
test public
Dependencies: HttpServer_snapshot_mbed-os
mcu_interrupts_typedef.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file mcu_interrupts_typedef.h 00025 * @brief Interrupt related FIT BSP. Data types. 00026 * 00027 * $Module: OSPL $ $PublicVersion: 0.90 $ (=R_OSPL_VERSION) 00028 * $Rev: 35 $ 00029 * $Date:: 2014-04-15 21:38:18 +0900#$ 00030 */ 00031 00032 #ifndef MCU_INTERRUPTS_TYPEDEF_H 00033 #define MCU_INTERRUPTS_TYPEDEF_H 00034 00035 00036 /****************************************************************************** 00037 Includes <System Includes> , "Project Includes" 00038 ******************************************************************************/ 00039 #include "r_typedefs.h" 00040 #include "r_multi_compiler_typedef.h" 00041 #if IS_MBED_USED 00042 #include "cmsis.h" 00043 #else 00044 #include "Renesas_RZ_A1.h" 00045 #endif 00046 00047 #ifdef __cplusplus 00048 extern "C" { 00049 #endif /* __cplusplus */ 00050 00051 00052 /****************************************************************************** 00053 Typedef definitions 00054 ******************************************************************************/ 00055 00056 /** 00057 * @enum bsp_int_err_t 00058 * @brief Error code defined by FIT BSP. 00059 * 00060 * - BSP_INT_SUCCESS - 0 00061 * - BSP_INT_ERR_NO_REGISTERED_CALLBACK - 0x2101 00062 * - BSP_INT_ERR_INVALID_ARG - 1 00063 * - BSP_INT_ERR_UNSUPPORTED - 15 00064 */ 00065 typedef enum { 00066 BSP_INT_SUCCESS = 0, 00067 BSP_INT_ERR_NO_REGISTERED_CALLBACK = 0x2101, 00068 BSP_INT_ERR_INVALID_ARG = 1, 00069 BSP_INT_ERR_UNSUPPORTED = 15 00070 } 00071 bsp_int_err_t; 00072 00073 00074 /** 00075 * @brief Interrupt Handler 00076 * 00077 * @param int_sense uint32_t 00078 * @return None 00079 */ 00080 typedef void (* bsp_int_cb_t )(void); 00081 00082 00083 /** 00084 * @enum bsp_int_cmd_t 00085 * @brief Control command related to the interrupt. 00086 * 00087 * - BSP_INT_CMD_INTERRUPT_ENABLE - 0 00088 * - BSP_INT_CMD_INTERRUPT_DISABLE - 1 00089 */ 00090 typedef enum { 00091 BSP_INT_CMD_INTERRUPT_ENABLE, 00092 BSP_INT_CMD_INTERRUPT_DISABLE 00093 } bsp_int_cmd_t; 00094 00095 00096 /****************************************************************************** 00097 Macro definitions 00098 ******************************************************************************/ 00099 00100 /** 00101 * @def FIT_NO_PTR 00102 * @brief None as pointer type 00103 */ 00104 #define FIT_NO_PTR ( (void*) 0 ) 00105 00106 00107 /** 00108 * @def FIT_NO_FUNC 00109 * @brief None as function pointer type 00110 */ 00111 #define FIT_NO_FUNC ( (void*) 0 ) 00112 00113 00114 /** 00115 * @enum bsp_int_src_t 00116 * @brief Interrupt number. 00117 */ 00118 typedef IRQn_Type bsp_int_src_t; 00119 #if defined(TARGET_RZ_A1XX) 00120 00121 #define BSP_INT_SRC_SW0 ((IRQn_Type)(0)) /* GIC software interrupt */ 00122 #define BSP_INT_SRC_SW1 ((IRQn_Type)(1)) /* */ 00123 #define BSP_INT_SRC_SW2 ((IRQn_Type)(2)) /* */ 00124 #define BSP_INT_SRC_SW3 ((IRQn_Type)(3)) /* */ 00125 #define BSP_INT_SRC_SW4 ((IRQn_Type)(4)) /* */ 00126 #define BSP_INT_SRC_SW5 ((IRQn_Type)(5)) /* */ 00127 #define BSP_INT_SRC_SW6 ((IRQn_Type)(6)) /* */ 00128 #define BSP_INT_SRC_SW7 ((IRQn_Type)(7)) /* */ 00129 #define BSP_INT_SRC_SW8 ((IRQn_Type)(8)) /* */ 00130 #define BSP_INT_SRC_SW9 ((IRQn_Type)(9)) /* */ 00131 #define BSP_INT_SRC_SW10 ((IRQn_Type)(10)) /* */ 00132 #define BSP_INT_SRC_SW11 ((IRQn_Type)(11)) /* */ 00133 #define BSP_INT_SRC_SW12 ((IRQn_Type)(12)) /* */ 00134 #define BSP_INT_SRC_SW13 ((IRQn_Type)(13)) /* */ 00135 #define BSP_INT_SRC_SW14 ((IRQn_Type)(14)) /* */ 00136 #define BSP_INT_SRC_SW15 ((IRQn_Type)(15)) /* */ 00137 #define BSP_INT_SRC_PMUIRQ0 ((IRQn_Type)(16)) /* CPU */ 00138 #define BSP_INT_SRC_COMMRX0 ((IRQn_Type)(17)) /* */ 00139 #define BSP_INT_SRC_COMMTX0 ((IRQn_Type)(18)) /* */ 00140 #define BSP_INT_SRC_CTIIRQ0 ((IRQn_Type)(19)) /* */ 00141 #define BSP_INT_SRC_IRQ0 ((IRQn_Type)(32)) /* IRQ */ 00142 #define BSP_INT_SRC_IRQ1 ((IRQn_Type)(33)) /* */ 00143 #define BSP_INT_SRC_IRQ2 ((IRQn_Type)(34)) /* */ 00144 #define BSP_INT_SRC_IRQ3 ((IRQn_Type)(35)) /* */ 00145 #define BSP_INT_SRC_IRQ4 ((IRQn_Type)(36)) /* */ 00146 #define BSP_INT_SRC_IRQ5 ((IRQn_Type)(37)) /* */ 00147 #define BSP_INT_SRC_IRQ6 ((IRQn_Type)(38)) /* */ 00148 #define BSP_INT_SRC_IRQ7 ((IRQn_Type)(39)) /* */ 00149 #define BSP_INT_SRC_PL310ERR ((IRQn_Type)(40)) /* Level 