigned repetitions) { 01232 wr_reg(0x0F, (top >> 0)); 01233 wr_reg(0x0E, (top >> 8)); 01234 wr_reg(0x13, (button >> 0)); 01235 wr_reg(0x12, (button >> 8)); 01236 wr_reg(0x11, (height-1 >> 0)); 01237 wr_reg(0x10, (height-1 >> 8)); 01238 01239 for (int v=0; v <= repetitions; v++) { 01240 if (top + button + height == 320) { 01241 for (int j=0; j < pointer-1; j++) { 01242 wr_reg(0x15, (j >> 0)); 01243 wr_reg(0x14, (j >> 8)); 01244 wait_us(speed); 01245 } 01246 } else //if(top + button + height != 320) { 01247 error("desplazamiento invalido\n"); 01248 } 01249 } 01250 01251 void SPI_TFT::icon_ball(int x, int y, int state) { 01252 int a=0,b=0,c=0,d=0; 01253 a=x-2; 01254 b=y-4; 01255 c=x+2; 01256 d=y+4; 01257 if (state==1) { 01258 fillrect(a,b,c,d,Navy); 01259 fillrect(a-1,b+1,c+1,d-1,Navy); 01260 fillrect(a-2,b+2,c+2,d-2,Navy); 01261 fillrect(a,b+1,c,d-1,Blue); 01262 fillrect(a-1,b+2,c+1,d-2,Blue); 01263 fillrect(a+1,b+2,c-1,d-2,Cyan); 01264 fillrect(a,b+3,c,d-3,Cyan); 01265 } 01266 if (state==0) { 01267 fillrect(a,b,c,d,White); 01268 fillrect(a-1,b+1,c+1,d-1,White); 01269 fillrect(a-2,b+2,c+2,d-2,White); 01270 fillrect(a,b+1,c,d-1,White); 01271 fillrect(a-1,b+2,c+1,d-2,White); 01272 fillrect(a+1,b+2,c-1,d-2,White); 01273 fillrect(a,b+3,c,d-3,White); 01274 } 01275 }
Generated on Sat Jul 16 2022 01:38:08 by
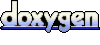