
test public
Dependencies: HttpServer_snapshot_mbed-os
r_ospl.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file r_ospl.h 00025 * @brief OS Porting Layer. Main Header. Functions. 00026 * 00027 * $Module: OSPL $ $PublicVersion: 0.90 $ (=R_OSPL_VERSION) 00028 * $Rev: 35 $ 00029 * $Date:: 2014-04-15 21:38:18 +0900#$ 00030 */ 00031 00032 #ifndef R_OSPL_H 00033 #define R_OSPL_H 00034 00035 00036 /****************************************************************************** 00037 Includes <System Includes> , "Project Includes" 00038 ******************************************************************************/ 00039 #include "Project_Config.h" 00040 #include "ospl_platform.h" 00041 #include "r_ospl_typedef.h" 00042 #include "r_multi_compiler_typedef.h" 00043 #include "locking.h" 00044 #include "r_static_an_tag.h" 00045 #include "r_ospl_debug.h" 00046 #if ! R_OSPL_IS_PREEMPTION 00047 #include "r_ospl_os_less.h" 00048 #endif 00049 00050 #ifdef __cplusplus 00051 extern "C" { 00052 #endif /* __cplusplus */ 00053 00054 00055 /****************************************************************************** 00056 Typedef definitions 00057 ******************************************************************************/ 00058 /* In "r_ospl_typedef.h" */ 00059 00060 /****************************************************************************** 00061 Macro definitions 00062 ******************************************************************************/ 00063 /* In "r_ospl_typedef.h" */ 00064 00065 /****************************************************************************** 00066 Variable Externs 00067 ******************************************************************************/ 00068 /* In "r_ospl_typedef.h" */ 00069 00070 /****************************************************************************** 00071 Functions Prototypes 00072 ******************************************************************************/ 00073 00074 00075 /* Section: Version and initialize */ 00076 /** 00077 * @brief Returns version number of OSPL 00078 * 00079 * @par Parameters 00080 * None 00081 * @return Version number of OSPL 00082 * 00083 * @par Description 00084 * Return value is same as "R_OSPL_VERSION" macro. 00085 */ 00086 int32_t R_OSPL_GetVersion(void); 00087 00088 00089 /** 00090 * @brief Returns whether the environment is supported preemption 00091 * 00092 * @par Parameters 00093 * None 00094 * @return Whether the environment is RTOS supported preemption 00095 * 00096 * @par Description 00097 * Return value is same as "R_OSPL_IS_PREEMPTION" macro. 00098 */ 00099 bool_t R_OSPL_IsPreemption(void); 00100 00101 00102 /** 00103 * @brief Initializes the internal of OSPL 00104 * 00105 * @param NullConfig Specify NULL 00106 * @return None 00107 * 00108 * @par Description 00109 * Initializes internal mutual exclusion objects. 00110 * However, "R_OSPL_Initialize" function does not have to be called for 00111 * OSPL of "R_OSPL_IS_PREEMPTION = 0". 00112 * "E_ACCESS_DENIED" error is raised, when the OSPL API that it is 00113 * necessary to call "R_OSPL_Initialize" before calling the API was called. 00114 */ 00115 errnum_t R_OSPL_Initialize( const void *const NullConfig ); 00116 00117 00118 /* Section: Standard functions */ 00119 /** 00120 * @brief No operation from C++ specification 00121 * 00122 * @par Parameters 00123 * None 00124 * @return None 00125 * 00126 * @par Description 00127 * Compatible with __noop (MS C++). But our naming rule is not match. 00128 */ 00129 INLINE void R_NOOP(void) {} 00130 00131 00132 /** 00133 * @brief Returns element count of the array 00134 * 00135 * @param Array An array 00136 * @return Count of specified array's element 00137 * 00138 * @par Description 00139 * Compatible with _countof (MS C++) and ARRAY_SIZE (Linux). 00140 * But our naming rule is not match. 00141 * 00142 * @par Example 00143 * @code 00144 * uint32_t array[10]; 00145 * R_COUNT_OF( array ) // = 10 00146 * @endcode 00147 * 00148 * @par Example 00149 * Array argument must not be specified the pointer using like array. 00150 * @code 00151 * uint32_t array[10]; 00152 * func( array ); 00153 * 00154 * void func( uint32_t array[] ) // "array" is a pointer 00155 * { 00156 * R_COUNT_OF( array ) // NG 00157 * } 00158 * @endcode 00159 */ 00160 /* ->MISRA 19.7 : Cannot function */ /* ->SEC M5.1.3 */ 00161 #define R_COUNT_OF( Array ) ( sizeof( Array ) / sizeof( *(Array) ) ) 00162 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 00163 00164 00165 /* Section: Error handling and debugging (1) */ 00166 00167 00168 /** 00169 * @def IF 00170 * @brief Breaks and transits to error state, if condition expression is not 0 00171 * @param Condition Condition expression 00172 * @return None 00173 * 00174 * @par Example 00175 * @code 00176 * e= TestFunction(); IF(e){goto fin;} 00177 * @endcode 00178 * 00179 * @par Description 00180 * "IF" is as same as general "if", if "R_OSPL_ERROR_BREAK" macro was 00181 * defined to be 0. The following descriptions are available, 00182 * if "R_OSPL_ERROR_BREAK" macro was defined to be 1. 00183 * 00184 * "IF" macro supports to find the code raising an error. 00185 * 00186 * If the "if statement" that is frequently seen in guard condition and 00187 * after calling functions was changed to "IF" macro, the CPU breaks 00188 * at raising an error. Then the status (values of variables) can be 00189 * looked immediately and the code (call stack) can be looked. Thus, 00190 * debug work grows in efficiency. 00191 * 00192 * "IF" macro promotes recognizing normal code and exceptional code. 00193 * Reading speed will grow up by skipping exceptional code. 00194 * 00195 * Call "R_OSPL_SET_BREAK_ERROR_ID" function, if set to break at the code 00196 * raising an error. 00197 * 00198 * Whether the state was error state or the error raised count is stored 00199 * in the thread local storage. In Release configuration, the variable 00200 * of error state and the error raised count is deleted. Manage the error 00201 * code using auto variable and so on at out of OSPL. 00202 * 00203 * The error state is resolved by calling "R_OSPL_CLEAR_ERROR" function. 00204 * If "R_DEBUG_BREAK_IF_ERROR" macro was called with any error state, 00205 * the process breaks at the macro. 00206 */ 00207 #if R_OSPL_ERROR_BREAK 00208 00209 /* ->MISRA 19.4 : Abnormal termination. Compliant with C language syntax. */ /* ->SEC M1.8.2 */ 00210 #define IF( Condition ) \ 00211 if ( IS( R_OSPL_OnRaisingErrorForMISRA( \ 00212 IS( (int_fast32_t)( Condition ) ), __FILE__, __LINE__ ) ) ) 00213 /* (int_fast32_t) cast is for QAC warning of implicit cast unsigned to signed */ 00214 /* != 0 is for QAC warning of MISRA 13.2 Advice */ 00215 /* <-MISRA 19.4 */ /* <-SEC M1.8.2 */ 00216 00217 #else /* ! R_OSPL_ERROR_BREAK */ 00218 00219 /* ->MISRA 19.4 : Abnormal termination. Compliant with C language syntax. */ /* ->SEC M1.8.2 */ 00220 #define IF if 00221 /* <-MISRA 19.4 */ /* <-SEC M1.8.2 */ 00222 #endif 00223 00224 00225 /** 00226 * @def IF_D 00227 * @brief It is same as "IF" (for Debug configuration only) 00228 * @param Condition Condition expression 00229 * @return None 00230 * 00231 * @par Description 00232 * In Release configuration, the result of condition expression is always "false". 00233 * The release configuration is the configuration defined "R_OSPL_NDEBUG". 00234 */ 00235 /* ->MISRA 19.4 : Compliant with C language syntax. */ /* ->SEC M1.8.2 */ 00236 /* ->MISRA 19.7 : Cannot function */ /* ->SEC M5.1.3 */ 00237 #ifndef R_OSPL_NDEBUG 00238 #define IF_D( Condition ) IF ( Condition ) 00239 #else 00240 #define IF_D( Condition ) if ( false ) 00241 #endif 00242 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 00243 /* <-MISRA 19.4 */ /* <-SEC M1.8.2 */ 00244 00245 00246 /** 00247 * @def ASSERT_R 00248 * @brief Assertion (Programming By Contract) 00249 * @param Condition The condition expression expected true 00250 * @param goto_fin_Statement The operation doing at condition is false 00251 * @return None 00252 * 00253 * @par Description 00254 * It is possible to write complex sentence divided by ";" in 00255 * "goto_fin_Statement" argument. 00256 * 00257 * @par - Case of defined "R_OSPL_ERROR_BREAK" to be 0 00258 * If the result of condition expression is 0(false), do "StatementsForError". 00259 * If operations did nothing, write "R_NOOP()" at "StatementsForError" argument. 00260 * 00261 * @par - Case of defined "R_OSPL_ERROR_BREAK" to be 1 00262 * If the result of condition expression is 0(false), the error state 00263 * will become active and the operation of "StatementForError" argument 00264 * will be done. 00265 */ 00266 #ifndef __cplusplus 00267 #define ASSERT_R( Condition, goto_fin_Statement ) \ 00268 do{ IF(!(Condition)) { goto_fin_Statement; } } while(0) /* do-while is CERT standard PRE10-C */ 00269 #else 00270 #define ASSERT_R( Condition, goto_fin_Statement ) \ 00271 { IF(!