
test public
Dependencies: HttpServer_snapshot_mbed-os
USBHostMIDI.h
00001 /* Copyright (c) 2014 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBHOSTMIDI_H 00020 #define USBHOSTMIDI_H 00021 00022 #include "USBHostConf.h" 00023 00024 #if USBHOST_MIDI 00025 00026 #include "USBHost.h" 00027 00028 /** 00029 * A class to communicate a USB MIDI device 00030 */ 00031 class USBHostMIDI : public IUSBEnumerator { 00032 public: 00033 /** 00034 * Constructor 00035 */ 00036 USBHostMIDI(); 00037 #if defined(TARGET_RZ_A2XX) 00038 ~USBHostMIDI(); 00039 #endif 00040 00041 /** 00042 * Check if a USB MIDI device is connected 00043 * 00044 * @returns true if a midi device is connected 00045 */ 00046 bool connected(); 00047 00048 /** 00049 * Try to connect a midi device 00050 * 00051 * @return true if connection was successful 00052 */ 00053 bool connect(); 00054 00055 /** 00056 * Attach a callback called when miscellaneous function code is received 00057 * 00058 * @param ptr function pointer 00059 * prototype: void onMiscellaneousFunctionCode(uint8_t data1, uint8_t data2, uint8_t data3); 00060 */ 00061 inline void attachMiscellaneousFunctionCode(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00062 miscellaneousFunctionCode = fn; 00063 } 00064 00065 /** 00066 * Attach a callback called when cable event is received 00067 * 00068 * @param ptr function pointer 00069 * prototype: void onCableEvent(uint8_t data1, uint8_t data2, uint8_t data3); 00070 */ 00071 inline void attachCableEvent(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00072 cableEvent = fn; 00073 } 00074 00075 /** 00076 * Attach a callback called when system exclusive is received 00077 * 00078 * @param ptr function pointer 00079 * prototype: void onSystemCommonTwoBytes(uint8_t data1, uint8_t data2); 00080 */ 00081 inline void attachSystemCommonTwoBytes(void (*fn)(uint8_t, uint8_t)) { 00082 systemCommonTwoBytes = fn; 00083 } 00084 00085 /** 00086 * Attach a callback called when system exclusive is received 00087 * 00088 * @param ptr function pointer 00089 * prototype: void onSystemCommonThreeBytes(uint8_t data1, uint8_t data2, uint8_t data3); 00090 */ 00091 inline void attachSystemCommonThreeBytes(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00092 systemCommonThreeBytes = fn; 00093 } 00094 00095 /** 00096 * Attach a callback called when system exclusive is received 00097 * 00098 * @param ptr function pointer 00099 * prototype: void onSystemExclusive(uint8_t *data, uint16_t length, bool hasNextData); 00100 */ 00101 inline void attachSystemExclusive(void (*fn)(uint8_t *, uint16_t, bool)) { 00102 systemExclusive = fn; 00103 } 00104 00105 /** 00106 * Attach a callback called when note on is received 00107 * 00108 * @param ptr function pointer 00109 * prototype: void onNoteOn(uint8_t channel, uint8_t note, uint8_t velocity); 00110 */ 00111 inline void attachNoteOn(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00112 noteOn = fn; 00113 } 00114 00115 /** 00116 * Attach a callback called when note off is received 00117 * 00118 * @param ptr function pointer 00119 * prototype: void onNoteOff(uint8_t channel, uint8_t note, uint8_t velocity); 00120 */ 00121 inline void attachNoteOff(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00122 noteOff = fn; 00123 } 00124 00125 /** 00126 * Attach a callback called when poly keypress is received 00127 * 00128 * @param ptr function pointer 00129 * prototype: void onPolyKeyPress(uint8_t