
test public
Dependencies: HttpServer_snapshot_mbed-os
R_BSP_Spdif.cpp
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2018 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #include "R_BSP_Spdif.h" 00025 #if (R_BSP_SPDIF_ENABLE == 1) 00026 #include "r_bsp_cmn.h" 00027 #include "spdif_if.h" 00028 #include "spdif_api.h" 00029 00030 R_BSP_Spdif::R_BSP_Spdif(PinName audio_clk, PinName tx, PinName rx) : spdif_ch(-1) { 00031 int32_t wk_channel; 00032 00033 wk_channel = spdif_init(audio_clk, tx, rx); 00034 if (wk_channel != NC) { 00035 spdif_ch = wk_channel; 00036 } 00037 } 00038 00039 R_BSP_Spdif::~R_BSP_Spdif() { 00040 // do nothing 00041 } 00042 00043 void R_BSP_Spdif::init(const spdif_channel_cfg_t* const p_ch_cfg, int32_t max_write_num, int32_t max_read_num) { 00044 if (spdif_ch >= 0) { 00045 init_channel(R_SPDIF_MakeCbTbl_mbed(), spdif_ch, (void *)p_ch_cfg, max_write_num, max_read_num); 00046 } 00047 } 00048 00049 bool R_BSP_Spdif::ConfigChannel(const spdif_channel_cfg_t* const p_ch_cfg) { 00050 return ioctl(SPDIF_CONFIG_CHANNEL, (void *)p_ch_cfg); 00051 } 00052 00053 bool R_BSP_Spdif::SetChannelStatus(uint32_t status_Lch, uint32_t status_Rch) { 00054 spdif_channel_status_t channel_status; 00055 00056 channel_status.Lch = status_Lch; 00057 channel_status.Rch = status_Rch; 00058 00059 return ioctl(SPDIF_SET_CHANNEL_STATUS, (void *)&channel_status); 00060 } 00061 00062 bool R_BSP_Spdif::GetChannelStatus(uint32_t * p_status_Lch, uint32_t * p_status_Rch) { 00063 spdif_channel_status_t channel_status; 00064 00065 if (ioctl(SPDIF_GET_CHANNEL_STATUS, (void *)&channel_status) != false) { 00066 if (p_status_Lch != NULL) { 00067 *p_status_Lch = channel_status.Lch; 00068 } 00069 if (p_status_Rch != NULL) { 00070 *p_status_Rch = channel_status.Rch; 00071 } 00072 } 00073 00074 return true; 00075 } 00076 00077 bool R_BSP_Spdif::SetTransAudioBit(int direction, char length) { 00078 spdif_chcfg_audio_bit_t audio_bit; 00079 int control_type; 00080 00081 if (direction == 0) { 00082 control_type = SPDIF_SET_TRANS_AUDIO_BIT; 00083 } else { 00084 control_type = SPDIF_SET_RECV_AUDIO_BIT; 00085 } 00086 00087 switch (length) { 00088 case 16: 00089 audio_bit = SPDIF_CFG_AUDIO_BIT_16; 00090 break; 00091 case 20: 00092 audio_bit = SPDIF_CFG_AUDIO_BIT_20; 00093 break; 00094 case 24: 00095 audio_bit = SPDIF_CFG_AUDIO_BIT_24; 00096 break; 00097 default: 00098 return false; 00099 } 00100 00101 return ioctl(control_type, (void *)&audio_bit); 00102 } 00103 00104 #endif /* R_BSP_SPDIF_ENABLE */
Generated on Wed Jul 13 2022 05:33:36 by
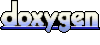