(Condition)) { goto_fin_Statement; } } /* no C5236(I) */ 00272 #endif 00273 00274 00275 /** 00276 * @def ASSERT_D 00277 * @brief Assertion (Programming By Contract) (for Debug configuration only) 00278 * @param Condition The condition expression expected true 00279 * @param goto_fin_Statement The operation doing at condition is false 00280 * @return None 00281 * 00282 * @par Description 00283 * This does nothing in Release configuration. 00284 * Release configuration is the configuration defined "R_OSPL_NDEBUG" 00285 * as same as standard library. 00286 */ 00287 #ifndef R_OSPL_NDEBUG 00288 #define ASSERT_D ASSERT_R 00289 #else 00290 /* ->MISRA 19.7 : Function's argument can not get "goto_fin_Statement" */ /* ->SEC M5.1.3 */ 00291 #define ASSERT_D( Condition, goto_fin_Statement ) R_NOOP() 00292 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 00293 #endif 00294 00295 00296 /** 00297 * @brief Sub routine of IF macro 00298 * 00299 * @param Condition Condition in IF macro 00300 * @param File File name 00301 * @param Line Line number 00302 * @return "Condition" argument 00303 * 00304 * @par Description 00305 * - This part is for compliant to MISRA 2004 - 19.7. 00306 */ 00307 bool_t R_OSPL_OnRaisingErrorForMISRA( bool_t const Condition, const char_t *const File, 00308 int_t const Line ); 00309 00310 00311 /*********************************************************************** 00312 * Class: r_ospl_thread_id_t 00313 ************************************************************************/ 00314 00315 /** 00316 * @brief Get running thread ID (for OS less and OS-using environment) 00317 * 00318 * @par Parameters 00319 * None 00320 * @return The current running thread ID 00321 * 00322 * @par Description 00323 * - It is possible to use this function for both OS less and OS - using environment. 00324 * For OS less, returns thread ID passed to "R_OSPL_THREAD_SetCurrentId" function. 00325 * "NULL" is returned, if in interrupt context. 00326 */ 00327 r_ospl_thread_id_t R_OSPL_THREAD_GetCurrentId(void); 00328 00329 00330 /** 00331 * @brief Set one or some bits to 1 00332 * 00333 * @param ThreadId The thread ID attached the target event 00334 * @param SetFlags The value of bit flags that target bit is 1 00335 * @return None 00336 * 00337 * @par Description 00338 * For OS less, there is the area disabled all interrupts. 00339 * 00340 * - For OS - using environment, the thread waiting in "R_OSPL_EVENT_Wait" 00341 * function might wake up soon. 00342 * 00343 * Do nothing, when "ThreadId" = "NULL" 00344 */ 00345 void R_OSPL_EVENT_Set( r_ospl_thread_id_t const ThreadId, bit_flags32_t const SetFlags ); 00346 00347 00348 /** 00349 * @brief Set one or some bits to 0 00350 * 00351 * @param ThreadId The thread ID attached the target event 00352 * @param ClearFlags1 The value of bit flags that clearing bit is 1 00353 * @return None 00354 * 00355 * @par Description 00356 * It is not necessary to call this function after called "R_OSPL_EVENT_Wait" 00357 * function. 00358 * 00359 * The way that all bit flags is cleared is setting "R_OSPL_EVENT_ALL_BITS" 00360 * (=0x0000FFFF) at "ClearFlags1" argument. 00361 * 00362 * When other thread was nofied by calling "R_OSPL_EVENT_Set", "R_OSPL_EVENT_Clear" 00363 * must not be called from caller (notifier) thread. 00364 * 00365 * For OS less, there is the area disabled all interrupts. 00366 * 00367 * Do nothing, when "ThreadId" = "NULL" 00368 */ 00369 void R_OSPL_EVENT_Clear( r_ospl_thread_id_t const ThreadId, bit_flags32_t const ClearFlags1 ); 00370 00371 00372 /** 00373 * @brief Get 16bit flags value 00374 * 00375 * @param ThreadId The thread ID attached the target event 00376 * @return The value of 16bit flags 00377 * 00378 * @par Description 00379 * This API cannot be used in newest specification. 00380 * 00381 * In receiving the event, call "R_OSPL_EVENT_Wait" function instead of 00382 * "R_OSPL_EVENT_Get" function or call "R_OSPL_EVENT_Clear" function 00383 * passed the NOT operated value of flags got by "R_OSPL_EVENT_Get" function. 00384 */ 00385 #if ( ! defined( osCMSIS ) || osCMSIS <= 0x10001 ) && R_OSPL_VERSION < 85 00386 bit_flags32_t R_OSPL_EVENT_Get( r_ospl_thread_id_t const ThreadId ); 00387 #endif 00388 00389 00390 /** 00391 * @brief Waits for setting the flags in 16bit and clear received flags 00392 * 00393 * @param WaigingFlags The bit flags set to 1 waiting or "R_OSPL_ANY_FLAG" 00394 * @param out_GotFlags NULL is permitted. Output: 16 bit flags or "R_OSPL_TIMEOUT" 00395 * @param Timeout_msec Time out (millisecond) or "R_OSPL_INFINITE" 00396 * @return Error code. If there is no error, the return value is 0. 00397 * 00398 * @par Description 00399 * Waits in this function until the flags become passed flags pattern 00400 * by "R_OSPL_EVENT_Set" function. 00401 * 00402 * Check "r_ospl_async_t::ReturnValue", when the asynchronous operation 00403 * was ended. 00404 */ 00405 errnum_t R_OSPL_EVENT_Wait( bit_flags32_t const WaigingFlags, bit_flags32_t *const out_GotFlags, 00406 uint32_t const Timeout_msec ); 00407 /* Unsigned flag (bit_flags32_t) is for QAC 4130 */ 00408 00409 00410 /*********************************************************************** 00411 * Class: r_ospl_flag32_t 00412 ************************************************************************/ 00413 00414 /** 00415 * @brief Clears all flags in 32bit to 0 00416 * 00417 * @param self The value of 32bit flags 00418 * @return None 00419 * 00420 * @par Description 00421 * Operates following operation. 00422 * @code 00423 * volatile bit_flags32_t self->flags; 00424 * self->flags = 0; 00425 * @endcode 00426 */ 00427 void R_OSPL_FLAG32_InitConst( volatile r_ospl_flag32_t *const self ); 00428 00429 00430 /** 00431 * @brief Set one or some bits to 1 00432 * 00433 * @param self The value of 32bit flags 00434 * @param SetFlags The value of bit flags that target bit is 1 00435 * @return None 00436 * 00437 * @par Description 00438 * Operates following operation. 00439 * @code 00440 * volatile bit_flags32_t self->Flags; 00441 * bit_flags32_t SetFlags; 00442 * self->Flags |= SetFlags; 00443 * @endcode 00444 * This function is not atomic because "|=" operator is "Read Modify Write" operation. 00445 */ 00446 void R_OSPL_FLAG32_Set( volatile r_ospl_flag32_t *const self, bit_flags32_t const SetFlags ); 00447 00448 00449 /** 00450 * @brief Set one or some bits to 0 00451 * 00452 * @param self The value of 32bit flags 00453 * @param ClearFlags1 The value of bit flags that clearing bit is 1 00454 * @return None 00455 * 00456 * @par Description 00457 * Operates following operation. 00458 * @code 00459 * volatile bit_flags32_t self->Flags; 00460 * bit_flags32_t ClearFlags1; 00461 * 00462 * self->Flags &= ~ClearFlags1; 00463 * @endcode 00464 * 00465 * Set "R_OSPL_FLAG32_ALL_BITS", if you wanted to clear all bits. 00466 * 00467 * This function is not atomic because "&=" operator is "Read Modify Write" operation. 00468 */ 00469 void R_OSPL_FLAG32_Clear( volatile r_ospl_flag32_t *const self, bit_flags32_t const ClearFlags1 ); 00470 00471 00472 /** 00473 * @brief Get 32bit flags value 00474 * 00475 * @param self The value of 32bit flags 00476 * @return The value of 32bit flags 00477 * 00478 * @par Description 00479 * In receiving the event, call "R_OSPL_FLAG32_GetAndClear" function 00480 * instead of "R_OSPL_FLAG32_Get" function or call "R_OSPL_FLAG32_Clear" 00481 * function passed the NOT operated value of flags got by "R_OSPL_FLAG32_Get" 00482 * function. 00483 * 00484 * @code 00485 * Operates following operation. 00486 * volatile bit_flags32_t self->Flags; 00487 * bit_flags32_t return_flags; 00488 * 00489 * return_flags = self->Flags; 00490 * 00491 * return return_flags; 00492 * @endcode 00493 */ 00494 bit_flags32_t R_OSPL_FLAG32_Get( volatile const r_ospl_flag32_t *const self ); 00495 00496 00497 /** 00498 * @brief Get 32bit flags value 00499 * 00500 * @param self The value of 32bit flags 00501 * @return The value of 32bit flags 00502 * 00503 * @par Description 00504 * Operates following operation. 00505 * @code 00506 * volatile bit_flags32_t self->Flags; 00507 * bit_flags32_t return_flags; 00508 * 00509 * return_flags = self->Flags; 00510 * self->Flags = 0; 00511 * 00512 * return return_flags; 00513 * @endcode 00514 * 00515 * This function is not atomic because the value might be set before clearing to 0. 00516 */ 00517 bit_flags32_t R_OSPL_FLAG32_GetAndClear( volatile r_ospl_flag32_t *const self ); 00518 00519 00520 /*********************************************************************** 00521 * Class: r_ospl_queue_t 00522 ************************************************************************/ 00523 00524 /** 00525 * @brief Initializes a queue 00526 * 00527 * @param out_self Output: Address of initialized queue object 00528 * @param QueueDefine Initial attributes of queue and work area 00529 * @return Error code. If there is no error, the return value is 0 00530 * 00531 * @par Description 00532 * It is not possible to call this function from the library. 