
test public
Dependencies: HttpServer_snapshot_mbed-os
dcache-control.c
00001 /* Dcache Control Library 00002 * Copyright (C) 2017 dkato 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "cmsis.h" 00018 #include "dcache-control.h" 00019 00020 void dcache_clean(void * p_buf, uint32_t size) { 00021 uint32_t start_addr = (uint32_t)p_buf & 0xFFFFFFE0; 00022 uint32_t end_addr = (uint32_t)p_buf + size; 00023 uint32_t addr; 00024 00025 /* Data cache clean */ 00026 for (addr = start_addr; addr < end_addr; addr += 0x20) { 00027 L1C_CleanDCacheMVA((void *)addr); 00028 } 00029 } 00030 00031 void dcache_invalid(void * p_buf, uint32_t size) { 00032 uint32_t start_addr = (uint32_t)p_buf & 0xFFFFFFE0; 00033 uint32_t end_addr = (uint32_t)p_buf + size; 00034 uint32_t addr; 00035 00036 /* Data cache invalid */ 00037 for (addr = start_addr; addr < end_addr; addr += 0x20) { 00038 L1C_InvalidateDCacheMVA((void *)addr); 00039 } 00040 } 00041
Generated on Wed Jul 13 2022 05:33:36 by
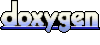