
test public
Dependencies: HttpServer_snapshot_mbed-os
WM8978_RBSP.cpp
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2018 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #include "mbed.h" 00025 #include "WM8978_RBSP.h" 00026 #include "pinmap.h" 00027 00028 00029 WM8978_RBSP::WM8978_RBSP(PinName mosi, PinName miso, PinName sclk, PinName ssel, 00030 PinName sck, PinName ws, PinName tx, PinName rx, PinName audio_clk, 00031 uint8_t int_level, int32_t max_write_num, int32_t max_read_num) 00032 : mSpi_(mosi, miso, sclk, ssel), mI2s_(sck, ws, tx, rx, audio_clk) { 00033 00034 // I2S Mode 00035 ssif_cfg.enabled = true; 00036 ssif_cfg.int_level = int_level; 00037 if ((int32_t)audio_clk == NC) { 00038 ssif_cfg.slave_mode = true; 00039 } else { 00040 ssif_cfg.slave_mode = false; 00041 } 00042 ssif_cfg.sample_freq = 44100u; 00043 ssif_cfg.clk_select = SSIF_CFG_CKS_AUDIO_CLK; 00044 ssif_cfg.multi_ch = SSIF_CFG_MULTI_CH_1; 00045 ssif_cfg.data_word = SSIF_CFG_DATA_WORD_16; 00046 ssif_cfg.system_word = SSIF_CFG_SYSTEM_WORD_32; 00047 ssif_cfg.bclk_pol = SSIF_CFG_FALLING; 00048 ssif_cfg.ws_pol = SSIF_CFG_WS_LOW; 00049 ssif_cfg.padding_pol = SSIF_CFG_PADDING_LOW; 00050 ssif_cfg.serial_alignment = SSIF_CFG_DATA_FIRST; 00051 ssif_cfg.parallel_alignment = SSIF_CFG_LEFT; 00052 ssif_cfg.ws_delay = SSIF_CFG_DELAY; 00053 ssif_cfg.noise_cancel = SSIF_CFG_ENABLE_NOISE_CANCEL; 00054 ssif_cfg.tdm_mode = SSIF_CFG_DISABLE_TDM; 00055 ssif_cfg.romdec_direct.mode = SSIF_CFG_DISABLE_ROMDEC_DIRECT; 00056 ssif_cfg.romdec_direct.p_cbfunc = NULL; 00057 mI2s_.init(&ssif_cfg, max_write_num, max_read_num); 00058 00059 mSpi_.format(16); 00060 00061 activateDigitalInterface_(); 00062 00063 power(false); // Power off 00064 } 00065 00066 // Public Functions 00067 bool WM8978_RBSP::outputVolume(float leftVolumeOut, float rightVolumeOut) { 00068 // check values are in range 00069 if ((leftVolumeOut < 0.0) || (leftVolumeOut > 1.0) 00070 || (rightVolumeOut < 0.0) || (rightVolumeOut > 1.0)) { 00071 return false; 00072 } 00073 return true; 00074 } 00075 00076 bool WM8978_RBSP::micVolume(float VolumeIn) { 00077 // check values are in range 00078 if ((VolumeIn < 0.0) || (VolumeIn > 1.0)) { 00079 return false; 00080 } 00081 return true; 00082 } 00083 00084 void WM8978_RBSP::power(bool type) { 00085 00086 } 00087 00088 bool WM8978_RBSP::format(char length) { 00089 if (length != 16) { 00090 return false; 00091 } 00092 return true; 00093 } 00094 00095 bool WM8978_RBSP::frequency(int hz) { 00096 if (hz != 44100) { 00097 return false; 00098 } 00099 return true; 00100 } 00101 00102 // Private Functions 00103 void WM8978_RBSP::activateDigitalInterface_(void) { 00104 uint32_t reg_cmd; 00105 uint32_t reg_tmp; 00106 00107 /* ==== Soft Reset. ==== */ 00108 set_register(WM8978_REGADR_SOFT_RESET, WM8978_RESET_INI_VALUE); 00109 00110 /* ==== Set L/RMIXEN = 1 and DACENL/R = 1 in register R3. ==== */ 00111 reg_cmd = WM8978_MANAGE3_INI_VALUE; 00112 reg_cmd |= (WM8978_MANAGE3_RMIXEN_ON | 00113 WM8978_MANAGE3_LMIXEN_ON | 00114 WM8978_MANAGE3_DACENL_ON | 00115 WM8978_MANAGE3_DACENR_ON); 00116 set_register(WM8978_REGADR_POW_MANAGE3, reg_cmd); 00117 00118 /* ==== Set BUFIOEN = 1 and VMIDSEL[1:0] to required value in register R1. ==== */ 00119 reg_cmd = WM8978_MANAGE1_INI_VALUE; 00120 reg_cmd |= (WM8978_MANAGE1_BUFIOEN_ON | 00121 WM8978_MANAGE1_VMIDSEL_75K | 00122 WM8978_MANAGE1_MICBEN_ON | 00123 WM8978_MANAGE1_PLLEN_ON); 00124 