2 cache */ 00150 #define BSP_INT_SRC_DMAINT0 ((IRQn_Type)(41)) /* Direct memory access controller */ 00151 #define BSP_INT_SRC_DMAINT1 ((IRQn_Type)(42)) /* */ 00152 #define BSP_INT_SRC_DMAINT2 ((IRQn_Type)(43)) /* */ 00153 #define BSP_INT_SRC_DMAINT3 ((IRQn_Type)(44)) /* */ 00154 #define BSP_INT_SRC_DMAINT4 ((IRQn_Type)(45)) /* */ 00155 #define BSP_INT_SRC_DMAINT5 ((IRQn_Type)(46)) /* */ 00156 #define BSP_INT_SRC_DMAINT6 ((IRQn_Type)(47)) /* */ 00157 #define BSP_INT_SRC_DMAINT7 ((IRQn_Type)(48)) /* */ 00158 #define BSP_INT_SRC_DMAINT8 ((IRQn_Type)(49)) /* */ 00159 #define BSP_INT_SRC_DMAINT9 ((IRQn_Type)(50)) /* */ 00160 #define BSP_INT_SRC_DMAINT10 ((IRQn_Type)(51)) /* */ 00161 #define BSP_INT_SRC_DMAINT11 ((IRQn_Type)(52)) /* */ 00162 #define BSP_INT_SRC_DMAINT12 ((IRQn_Type)(53)) /* */ 00163 #define BSP_INT_SRC_DMAINT13 ((IRQn_Type)(54)) /* */ 00164 #define BSP_INT_SRC_DMAINT14 ((IRQn_Type)(55)) /* */ 00165 #define BSP_INT_SRC_DMAINT15 ((IRQn_Type)(56)) /* */ 00166 #define BSP_INT_SRC_DMAERR ((IRQn_Type)(57)) /* */ 00167 #define BSP_INT_SRC_USBI0 ((IRQn_Type)(73)) /* USB 2.0 host/function module */ 00168 #define BSP_INT_SRC_USBI1 ((IRQn_Type)(74)) /* */ 00169 #define BSP_INT_SRC_S0_VI_VSYNC0 ((IRQn_Type)(75)) /* Video display controller 5 */ 00170 #define BSP_INT_SRC_S0_LO_VSYNC0 ((IRQn_Type)(76)) /* */ 00171 #define BSP_INT_SRC_S0_VSYNCERR0 ((IRQn_Type)(77)) /* */ 00172 #define BSP_INT_SRC_GR3_VLINE0 ((IRQn_Type)(78)) /* */ 00173 #define BSP_INT_SRC_S0_VFIELD0 ((IRQn_Type)(79)) /* */ 00174 #define BSP_INT_SRC_IV1_VBUFERR0 ((IRQn_Type)(80)) /* */ 00175 #define BSP_INT_SRC_IV3_VBUFERR0 ((IRQn_Type)(81)) /* */ 00176 #define BSP_INT_SRC_IV5_VBUFERR0 ((IRQn_Type)(82)) /* */ 00177 #define BSP_INT_SRC_IV6_VBUFERR0 ((IRQn_Type)(83)) /* */ 00178 #define BSP_INT_SRC_S0_WLINE0 ((IRQn_Type)(84)) /* */ 00179 #define BSP_INT_SRC_S1_VI_VSYNC0 ((IRQn_Type)(85)) /* */ 00180 #define BSP_INT_SRC_S1_LO_VSYNC0 ((IRQn_Type)(86)) /* */ 00181 #define BSP_INT_SRC_S1_VSYNCERR0 ((IRQn_Type)(87)) /* */ 00182 #define BSP_INT_SRC_S1_VFIELD0 ((IRQn_Type)(88)) /* */ 00183 #define BSP_INT_SRC_IV2_VBUFERR0 ((IRQn_Type)(89)) /* */ 00184 #define BSP_INT_SRC_IV4_VBUFERR0 ((IRQn_Type)(90)) /* */ 00185 #define BSP_INT_SRC_S1_WLINE0 ((IRQn_Type)(91)) /* */ 00186 #define BSP_INT_SRC_OIR_VI_VSYNC0 ((IRQn_Type)(92)) /* */ 00187 #define BSP_INT_SRC_OIR_LO_VSYNC0 ((IRQn_Type)(93)) /* */ 00188 #define BSP_INT_SRC_OIR_VSYNCERR0 ((IRQn_Type)(94)) /* */ 00189 #define BSP_INT_SRC_OIR_VFIELD0 ((IRQn_Type)(95)) /* */ 00190 #define BSP_INT_SRC_IV7_VBUFERR0 ((IRQn_Type)(96)) /* */ 00191 #define BSP_INT_SRC_IV8_VBUFERR0 ((IRQn_Type)(97)) /* */ 00192 #define BSP_INT_SRC_OIR_WLINE0 ((IRQn_Type)(98)) /* */ 00193 #define BSP_INT_SRC_S0_VI_VSYNC1 ((IRQn_Type)(99)) /* */ 00194 #define BSP_INT_SRC_S0_LO_VSYNC1 ((IRQn_Type)(100)) /* */ 00195 #define BSP_INT_SRC_S0_VSYNCERR1 ((IRQn_Type)(101)) /* */ 00196 #define BSP_INT_SRC_GR3_VLINE1 ((IRQn_Type)(102)) /* */ 00197 #define BSP_INT_SRC_S0_VFIELD1 ((IRQn_Type)(103)) /* */ 00198 #define BSP_INT_SRC_IV1_VBUFERR1 ((IRQn_Type)(104)) /* */ 00199 #define BSP_INT_SRC_IV3_VBUFERR1 ((IRQn_Type)(105)) /* */ 00200 #define BSP_INT_SRC_IV5_VBUFERR1 ((IRQn_Type)(106)) /* */ 00201 #define BSP_INT_SRC_IV6_VBUFERR1 ((IRQn_Type)(107)) /* */ 00202 #define BSP_INT_SRC_S0_WLINE1 ((IRQn_Type)(108)) /* */ 00203 #define BSP_INT_SRC_S1_VI_VSYNC1 ((IRQn_Type)(109)) /* */ 00204 #define BSP_INT_SRC_S1_LO_VSYNC1 ((IRQn_Type)(110)) /* */ 00205 #define BSP_INT_SRC_S1_VSYNCERR1 ((IRQn_Type)(111)) /* */ 00206 #define BSP_INT_SRC_S1_VFIELD1 ((IRQn_Type)(112)) /* */ 00207 #define BSP_INT_SRC_IV2_VBUFERR1 ((IRQn_Type)(113)) /* */ 00208 #define BSP_INT_SRC_IV4_VBUFERR1 ((IRQn_Type)(114)) /* */ 00209 #define BSP_INT_SRC_S1_WLINE1 ((IRQn_Type)(115)) /* */ 00210 #define BSP_INT_SRC_OIR_VI_VSYNC1 ((IRQn_Type)(116)) /* */ 00211 #define BSP_INT_SRC_OIR_LO_VSYNC1 ((IRQn_Type)(117)) /* */ 00212 #define BSP_INT_SRC_OIR_VLINE1 ((IRQn_Type)(118)) /* */ 00213 #define BSP_INT_SRC_OIR_VFIELD1 ((IRQn_Type)(119)) /* */ 00214 #define BSP_INT_SRC_IV7_VBUFERR1 ((IRQn_Type)(120)) /* */ 00215 #define BSP_INT_SRC_IV8_VBUFERR1 ((IRQn_Type)(121)) /* */ 00216 #define BSP_INT_SRC_OIR_WLINE1 ((IRQn_Type)(122)) /* */ 00217 #define BSP_INT_SRC_IMRDI ((IRQn_Type)(123)) /* Image renderer */ 00218 #define BSP_INT_SRC_IMR2I0 ((IRQn_Type)(124)) /* */ 00219 #define