
test public
Dependencies: HttpServer_snapshot_mbed-os
RomRamBlockDevice.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "RomRamBlockDevice.h" 00018 #if defined(TARGET_RENESAS) 00019 #include "mbed_drv_cfg.h" 00020 00021 #else 00022 #define FLASH_BASE 0xFFFFFFFF 00023 #define FLASH_SIZE 0xFFFFFFFF 00024 #endif 00025 00026 RomRamBlockDevice::RomRamBlockDevice(bd_size_t size, bd_size_t block) 00027 : _read_size(block), _program_size(block), _erase_size(block) 00028 , _count(size / block), _blocks(0), _rom_start(FLASH_BASE), _rom_end(FLASH_BASE + FLASH_SIZE - 1) 00029 { 00030 MBED_ASSERT(_count * _erase_size == size); 00031 } 00032 00033 RomRamBlockDevice::RomRamBlockDevice(bd_size_t size, bd_size_t read, bd_size_t program, bd_size_t erase) 00034 : _read_size(read), _program_size(program), _erase_size(erase) 00035 , _count(size / erase), _blocks(0), _rom_start(FLASH_BASE), _rom_end(FLASH_BASE + FLASH_SIZE - 1) 00036 { 00037 MBED_ASSERT(_count * _erase_size == size); 00038 } 00039 00040 RomRamBlockDevice::~RomRamBlockDevice() 00041 { 00042 if (_blocks) { 00043 for (size_t i = 0; i < _count; i++) { 00044 free(_blocks[i]); 00045 } 00046 00047 delete[] _blocks; 00048 _blocks = 0; 00049 } 00050 } 00051 00052 void RomRamBlockDevice::SetRomAddr(uint32_t rom_start_addr, uint32_t rom_end_addr) { 00053 _rom_start = rom_start_addr; 00054 _rom_end = rom_end_addr; 00055 } 00056 00057 int RomRamBlockDevice::init() 00058 { 00059 if (!_blocks) { 00060 _blocks = new uint8_t*[_count]; 00061 for (size_t i = 0; i < _count; i++) { 00062 _blocks[i] = 0; 00063 } 00064 } 00065 00066 return BD_ERROR_OK; 00067 } 00068 00069 int RomRamBlockDevice::deinit() 00070 { 00071 // Heapory is lazily cleaned up in destructor to allow 00072 // data to live across de/reinitialization 00073 return BD_ERROR_OK; 00074 } 00075 00076 bd_size_t RomRamBlockDevice::get_read_size() const 00077 { 00078 return _read_size; 00079 } 00080 00081 bd_size_t RomRamBlockDevice::get_program_size() const 00082 { 00083 return _program_size; 00084 } 00085 00086 bd_size_t RomRamBlockDevice::get_erase_size() const 00087 { 00088 return _erase_size; 00089 } 00090 00091 bd_size_t RomRamBlockDevice::size() const 00092 { 00093 return _count * _erase_size; 00094 } 00095 00096 int RomRamBlockDevice::read(void *b, bd_addr_t addr, bd_size_t size) 00097 { 00098 MBED_ASSERT(is_valid_read(addr, size)); 00099 uint8_t *buffer = static_cast<uint8_t*>(b); 00100 00101 while (size > 0) { 00102 bd_addr_t hi = addr / _erase_size; 00103 bd_addr_t lo = addr % _erase_size; 00104 00105 if (_blocks[hi]) { 00106 memcpy(buffer, &_blocks[hi][lo], _read_size); 00107 } else { 00108 memset(buffer, 0, _read_size); 00109 } 00110 00111 buffer += _read_size; 00112 addr += _read_size; 00113 size -= _read_size; 00114 } 00115 00116 return 0; 00117 } 00118 00119 int RomRamBlockDevice::program(const void *b, bd_addr_t addr, bd_size_t size) 00120 { 00121 MBED_ASSERT(is_valid_program(addr, size)); 00122 const uint8_t *buffer = static_cast<const uint8_t*>(b); 00123 00124 while (size > 0) { 00125 bd_addr_t hi = addr / _erase_size; 00126 bd_addr_t lo = addr % _erase_size; 00127 00128 if (isRomAddress(buffer)) { 00129 if (!isRomAddress(_blocks[hi])) { 00130 free(_blocks[hi]); 00131 } 00132 _blocks[hi] = (uint8_t*)buffer; 00133 } else { 00134 if (!_blocks[hi]) { 00135 _blocks[hi] = (uint8_t*)malloc(_erase_size); 00136 if (!_blocks[hi]) { 00137 return BD_ERROR_DEVICE_ERROR; 00138 } 00139 } 00140 memcpy(&_blocks[hi][lo], buffer, _program_size); 00141 } 00142 00143 buffer += _program_size; 00144 addr += _program_size; 00145 size -= _program_size; 00146 } 00147 00148 return 0; 00149 } 00150 00151 int RomRamBlockDevice::erase(bd_addr_t addr, bd_size_t size) 00152 { 00153 MBED_ASSERT(is_valid_erase(addr, size)); 00154 // TODO assert on programming unerased blocks 00155 00156 return 0; 00157 } 00158 00159 bool RomRamBlockDevice::isRomAddress(const uint8_t *address) { 00160 if (((uint32_t)address >= _rom_start) 00161 && ((uint32_t)address <= (_rom_end - _erase_size + 1))) { 00162 return true; 00163 } 00164 return false; 00165 } 00166 00167 const char *RomRamBlockDevice::get_type() const 00168 { 00169 return "ROMRAM"; 00170 } 00171
Generated on Wed Jul 13 2022 05:33:37 by
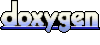