00533 * This function is called from porting layer of the driver and send 00534 * created queue to the driver. 00535 * 00536 * OSPL does not have finalizing function (portabled with CMSIS). 00537 * An object specified "QueueDefine" argument can be specified to the 00538 * create function 1 times only. Some OS does not have this limitation. 00539 * 00540 * The address of a variable as "r_ospl_queue_t*" type is set at 00541 * "out_self" argument. 00542 * Internal variables of the queue are stored in the variable specified 00543 * with "QueueDefine" argument. 00544 */ 00545 errnum_t R_OSPL_QUEUE_Create( r_ospl_queue_t **out_self, r_ospl_queue_def_t *QueueDefine ); 00546 00547 00548 /** 00549 * @brief Gets status of the queue 00550 * 00551 * @param self A queue object 00552 * @param out_Status Output: Pointer to the status structure 00553 * @return Error code. If there is no error, the return value is 0 00554 * 00555 * @par Description 00556 * Got status are the information at calling moment. 00557 * If ohter threads were run, the status will be changed. 00558 * See "R_DRIVER_GetAsyncStatus" function about pointer type of 00559 * "out_Status" argument 00560 */ 00561 errnum_t R_OSPL_QUEUE_GetStatus( r_ospl_queue_t *self, const r_ospl_queue_status_t **out_Status ); 00562 00563 00564 /** 00565 * @brief Allocates an element from the queue object 00566 * 00567 * @param self A queue object 00568 * @param out_Address Output: Address of allocated element 00569 * @param Timeout_msec Timeout (msec) or R_OSPL_INFINITE 00570 * @return Error code. If there is no error, the return value is 0 00571 * 00572 * @par Description 00573 * An error will be raised, if "Timeout_msec != 0" in interrupt context. 00574 * It becomes "*out_Address = NULL", when it was timeout and 00575 * "Timeout_msec = 0". 00576 * E_TIME_OUT error is raised, when it was timeout and "Timeout_msec != 0". 00577 */ 00578 errnum_t R_OSPL_QUEUE_Allocate( r_ospl_queue_t *self, void *out_Address, uint32_t Timeout_msec ); 00579 00580 00581 /** 00582 * @brief Sends the element to the queue 00583 * 00584 * @param self A queue object 00585 * @param Address Address of element to put 00586 * @return Error code. If there is no error, the return value is 0 00587 * 00588 * @par Description 00589 * It is correct, even if other thread put to the queue or get from 00590 * the queue from calling "R_OSPL_QUEUE_Allocate" to calling 00591 * "R_OSPL_QUEUE_Put". 00592 * 00593 * The message put to the queue by this function receives the thread 00594 * calling "R_OSPL_QUEUE_Get" function. 00595 */ 00596 errnum_t R_OSPL_QUEUE_Put( r_ospl_queue_t *self, void *Address ); 00597 00598 00599 /** 00600 * @brief Receives the element from the queue 00601 * 00602 * @param self A queue object 00603 * @param out_Address Output: Address of received element 00604 * @param Timeout_msec Timeout (msec) or R_OSPL_INFINITE 00605 * @return Error code. If there is no error, the return value is 0 00606 * 00607 * @par Description 00608 * Call "R_OSPL_QUEUE_Free" function after finishing to access to 00609 * the element. Don't access the memory area of the element after 00610 * calling "R_OSPL_QUEUE_Free". 00611 * 00612 * "E_NOT_THREAD" error is raised, if "Timeout_msec = 0" was specified 00613 * from interrupt context. It is not possible to wait for put data to 00614 * the queue in interrupt context. 00615 * 00616 * "*out_Address" is NULL and any errors are not raised, if it becomed 00617 * to timeout and "Timeout_msec = 0". "E_TIME_OUT" is raised, 00618 * if "Timeout_msec != 0". 00619 * 00620 * Specify "Timeout_msec = 0", call the following functions by the following 00621 * order and use an event for preventing to block to receive other events 00622 * by the thread having waited for the queue. 00623 * 00624 * Sending Side 00625 * - R_OSPL_QUEUE_Allocate 00626 * - R_OSPL_QUEUE_Put 00627 * - R_OSPL_EVENT_Set 00628 * 00629 * Receiving Side 00630 * - R_OSPL_EVENT_Wait 00631 * - R_OSPL_QUEUE_Get 00632 * - R_OSPL_QUEUE_Free 00633 * 00634 * In OS less environment, "R_OSPL_QUEUE_Get" supports pseudo multi 00635 * threading. See "R_OSPL_THREAD_GetIsWaiting" function. 00636 */ 00637 errnum_t R_OSPL_QUEUE_Get( r_ospl_queue_t *self, void *out_Address, uint32_t Timeout_msec ); 00638 00639 00640 /** 00641 * @brief Releases the element to the queue object 00642 * 00643 * @param self A queue object 00644 * @param Address Address of received element 00645 * @return Error code. If there is no error, the return value is 0 00646 * 00647 * @par Description 00648 * It is correct, even if other thread put to the queue or get from 00649 * the queue from calling "R_OSPL_QUEUE_Get" to calling "R_OSPL_QUEUE_Free". 00650 */ 00651 errnum_t R_OSPL_QUEUE_Free( r_ospl_queue_t *self, void *Address ); 00652 00653 00654 /** 00655 * @brief Print status of the queue object 00656 * 00657 * @param self A queue object 00658 * @return Error code. If there is no error, the return value is 0 00659 */ 00660 #ifndef R_OSPL_NDEBUG 00661 errnum_t R_OSPL_QUEUE_Print( r_ospl_queue_t *self ); 00662 #endif 00663 00664 00665 /*********************************************************************** 00666 * Class: r_ospl_async_t 00667 ************************************************************************/ 00668 00669 /** 00670 * @brief CopyExceptAThread 00671 * 00672 * @param Source Source 00673 * @param Destination Destination 00674 * @return None 00675 */ 00676 void R_OSPL_ASYNC_CopyExceptAThread( const r_ospl_async_t *const Source, 00677 r_ospl_async_t *const Destination ); 00678 00679 00680 /*********************************************************************** 00681 * Class: r_ospl_caller_t 00682 ************************************************************************/ 00683 00684 /** 00685 * @brief Calls the interrupt callback function. It is called from OS porting layer in the driver 00686 * 00687 * @param self The internal parameters about interrupt operations 00688 * @param InterruptSource The source of the interrupt 00689 * @return None 00690 */ 00691 void R_OSPL_CallInterruptCallback( const r_ospl_caller_t *const self, 00692 const r_ospl_interrupt_t *const InterruptSource ); 00693 00694 00695 /** 00696 * @brief Initialize <r_ospl_caller_t>. 00697 * 00698 * @param self The internal parameters about interrupt operations 00699 * @param Async <r_ospl_async_t> 00700 * @return None 00701 */ 00702 void R_OSPL_CALLER_Initialize( r_ospl_caller_t *const self, r_ospl_async_t *const Async, 00703 volatile void *const PointerToState, int_t const StateValueOfOnInterrupting, 00704 void *const I_Lock, const r_ospl_i_lock_vtable_t *const I_LockVTable ); 00705 00706 00707 /** 00708 * @brief GetRootChannelNum. 00709 * 00710 * @param self The internal parameters about interrupt operations 00711 * @return RootChannelNum 00712 */ 00713 INLINE int_fast32_t R_OSPL_CALLER_GetRootChannelNum( const r_ospl_caller_t *const self ); 00714 00715 00716 /* Section: Interrupt */ 00717 /** 00718 * @brief Interrupt callback function for unregisterd interrupt. 00719 * 00720 * @param int_sense (See INTC driver) 00721 * @return None 00722 */ 00723 void R_OSPL_OnInterruptForUnregistered( uint32_t const int_sense ); 00724 00725 00726 /** 00727 * @brief Releases all disabled interrupts 00728 * 00729 * @par Parameters 00730 * None 00731 * @return None 00732 * 00733 * @par Description 00734 * Driver user should not call this function. 00735 * Call this function at the end of area of all interrupts disabled. 00736 * Do not release, if all interrupts was already disabled by caller function. 00737 * This function does not release disabled NMI. 00738 */ 00739 void R_OSPL_EnableAllInterrupt(void); 00740 00741 00742 /** 00743 * @brief Disables all interrupts 00744 * 00745 * @par Parameters 00746 * None 00747 * @return None 00748 * 00749 * @par Description 00750 * Driver user should not call this function. 00751 * Call this function at begin of area of all interrupts disabled. 00752 * This function does not disable NMI. 00753 * 00754 * @par Example 00755 * @code 00756 * void Func() 00757 * { 00758 * R_OSPL_DisableAllInterrupt(); 00759 * 00760 * // All interrupt disabled 00761 * 00762 * R_OSPL_EnableAllInterrupt(); 00763 * } 00764 * @endcode 00765 */ 00766 void R_OSPL_DisableAllInterrupt(void); 00767 00768 00769 /** 00770 * @brief Sets the priority of the interrupt line. 00771 * 00772 * @param IRQ_Num Interrupt request number 00773 * @param Priority Priority. The less the prior. 