
nucleo_spi_max7219_led8x8
Fork of 06_spi_max7219_led8x8 by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* nucleo_spi_max7219_led8x8 00002 * 00003 * Simple demo to drive a 8x8-as LED matrix by a MAX7219 LED driver IC 00004 * After initialisation two characters (H and W) are displayed alternatively. 00005 * The MAX7219 IC is driven by hardware SPI: SPI0 module at PTD1, PTD2, PTD3. 00006 */ 00007 00008 #include "mbed.h" 00009 //#include "max7219.h" //此头文件未使用 00010 SPI spi(D11, D12, D13); // Arduino compatible MOSI, MISO, SCLK 00011 //nucleo MOSI, MISO, SCLK 00012 // PTD2, PTD3, PTD1 00013 DigitalOut cs(D10); // Chip select 00014 00015 const unsigned char led1[]= { 00016 0x0,0x66,0x99,0x81,0x42,0x24,0x18,0x0 00017 }; //心 00018 const unsigned char led2[]= { 00019 0x3C,0x24,0x24,0xFF,0xFF,0x24,0x24,0x3C 00020 }; //中 00021 00022 /// Send two bytes to SPI bus 00023 void SPI_Write2(unsigned char MSB, unsigned char LSB) 00024 { 00025 cs = 0; // Set CS Low 00026 spi.write(MSB); // Send two bytes 00027 spi.write(LSB); 00028 cs = 1; // Set CS High 00029 } 00030 00031 /// MAX7219 initialisation 00032 void Init_MAX7219(void) 00033 { 00034 SPI_Write2(0x09, 0x00); // Decoding off 00035 SPI_Write2(0x0A, 0x08); // Brightness to intermediate 00036 SPI_Write2(0x0B, 0x07); // Scan limit = 7 00037 SPI_Write2(0x0C, 0x01); // Normal operation mode 00038 SPI_Write2(0x0F, 0x0F); // Enable display test 00039 wait_ms(500); // 500 ms delay 00040 SPI_Write2(0x01, 0x00); // Clear row 0. 00041 SPI_Write2(0x02, 0x00); // Clear row 1. 00042 SPI_Write2(0x03, 0x00); // Clear row 2. 00043 SPI_Write2(0x04, 0x00); // Clear row 3. 00044 SPI_Write2(0x05, 0x00); // Clear row 4. 00045 SPI_Write2(0x06, 0x00); // Clear row 5. 00046 SPI_Write2(0x07, 0x00); // Clear row 6. 00047 SPI_Write2(0x08, 0x00); // Clear row 7. 00048 SPI_Write2(0x0F, 0x00); // Disable display test 00049 wait_ms(500); // 500 ms delay 00050 } 00051 00052 int main() 00053 { 00054 cs = 1; // CS initially High 00055 spi.format(8,0); // 8-bit format, mode 0,0 00056 spi.frequency(1000000); // SCLK = 1 MHz 00057 Init_MAX7219(); // Initialize the LED controller 00058 00059 while (1) { 00060 for(int i=1; i<9; i++) // Write first character (8 rows) 00061 SPI_Write2(i,led1[i-1]); 00062 wait(1); // 1 sec delay 00063 for(int i=1; i<9; i++) // Write second character 00064 SPI_Write2(i,led2[i-1]); 00065 wait(1); // 1 sec delay 00066 } 00067 }
Generated on Tue Jul 26 2022 04:27:54 by
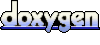