
Wrappper for VGAII demo by Jim Hamblem that includes a printf function that can print infinitely.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // 00002 // TFT_4DGL is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // TFT_4DGL is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // TFT_4DGL is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with TFT_4DGL. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "TFT_4DGL.h" 00021 #include <stdarg.h> 00022 #include <stdio.h> 00023 00024 // overwrite 4DGL library screen size settings in TFT_4DGL.h 00025 #define SIZE_X 479 00026 #define SIZE_Y 639 00027 // 00028 00029 TFT_4DGL ecran(p9,p10,p11); // serial tx, serial rx, reset pin; 00030 00031 00032 int outputrow = 1; 00033 int j = 0; 00034 char buffer[20480]; //working at 4608 00035 char printbuf[256]; 00036 int linenum=1; 00037 00038 void myprintf(const char* format, ...) { 00039 char dest[256]; 00040 if(j>80){ 00041 j=0; 00042 } 00043 va_list argptr; 00044 va_start(argptr, format); 00045 vsprintf(dest, format, argptr); 00046 va_end(argptr); 00047 for ( int i = 0; i < 256; i++) { 00048 buffer[256*j+i]= dest[i]; 00049 } 00050 j++; 00051 int linenum = j; 00052 00053 for (int k = 0; k <256; k++) { 00054 printbuf[k] = buffer[256*(linenum-1)+k]; 00055 } 00056 ecran.rectangle(0, 8*outputrow, 639, 8*outputrow+31, BLACK); 00057 ecran.text_string( dest, 0 , outputrow , FONT_8X8, WHITE); 00058 outputrow+= 4; 00059 if(outputrow>58){ 00060 outputrow =1; 00061 } 00062 } 00063 00064 00065 00066 int main() { 00067 // char s[500]; 00068 // int x = 0, y = 0, status, xc = 0, yc = 0; 00069 00070 ecran.baudrate(115200); 00071 // added - Set Display to 640 by 480 mode 00072 ecran.display_control(0x0c, 0x01); 00073 for(int i=0; i < 300; i++){ 00074 myprintf("This is line %i.Because printing to through the vga is slow, any prints off the screen will wrap around to the top of the screen and override the the existing text on the screen 1 line at a time. Therefore, the user has the ability to print infinitely.", i); 00075 } 00076 } 00077 00078 /* 00079 DigitalIn up(p22); 00080 DigitalIn down(p21); 00081 DigitalOut led1(LED1); 00082 DigitalOut led2(LED2); 00083 DigitalOut led3(LED3); 00084 DigitalOut led4(LED4); 00085 00086 void cursor() { 00087 DigitalIn up(p22); 00088 DigitalIn down(p21); 00089 DigitalOut led1(LED1); 00090 DigitalOut led2(LED2); 00091 DigitalOut led3(LED3); 00092 DigitalOut led4(LED4); 00093 while (1) { 00094 up.mode(PullUp); 00095 down.mode(PullUp); 00096 if (!up) { 00097 led1 = 1; 00098 if (linenum>0) { 00099 linenum--; 00100 for (int k = 0; k <256; k++) { 00101 printbuf[k] = buffer[256*(linenum-1)+k]; 00102 } 00103 ecran.rectangle(0, 0, 639, 59, BLACK); 00104 ecran.text_string( printbuf, 0 , 1 , FONT_8X8, WHITE); 00105 } 00106 } 00107 if (up) { 00108 led3 = 1; 00109 led1=0; 00110 } 00111 if (!down) { 00112 led2 = 1; 00113 if (linenum<j) { 00114 linenum++; 00115 for (int k = 0; k <256; k++) { 00116 printbuf[k] = buffer[256*(linenum-1)+k]; 00117 } 00118 ecran.rectangle(0, 0, 639, 59, BLACK); 00119 ecran.text_string( printbuf, 0 , 1 , FONT_8X8, WHITE); 00120 } 00121 } 00122 if (down) { 00123 led4 = 1; 00124 led2=0; 00125 } 00126 } 00127 } 00128 */
Generated on Fri Jul 15 2022 06:03:34 by
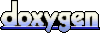