00774 * @return Error code. If there is no error, the return value is 0 00775 */ 00776 errnum_t R_OSPL_SetInterruptPriority( bsp_int_src_t const IRQ_Num, int_fast32_t const Priority ); 00777 00778 00779 /* Section: Locking channel */ 00780 /** 00781 * @brief Locks by channel number. 00782 * 00783 * @param ChannelNum Locking channel number or "R_OSPL_UNLOCKED_CHANNEL" 00784 * @param out_ChannelNum Output: Locked channel number, (in) NULL is permitted 00785 * @param HardwareIndexMin Hardware index of channel number = 0 00786 * @param HardwareIndexMax Hardware index of max channel number 00787 * @return Error code. If there is no error, the return value is 0 00788 * 00789 * @par Description 00790 * This function is called from the internal of "R_DRIVER_Initialize" 00791 * function or "R_DRIVER_LockChannel" function. 00792 * This function calls "R_BSP_HardwareLock". 00793 */ 00794 errnum_t R_OSPL_LockChannel( int_fast32_t ChannelNum, int_fast32_t *out_ChannelNum, 00795 mcu_lock_t HardwareIndexMin, mcu_lock_t HardwareIndexMax ); 00796 00797 00798 /** 00799 * @brief Unlocks by channel number. 00800 * 00801 * @param ChannelNum Channel number 00802 * @param e Raising error code, If there is no error, 0 00803 * @param HardwareIndexMin Hardware index of channel number = 0 00804 * @param HardwareIndexMax Hardware index of max channel number 00805 * @return Error code. If there is no error, the return value is 0 00806 * 00807 * @par Description 00808 * This function is called from the internal of "R_DRIVER_Finalize" 00809 * function or "R_DRIVER_UnlockChannel" function. 00810 * This function calls "R_BSP_HardwareUnlock". 00811 */ 00812 errnum_t R_OSPL_UnlockChannel( int_fast32_t ChannelNum, errnum_t e, 00813 mcu_lock_t HardwareIndexMin, mcu_lock_t HardwareIndexMax ); 00814 00815 00816 /*********************************************************************** 00817 * Class: r_ospl_c_lock_t 00818 ************************************************************************/ 00819 00820 /** 00821 * @brief Initializes the C-lock object 00822 * 00823 * @param self C-lock object 00824 * @return None 00825 * 00826 * @par Description 00827 * If *self is global variable or static variable initialized 0, 00828 * this function does not have to be called. 00829 */ 00830 void R_OSPL_C_LOCK_InitConst( r_ospl_c_lock_t *const self ); 00831 00832 00833 /** 00834 * @brief Locks the target, if lockable state. 00835 * 00836 * @param self C-lock object 00837 * @return Error code. If there is no error, the return value is 0. 00838 * 00839 * @par Description 00840 * Even if lock owner called this function, if lock object was already 00841 * locked, E_ACCESS_DENIED error is raised. 00842 * 00843 * "R_OSPL_C_LOCK_Lock" does not do exclusive control. 00844 */ 00845 errnum_t R_OSPL_C_LOCK_Lock( r_ospl_c_lock_t *const self ); 00846 00847 00848 /** 00849 * @brief Unlocks the target. 00850 * 00851 * @param self C-lock object 00852 * @return Error code. If there is no error, the return value is 0. 00853 * 00854 * @par Description 00855 * If this function was called with unlocked object, this function 00856 * does nothing and raises "E_ACCESS_DENIED" error. 00857 * 00858 * If self == NULL, this function does nothing and raises no error. 00859 * E_NOT_THREAD error is raised, if this function was called from the 00860 * interrupt context. 00861 * 00862 * - I - lock does not do in this function. 00863 * 00864 * "R_OSPL_C_LOCK_Unlock" does not do exclusive control. 00865 */ 00866 errnum_t R_OSPL_C_LOCK_Unlock( r_ospl_c_lock_t *const self ); 00867 00868 00869 /*********************************************************************** 00870 * Class: r_ospl_i_lock_vtable_t 00871 ************************************************************************/ 00872 00873 /** 00874 * @brief Do nothing. This is registered to r_ospl_i_lock_vtable_t::Lock. 00875 * 00876 * @param self_ I-lock object 00877 * @return false 00878 */ 00879 bool_t R_OSPL_I_LOCK_LockStub( void *const self_ ); 00880 00881 00882 /** 00883 * @brief Do nothing. This is registered to r_ospl_i_lock_vtable_t::Unlock. 00884 * 00885 * @param self_ I-lock object 00886 * @return None 00887 */ 00888 void R_OSPL_I_LOCK_UnlockStub( void *const self_ ); 00889 00890 00891 /** 00892 * @brief Do nothing. This is registered to r_ospl_i_lock_vtable_t::RequestFinalize. 00893 * 00894 * @param self_ I-lock object 00895 * @return None 00896 */ 00897 void R_OSPL_I_LOCK_RequestFinalizeStub( void *const self_ ); 00898 00899 00900 /** 00901 * @brief Get root channel number 00902 * 00903 * @param self <r_ospl_caller_t> object 00904 * @return Root channel number 00905 */ 00906 INLINE int_fast32_t R_OSPL_CALLER_GetRootChannelNum( const r_ospl_caller_t *const self ) 00907 { 00908 int_fast32_t root_channel_num; 00909 00910 IF_DQ( self == NULL ) { 00911 root_channel_num = 0; 00912 } 00913 else { 00914 root_channel_num = self->I_LockVTable->GetRootChannelNum( self->I_Lock ); 00915 } 00916 00917 return root_channel_num; 00918 } 00919 00920 00921 /* Section: Memory Operation */ 00922 /** 00923 * @brief Flushes cache memory 00924 * 00925 * @param FlushType The operation of flush 00926 * @return None 00927 * 00928 * @par Description 00929 * Call the function of the driver after flushing input output buffer 00930 * in the cache memory, If the data area accessing by the hardware is 00931 * on cache and the driver did not manage the cache memory. 00932 * Whether the driver manages the cache memory is depend on the driver 00933 * specification. 00934 */ 00935 void R_OSPL_MEMORY_Flush( r_ospl_flush_t const FlushType ); 00936 00937 00938 /** 00939 * @brief Flushes cache memory with the range of virtual address. 00940 * 00941 * @param FlushType The operation of flush 00942 * @return None 00943 * 00944 * @par Description 00945 * Align "StartAddress" argument and "Length" argument to cache line size. 00946 * If not aligned, E_OTHERS error is raised. 00947 * Refer to : R_OSPL_MEMORY_GetSpecification 00948 * 00949 * If the data area written by the hardware and read from CPU was in cache 00950 * rea, when the hardware started without invalidate 00951 * ("R_OSPL_FLUSH_WRITEBACK_INVALIDATE" or "R_OSPL_FLUSH_INVALIDATE"), 00952 * invalidate the data area and read it after finished to write by hardware. 00953 * (If the driver does not manage the cache memory.) 00954 */ 00955 errnum_t R_OSPL_MEMORY_RangeFlush( r_ospl_flush_t const FlushType, 00956 const void *const StartAddress, size_t const Length ); 00957 00958 00959 /** 00960 * @brief Gets the specification about memory and cache memory. 00961 * 00962 * @param out_MemorySpec The specification about memory and cache memory 00963 * @return None 00964 */ 00965 void R_OSPL_MEMORY_GetSpecification( r_ospl_memory_spec_t *const out_MemorySpec ); 00966 00967 00968 /** 00969 * @brief Set a memory barrier. 00970 * 00971 * @par Parameters 00972 * None 00973 * @return None 00974 * 00975 * @par Description 00976 * In ARM, This function calls DSB assembler operation. 00977 * This effects to L1 cache only. 00978 */ 00979 void R_OSPL_MEMORY_Barrier(void); 00980 00981 00982 /** 00983 * @brief Set a instruction barrier. 00984 * 00985 * @par Parameters 00986 * None 00987 * @return None 00988 * 00989 * @par Description 00990 * In ARM, This function calls ISB assembler operation. 00991 */ 00992 void R_OSPL_InstructionSyncBarrier(void); 00993 00994 00995 /** 00996 * @brief Changes to physical address 00997 * 00998 * @param Address Virtual address 00999 * @param out_PhysicalAddress Output: Physical address 01000 * @return Error code. If there is no error, the return value is 0. 01001 * 01002 * @par Description 01003 * This function must be modified by MMU setting. 01004 */ 01005 errnum_t R_OSPL_ToPhysicalAddress( const volatile void *const Address, uintptr_t *const out_PhysicalAddress ); 01006 01007 01008 /** 01009 * @brief Changes to the address in the L1 cache area 01010 * 01011 * @param Address Virtual address 01012 * @param out_CachedAddress Output: Virtual address for cached area 01013 * @return Error code. If there is no error, the return value is 0. 01014 * 01015 * @par Description 01016 * This function must be modified by MMU setting. 01017 * If "E_ACCESS_DENIED" error was raised, you may know the variable by 01018 * looking at value of "Address" argument and map file. 01019 */ 01020 errnum_t R_OSPL_ToCachedAddress( const volatile void *const Address, void *const out_CachedAddress ); 01021 01022 01023 /** 01024 * @brief Changes to the address in the L1 uncached area 01025 * 01026 * @param Address Virtual address 01027 * @param out_UncachedAddress Output: Virtual address for uncached area 01028 * @return Error code. If there is no error, the return value is 0. 01029 * 01030 * @par Description 01031 * This function must be modified by MMU setting. 