
test public
Dependencies: HttpServer_snapshot_mbed-os
SoundlessSpeaker.h
00001 /* mbed SoundlessSpeaker Library 00002 * Copyright (C) 2016 dkato 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef SOUNDLESS_SPEAKER_H 00018 #define SOUNDLESS_SPEAKER_H 00019 00020 #include "mbed.h" 00021 #include "AUDIO_RBSP.h" 00022 00023 /** SoundlessSpeaker class 00024 * 00025 */ 00026 class SoundlessSpeaker : public AUDIO_RBSP { 00027 public: 00028 /** Create a SoundlessSpeaker 00029 * 00030 */ 00031 SoundlessSpeaker(); 00032 00033 virtual ~SoundlessSpeaker() {} 00034 00035 virtual void power(bool type = true) { 00036 return; 00037 } 00038 00039 /** Set I2S interface bit length and mode 00040 * 00041 * @param length Set bit length to 8 or 16 bits 00042 * @return true = success, false = failure 00043 */ 00044 virtual bool format(char length); 00045 00046 /** Set sample frequency 00047 * 00048 * @param frequency Sample frequency of data in Hz 00049 * @return true = success, false = failure 00050 * 00051 * Supports the following frequencies: 8kHz, 8.021kHz, 32kHz, 44.1kHz, 48kHz 00052 * Default is 44.1kHz 00053 */ 00054 virtual bool frequency(int hz); 00055 00056 /** Enqueue asynchronous write request 00057 * 00058 * @param p_data Location of the data 00059 * @param data_size Number of bytes to write 00060 * @return Number of bytes written on success. negative number on error. 00061 */ 00062 virtual int write(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf = NULL); 00063 00064 virtual int read(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf = NULL) { 00065 return -1; 00066 } 00067 00068 virtual bool outputVolume(float leftVolumeOut, float rightVolumeOut) { 00069 return false; 00070 } 00071 00072 virtual bool micVolume(float VolumeIn) { 00073 return false; 00074 } 00075 00076 private: 00077 Timer _t; 00078 int _next_time; 00079 int _length; 00080 int _hz; 00081 int _byte_per_sec; 00082 }; 00083 00084 #endif // SOUNDLESS_SPEAKER_H
Generated on Wed Jul 13 2022 05:33:37 by
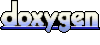