
test public
Dependencies: HttpServer_snapshot_mbed-os
TouchKey_4_3inch.h
00001 /* mbed Microcontroller Library 00002 * Copyright (C) 2016 Renesas Electronics Corporation. All rights reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 /**************************************************************************//** 00017 * @file TouchKey_4_3inch.h 00018 * @brief TouchKey_4_3inch API 00019 ******************************************************************************/ 00020 00021 #ifndef TOUCH_KEY_4_3INCH_H 00022 #define TOUCH_KEY_4_3INCH_H 00023 00024 #include "TouchKey.h" 00025 00026 /** 00027 * The class to acquire touch coordinates. (GR-PEACH 4.3inch LCD Shield edition) 00028 */ 00029 class TouchKey_4_3inch : public TouchKey { 00030 00031 public: 00032 00033 /** Create a TouchKey_4_3inch object 00034 * 00035 * @param tprst tprst pin 00036 * @param tpint tpint pin 00037 * @param sda I2C data line pin 00038 * @param scl I2C clock line pin 00039 */ 00040 TouchKey_4_3inch(PinName tprst, PinName tpint, PinName sda = I2C_SDA, PinName scl = I2C_SCL); 00041 virtual int GetMaxTouchNum(void); 00042 virtual int GetCoordinates(int touch_buff_num, touch_pos_t * p_touch); 00043 00044 private: 00045 typedef struct { 00046 uint8_t y_h: 3, 00047 reserved: 1, 00048 x_h: 3, 00049 valid: 1; 00050 uint8_t x_l; 00051 uint8_t y_l; 00052 } xyz_data_t; 00053 00054 typedef struct { 00055 uint8_t fingers: 3, 00056 reserved: 5; 00057 uint8_t keys; 00058 xyz_data_t xyz_data[2]; 00059 } stx_report_data_t; 00060 00061 I2C i2c; 00062 }; 00063 00064 #endif 00065 00066 00067
Generated on Wed Jul 13 2022 05:33:37 by
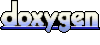