BSP_INT_SRC_IMR2I1 ((IRQn_Type)(125)) /* */ 00220 #define BSP_INT_SRC_JEDI ((IRQn_Type)(126)) /* JPEG Codec unit */ 00221 #define BSP_INT_SRC_JDTI ((IRQn_Type)(127)) /* */ 00222 #define BSP_INT_SRC_CMP0 ((IRQn_Type)(128)) /* Display out comparison unit */ 00223 #define BSP_INT_SRC_CMP1 ((IRQn_Type)(129)) /* */ 00224 #define BSP_INT_SRC_INT0 ((IRQn_Type)(130)) /* OpenVG-Compliant Renesas graphics processor */ 00225 #define BSP_INT_SRC_INT1 ((IRQn_Type)(131)) /* */ 00226 #define BSP_INT_SRC_INT2 ((IRQn_Type)(132)) /* */ 00227 #define BSP_INT_SRC_INT3 ((IRQn_Type)(133)) /* */ 00228 #define BSP_INT_SRC_OSTM0TINT ((IRQn_Type)(134)) /* OS timer */ 00229 #define BSP_INT_SRC_OSTM1TINT ((IRQn_Type)(135)) /* */ 00230 #define BSP_INT_SRC_CMI ((IRQn_Type)(136)) /* Bus state controller */ 00231 #define BSP_INT_SRC_WTOUT ((IRQn_Type)(137)) /* */ 00232 #define BSP_INT_SRC_ITI ((IRQn_Type)(138)) /* Watchdog timer */ 00233 #define BSP_INT_SRC_TGI0A ((IRQn_Type)(139)) /* Multi-function timer pulse unit 2 */ 00234 #define BSP_INT_SRC_TGI0B ((IRQn_Type)(140)) /* */ 00235 #define BSP_INT_SRC_TGI0C ((IRQn_Type)(141)) /* */ 00236 #define BSP_INT_SRC_TGI0D ((IRQn_Type)(142)) /* */ 00237 #define BSP_INT_SRC_TGI0V ((IRQn_Type)(143)) /* */ 00238 #define BSP_INT_SRC_TGI0E ((IRQn_Type)(144)) /* */ 00239 #define BSP_INT_SRC_TGI0F ((IRQn_Type)(145)) /* */ 00240 #define BSP_INT_SRC_TGI1A ((IRQn_Type)(146)) /* */ 00241 #define BSP_INT_SRC_TGI1B ((IRQn_Type)(147)) /* */ 00242 #define BSP_INT_SRC_TGI1V ((IRQn_Type)(148)) /* */ 00243 #define BSP_INT_SRC_TGI1U ((IRQn_Type)(149)) /* */ 00244 #define BSP_INT_SRC_TGI2A ((IRQn_Type)(150)) /* */ 00245 #define BSP_INT_SRC_TGI2B ((IRQn_Type)(151)) /* */ 00246 #define BSP_INT_SRC_TGI2V ((IRQn_Type)(152)) /* */ 00247 #define BSP_INT_SRC_TGI2U ((IRQn_Type)(153)) /* */ 00248 #define BSP_INT_SRC_TGI3A ((IRQn_Type)(154)) /* */ 00249 #define BSP_INT_SRC_TGI3B ((IRQn_Type)(155)) /* */ 00250 #define BSP_INT_SRC_TGI3C ((IRQn_Type)(156)) /* */ 00251 #define BSP_INT_SRC_TGI3D ((IRQn_Type)(157)) /* */ 00252 #define BSP_INT_SRC_TGI3V ((IRQn_Type)(158)) /* */ 00253 #define BSP_INT_SRC_TGI4A ((IRQn_Type)(159)) /* */ 00254 #define BSP_INT_SRC_TGI4B ((IRQn_Type)(160)) /* */ 00255 #define BSP_INT_SRC_TGI4C ((IRQn_Type)(161)) /* */ 00256 #define BSP_INT_SRC_TGI4D ((IRQn_Type)(162)) /* */ 00257 #define BSP_INT_SRC_TGI4V ((IRQn_Type)(163)) /* */ 00258 #define BSP_INT_SRC_CMI1 ((IRQn_Type)(164)) /* Motor control PWM timer */ 00259 #define BSP_INT_SRC_CMI2 ((IRQn_Type)(165)) /* */ 00260 #define BSP_INT_SRC_SGDEI0 ((IRQn_Type)(166)) /* Sound generator */ 00261 #define BSP_INT_SRC_SGDEI1 ((IRQn_Type)(167)) /* */ 00262 #define BSP_INT_SRC_SGDEI2 ((IRQn_Type)(168)) /* */ 00263 #define BSP_INT_SRC_SGDEI3 ((IRQn_Type)(169)) /* */ 00264 #define BSP_INT_SRC_ADI ((IRQn_Type)(170)) /* 12bit A/D converter */ 00265 #define BSP_INT_SRC_LMTI ((IRQn_Type)(171)) /* */ 00266 #define BSP_INT_SRC_SSII0 ((IRQn_Type)(172)) /* Serial sound interface */ 00267 #define BSP_INT_SRC_SSIRXI0 ((IRQn_Type)(173)) /* */ 00268 #define BSP_INT_SRC_SSITXI0 ((IRQn_Type)(174)) /* */ 00269 #define BSP_INT_SRC_SSII1 ((IRQn_Type)(175)) /* */ 00270 #define BSP_INT_SRC_SSIRXI1 ((IRQn_Type)(176)) /* */ 00271 #define BSP_INT_SRC_SSITXI1 ((IRQn_Type)(177)) /* */ 00272 #define BSP_INT_SRC_SSII2 ((IRQn_Type)(178)) /* */ 00273 #define BSP_INT_SRC_SSIRTI2 ((IRQn_Type)(179)) /* */ 00274 #define BSP_INT_SRC_SSII3 ((IRQn_Type)(180)) /* */ 00275 #define BSP_INT_SRC_SSIRXI3 ((IRQn_Type)(181)) /* */ 00276 #define BSP_INT_SRC_SSITXI3 ((IRQn_Type)(182)) /* */ 00277 #define BSP_INT_SRC_SSII4 ((IRQn_Type)(183)) /* */ 00278 #define BSP_INT_SRC_SSIRTI4 ((IRQn_Type)(184)) /* */ 00279 #define BSP_INT_SRC_SSII5 ((IRQn_Type)(185)) /* */ 00280 #define BSP_INT_SRC_SSIRXI5 ((IRQn_Type)(186)) /* */ 00281 #define BSP_INT_SRC_SSITXI5 ((IRQn_Type)(187)) /* */ 00282 #define BSP_INT_SRC_SPDIFI ((IRQn_Type)(188)) /* Renesas SPDIF interface */ 00283 #define BSP_INT_SRC_INTIICTEI0 ((IRQn_Type)(189)) /* I2C interface */ 00284 #define BSP_INT_SRC_INTIICRI0 ((IRQn_Type)(190)) /* */ 00285 #define BSP_INT_SRC_INTIICTI0 ((IRQn_Type)(191)) /* */ 00286 #define BSP_INT_SRC_INTIICSPI0 ((IRQn_Type)(192)) /* */ 00287 #define BSP_INT_SRC_INTIICSTI0 ((IRQn_Type)(193)) /* */ 00288 #define BSP_INT_SRC_INTIICNAKI0 ((IRQn_Type)(194)) /* */ 00289 #define BSP_INT_SRC_INTIICALI0 ((IRQn_Type)(195)) /* */ 