
Simple 8x8 LED Matrix controller which interfaces with a Processing GUI over serial to display sketches
Dependencies: Multi_WS2811 mbed
Fork of Multi_WS2811_test by
main.cpp
00001 #include "mbed.h" 00002 #include "WS2811.h" 00003 00004 // per LED: 3 * 20 mA = 60mA max 00005 // 60 LEDs: 60 * 60mA = 3600 mA max 00006 // 120 LEDs: 7200 mA max 00007 unsigned const nLEDs = MAX_LEDS_PER_STRIP; 00008 00009 unsigned const DATA_OUT_PIN1 = 7; 00010 unsigned const DATA_OUT_PIN2 = 0; 00011 unsigned const DATA_OUT_PIN3 = 3; 00012 unsigned const DATA_OUT_PIN4 = 4; 00013 unsigned const DATA_OUT_PIN5 = 5; 00014 unsigned const DATA_OUT_PIN6 = 6; 00015 unsigned const DATA_OUT_PIN7 = 10; 00016 unsigned const DATA_OUT_PIN8 = 11; 00017 00018 Serial pc(USBTX, USBRX); 00019 00020 static void clearScreen(WS2811 &strip) 00021 { 00022 unsigned nLEDs = strip.numPixels(); 00023 for (unsigned i = 0; i < nLEDs; i++) { 00024 strip.setPixelColor(i, 0, 0, 0); 00025 } 00026 strip.show(); 00027 } 00028 00029 int main(void) 00030 { 00031 pc.baud(9600); 00032 WS2811 *lightStrip; 00033 WS2811 lightStrip1(nLEDs, DATA_OUT_PIN1); 00034 WS2811 lightStrip2(nLEDs, DATA_OUT_PIN2); 00035 WS2811 lightStrip3(nLEDs, DATA_OUT_PIN3); 00036 WS2811 lightStrip4(nLEDs, DATA_OUT_PIN4); 00037 WS2811 lightStrip5(nLEDs, DATA_OUT_PIN5); 00038 WS2811 lightStrip6(nLEDs, DATA_OUT_PIN6); 00039 WS2811 lightStrip7(nLEDs, DATA_OUT_PIN7); 00040 WS2811 lightStrip8(nLEDs, DATA_OUT_PIN8); 00041 00042 lightStrip1.begin(); 00043 lightStrip2.begin(); 00044 lightStrip3.begin(); 00045 lightStrip4.begin(); 00046 lightStrip5.begin(); 00047 lightStrip6.begin(); 00048 lightStrip7.begin(); 00049 lightStrip8.begin(); 00050 00051 uint8_t q = 0; 00052 char char_buff[10]; 00053 char curr_char = 0; 00054 00055 for (;;) { 00056 /* I have defined a custom UART packet protocol 00057 * A general packet may look like: 00058 * 00059 * array[0] x-coordinate [0, 31] 00060 * array[1] y-coordinate [0, 15] 00061 * array[2] R (5-bit) 00062 * array[3] G (5-bit) 00063 * array[4] B (5-bit) 00064 * array[5] 254 (delimiter) 00065 */ 00066 while(curr_char != 255 && q < 7) 00067 { 00068 /* If there is UART traffic */ 00069 if(pc.readable()) 00070 { 00071 curr_char = pc.getc(); 00072 char_buff[q] = curr_char; 00073 q++; 00074 } 00075 } 00076 00077 if((char_buff[0] == 254) && (q >= 5)){ 00078 switch(int(char_buff[2])){ 00079 case 0: 00080 lightStrip = &lightStrip1; 00081 break; 00082 case 1: 00083 lightStrip = &lightStrip2; 00084 break; 00085 case 2: 00086 lightStrip = &lightStrip3; 00087 break; 00088 case 3: 00089 lightStrip = &lightStrip4; 00090 break; 00091 case 4: 00092 lightStrip = &lightStrip5; 00093 break; 00094 case 5: 00095 lightStrip = &lightStrip6; 00096 break; 00097 case 6: 00098 lightStrip = &lightStrip7; 00099 break; 00100 case 7: 00101 lightStrip = &lightStrip8; 00102 break; 00103 default: 00104 lightStrip = &lightStrip1; 00105 q = 0; 00106 break; 00107 } 00108 00109 (*lightStrip).setPixelColor(int(char_buff[1]), int(char_buff[3]), int(char_buff[4]), int(char_buff[5])); 00110 00111 (*lightStrip).show(); 00112 pc.putc(48+int(char_buff[0])); 00113 } 00114 else{ 00115 //clearScreen(*lightStrip); 00116 } 00117 q = 0; 00118 curr_char = 0; 00119 WS2811::startDMA(); 00120 00121 } 00122 } 00123
Generated on Thu Jul 14 2022 04:07:17 by
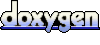