01032 * If "E_ACCESS_DENIED" error was raised, you may know the variable by 01033 * looking at value of "Address" argument and map file. 01034 */ 01035 errnum_t R_OSPL_ToUncachedAddress( const volatile void *const Address, void *const out_UncachedAddress ); 01036 01037 01038 /** 01039 * @brief Gets the level of cache for flushing the memory indicated by the address. 01040 * 01041 * @param Address The address in flushing memory 01042 * @param out_Level Output: 0=Not need to flush, 1=L1 cache only, 2=both of L1 and L2 cache 01043 * @return Error code. If there is no error, the return value is 0. 01044 */ 01045 errnum_t R_OSPL_MEMORY_GetLevelOfFlush( const void *Address, int_fast32_t *out_Level ); 01046 01047 01048 /** 01049 * @brief Get 2nd cache attribute of AXI bus for peripheral (not CPU) from physical address. 01050 * 01051 * @param PhysicalAddress The physical address in the memory area 01052 * @param out_CacheAttribute Output: Cache_attribute, AWCACHE[3:0], ARCACHE[3:0] 01053 * @return Error code. If there is no error, the return value is 0. 01054 */ 01055 errnum_t R_OSPL_AXI_Get2ndCacheAttribute( uintptr_t const PhysicalAddress, 01056 r_ospl_axi_cache_attribute_t *const out_CacheAttribute ); 01057 01058 01059 /** 01060 * @brief Gets protection attribute of AXI bus from the address 01061 * 01062 * @param PhysicalAddress The physical address in the memory area 01063 * @param out_CacheAttribute Output: The protection attribute of AXI bus AWPROT[2:0], ARPROT[2:0] 01064 * @return Error code. If there is no error, the return value is 0. 01065 */ 01066 errnum_t R_OSPL_AXI_GetProtection( uintptr_t const physical_address, 01067 r_ospl_axi_protection_t *const out_protection ); 01068 01069 01070 /* Section: Timer */ 01071 /** 01072 * @brief Waits for a while until passed time 01073 * 01074 * @param DelayTime_msec Time of waiting (millisecond) 01075 * @return Error code. If there is no error, the return value is 0. 01076 * 01077 * @par Description 01078 * Maximum value is "R_OSPL_MAX_TIME_OUT" (=65533). 01079 */ 01080 errnum_t R_OSPL_Delay( uint32_t const DelayTime_msec ); 01081 01082 01083 /** 01084 * @brief Set up the free running timer 01085 * 01086 * @param out_Specification NULL is permitted. Output: The precision of the free run timer 01087 * @return Error code. If there is no error, the return value is 0. 01088 * 01089 * @par Description 01090 * The free running timer does not stop. 01091 * 01092 * If the counter of the free running timer was overflow, the counter returns to 0. 01093 * Even in interrupt handler, the counter does count up. 01094 * OSPL free running timer does not use any interrupt. 01095 * 01096 * Using timer can be selected by "R_OSPL_FTIMER_IS" macro. 01097 * 01098 * If the free running timer was already set up, this function does not set up it, 01099 * outputs to "out_Specification" argument and does not raise any error. 01100 * 01101 * When OSPL API function with timeout or "R_OSPL_Delay" function was called, 01102 * "R_OSPL_FTIMER_InitializeIfNot" function is callbacked from these functions. 01103 * 01104 * There is all interrupt disabled area inside. 01105 */ 01106 errnum_t R_OSPL_FTIMER_InitializeIfNot( r_ospl_ftimer_spec_t *const out_Specification ); 01107 01108 01109 /** 01110 * @brief Gets the specification of free running timer. 01111 * 01112 * @param out_Specification Output: The precision of the free run timer 01113 * @return None 01114 */ 01115 void R_OSPL_FTIMER_GetSpecification( r_ospl_ftimer_spec_t *const out_Specification ); 01116 01117 01118 /** 01119 * @brief Get current time of free running timer. 01120 * 01121 * @par Parameters 01122 * None 01123 * @return The current clock count of free run timer 01124 * 01125 * @par Description 01126 * Call "R_OSPL_FTIMER_InitializeIfNot" function before calling this function. 01127 * Call "R_OSPL_FTIMER_IsPast" function, when it is determined whether time passed. 01128 * 01129 * @par Example 01130 * @code 01131 * errnum_t e; 01132 * r_ospl_ftimer_spec_t ts; 01133 * uint32_t start; 01134 * uint32_t end; 01135 * 01136 * e= R_OSPL_FTIMER_InitializeIfNot( &ts ); IF(e){goto fin;} 01137 * start = R_OSPL_FTIMER_Get(); 01138 * 01139 * // The section of measuring 01140 * 01141 * end = R_OSPL_FTIMER_Get(); 01142 * printf( "%d msec\n", R_OSPL_FTIMER_CountToTime( 01143 * &ts, end - start ) ); 01144 * @endcode 01145 */ 01146 uint32_t R_OSPL_FTIMER_Get(void); 01147 01148 01149 /** 01150 * @brief Returns whether specified time was passed 01151 * 01152 * @param ts Precision of the free running timer 01153 * @param Now Count of current time 01154 * @param TargetTime Count of target time 01155 * @param out_IsPast Output: Whether the target time was past or not 01156 * @return Error code. If there is no error, the return value is 0. 01157 */ 01158 errnum_t R_OSPL_FTIMER_IsPast( const r_ospl_ftimer_spec_t *const ts, 01159 uint32_t const Now, uint32_t const TargetTime, bool_t *const out_IsPast ); 01160 01161 01162 /** 01163 * @brief Change from mili-second unit to free running timer unit 01164 * 01165 * @param ts Precision of the free running timer 01166 * @param msec The value of mili-second unit 01167 * @return The value of free running timer unit 01168 * 01169 * @par Description 01170 * The fractional part is been round up. (For waiting time must be more 01171 * than specified time.) 01172 * 01173 * This function calculates like the following formula. 01174 * @code 01175 * ( msec * ts->msec_Denominator + ts->msec_Numerator - 1 ) / ts->msec_Numerator 01176 * @endcode 01177 * 01178 * - Attention: If "ts - >msec_Denominator" was more than "ts->msec_Numerator", 01179 * take care of overflow. 01180 */ 01181 INLINE uint32_t R_OSPL_FTIMER_TimeToCount( const r_ospl_ftimer_spec_t *const ts, 01182 uint32_t const msec ) 01183 { 01184 uint32_t count; 01185 01186 IF_DQ( ts == NULL ) { 01187 count = 0; 01188 } 01189 else { 01190 count = ( ((msec * ts->msec_Denominator) + ts->msec_Numerator) - 1u ) / ts->msec_Numerator; 01191 } 01192 return count; 01193 } 01194 01195 01196 /** 01197 * @brief Change from free running timer unit to mili-second unit 01198 * 01199 * @param ts Precision of the free running timer 01200 * @param Count The value of free running timer unit 01201 * @return The value of mili-second unit 01202 * 01203 * @par Description 01204 * The fractional part is been round down. (Because overflow does not 01205 * occur, when "Count = r_ospl_ftimer_spec_t::MaxCount" ) 01206 * 01207 * This function calculates like the following formula. 01208 * @code 01209 * ( Count * ts->msec_Numerator ) / ts->msec_Denominator 01210 * @endcode 01211 */ 01212 INLINE uint32_t R_OSPL_FTIMER_CountToTime( const r_ospl_ftimer_spec_t *const ts, 01213 uint32_t const Count ) 01214 { 01215 uint32_t time; 01216 01217 IF_DQ( ts == NULL ) { 01218 time = 0; 01219 } 01220 else { 01221 time = ( Count * ts->msec_Numerator ) / ts->msec_Denominator; 01222 } 01223 return time; 01224 } 01225 01226 01227 /*********************************************************************** 01228 * Class: r_ospl_table_t 01229 ************************************************************************/ 01230 01231 /** 01232 * @brief Initializes an index table 01233 * 01234 * @param self Index table object 01235 * @param Area First address of the index table 01236 * @param AreaByteSize Size of the index table. See <R_OSPL_TABLE_SIZE> 01237 * @param Is_T_Lock Whether to call <R_OSPL_Start_T_Lock> 01238 * @return None 01239 */ 01240 void R_OSPL_TABLE_InitConst( r_ospl_table_t *const self, 01241 void *const Area, size_t const AreaByteSize, bool_t const Is_T_Lock ); 01242 01243 01244 /** 01245 * @brief Returns index from related key 01246 * 01247 * @param self Index table object 01248 * @param Key Key number 01249 * @param out_Index Output: Related index 01250 * @param TypeOfIfNot Behavior when key was not registerd. See <r_ospl_if_not_t> 01251 * @return Error code. If there is no error, the return value is 0. 01252 */ 01253 errnum_t R_OSPL_TABLE_GetIndex( r_ospl_table_t *const self, const void *const Key, 01254 int_fast32_t *const out_Index, r_ospl_if_not_t const TypeOfIfNot ); 01255 01256 01257 /** 01258 * @brief Separates relationship of specified key and related index 01259 * 01260 * @param self Index table object 01261 * @param Key Key number 01262 * @return None 01263 * 01264 * @par Description 01265 * Error is not raised, even if specified key was already separated. 