00290 #define BSP_INT_SRC_INTIICTMOI0 ((IRQn_Type)(196)) /* */ 00291 #define BSP_INT_SRC_INTIICTEI1 ((IRQn_Type)(197)) /* */ 00292 #define BSP_INT_SRC_INTIICRI1 ((IRQn_Type)(198)) /* */ 00293 #define BSP_INT_SRC_INTIICTI1 ((IRQn_Type)(199)) /* */ 00294 #define BSP_INT_SRC_INTIICSPI1 ((IRQn_Type)(200)) /* */ 00295 #define BSP_INT_SRC_INTIICSTI1 ((IRQn_Type)(201)) /* */ 00296 #define BSP_INT_SRC_INTIICNAKI1 ((IRQn_Type)(202)) /* */ 00297 #define BSP_INT_SRC_INTIICALI1 ((IRQn_Type)(203)) /* */ 00298 #define BSP_INT_SRC_INTIICTMOI1 ((IRQn_Type)(204)) /* */ 00299 #define BSP_INT_SRC_INTIICTEI2 ((IRQn_Type)(205)) /* */ 00300 #define BSP_INT_SRC_INTIICRI2 ((IRQn_Type)(206)) /* */ 00301 #define BSP_INT_SRC_INTIICTI2 ((IRQn_Type)(207)) /* */ 00302 #define BSP_INT_SRC_INTIICSPI2 ((IRQn_Type)(208)) /* */ 00303 #define BSP_INT_SRC_INTIICSTI2 ((IRQn_Type)(209)) /* */ 00304 #define BSP_INT_SRC_INTIICNAKI2 ((IRQn_Type)(210)) /* */ 00305 #define BSP_INT_SRC_INTIICALI2 ((IRQn_Type)(211)) /* */ 00306 #define BSP_INT_SRC_INTIICTMOI2 ((IRQn_Type)(212)) /* */ 00307 #define BSP_INT_SRC_INTIICTEI3 ((IRQn_Type)(213)) /* */ 00308 #define BSP_INT_SRC_INTIICRI3 ((IRQn_Type)(214)) /* */ 00309 #define BSP_INT_SRC_INTIICTI3 ((IRQn_Type)(215)) /* */ 00310 #define BSP_INT_SRC_INTIICSPI3 ((IRQn_Type)(216)) /* */ 00311 #define BSP_INT_SRC_INTIICSTI3 ((IRQn_Type)(217)) /* */ 00312 #define BSP_INT_SRC_INTIICNAKI3 ((IRQn_Type)(218)) /* */ 00313 #define BSP_INT_SRC_INTIICALI3 ((IRQn_Type)(219)) /* */ 00314 #define BSP_INT_SRC_INTIICTMOI3 ((IRQn_Type)(220)) /* */ 00315 #define BSP_INT_SRC_BRI0 ((IRQn_Type)(221)) /* Serial Communication Interface with FIFO */ 00316 #define BSP_INT_SRC_ERI0 ((IRQn_Type)(222)) /* */ 00317 #define BSP_INT_SRC_RXI0 ((IRQn_Type)(223)) /* */ 00318 #define BSP_INT_SRC_TXI0 ((IRQn_Type)(224)) /* */ 00319 #define BSP_INT_SRC_BRI1 ((IRQn_Type)(225)) /* */ 00320 #define BSP_INT_SRC_ERI1 ((IRQn_Type)(226)) /* */ 00321 #define BSP_INT_SRC_RXI1 ((IRQn_Type)(227)) /* */ 00322 #define BSP_INT_SRC_TXI1 ((IRQn_Type)(228)) /* */ 00323 #define BSP_INT_SRC_BRI2 ((IRQn_Type)(229)) /* */ 00324 #define BSP_INT_SRC_ERI2 ((IRQn_Type)(230)) /* */ 00325 #define BSP_INT_SRC_RXI2 ((IRQn_Type)(231)) /* */ 00326 #define BSP_INT_SRC_TXI2 ((IRQn_Type)(232)) /* */ 00327 #define BSP_INT_SRC_BRI3 ((IRQn_Type)(233)) /* */ 00328 #define BSP_INT_SRC_ERI3 ((IRQn_Type)(234)) /* */ 00329 #define BSP_INT_SRC_RXI3 ((IRQn_Type)(235)) /* */ 00330 #define BSP_INT_SRC_TXI3 ((IRQn_Type)(236)) /* */ 00331 #define BSP_INT_SRC_BRI4 ((IRQn_Type)(237)) /* */ 00332 #define BSP_INT_SRC_ERI4 ((IRQn_Type)(238)) /* */ 00333 #define BSP_INT_SRC_RXI4 ((IRQn_Type)(239)) /* */ 00334 #define BSP_INT_SRC_TXI4 ((IRQn_Type)(240)) /* */ 00335 #define BSP_INT_SRC_BRI5 ((IRQn_Type)(241)) /* */ 00336 #define BSP_INT_SRC_ERI5 ((IRQn_Type)(242)) /* */ 00337 #define BSP_INT_SRC_RXI5 ((IRQn_Type)(243)) /* */ 00338 #define BSP_INT_SRC_TXI5 ((IRQn_Type)(244)) /* */ 00339 #define BSP_INT_SRC_BRI6 ((IRQn_Type)(245)) /* */ 00340 #define BSP_INT_SRC_ERI6 ((IRQn_Type)(246)) /* */ 00341 #define BSP_INT_SRC_RXI6 ((IRQn_Type)(247)) /* */ 00342 #define BSP_INT_SRC_TXI6 ((IRQn_Type)(248)) /* */ 00343 #define BSP_INT_SRC_BRI7 ((IRQn_Type)(249)) /* */ 00344 #define BSP_INT_SRC_ERI7 ((IRQn_Type)(250)) /* */ 00345 #define BSP_INT_SRC_RXI7 ((IRQn_Type)(251)) /* */ 00346 #define BSP_INT_SRC_TXI7 ((IRQn_Type)(252)) /* */ 00347 #define BSP_INT_SRC_INTRCANGERR ((IRQn_Type)(253)) /* CAN interface */ 00348 #define BSP_INT_SRC_INTRCANGRECC ((IRQn_Type)(254)) /* */ 00349 #define BSP_INT_SRC_INTRCAN0REC ((IRQn_Type)(255)) /* */ 00350 #define BSP_INT_SRC_INTRCAN0ERR ((IRQn_Type)(256)) /* */ 00351 #define BSP_INT_SRC_INTRCAN0TRX ((IRQn_Type)(257)) /* */ 00352 #define BSP_INT_SRC_INTRCAN1REC ((IRQn_Type)(258)) /* */ 00353 #define BSP_INT_SRC_INTRCAN1ERR ((IRQn_Type)(259)) /* */ 00354 #define BSP_INT_SRC_INTRCAN1TRX ((IRQn_Type)(260)) /* */ 00355 #define BSP_INT_SRC_INTRCAN2REC ((IRQn_Type)(261)) /* */ 00356 #define BSP_INT_SRC_INTRCAN2ERR ((IRQn_Type)(262)) /* */ 00357 #define BSP_INT_SRC_INTRCAN2TRX ((IRQn_Type)(263)) /* */ 00358 #define BSP_INT_SRC_INTRCAN3REC ((IRQn_Type)(264)) /* */ 00359 #define BSP_INT_SRC_INTRCAN3ERR ((IRQn_Type)(265)) /* */ 00360 #define BSP_INT_SRC_INTRCAN3TRX ((IRQn_Type)(266)) /* */ 00361 #define BSP_INT_SRC_INTRCAN4REC ((IRQn_Type)(267)) /* */ 00362 #define BSP_INT_SRC_INTRCAN4ERR ((IRQn_Type)(268)) /* */ 00363 #define BSP_INT_SRC_INTRCAN4TRX ((IRQn_Type)(269)) /* */ 00364 #define BSP_INT_SRC_SPEI0 ((IRQn_Type)(270)) /* Renesas serial peripheral interface */ 00365 #define BSP_INT_SRC_SPRI0 ((IRQn_Type)(271)) /* */ 00366 #define BSP_INT_SRC_SPTI0 ((IRQn_Type)(272)) /* */ 00367 #define BSP_INT_SRC_SPEI1 ((IRQn_Type)(273)) /* */ 00368 #define BSP_INT_SRC_SPRI1 ((IRQn_Type)(274)) /* */ 00369 #define BSP_INT_SRC_SPTI1 ((IRQn_Type)(275)) /* */ 00370 #define BSP_INT_SRC_SPEI2 ((IRQn_Type)(276)) /* */ 00371 #define BSP_INT_SRC_SPRI2 ((IRQn_Type)(277)) /* */ 00372 #define BSP_INT_SRC_SPTI2 ((IRQn_Type)(278)) /* */ 00373 #define BSP_INT_SRC_SPEI3 ((IRQn_Type)(279)) /* */ 00374 #define BSP_INT_SRC_SPRI3 ((IRQn_Type)(280)) /* */ 00375 #define BSP_INT_SRC_SPTI3 ((IRQn_Type)(281)) /* */ 00376 #define BSP_INT_SRC_SPEI4 ((IRQn_Type)(282)) /* */ 00377 #define BSP_INT_SRC_SPRI4 ((IRQn_Type)(283)) /* */ 00378 #define BSP_INT_SRC_SPTI4 ((IRQn_Type)(284)) /* */ 00379 #define BSP_INT_SRC_IEBBTD ((IRQn_Type)(285)) /* IEBusTM controller */ 00380 #define BSP_INT_SRC_IEBBTERR ((IRQn_Type)(286)) /* */ 00381 #define BSP_INT_SRC_IEBBTSTA ((IRQn_Type)(287)) /* */ 00382 #define BSP_INT_SRC_IEBBTV ((IRQn_Type)(288)) /* */ 00383 #define BSP_INT_SRC_ISY ((IRQn_Type)(289)) /* CD-ROM decoder */ 00384 #define BSP_INT_SRC_IERR ((IRQn_Type)(290)) /* */ 00385 #define BSP_INT_SRC_ITARG ((IRQn_Type)(291)) /* */ 00386 #define BSP_INT_SRC_ISEC ((IRQn_Type)(292)) /* */ 00387 #define BSP_INT_SRC_IBUF ((IRQn_Type)(293)) /* */ 00388 #define BSP_INT_SRC_IREADY ((IRQn_Type)(294)) /* */ 00389 #define BSP_INT_SRC_FLSTE ((IRQn_Type)(295)) /* NAND Flash memory controller */ 00390 #define BSP_INT_SRC_FLTENDI ((IRQn_Type)(296)) /* */ 00391 #define BSP_INT_SRC_FLTREQ0I ((IRQn_Type)(297)) /* */ 00392 #define BSP_INT_SRC_FLTREQ1I ((IRQn_Type)(298)) /* */ 00393 #define BSP_INT_SRC_MMC0 ((IRQn_Type)(299)) /* MMC Host interface */ 00394 #define BSP_INT_SRC_MMC1 ((IRQn_Type)(300)) /* */ 00395 #define BSP_INT_SRC_MMC2 ((IRQn_Type)(301)) /* */ 00396 #define BSP_INT_SRC_SDHI0_3 ((IRQn_Type)(302)) /* SD Host interface */ 00397 #define BSP_INT_SRC_SDHI0_0 ((IRQn_Type)(303)) /* */ 00398 #define BSP_INT_SRC_SDHI0_1 ((IRQn_Type)(304)) /* */ 00399 #define BSP_INT_SRC_SDHI1_3 ((IRQn_Type)(305)) /* */ 00400 #define BSP_INT_SRC_SDHI1_0 ((IRQn_Type)(306)) /* */ 00401 #define BSP_INT_SRC_SDHI1_1 ((IRQn_Type)(307)) /* */ 00402 #define BSP_INT_SRC_ARM ((IRQn_Type)(308)) /* Real time clock */ 00403 #define BSP_INT_SRC_PRD ((IRQn_Type)(309)) /* */ 00404 #define BSP_INT_SRC_CUP ((IRQn_Type)(310)) /* */ 00405 #define BSP_INT_SRC_SCUAI0 ((IRQn_Type)(311)) /* SCUX */ 00406 #define BSP_INT_SRC_SCUAI1 ((IRQn_Type)(312)) /* */ 00407 #define BSP_INT_SRC_SCUFDI0 ((IRQn_Type)(313)) /* */ 00408 #define BSP_INT_SRC_SCUFDI1 ((IRQn_Type)(314)) /* */ 00409 #define BSP_INT_SRC_SCUFDI2 ((IRQn_Type)(315)) /* */ 00410 #define BSP_INT_SRC_SCUFDI3 ((IRQn_Type)(316)) /* */ 00411 #define BSP_INT_SRC_SCUFUI0 ((IRQn_Type)(317)) /* */ 00412 #define BSP_INT_SRC_SCUFUI1 ((IRQn_Type)(318)) /* */ 00413 #define BSP_INT_SRC_SCUFUI2 ((IRQn_Type)(319)) /* */ 00414 #define BSP_INT_SRC_SCUFUI3 ((IRQn_Type)(320)) /* */ 00415 #define BSP_INT_SRC_SCUDVI0 ((IRQn_Type)(321)) /* */ 00416 #define BSP_INT_SRC_SCUDVI1 ((IRQn_Type)(322)) /* */ 00417 #define BSP_INT_SRC_SCUDVI2 ((IRQn_Type)(323)) /* */ 00418 #define BSP_INT_SRC_SCUDVI3 ((IRQn_Type)(324)) /* */ 00419 #define BSP_INT_SRC_MLB_CINT ((IRQn_Type)(325)) /* Media local bus */ 00420 #define BSP_INT_SRC_MLB_SINT ((IRQn_Type)(326)) /* */ 00421 #define BSP_INT_SRC_DRC0 ((IRQn_Type)(327)) /* Dynamic range compalator */ 00422 #define BSP_INT_SRC_DRC1 ((IRQn_Type)(328)) /* */ 00423 #define BSP_INT_SRC_LINI0_INT_T ((IRQn_Type)(331)) /* LIN/UART interface */ 00424 #define BSP_INT_SRC_LINI0_INT_R ((IRQn_Type)(332)) /* */ 00425 #define BSP_INT_SRC_LINI0_INT_S ((IRQn_Type)(333)) /* */ 00426 #define BSP_INT_SRC_LINI0_INT_M ((IRQn_Type)(334)) /* */ 00427 #define BSP_INT_SRC_LINI1_INT_T ((IRQn_Type)(335)) /* */ 00428 #define BSP_INT_SRC_LINI1_INT_R ((IRQn_Type)(336)) /* */ 00429 #define BSP_INT_SRC_LINI1_INT_S ((IRQn_Type)(337)) /* */ 00430 #define BSP_INT_SRC_LINI1_INT_M ((IRQn_Type)(338)) /* */ 00431 #define BSP_INT_SRC_SCI_ERI0 ((IRQn_Type)(347)) /* Serial communication interface */ 00432 #define BSP_INT_SRC_SCI_RXI0 ((IRQn_Type)(348)) /* */ 