
test public
Dependencies: HttpServer_snapshot_mbed-os
TouchKey_RSK_TFT.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (C) 2016 Renesas Electronics Corporation. All rights reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "TouchKey_RSK_TFT.h" 00018 00019 TouchKey_RSK_TFT::TouchKey_RSK_TFT(PinName tprst, PinName tpint, PinName sda, PinName scl) : 00020 TouchKey(tprst, tpint), i2c(sda, scl) { 00021 } 00022 00023 int TouchKey_RSK_TFT::GetMaxTouchNum(void) { 00024 return 5; 00025 } 00026 00027 int TouchKey_RSK_TFT::GetCoordinates(int touch_buff_num, touch_pos_t * p_touch) { 00028 char buf[32]; 00029 uint32_t wk_x; 00030 uint32_t wk_y; 00031 touch_pos_t * wk_touch; 00032 int count = 0; 00033 int i; 00034 int read_size; 00035 00036 if (touch_buff_num > GetMaxTouchNum()) { 00037 touch_buff_num = GetMaxTouchNum(); 00038 } 00039 read_size = 2 + 6 * touch_buff_num; 00040 00041 if (p_touch != NULL) { 00042 for (i = 0; i < touch_buff_num; i++) { 00043 wk_touch = &p_touch[i]; 00044 wk_touch->x = 0; 00045 wk_touch->y = 0; 00046 wk_touch->valid = false; 00047 } 00048 if (i2c.read((0x38 << 1), buf, read_size) == 0) { 00049 for (i = 0; i < touch_buff_num; i++) { 00050 if (buf[2] > i) { 00051 wk_touch = &p_touch[i]; 00052 wk_x = (((uint32_t)buf[3 + (6 * i)] & 0x0F) << 8) | buf[4 + (6 * i)]; 00053 if (wk_x < 800) { 00054 wk_touch->x = 800 - wk_x; 00055 } else { 00056 wk_touch->x = 0; 00057 } 00058 wk_y = (((uint32_t)buf[5 + (6 * i)] & 0x0F) << 8) | buf[6 + (6 * i)]; 00059 if (wk_y < 480) { 00060 wk_touch->y = 480 - wk_y; 00061 } else { 00062 wk_touch->y = 0; 00063 } 00064 wk_touch->valid = 1; 00065 count++; 00066 } 00067 } 00068 } 00069 } 00070 00071 return count; 00072 } 00073 00074
Generated on Wed Jul 13 2022 05:33:37 by
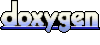