01266 */ 01267 void R_OSPL_TABLE_Free( r_ospl_table_t *const self, const void *const Key ); 01268 01269 01270 /** 01271 * @brief Print status of specified index table object (for debug) 01272 * 01273 * @param self Index table object 01274 * @return None 01275 */ 01276 #if R_OSPL_DEBUG_TOOL 01277 void R_OSPL_TABLE_Print( r_ospl_table_t *const self ); 01278 #endif 01279 01280 01281 /* Section: Bit flags */ 01282 /** 01283 * @brief Evaluate whether any passed bits are 1 or not 01284 * 01285 * @param Variable The value of target bit flags 01286 * @param ConstValue The value that investigating bits are 1 01287 * @return Whether the any passed bit are 1 01288 */ 01289 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01290 #define IS_BIT_SET( Variable, ConstValue ) \ 01291 ( BIT_And_Sub( Variable, ConstValue ) != 0u ) 01292 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01293 01294 01295 /** 01296 * @brief Evaluate whether any passed bits are 1 or not 01297 * 01298 * @param Variable The value of target bit flags 01299 * @param OrConstValue The value that investigating bits are 1 01300 * @return Whether the any passed bit are 1 01301 */ 01302 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01303 #define IS_ANY_BITS_SET( Variable, OrConstValue ) \ 01304 ( BIT_And_Sub( Variable, OrConstValue ) != 0u ) 01305 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01306 01307 01308 /** 01309 * @brief Evaluate whether all passed bits are 1 or not 01310 * 01311 * @param Variable The value of target bit flags 01312 * @param OrConstValue The value that investigating bits are 1 01313 * @return Whether the all passed bit are 1 01314 */ 01315 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01316 #define IS_ALL_BITS_SET( Variable, OrConstValue ) \ 01317 ( BIT_And_Sub( Variable, OrConstValue ) == (OrConstValue) ) 01318 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01319 01320 01321 /** 01322 * @brief Evaluate whether the passed bit is 0 or not 01323 * 01324 * @param Variable The value of target bit flags 01325 * @param ConstValue The value that investigating bit is 1 01326 * @return Whether the passed bit is 0 01327 */ 01328 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01329 #define IS_BIT_NOT_SET( Variable, ConstValue ) \ 01330 ( BIT_And_Sub( Variable, ConstValue ) == 0u ) 01331 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01332 01333 01334 /** 01335 * @brief Evaluate whether any passed bits are 0 or not 01336 * 01337 * @param Variable The value of target bit flags 01338 * @param OrConstValue The value that investigating bits are 1 01339 * @return Whether the any passed bit are 0 01340 */ 01341 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01342 #define IS_ANY_BITS_NOT_SET( Variable, OrConstValue ) \ 01343 ( BIT_And_Sub( Variable, OrConstValue ) != (OrConstValue) ) 01344 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01345 01346 01347 /** 01348 * @brief Evaluate whether all passed bits are 0 or not 01349 * 01350 * @param Variable The value of target bit flags 01351 * @param OrConstValue The value that investigating bits are 1 01352 * @return Whether the all passed bit are 0 01353 */ 01354 /* ->MISRA 19.7 : For return _Bool type */ /* ->SEC M5.1.3 */ 01355 #define IS_ALL_BITS_NOT_SET( Variable, OrConstValue ) \ 01356 ( BIT_And_Sub( Variable, OrConstValue ) == 0u ) 01357 /* <-MISRA 19.7 */ /* <-SEC M5.1.3 */ 01358 01359 01360 /** 01361 * @brief Sub routine of bitwise operation 01362 * 01363 * @param Variable The value of target bit flags 01364 * @param ConstValue The value that investigating bits are 1 01365 * @return Whether the all passed bit are 0 01366 * 01367 * @par Description 01368 * - This part is for compliant to MISRA 2004 - 19.7. 01369 */ 01370 INLINE uint_fast32_t BIT_And_Sub( bit_flags_fast32_t const Variable, 01371 bit_flags_fast32_t const ConstValue ) 01372 { 01373 return ((Variable) & (ConstValue)); 01374 } 01375 01376 01377 /*********************************************************************** 01378 * About: IS_BIT_SET__Warning 01379 * 01380 * - This is for QAC-3344 warning : MISRA 13.2 Advice : Tests of a value against 01381 * zero should be made explicit, unless the operand is effectively Boolean. 01382 * - This is for QAC-1253 warning : SEC M1.2.2 : A "U" suffix shall be applied 01383 * to all constants of unsigned type. 01384 ************************************************************************/ 01385 01386 01387 /* Section: Error handling and debugging (2) */ 01388 /** 01389 * @brief Breaks here 01390 * 01391 * @par Parameters 01392 * None 01393 * @return None 01394 * 01395 * @par Description 01396 * Does break by calling "R_DebugBreak" function. 01397 * This macro is not influenced the setting of "R_OSPL_ERROR_BREAK" macro. 01398 */ 01399 #define R_DEBUG_BREAK() R_DebugBreak(__FILE__,__LINE__) 01400 01401 01402 /** 01403 * @brief The function callbacked from OSPL for breaking 01404 * 01405 * @param Variable The value of target bit flags 01406 * @param ConstValue The value that investigating bits are 1 01407 * @return Whether the all passed bit are 0 01408 * 01409 * @par Description 01410 * Set a break point at this function. 01411 * In Release configuration, "File = NULL, Line = 0". 01412 * If "File = NULL", "Line" argument is error code. 01413 * This function can be customized by application developer. 01414 */ 01415 void R_DebugBreak( const char_t *const File, int_fast32_t const Line ); 01416 01417 01418 /** 01419 * @brief Breaks here, if it is error state 01420 * 01421 * @par Parameters 01422 * None 01423 * @return None 01424 * 01425 * @par Description 01426 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro was defined 01427 * to be 0. The following descriptions are available, if "R_OSPL_ERROR_BREAK" 01428 * macro was defined to be 1. 01429 * 01430 * Checks the error state of the current thread. 01431 * Call this macro from the last of each thread. 01432 * Does break by calling "R_DebugBreak" function. 01433 * 01434 * If an error was raised, this function calls "printf" with following message. 01435 * Set "error_ID" to "R_OSPL_SET_BREAK_ERROR_ID" 01436 * @code 01437 * <ERROR error_ID="0x1" file="../src/api.c(336)"/> 01438 * @endcode 01439 */ 01440 #if R_OSPL_ERROR_BREAK 01441 #define R_DEBUG_BREAK_IF_ERROR() R_OSPL_DebugBreakIfError(__FILE__,__LINE__) 01442 void R_OSPL_DebugBreakIfError( const char_t *const File, int_fast32_t const Line ); 01443 #else 01444 INLINE void R_DEBUG_BREAK_IF_ERROR(void) {} 01445 #endif 01446 01447 01448 /** 01449 * @brief Raises the error of system unrecoverable 01450 * 01451 * @param e Error code 01452 * @return None 01453 * 01454 * @par Description 01455 * The error of system unrecoverable is the error of impossible to 01456 * - self - recover by process or main system. Example, the heap area was 01457 * broken or there are not any responses from hardware. This error can 01458 * be recoverable by OS or the system controller(e.g. Software reset) 01459 * 01460 * Example, when an error of recovery process was raised, 01461 * "R_OSPL_RaiseUnrecoverable" function must be called. 01462 * 01463 * "R_OSPL_RaiseUnrecoverable" function can be customized by the 01464 * application. By default, it calls "R_DebugBreak" function and falls 01465 * into the infinite loop. 01466 */ 01467 void R_OSPL_RaiseUnrecoverable( errnum_t const e ); 01468 01469 01470 /** 01471 * @brief Merge the error code raised in the finalizing operation 01472 * 01473 * @param CurrentError Current error code 01474 * @param AppendError New append error code 01475 * @return Merged error code 01476 * 01477 * @par Description 01478 * When the state was error state, if other new error was raised, 01479 * new error code is ignored. 01480 * - If "CurrentError != 0", this function returns "CurrentError" argument. 01481 * - If "CurrentError == 0", this function returns "AppendError" argument. 01482 * 01483 * This function can be modify by user. 01484 * 01485 * @par Example 01486 * @code 01487 * ee= Sample(); 01488 * e= R_OSPL_MergeErrNum( e, ee ); 01489 * return e; 01490 * @endcode 01491 */ 01492 INLINE errnum_t R_OSPL_MergeErrNum( errnum_t const CurrentError, errnum_t const AppendError ) 01493 { 01494 errnum_t e; 01495 01496 if ( CurrentError != 0 ) { 01497 e = CurrentError; 01498 } else { 01499 e = AppendError; 01500 } 01501 return e; 01502 } 01503 01504 01505 /** 01506 * @brief Sets an error code to TLS (Thread Local Storage). 01507 * 01508 * @param e Raising error code 01509 * @return None 01510 * 01511 * @par Description 01512 * Usually error code is returned. If API function cannot return any 01513 * error code, API function can have the specification of setting error 01514 * code by "R_OSPL_SetErrNum". 01515 * 01516 * There is this function, if "R_OSPL_TLS_ERROR_CODE" macro was defined 01517 * to be 1. 01518 * This function does nothing, if any error code was stored already in TLS. 01519 * The state does not change to error state, if "R_OSPL_SetErrNum" function 01520 * was called only. See "R_OSPL_GET_ERROR_ID". 01521 */ 01522 #if R_OSPL_TLS_ERROR_CODE 01523 void R_OSPL_SetErrNum( errnum_t const e ); 01524 #endif 01525 01526 01527 /** 01528 * @brief Returns the error code from TLS (Thread Local Storage). 