00433 #define BSP_INT_SRC_SCI_TXI0 ((IRQn_Type)(349)) /* */ 00434 #define BSP_INT_SRC_SCI_TEI0 ((IRQn_Type)(350)) /* */ 00435 #define BSP_INT_SRC_SCI_ERI1 ((IRQn_Type)(351)) /* */ 00436 #define BSP_INT_SRC_SCI_RXI1 ((IRQn_Type)(352)) /* */ 00437 #define BSP_INT_SRC_SCI_TXI1 ((IRQn_Type)(353)) /* */ 00438 #define BSP_INT_SRC_SCI_TEI1 ((IRQn_Type)(354)) /* */ 00439 #define BSP_INT_SRC_AVBI_DATA ((IRQn_Type)(355)) /* EthernetAVB */ 00440 #define BSP_INT_SRC_AVBI_ERROR ((IRQn_Type)(356)) /* */ 00441 #define BSP_INT_SRC_AVBI_MANAGE ((IRQn_Type)(357)) /* */ 00442 #define BSP_INT_SRC_AVBI_MAC ((IRQn_Type)(358)) /* */ 00443 #define BSP_INT_SRC_ETHERI ((IRQn_Type)(359)) /* Ethernet controller */ 00444 #define BSP_INT_SRC_CEUI ((IRQn_Type)(364)) /* Capture engine unit */ 00445 #define BSP_INT_SRC_H2XMLB_ERRINT ((IRQn_Type)(381)) /* Internal bus */ 00446 #define BSP_INT_SRC_H2XIC1_ERRINT ((IRQn_Type)(382)) /* */ 00447 #define BSP_INT_SRC_X2HPERI1_ERRINT ((IRQn_Type)(383)) /* */ 00448 #define BSP_INT_SRC_X2HPERI2_ERRINT ((IRQn_Type)(384)) /* */ 00449 #define BSP_INT_SRC_X2HPERI34_ERRINT ((IRQn_Type)(385)) /* */ 00450 #define BSP_INT_SRC_X2HPERI5_ERRINT ((IRQn_Type)(386)) /* */ 00451 #define BSP_INT_SRC_X2HPERI67_ERRINT ((IRQn_Type)(387)) /* */ 00452 #define BSP_INT_SRC_X2HDBGR_ERRINT ((IRQn_Type)(388)) /* */ 00453 #define BSP_INT_SRC_X2HBSC_ERRINT ((IRQn_Type)(389)) /* */ 00454 #define BSP_INT_SRC_X2HSPI1_ERRINT ((IRQn_Type)(390)) /* */ 00455 #define BSP_INT_SRC_X2HSPI2_ERRINT ((IRQn_Type)(391)) /* */ 00456 #define BSP_INT_SRC_PRRI ((IRQn_Type)(392)) /* */ 00457 #define BSP_INT_SRC_IFEI0 ((IRQn_Type)(393)) /* Pixel format converter */ 00458 #define BSP_INT_SRC_OFFI0 ((IRQn_Type)(394)) /* */ 00459 #define BSP_INT_SRC_PFVEI0 ((IRQn_Type)(395)) /* */ 00460 #define BSP_INT_SRC_IFEI1 ((IRQn_Type)(396)) /* */ 00461 #define BSP_INT_SRC_OFFI1 ((IRQn_Type)(397)) /* */ 00462 #define BSP_INT_SRC_PFVEI1 ((IRQn_Type)(398)) /* */ 00463 #define BSP_INT_SRC_TINT0 ((IRQn_Type)(416)) /* Terminal interrupts */ 00464 #define BSP_INT_SRC_TINT1 ((IRQn_Type)(417)) /* */ 00465 #define BSP_INT_SRC_TINT2 ((IRQn_Type)(418)) /* */ 00466 #define BSP_INT_SRC_TINT3 ((IRQn_Type)(419)) /* */ 00467 #define BSP_INT_SRC_TINT4 ((IRQn_Type)(420)) /* */ 00468 #define BSP_INT_SRC_TINT5 ((IRQn_Type)(421)) /* */ 00469 #define BSP_INT_SRC_TINT6 ((IRQn_Type)(422)) /* */ 00470 #define BSP_INT_SRC_TINT7 ((IRQn_Type)(423)) /* */ 00471 #define BSP_INT_SRC_TINT8 ((IRQn_Type)(424)) /* */ 00472 #define BSP_INT_SRC_TINT9 ((IRQn_Type)(425)) /* */ 00473 #define BSP_INT_SRC_TINT10 ((IRQn_Type)(426)) /* */ 00474 #define BSP_INT_SRC_TINT11 ((IRQn_Type)(427)) /* */ 00475 #define BSP_INT_SRC_TINT12 ((IRQn_Type)(428)) /* */ 00476 #define BSP_INT_SRC_TINT13 ((IRQn_Type)(429)) /* */ 00477 #define BSP_INT_SRC_TINT14 ((IRQn_Type)(430)) /* */ 00478 #define BSP_INT_SRC_TINT15 ((IRQn_Type)(431)) /* */ 00479 #define BSP_INT_SRC_TINT16 ((IRQn_Type)(432)) /* */ 00480 #define BSP_INT_SRC_TINT17 ((IRQn_Type)(433)) /* */ 00481 #define BSP_INT_SRC_TINT18 ((IRQn_Type)(434)) /* */ 00482 #define BSP_INT_SRC_TINT19 ((IRQn_Type)(435)) /* */ 00483 #define BSP_INT_SRC_TINT20 ((IRQn_Type)(436)) /* */ 00484 #define BSP_INT_SRC_TINT21 ((IRQn_Type)(437)) /* */ 00485 #define BSP_INT_SRC_TINT22 ((IRQn_Type)(438)) /* */ 00486 #define BSP_INT_SRC_TINT23 ((IRQn_Type)(439)) /* */ 00487 #define BSP_INT_SRC_TINT24 ((IRQn_Type)(440)) /* */ 00488 #define BSP_INT_SRC_TINT25 ((IRQn_Type)(441)) /* */ 00489 #define BSP_INT_SRC_TINT26 ((IRQn_Type)(442)) /* */ 00490 #define BSP_INT_SRC_TINT27 ((IRQn_Type)(443)) /* */ 00491 #define BSP_INT_SRC_TINT28 ((IRQn_Type)(444)) /* */ 00492 #define BSP_INT_SRC_TINT29 ((IRQn_Type)(445)) /* */ 00493 #define BSP_INT_SRC_TINT30 ((IRQn_Type)(446)) /* */ 00494 #define BSP_INT_SRC_TINT31 ((IRQn_Type)(447)) /* */ 00495 #define BSP_INT_SRC_TINT32 ((IRQn_Type)(448)) /* */ 00496 #define BSP_INT_SRC_TINT33 ((IRQn_Type)(449)) /* */ 00497 #define BSP_INT_SRC_TINT34 ((IRQn_Type)(450)) /* */ 00498 #define BSP_INT_SRC_TINT35 ((IRQn_Type)(451)) /* */ 00499 #define BSP_INT_SRC_TINT36 ((IRQn_Type)(452)) /* */ 00500 #define BSP_INT_SRC_TINT37 ((IRQn_Type)(453)) /* */ 00501 #define BSP_INT_SRC_TINT38 ((IRQn_Type)(454)) /* */ 00502 #define BSP_INT_SRC_TINT39 ((IRQn_Type)(455)) /* */ 00503 #define BSP_INT_SRC_TINT40 ((IRQn_Type)(456)) /* */ 00504 #define BSP_INT_SRC_TINT41 ((IRQn_Type)(457)) /* */ 