set_register(WM8978_REGADR_POW_MANAGE1, reg_cmd); 00125 00126 /* ==== Set BIASEN = 1 in register R1. ==== */ 00127 reg_cmd |= WM8978_MANAGE1_BIASEN_ON; 00128 set_register(WM8978_REGADR_POW_MANAGE1, reg_cmd); 00129 00130 /* ==== Set L/ROUT1EN = 1 in register R2. ==== */ 00131 reg_cmd = WM8978_MANAGE2_INI_VALUE; 00132 reg_cmd |= (WM8978_MANAGE2_LOUT1EN_ON | 00133 WM8978_MANAGE2_ROUT1EN_ON | 00134 WM8978_MANAGE2_BOOSTENL_ON | 00135 WM8978_MANAGE2_BOOSTENR_ON); 00136 set_register(WM8978_REGADR_POW_MANAGE2, reg_cmd); 00137 00138 /* ==== Set INPPGAENL = 1 in register R2. ==== */ 00139 reg_cmd |= WM8978_MANAGE2_INPPGAENL_ON; 00140 set_register(WM8978_REGADR_POW_MANAGE2, reg_cmd); 00141 00142 /* ==== Set INPPGAENR = 1 in register R2. ==== */ 00143 reg_cmd |= WM8978_MANAGE2_INPPGAENR_ON; 00144 reg_tmp = reg_cmd; 00145 set_register(WM8978_REGADR_POW_MANAGE2, reg_cmd); 00146 00147 /* ==== Set L2_2INPPGA = 1 and R2_2INPPGA = 1 in register R44. ==== */ 00148 reg_cmd = WM8978_INPUTCTL_INI_VALUE; 00149 reg_cmd |= (WM8978_INPUTCTL_L2_2INPPGA_ON | WM8978_INPUTCTL_R2_2INPPGA_ON); 00150 set_register(WM8978_REGADR_INPUT_CTL, reg_cmd); 00151 00152 /* ==== Set LINPPGAGAIN = 0x0018 and MUTEL = 0 in register R45. ==== */ 00153 reg_cmd = WM8978_LINPPGAGAIN_INI_VOLL; 00154 reg_cmd &= ~WM8978_LINPPGAGAIN_MUTEL_ON; 00155 set_register(WM8978_REGADR_LINPPGAGAIN, reg_cmd); 00156 00157 /* ==== Set RINPPGAGAIN = 0x0018 and MUTEL = 0 in register R46. ==== */ 00158 reg_cmd = WM8978_RINPPGAGAIN_INI_VOLL; 00159 reg_cmd &= ~WM8978_RINPPGAGAIN_MUTER_BIT; 00160 set_register(WM8978_REGADR_RINPPGAGAIN, reg_cmd); 00161 00162 /* ==== Set ADCENL/ADCENR = 1 in register R2. ==== */ 00163 reg_cmd = reg_tmp; 00164 reg_cmd |= (WM8978_MANAGE2_ADCENL_ON | WM8978_MANAGE2_ADCENR_ON); 00165 set_register(WM8978_REGADR_POW_MANAGE2, reg_cmd); 00166 00167 /* ==== Set ADCOSR128 = 1 in register R14. ==== */ 00168 reg_cmd = WM8978_ADC_CTL_INI_VALUE; 00169 reg_cmd |= WM8978_ADC_CTL_ADCOSR128_ON; 00170 set_register(WM8978_REGADR_ADC_CTL, reg_cmd); 00171 00172 /* ==== Set HPFEN = 0 in register R14. ==== */ 00173 reg_cmd = WM8978_ADC_CTL_INI_VALUE; 00174 reg_cmd |= WM8978_ADC_CTL_ADCOSR128_ON; 00175 reg_cmd &= ~WM8978_ADC_CTL_HPFEN_BIT; 00176 set_register(WM8978_REGADR_ADC_CTL, reg_cmd); 00177 00178 /* ==== Set MCLKDIV = 0 in register R6. ==== */ 00179 if (ssif_cfg.slave_mode != false) { 00180 reg_cmd = WM8978_CLK_GEN_CTL_INI_VALUE; 00181 reg_cmd |= (0x2 << 2); 00182 reg_cmd |= 0x01; 00183 } else { 00184 reg_cmd = WM8978_CLK_GEN_CTL_INI_VALUE; 00185 reg_cmd &= ~WM8978_CLK_GEN_CTL_MCLKDIV_BIT; 00186 reg_cmd |= WM8978_CLK_GEN_CTL_MCLKDIV_DIV1; 00187 } 00188 set_register(WM8978_REGADR_CLK_GEN_CTL, reg_cmd); 00189 00190 /* ==== Set WL = 0 in register R4. ==== */ 00191 reg_cmd = WM8978_AUDIO_IF_INI_VALUE; 00192 reg_cmd &= ~WM8978_AUDIO_IF_WL_BIT; 00193 reg_cmd |= WM8978_AUDIO_IF_WL_16BIT; 00194 set_register(WM8978_REGADR_AUDIO_IF_CTL, reg_cmd); 00195 00196 /* ==== Set DACOSR128 = 1 in register R10. ==== */ 00197 reg_cmd = WM8978_DAC_CTL_INI_VALUE; 00198 reg_cmd |= WM8978_DAC_CTL_DACOSR128_ON; 00199 set_register(WM8978_REGADR_DAC_CTL, reg_cmd); 00200 } 00201 00202 void WM8978_RBSP::set_register(uint8_t reg_addr, uint16_t reg_cmd) { 00203 uint32_t reg_data; 00204 00205 reg_data = (((reg_addr << 9) & 0x0000FE00u) | (reg_cmd & 0x000001FFu)); 00206 mSpi_.write(reg_data); 00207 }
Generated on Wed Jul 13 2022 05:33:37 by
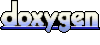