01529 * 01530 * @par Parameters 01531 * None 01532 * @return Error code 01533 * 01534 * @par Description 01535 * Usually error code is returned. If API function cannot return any 01536 * error code, API function may have the specification of getting error 01537 * code by "R_OSPL_GetErrNum". 01538 * 01539 * There is this function, if "R_OSPL_TLS_ERROR_CODE" macro was defined 01540 * to be 1. This function returns 0 after called "R_OSPL_CLEAR_ERROR" 01541 * function. 01542 */ 01543 #if R_OSPL_TLS_ERROR_CODE 01544 errnum_t R_OSPL_GetErrNum(void); 01545 #endif 01546 01547 01548 /** 01549 * @brief Clears the error state 01550 * 01551 * @par Parameters 01552 * None 01553 * @return None 01554 * 01555 * @par Description 01556 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro and 01557 * "R_OSPL_TLS_ERROR_CODE" macro were defined to be 0. The following 01558 * descriptions are available, if "R_OSPL_ERROR_BREAK" macro was 01559 * defined to be 1. 01560 * 01561 * Whether the state is the error state is stored in thread local 01562 * storage. "R_OSPL_GetErrNum" function returns 0 after called this 01563 * function. 01564 * 01565 * If the error state was not cleared, the following descriptions were caused. 01566 * - Breaks at "R_DEBUG_BREAK_IF_ERROR" macro 01567 * - "R_OSPL_SET_BREAK_ERROR_ID" function behaves not expected behavior 01568 * because the count of error is not counted up. 01569 */ 01570 #if R_OSPL_ERROR_BREAK || R_OSPL_TLS_ERROR_CODE 01571 void R_OSPL_CLEAR_ERROR(void); 01572 #else 01573 INLINE void R_OSPL_CLEAR_ERROR(void) {} /* QAC 3138 */ 01574 #endif 01575 01576 01577 /** 01578 * @brief Returns the number of current error 01579 * 01580 * @par Parameters 01581 * None 01582 * @return The number of current error 01583 * 01584 * @par Description 01585 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro was defined 01586 * to be 0. The following descriptions are available, if "R_OSPL_ERROR_BREAK" 01587 * macro was defined to be 1. 01588 * 01589 * This function returns 0, if any errors were not raised. 01590 * 01591 * This function returns 1, if first error was raised. 01592 * 01593 * After that, this function returns 2, if second error was raised after 01594 * calling "R_OSPL_CLEAR_ERROR" function. 01595 * This function does not return 0 after that the error was cleared by 01596 * calling "R_OSPL_CLEAR_ERROR". 01597 * The number of current error is running number in the whole of system 01598 * (all threads). 01599 * 01600 * Error is raised by following macros. 01601 * @code 01602 * IF, IF_D, ASSERT_R, ASSERT_D 01603 * @endcode 01604 * The process breaks at a moment of error raised, if the number of current 01605 * error was set to "R_OSPL_SET_BREAK_ERROR_ID" macro. 01606 */ 01607 #if R_OSPL_ERROR_BREAK 01608 int_fast32_t R_OSPL_GET_ERROR_ID(void); 01609 #else 01610 INLINE int_fast32_t R_OSPL_GET_ERROR_ID(void) 01611 { 01612 return -1; 01613 } 01614 #endif 01615 01616 01617 /** 01618 * @brief Register to break at raising error at the moment 01619 * 01620 * @param ID Breaking number of error 01621 * @return None 01622 * 01623 * @par Description 01624 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro was defined 01625 * to be 0. The following descriptions are available, if "R_OSPL_ERROR_BREAK" 01626 * macro was defined to be 1. 01627 * 01628 * Set a break point at "R_DebugBreak" function, when the process breaks 01629 * at the error raised code. 01630 * 01631 * The number of "ErrorID" argument can be known by "R_DEBUG_BREAK_IF_ERROR" 01632 * macro or "R_OSPL_GET_ERROR_ID" macro. 01633 * - In multi - threading environment, the number of "ErrorID" argument is the 01634 * number of raised errors in all threads. But when "ErrorID" argument was 01635 * set to be over 2, call "R_OSPL_SET_DEBUG_WORK" function before calling 01636 * "R_OSPL_SET_BREAK_ERROR_ID" function. 01637 * 01638 * The following code breaks at first error. 01639 * @code 01640 * R_OSPL_SET_BREAK_ERROR_ID( 1 ); 01641 * @endcode 01642 * 01643 * The following code breaks at next error after resuming from meny errors. 01644 * @code 01645 * R_OSPL_SET_BREAK_ERROR_ID( R_OSPL_GET_ERROR_ID() + 1 ); 01646 * @endcode 01647 */ 01648 #if R_OSPL_ERROR_BREAK 01649 void R_OSPL_SET_BREAK_ERROR_ID( int_fast32_t ID ); 01650 #else 01651 INLINE void R_OSPL_SET_BREAK_ERROR_ID( int_fast32_t const ID ) 01652 { 01653 R_UNREFERENCED_VARIABLE( ID ); 01654 } 01655 #endif 01656 01657 01658 /** 01659 * @brief Set the debug work area 01660 * 01661 * @param WorkArea Start address of work area 01662 * @param WorkAreaSize Size of work area (byte). See. <R_OSPL_DEBUG_WORK_SIZE> 01663 * @return None 01664 * 01665 * @par Description 01666 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro was defined 01667 * to be 0. The following descriptions are available, if "R_OSPL_ERROR_BREAK" 01668 * macro was defined to be 1. 01669 * 01670 * Set the debug work area, when "R_OSPL_SET_BREAK_ERROR_ID" function 01671 * supports multi thread. "E_NO_DEBUG_TLS" error is raised, if the debug 01672 * work area was not set, when errors was raised in 2 or more threads. 01673 * It is not necessary to call this function, if error handling did by one 01674 * thread only. 01675 * 01676 * @par Example 01677 * @code 01678 * #if R_OSPL_ERROR_BREAK 01679 * #define GS_MAX_THREAD 10 01680 * static uint8_t gs_DebugWorkArea[ R_OSPL_DEBUG_WORK_SIZE( GS_MAX_THREAD ) ]; 01681 * #endif 01682 * 01683 * R_OSPL_SET_DEBUG_WORK( gs_DebugWorkArea, sizeof(gs_DebugWorkArea) ); 01684 * @endcode 01685 */ 01686 #if R_OSPL_ERROR_BREAK 01687 void R_OSPL_SET_DEBUG_WORK( void *WorkArea, uint32_t WorkAreaSize ); 01688 #else 01689 INLINE void R_OSPL_SET_DEBUG_WORK( const void *const WorkArea, uint32_t const WorkAreaSize ) 01690 { 01691 R_UNREFERENCED_VARIABLE_2( WorkArea, WorkAreaSize ); 01692 } 01693 #endif 01694 01695 01696 /** 01697 * @brief Returns debbug information of current thread. 01698 * 01699 * @par Parameters 01700 * None 01701 * @return Debbug information of current thread. 01702 */ 01703 #if R_OSPL_ERROR_BREAK 01704 r_ospl_error_t *R_OSPL_GetCurrentThreadError(void); 01705 #endif 01706 01707 01708 /** 01709 * @brief Modifies count of objects that current thread has locked. 01710 * 01711 * @param Plus The value of adding to the counter. 01712 * @return None 01713 * 01714 * @par Description 01715 * The counter is subtracted, if this argument was minus. 01716 * 01717 * Drivers calls this function. 01718 * This function is not called from OSPL. 01719 * This function does nothing, if "R_OSPL_ERROR_BREAK" macro is 0. 01720 */ 01721 #if R_OSPL_ERROR_BREAK 01722 #if R_OSPL_IS_PREEMPTION 01723 void R_OSPL_MODIFY_THREAD_LOCKED_COUNT( int_fast32_t Plus ); 01724 #else 01725 INLINE void R_OSPL_MODIFY_THREAD_LOCKED_COUNT( int_fast32_t Plus ) {} 01726 #endif 01727 #else 01728 INLINE void R_OSPL_MODIFY_THREAD_LOCKED_COUNT( int_fast32_t Plus ) {} 01729 #endif 01730 01731 01732 /** 01733 * @brief Returns count of objects that current thread has locked. 01734 * 01735 * @par Parameters 01736 * None 01737 * @return Count of objects that current thread has locked 01738 * 01739 * @par Description 01740 * This function returns 0, if "R_OSPL_ERROR_BREAK" macro is 0. 01741 */ 01742 #if R_OSPL_ERROR_BREAK 01743 #if R_OSPL_IS_PREEMPTION 01744 int_fast32_t R_OSPL_GET_THREAD_LOCKED_COUNT(void); 01745 #else 01746 INLINE int_fast32_t R_OSPL_GET_THREAD_LOCKED_COUNT(void) 01747 { 01748 return 0; 01749 } 01750 #endif 01751 #else 01752 INLINE int_fast32_t R_OSPL_GET_THREAD_LOCKED_COUNT(void) 01753 { 01754 return 0; 01755 } 01756 #endif 01757 01758 01759 /* Section: Accessing to register bit field */ 01760 /** 01761 * @brief Reads modifies writes for bit field of 32bit register. 01762 * 01763 * @param in_out_Register Address of accessing register 01764 * @param Mask Mask of accessing bit field 01765 * @param Shift Shift count. Lowest bit number 01766 * @param Value Writing value before shift to the bit field 01767 * @return None 01768 */ 01769 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01770 01771 /* ->SEC R3.6.2(QAC-3345) */ 01772 /* Volatile access at left of "=" and right of "=". But this is not depend on compiler spcifications. */ 01773 /* ->SEC M1.2.2(QAC-1259) */ 01774 /* If "Value" is signed, this is depend on CPU bit width. This expects 32bit CPU. But driver code is no problem. */ 01775 01776 #define R_OSPL_SET_TO_32_BIT_REGISTER( in_out_Register, Mask, Shift, Value ) \ 01777 ( *(volatile uint32_t*)(in_out_Register) = (uint32_t)( \ 01778 ( ((uint32_t) *(volatile uint32_t*)(in_out_Register)) & \ 01779 ~(Mask) ) | ( (Mask) & ( ( (uint_fast32_t)(Value) << (Shift) ) & (Mask) ) ) ) ) 01780 /* This code is optimized well. */ 01781 01782 /* <-SEC M1.2.2(QAC-1259) */ 01783 /* <-SEC R3.6.2(QAC-3345) */ 01784 01785 #else 01786 01787 INLINE void R_OSPL_SET_TO_32_BIT_REGISTER( volatile uint32_t *const Register, 01788 uint32_t const Mask, int_fast32_t const Shift, uint32_t const Value ) 01789 { 01790 uint32_t reg_value; 01791 01792 IF_DQ ( Register == NULL ) {} 01793 else { 01794 reg_value = *Register; 01795 reg_value = ( reg_value & ~Mask ) | ( ( Value << Shift ) & Mask ); 01796 *Register = reg_value; 01797 } 01798 } 01799 01800 #endif 01801 01802 01803 /** 01804 * @brief Reads modifies writes for bit field of 16bit register. 