00505 #define BSP_INT_SRC_TINT42 ((IRQn_Type)(458)) /* */ 00506 #define BSP_INT_SRC_TINT43 ((IRQn_Type)(459)) /* */ 00507 #define BSP_INT_SRC_TINT44 ((IRQn_Type)(460)) /* */ 00508 #define BSP_INT_SRC_TINT45 ((IRQn_Type)(461)) /* */ 00509 #define BSP_INT_SRC_TINT46 ((IRQn_Type)(462)) /* */ 00510 #define BSP_INT_SRC_TINT47 ((IRQn_Type)(463)) /* */ 00511 #define BSP_INT_SRC_TINT48 ((IRQn_Type)(464)) /* */ 00512 #define BSP_INT_SRC_TINT49 ((IRQn_Type)(465)) /* */ 00513 #define BSP_INT_SRC_TINT50 ((IRQn_Type)(466)) /* */ 00514 #define BSP_INT_SRC_TINT51 ((IRQn_Type)(467)) /* */ 00515 #define BSP_INT_SRC_TINT52 ((IRQn_Type)(468)) /* */ 00516 #define BSP_INT_SRC_TINT53 ((IRQn_Type)(469)) /* */ 00517 #define BSP_INT_SRC_TINT54 ((IRQn_Type)(470)) /* */ 00518 #define BSP_INT_SRC_TINT55 ((IRQn_Type)(471)) /* */ 00519 #define BSP_INT_SRC_TINT56 ((IRQn_Type)(472)) /* */ 00520 #define BSP_INT_SRC_TINT57 ((IRQn_Type)(473)) /* */ 00521 #define BSP_INT_SRC_TINT58 ((IRQn_Type)(474)) /* */ 00522 #define BSP_INT_SRC_TINT59 ((IRQn_Type)(475)) /* */ 00523 #define BSP_INT_SRC_TINT60 ((IRQn_Type)(476)) /* */ 00524 #define BSP_INT_SRC_TINT61 ((IRQn_Type)(477)) /* */ 00525 #define BSP_INT_SRC_TINT62 ((IRQn_Type)(478)) /* */ 00526 #define BSP_INT_SRC_TINT63 ((IRQn_Type)(479)) /* */ 00527 #define BSP_INT_SRC_TINT64 ((IRQn_Type)(480)) /* */ 00528 #define BSP_INT_SRC_TINT65 ((IRQn_Type)(481)) /* */ 00529 #define BSP_INT_SRC_TINT66 ((IRQn_Type)(482)) /* */ 00530 #define BSP_INT_SRC_TINT67 ((IRQn_Type)(483)) /* */ 00531 #define BSP_INT_SRC_TINT68 ((IRQn_Type)(484)) /* */ 00532 #define BSP_INT_SRC_TINT69 ((IRQn_Type)(485)) /* */ 00533 #define BSP_INT_SRC_TINT70 ((IRQn_Type)(486)) /* */ 00534 #define BSP_INT_SRC_TINT71 ((IRQn_Type)(487)) /* */ 00535 #define BSP_INT_SRC_TINT72 ((IRQn_Type)(488)) /* */ 00536 #define BSP_INT_SRC_TINT73 ((IRQn_Type)(489)) /* */ 00537 #define BSP_INT_SRC_TINT74 ((IRQn_Type)(490)) /* */ 00538 #define BSP_INT_SRC_TINT75 ((IRQn_Type)(491)) /* */ 00539 #define BSP_INT_SRC_TINT76 ((IRQn_Type)(492)) /* */ 00540 #define BSP_INT_SRC_TINT77 ((IRQn_Type)(493)) /* */ 00541 #define BSP_INT_SRC_TINT78 ((IRQn_Type)(494)) /* */ 00542 #define BSP_INT_SRC_TINT79 ((IRQn_Type)(495)) /* */ 00543 #define BSP_INT_SRC_TINT80 ((IRQn_Type)(496)) /* */ 00544 #define BSP_INT_SRC_TINT81 ((IRQn_Type)(497)) /* */ 00545 #define BSP_INT_SRC_TINT82 ((IRQn_Type)(498)) /* */ 00546 #define BSP_INT_SRC_TINT83 ((IRQn_Type)(499)) /* */ 00547 #define BSP_INT_SRC_TINT84 ((IRQn_Type)(500)) /* */ 00548 #define BSP_INT_SRC_TINT85 ((IRQn_Type)(501)) /* */ 00549 #define BSP_INT_SRC_TINT86 ((IRQn_Type)(502)) /* */ 00550 #define BSP_INT_SRC_TINT87 ((IRQn_Type)(503)) /* */ 00551 #define BSP_INT_SRC_TINT88 ((IRQn_Type)(504)) /* */ 00552 #define BSP_INT_SRC_TINT89 ((IRQn_Type)(505)) /* */ 00553 #define BSP_INT_SRC_TINT90 ((IRQn_Type)(506)) /* */ 00554 #define BSP_INT_SRC_TINT91 ((IRQn_Type)(507)) /* */ 00555 #define BSP_INT_SRC_TINT92 ((IRQn_Type)(508)) /* */ 00556 #define BSP_INT_SRC_TINT93 ((IRQn_Type)(509)) /* */ 00557 #define BSP_INT_SRC_TINT94 ((IRQn_Type)(510)) /* */ 00558 #define BSP_INT_SRC_TINT95 ((IRQn_Type)(511)) /* */ 00559 #define BSP_INT_SRC_TINT96 ((IRQn_Type)(512)) /* */ 00560 #define BSP_INT_SRC_TINT97 ((IRQn_Type)(513)) /* */ 00561 #define BSP_INT_SRC_TINT98 ((IRQn_Type)(514)) /* */ 00562 #define BSP_INT_SRC_TINT99 ((IRQn_Type)(515)) /* */ 00563 #define BSP_INT_SRC_TINT100 ((IRQn_Type)(516)) /* */ 00564 #define BSP_INT_SRC_TINT101 ((IRQn_Type)(517)) /* */ 00565 #define BSP_INT_SRC_TINT102 ((IRQn_Type)(518)) /* */ 00566 #define BSP_INT_SRC_TINT103 ((IRQn_Type)(519)) /* */ 00567 #define BSP_INT_SRC_TINT104 ((IRQn_Type)(520)) /* */ 00568 #define BSP_INT_SRC_TINT105 ((IRQn_Type)(521)) /* */ 00569 #define BSP_INT_SRC_TINT106 ((IRQn_Type)(522)) /* */ 00570 #define BSP_INT_SRC_TINT107 ((IRQn_Type)(523)) /* */ 00571 #define BSP_INT_SRC_TINT108 ((IRQn_Type)(524)) /* */ 00572 #define BSP_INT_SRC_TINT109 ((IRQn_Type)(525)) /* */ 00573 #define BSP_INT_SRC_TINT110 ((IRQn_Type)(526)) /* */ 00574 #define BSP_INT_SRC_TINT111 ((IRQn_Type)(527)) /* */ 00575 #define BSP_INT_SRC_TINT112 ((IRQn_Type)(528)) /* */ 00576 #define BSP_INT_SRC_TINT113 ((IRQn_Type)(529)) /* */ 00577 #define BSP_INT_SRC_TINT114 ((IRQn_Type)(530)) /* */ 00578 #define BSP_INT_SRC_TINT115 ((IRQn_Type)(531)) /* */ 00579 #define BSP_INT_SRC_TINT116 ((IRQn_Type)(532)) /* */ 