channel, uint8_t note, uint8_t pressure); 00130 */ 00131 inline void attachPolyKeyPress(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00132 polyKeyPress = fn; 00133 } 00134 00135 /** 00136 * Attach a callback called when control change is received 00137 * 00138 * @param ptr function pointer 00139 * prototype: void onControlChange(uint8_t channel, uint8_t key, uint8_t value); 00140 */ 00141 inline void attachControlChange(void (*fn)(uint8_t, uint8_t, uint8_t)) { 00142 controlChange = fn; 00143 } 00144 00145 /** 00146 * Attach a callback called when program change is received 00147 * 00148 * @param ptr function pointer 00149 * prototype: void onProgramChange(uint8_t channel, uint8_t program); 00150 */ 00151 inline void attachProgramChange(void (*fn)(uint8_t, uint8_t)) { 00152 programChange = fn; 00153 } 00154 00155 /** 00156 * Attach a callback called when channel pressure is received 00157 * 00158 * @param ptr function pointer 00159 * prototype: void onChannelPressure(uint8_t channel, uint8_t pressure); 00160 */ 00161 inline void attachChannelPressure(void (*fn)(uint8_t, uint8_t)) { 00162 channelPressure = fn; 00163 } 00164 00165 /** 00166 * Attach a callback called when pitch bend is received 00167 * 00168 * @param ptr function pointer 00169 * prototype: void onPitchBend(uint8_t channel, uint16_t value); 00170 */ 00171 inline void attachPitchBend(void (*fn)(uint8_t, uint16_t)) { 00172 pitchBend = fn; 00173 } 00174 00175 /** 00176 * Attach a callback called when single byte is received 00177 * 00178 * @param ptr function pointer 00179 * prototype: void onSingleByte(uint8_t value); 00180 */ 00181 inline void attachSingleByte(void (*fn)(uint8_t)) { 00182 singleByte = fn; 00183 } 00184 00185 /** 00186 * Send a cable event with 3 bytes event 00187 * 00188 * @param data1 0-255 00189 * @param data2 0-255 00190 * @param data3 0-255 00191 * @return true if message sent successfully 00192 */ 00193 bool sendMiscellaneousFunctionCode(uint8_t data1, uint8_t data2, uint8_t data3); 00194 00195 /** 00196 * Send a cable event with 3 bytes event 00197 * 00198 * @param data1 0-255 00199 * @param data2 0-255 00200 * @param data3 0-255 00201 * @return true if message sent successfully 00202 */ 00203 bool sendCableEvent(uint8_t data1, uint8_t data2, uint8_t data3); 00204 00205 /** 00206 * Send a system common message with 2 bytes event 00207 * 00208 * @param data1 0-255 00209 * @param data2 0-255 00210 * @return true if message sent successfully 00211 */ 00212 bool sendSystemCommmonTwoBytes(uint8_t data1, uint8_t data2); 00213 00214 /** 00215 * Send a system common message with 3 bytes event 00216 * 00217 * @param data1 0-255 00218 * @param data2 0-255 00219 * @param data3 0-255 00220 * @return true if message sent successfully 00221 */ 00222 bool sendSystemCommmonThreeBytes(uint8_t data1, uint8_t data2, uint8_t data3); 00223 00224 /** 00225 * Send a system exclusive event 00226 * 00227 * @param buffer, starts with 0xF0, and end with 0xf7 00228 * @param length 00229 * @return true if message sent successfully 00230 */ 00231 bool sendSystemExclusive(uint8_t *buffer, int length); 00232 00233 /** 00234 * Send a note off event 00235 * 00236 * @param channel 0-15 00237 * @param note 0-127 00238 * @param velocity 0-127 00239 * @return true if message sent successfully 00240 */ 00241 bool sendNoteOff(uint8_t channel, uint8_t note, uint8_t velocity); 00242 00243 /** 00244 * Send a note on event 00245 * 00246 * @param channel 0-15 00247 * @param note 0-127 00248 * @param velocity 0-127 (0 means note off) 00249 * @return true if message sent successfully 00250 */ 00251 bool sendNoteOn(uint8_t channel, uint8_t note, uint8_t velocity); 00252 00253 /** 00254 * Send a poly keypress event 00255 * 00256 * @param channel 0-15 00257 * @param note 0-127 00258 * @param pressure 0-127 00259 * @return true if message sent successfully 00260 */ 00261 bool sendPolyKeyPress(uint8_t channel, uint8_t note, uint8_t pressure); 00262 00263 /** 00264 * Send a control change event 00265 * 00266 * @param channel 0-15 00267 * @param key 0-127 00268 * @param value 0-127 00269 * @return true if message sent successfully 00270 */ 00271 bool sendControlChange(uint8_t channel, uint8_t key, uint8_t value); 00272 00273 /** 00274 * Send a program change event 00275 * 00276 * @param channel 0-15 00277 * @param program 0-127 00278 * @return true if message sent successfully 00279 */ 00280 bool sendProgramChange(uint8_t channel, uint8_t program); 00281 00282 /** 00283 * Send a channel pressure event 00284 * 00285 * @param channel 0-15 00286 * @param pressure 0-127 00287 * @return true if message sent successfully 00288 */ 00289 bool sendChannelPressure(uint8_t channel, uint8_t pressure); 00290 00291 /** 00292 * Send a control change event 00293 * 00294 * @param channel 0-15 00295 * @param key 0(lower)-8191(center)-16383(higher) 00296 * @return true if message sent successfully 00297 */ 00298 bool sendPitchBend(uint8_t channel, uint16_t value); 00299 00300 /** 00301 * Send a single byte event 00302 * 00303 * @param data 0-255 00304 * @return true if message sent successfully 00305 */ 00306 bool sendSingleByte(uint8_t data); 00307 00308 protected: 00309 //From IUSBEnumerator 00310 virtual void setVidPid(uint16_t vid, uint16_t pid); 00311 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00312 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00313 00314 private: 00315 USBHost * host; 00316 USBDeviceConnected * dev; 00317 USBEndpoint * bulk_in; 00318 USBEndpoint * bulk_out; 00319 uint32_t size_bulk_in; 00320 uint32_t size_bulk_out; 00321 00322 bool dev_connected; 00323 00324 void init(); 00325 00326 #if defined(TARGET_RZ_A2XX) 00327 uint8_t * buf_in; 00328 uint8_t * buf_out; 00329 #else 00330 uint8_t buf[64]; 00331 #endif 00332 00333 void rxHandler(); 00334 00335 uint16_t sysExBufferPos; 00336 uint8_t sysExBuffer[64]; 00337 00338 void (*miscellaneousFunctionCode)(uint8_t, uint8_t, uint8_t); 00339 void (*cableEvent)(uint8_t, uint8_t, uint8_t); 00340 void (*systemCommonTwoBytes)(uint8_t, uint8_t); 00341 void (*systemCommonThreeBytes)(uint8_t, uint8_t, uint8_t); 00342 void (*systemExclusive)(uint8_t *, uint16_t, bool); 00343 void (*noteOff)(uint8_t, uint8_t, uint8_t); 00344 void (*noteOn)(uint8_t, uint8_t, uint8_t); 00345 void (*polyKeyPress)(uint8_t, uint8_t, uint8_t); 00346 void (*controlChange)(uint8_t, uint8_t, uint8_t); 00347 void (*programChange)(uint8_t, uint8_t); 00348 void (*channelPressure)(uint8_t, uint8_t); 00349 void (*pitchBend)(uint8_t, uint16_t); 00350 void (*singleByte)(uint8_t); 00351 00352 bool sendMidiBuffer(uint8_t data0, uint8_t data1, uint8_t data2, uint8_t data3); 00353 00354 int midi_intf; 00355 bool midi_device_found; 00356 00357 }; 00358 00359 #endif /* USBHOST_MIDI */ 00360 00361 #endif /* USBHOSTMIDI_H */
Generated on Wed Jul 13 2022 05:33:37 by
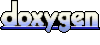