01805 * 01806 * @param in_out_Register Address of accessing register 01807 * @param Mask Mask of accessing bit field 01808 * @param Shift Shift count. Lowest bit number 01809 * @param Value Writing value before shift to the bit field 01810 * @return None 01811 */ 01812 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01813 01814 /* ->SEC R3.6.2(QAC-3345) */ 01815 /* Volatile access at left of "=" and right of "=". But this is not depend on compiler spcifications. */ 01816 /* ->SEC M1.2.2(QAC-1259) */ 01817 /* If "Value" is signed, this is depend on CPU bit width. This expects 32bit CPU. But driver code is no problem. */ 01818 01819 #define R_OSPL_SET_TO_16_BIT_REGISTER( in_out_Register, Mask, Shift, Value ) \ 01820 ( *(volatile uint16_t*)(in_out_Register) = (uint16_t)( \ 01821 ( ((uint16_t) *(volatile uint16_t*)(in_out_Register)) & \ 01822 ~(Mask) ) | ( (Mask) & ( ( (uint_fast16_t)(Value) << (Shift) ) & (Mask) ) ) ) ) 01823 /* This code is optimized well. */ 01824 01825 01826 /* <-SEC M1.2.2(QAC-1259) */ 01827 /* <-SEC R3.6.2(QAC-3345) */ 01828 01829 #else 01830 01831 INLINE void R_OSPL_SET_TO_16_BIT_REGISTER( volatile uint16_t *const Register, 01832 uint16_t const Mask, int_fast32_t const Shift, uint16_t const Value ) 01833 { 01834 uint16_t reg_value; 01835 01836 IF_DQ ( Register == NULL ) {} 01837 else { 01838 reg_value = *Register; 01839 reg_value = (uint16_t)( ( (uint_fast32_t) reg_value & ~(uint_fast32_t) Mask ) | 01840 ( ( (uint_fast32_t) Value << Shift ) & (uint_fast32_t) Mask ) ); 01841 /* Cast is for SEC R2.4.2 */ 01842 *Register = reg_value; 01843 } 01844 } 01845 01846 #endif 01847 01848 01849 /** 01850 * @brief Reads modifies writes for bit field of 8bit register. 01851 * 01852 * @param in_out_Register Address of accessing register 01853 * @param Mask Mask of accessing bit field 01854 * @param Shift Shift count. Lowest bit number 01855 * @param Value Writing value before shift to the bit field 01856 * @return None 01857 */ 01858 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01859 01860 /* ->SEC R3.6.2(QAC-3345) */ 01861 /* Volatile access at left of "=" and right of "=". But this is not depend on compiler spcifications. */ 01862 /* ->SEC M1.2.2(QAC-1259) */ 01863 /* If "Value" is signed, this is depend on CPU bit width. This expects 32bit CPU. But driver code is no problem. */ 01864 01865 01866 #define R_OSPL_SET_TO_8_BIT_REGISTER( in_out_Register, Mask, Shift, Value ) \ 01867 ( *(volatile uint8_t*)(in_out_Register) = (uint8_t)( \ 01868 ( ((uint8_t) *(volatile uint8_t*)(in_out_Register)) & \ 01869 ~(Mask) ) | ( (Mask) & ( ( (uint_fast8_t)(Value) << (Shift) ) & (Mask) ) ) ) ) 01870 /* This code is optimized well. */ 01871 01872 /* <-SEC M1.2.2(QAC-1259) */ 01873 /* <-SEC R3.6.2(QAC-3345) */ 01874 01875 #else 01876 01877 INLINE void R_OSPL_SET_TO_8_BIT_REGISTER( volatile uint8_t *const Register, 01878 uint8_t const Mask, int_fast32_t const Shift, uint8_t const Value ) 01879 { 01880 uint8_t reg_value; 01881 01882 IF_DQ ( Register == NULL ) {} 01883 else { 01884 reg_value = *Register; 01885 reg_value = (uint8_t)( ( (uint_fast32_t) reg_value & ~(uint_fast32_t) Mask ) | 01886 ( ( (uint_fast32_t) Value << Shift ) & (uint_fast32_t) Mask ) ); 01887 /* Cast is for SEC R2.4.2 */ 01888 *Register = reg_value; 01889 } 01890 } 01891 01892 #endif 01893 01894 01895 /** 01896 * @brief Reads for bit field of 32bit register. 01897 * 01898 * @param RegisterValueAddress Address of accessing register 01899 * @param Mask Mask of accessing bit field 01900 * @param Shift Shift count. Lowest bit number 01901 * @return Read value after shift 01902 */ 01903 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01904 01905 /* ->SEC R3.6.2(QAC-3345) */ 01906 /* Volatile access at &(get address), cast and *(memory load). But this is not double volatile access. */ 01907 /* RegisterValueAddress is for avoid QAC-0310,QAC-3345 by cast code at caller. */ 01908 01909 #define R_OSPL_GET_FROM_32_BIT_REGISTER( RegisterValueAddress, Mask, Shift ) \ 01910 ( (uint32_t)( ( (uint32_t)*(volatile const uint32_t*) (RegisterValueAddress) \ 01911 & (uint_fast32_t)(Mask) ) >> (Shift) ) ) 01912 /* This code is optimized well. */ 01913 01914 /* <-SEC R3.6.2(QAC-3345) */ 01915 01916 #else /* __QAC_ARM_H__ */ /* This code must be tested defined "__QAC_ARM_H__" */ 01917 01918 01919 /* This inline functions is not expanded on __CC_ARM 5.15 */ 01920 INLINE uint32_t R_OSPL_GET_FROM_32_BIT_REGISTER( volatile const uint32_t *const RegisterAddress, 01921 uint32_t const Mask, int_fast32_t const Shift ) 01922 { 01923 uint32_t reg_value; 01924 01925 IF_DQ ( RegisterAddress == NULL ) { 01926 enum { num = 0x0EDEDEDE }; /* SEC M1.10.1 */ 01927 reg_value = num; 01928 } 01929 else { 01930 reg_value = *RegisterAddress; 01931 reg_value = ( reg_value & Mask ) >> Shift; 01932 } 01933 return reg_value; 01934 } 01935 01936 #endif 01937 01938 01939 /** 01940 * @brief Reads for bit field of 16bit register. 01941 * 01942 * @param RegisterValueAddress Address of accessing register 01943 * @param Mask Mask of accessing bit field 01944 * @param Shift Shift count. Lowest bit number 01945 * @return Read value after shift 01946 */ 01947 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01948 01949 /* ->SEC R3.6.2(QAC-3345) */ 01950 /* Volatile access at &(get address), cast and *(memory load). But this is not double volatile access. */ 01951 /* RegisterValueAddress is for avoid QAC-0310,QAC-3345 by cast code at caller. */ 01952 01953 #define R_OSPL_GET_FROM_16_BIT_REGISTER( RegisterValueAddress, Mask, Shift ) \ 01954 ( (uint16_t)( ( (uint_fast32_t)*(volatile const uint16_t*) (RegisterValueAddress) \ 01955 & (uint_fast16_t)(Mask) ) >> (Shift) ) ) 01956 /* This code is optimized well. */ 01957 01958 /* <-SEC R3.6.2(QAC-3345) */ 01959 01960 #else /* __QAC_ARM_H__ */ /* This code must be tested defined "__QAC_ARM_H__" */ 01961 01962 /* This inline functions is not expanded on __CC_ARM 5.15 */ 01963 INLINE uint16_t R_OSPL_GET_FROM_16_BIT_REGISTER( volatile const uint16_t *const RegisterAddress, 01964 uint16_t const Mask, int_fast32_t const Shift ) 01965 { 01966 uint16_t reg_value; 01967 01968 IF_DQ ( RegisterAddress == NULL ) { 01969 enum { num = 0xDEDE }; /* SEC M1.10.1 */ 01970 reg_value = num; 01971 } 01972 else { 01973 reg_value = *RegisterAddress; 01974 reg_value = (uint16_t)( ( (uint_fast32_t) reg_value & (uint_fast32_t) Mask ) >> Shift ); 01975 /* Cast is for SEC R2.4.2 */ 01976 } 01977 return reg_value; 01978 } 01979 01980 #endif 01981 01982 01983 /** 01984 * @brief Reads for bit field of 8bit register. 01985 * 01986 * @param RegisterValueAddress Address of accessing register 01987 * @param Mask Mask of accessing bit field 01988 * @param Shift Shift count. Lowest bit number 01989 * @return Read value after shift 01990 */ 01991 #if R_OSPL_BIT_FIELD_ACCESS_MACRO 01992 01993 /* ->SEC R3.6.2(QAC-3345) */ 01994 /* Volatile access at &(get address), cast and *(memory load). But this is not double volatile access. */ 01995 /* RegisterValueAddress is for avoid QAC-0310,QAC-3345 by cast code at caller. */ 01996 01997 #define R_OSPL_GET_FROM_8_BIT_REGISTER( RegisterValueAddress, Mask, Shift ) \ 01998 ( (uint8_t)( ( (uint_fast32_t)*(volatile const uint8_t*) (RegisterValueAddress) \ 01999 & (uint_fast8_t)(Mask) ) >> (Shift) ) ) 02000 /* This code is optimized well. */ 02001 02002 /* <-SEC R3.6.2(QAC-3345) */ 02003 02004 #else /* __QAC_ARM_H__ */ /* This code must be tested defined "__QAC_ARM_H__" */ 02005 02006 /* This inline functions is not expanded on __CC_ARM 5.15 */ 02007 INLINE uint8_t R_OSPL_GET_FROM_8_BIT_REGISTER( volatile const uint8_t *const RegisterAddress, 02008 uint8_t const Mask, int_fast32_t const Shift ) 02009 { 02010 uint8_t reg_value; 02011 02012 IF_DQ ( RegisterAddress == NULL ) { 02013 enum { num = 0xDE }; /* SEC M1.10.1 */ 02014 reg_value = num; 02015 } 02016 else { 02017 reg_value = *RegisterAddress; 02018 reg_value = (uint8_t)( ( (uint_fast32_t) reg_value & (uint_fast32_t) Mask ) >> Shift ); 02019 /* Cast is for SEC R2.4.2 */ 02020 } 02021 return reg_value; 02022 } 02023 02024 #endif 02025 02026 02027 /*********************************************************************** 02028 * End of File: 02029 ************************************************************************/ 02030 02031 #ifdef __cplusplus 02032 } /* extern "C" */ 02033 #endif /* __cplusplus */ 02034 02035 #endif /* R_OSPL_H */ 02036
Generated on Wed Jul 13 2022 05:33:36 by
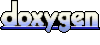