00580 #define BSP_INT_SRC_TINT117 ((IRQn_Type)(533)) /* */ 00581 #define BSP_INT_SRC_TINT118 ((IRQn_Type)(534)) /* */ 00582 #define BSP_INT_SRC_TINT119 ((IRQn_Type)(535)) /* */ 00583 #define BSP_INT_SRC_TINT120 ((IRQn_Type)(536)) /* */ 00584 #define BSP_INT_SRC_TINT121 ((IRQn_Type)(537)) /* */ 00585 #define BSP_INT_SRC_TINT122 ((IRQn_Type)(538)) /* */ 00586 #define BSP_INT_SRC_TINT123 ((IRQn_Type)(539)) /* */ 00587 #define BSP_INT_SRC_TINT124 ((IRQn_Type)(540)) /* */ 00588 #define BSP_INT_SRC_TINT125 ((IRQn_Type)(541)) /* */ 00589 #define BSP_INT_SRC_TINT126 ((IRQn_Type)(542)) /* */ 00590 #define BSP_INT_SRC_TINT127 ((IRQn_Type)(543)) /* */ 00591 #define BSP_INT_SRC_TINT128 ((IRQn_Type)(544)) /* */ 00592 #define BSP_INT_SRC_TINT129 ((IRQn_Type)(545)) /* */ 00593 #define BSP_INT_SRC_TINT130 ((IRQn_Type)(546)) /* */ 00594 #define BSP_INT_SRC_TINT131 ((IRQn_Type)(547)) /* */ 00595 #define BSP_INT_SRC_TINT132 ((IRQn_Type)(548)) /* */ 00596 #define BSP_INT_SRC_TINT133 ((IRQn_Type)(549)) /* */ 00597 #define BSP_INT_SRC_TINT134 ((IRQn_Type)(550)) /* */ 00598 #define BSP_INT_SRC_TINT135 ((IRQn_Type)(551)) /* */ 00599 #define BSP_INT_SRC_TINT136 ((IRQn_Type)(552)) /* */ 00600 #define BSP_INT_SRC_TINT137 ((IRQn_Type)(553)) /* */ 00601 #define BSP_INT_SRC_TINT138 ((IRQn_Type)(554)) /* */ 00602 #define BSP_INT_SRC_TINT139 ((IRQn_Type)(555)) /* */ 00603 #define BSP_INT_SRC_TINT140 ((IRQn_Type)(556)) /* */ 00604 #define BSP_INT_SRC_TINT141 ((IRQn_Type)(557)) /* */ 00605 #define BSP_INT_SRC_TINT142 ((IRQn_Type)(558)) /* */ 00606 #define BSP_INT_SRC_TINT143 ((IRQn_Type)(559)) /* */ 00607 #define BSP_INT_SRC_TINT144 ((IRQn_Type)(560)) /* */ 00608 #define BSP_INT_SRC_TINT145 ((IRQn_Type)(561)) /* */ 00609 #define BSP_INT_SRC_TINT146 ((IRQn_Type)(562)) /* */ 00610 #define BSP_INT_SRC_TINT147 ((IRQn_Type)(563)) /* */ 00611 #define BSP_INT_SRC_TINT148 ((IRQn_Type)(564)) /* */ 00612 #define BSP_INT_SRC_TINT149 ((IRQn_Type)(565)) /* */ 00613 #define BSP_INT_SRC_TINT150 ((IRQn_Type)(566)) /* */ 00614 #define BSP_INT_SRC_TINT151 ((IRQn_Type)(567)) /* */ 00615 #define BSP_INT_SRC_TINT152 ((IRQn_Type)(568)) /* */ 00616 #define BSP_INT_SRC_TINT153 ((IRQn_Type)(569)) /* */ 00617 #define BSP_INT_SRC_TINT154 ((IRQn_Type)(570)) /* */ 00618 #define BSP_INT_SRC_TINT155 ((IRQn_Type)(571)) /* */ 00619 #define BSP_INT_SRC_TINT156 ((IRQn_Type)(572)) /* */ 00620 #define BSP_INT_SRC_TINT157 ((IRQn_Type)(573)) /* */ 00621 #define BSP_INT_SRC_TINT158 ((IRQn_Type)(574)) /* */ 00622 #define BSP_INT_SRC_TINT159 ((IRQn_Type)(575)) /* */ 00623 #define BSP_INT_SRC_TINT160 ((IRQn_Type)(576)) /* */ 00624 #define BSP_INT_SRC_TINT161 ((IRQn_Type)(577)) /* */ 00625 #define BSP_INT_SRC_TINT162 ((IRQn_Type)(578)) /* */ 00626 #define BSP_INT_SRC_TINT163 ((IRQn_Type)(579)) /* */ 00627 #define BSP_INT_SRC_TINT164 ((IRQn_Type)(580)) /* */ 00628 #define BSP_INT_SRC_TINT165 ((IRQn_Type)(581)) /* */ 00629 #define BSP_INT_SRC_TINT166 ((IRQn_Type)(582)) /* */ 00630 #define BSP_INT_SRC_TINT167 ((IRQn_Type)(583)) /* */ 00631 #define BSP_INT_SRC_TINT168 ((IRQn_Type)(584)) /* */ 00632 #define BSP_INT_SRC_TINT169 ((IRQn_Type)(585)) /* */ 00633 #define BSP_INT_SRC_TINT170 ((IRQn_Type)(586)) /* */ 00634 00635 #elif defined(TARGET_RZ_A2XX) 00636 00637 #define BSP_INT_SRC_JEDI ((IRQn_Type)(84)) /* JPEG Codec unit */ 00638 #define BSP_INT_SRC_JDTI ((IRQn_Type)(85)) /* */ 00639 00640 #endif 00641 00642 INLINE IRQn_Type R_CAST_bsp_int_src_t_to_IRQn_Type( bsp_int_src_t const Value ) 00643 { 00644 return (IRQn_Type) Value; 00645 } 00646 00647 00648 /****************************************************************************** 00649 Variable Externs 00650 ******************************************************************************/ 00651 00652 /****************************************************************************** 00653 Functions Prototypes 00654 ******************************************************************************/ 00655 /* In "mcu_interrupts.h" */ 00656 00657 00658 /*********************************************************************** 00659 * End of File: 00660 ************************************************************************/ 00661 #ifdef __cplusplus 00662 } /* extern "C" */ 00663 #endif /* __cplusplus */ 00664 00665 #endif /* MCU_INTERRUPTS_TYPEDEF_H */ 00666
Generated on Wed Jul 13 2022 05:33:36 by
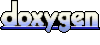