
test public
Dependencies: HttpServer_snapshot_mbed-os
ESP32Interface.h
00001 /* ESP32 implementation of NetworkInterfaceAPI 00002 * Copyright (c) 2017 Renesas Electronics Corporation 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef ESP32_INTERFACE_H 00018 #define ESP32_INTERFACE_H 00019 00020 #include "mbed.h" 00021 #include "ESP32Stack.h" 00022 00023 /** ESP32Interface class 00024 * Implementation of the NetworkStack for the ESP32 00025 */ 00026 class ESP32Interface : public ESP32Stack, public WiFiInterface 00027 { 00028 public: 00029 /** ESP32Interface lifetime 00030 * Configuration defined in mbed_lib.json 00031 */ 00032 ESP32Interface(); 00033 00034 /** ESP32Interface lifetime 00035 * @param en EN pin (If not used this pin, please set "NC") 00036 * @param io0 IO0 pin (If not used this pin, please set "NC") 00037 * @param tx TX pin 00038 * @param rx RX pin 00039 * @param debug Enable debugging 00040 * @param rts RTS pin 00041 * @param cts CTS pin 00042 * @param baudrate The baudrate of the serial port (default = 230400). 00043 */ 00044 ESP32Interface(PinName en, PinName io0, PinName tx, PinName rx, bool debug = false, 00045 PinName rts = NC, PinName cts = NC, int baudrate = 230400); 00046 00047 /** ESP32Interface lifetime 00048 * @param tx TX pin 00049 * @param rx RX pin 00050 * @param debug Enable debugging 00051 */ 00052 ESP32Interface(PinName tx, PinName rx, bool debug = false); 00053 00054 /** Set a static IP address 00055 * 00056 * Configures this network interface to use a static IP address. 00057 * Implicitly disables DHCP, which can be enabled in set_dhcp. 00058 * Requires that the network is disconnected. 00059 * 00060 * @param ip_address Null-terminated representation of the local IP address 00061 * @param netmask Null-terminated representation of the local network mask 00062 * @param gateway Null-terminated representation of the local gateway 00063 * @return 0 on success, negative error code on failure 00064 */ 00065 virtual nsapi_error_t set_network( 00066 const char *ip_address, const char *netmask, const char *gateway); 00067 00068 /** Enable or disable DHCP on the network 00069 * 00070 * Enables DHCP on connecting the network. Defaults to enabled unless 00071 * a static IP address has been assigned. Requires that the network is 00072 * disconnected. 00073 * 00074 * @param dhcp True to enable DHCP 00075 * @return 0 on success, negative error code on failure 00076 */ 00077 virtual nsapi_error_t set_dhcp(bool dhcp); 00078 00079 /** Start the interface 00080 * 00081 * Attempts to connect to a WiFi network. Requires ssid and passphrase to be set. 00082 * If passphrase is invalid, NSAPI_ERROR_AUTH_ERROR is returned. 00083 * 00084 * @return 0 on success, negative error code on failure 00085 */ 00086 virtual int connect(); 00087 00088 /** Start the interface 00089 * 00090 * Attempts to connect to a WiFi network. 00091 * 00092 * @param ssid Name of the network to connect to 00093 * @param pass Security passphrase to connect to the network 00094 * @param security Type of encryption for connection (Default: NSAPI_SECURITY_NONE) 00095 * @param channel This parameter is not supported, setting it to anything else than 0 will result in NSAPI_ERROR_UNSUPPORTED 00096 * @return 0 on success, or error code on failure 00097 */ 00098 virtual int connect(const char *ssid, const char *pass, nsapi_security_t security = NSAPI_SECURITY_NONE, 00099 uint8_t channel = 0); 00100 00101 /** Set the WiFi network credentials 00102 * 00103 * @param ssid Name of the network to connect to 00104 * @param pass Security passphrase to connect to the network 00105 * @param security Type of encryption for connection 00106 * (defaults to NSAPI_SECURITY_NONE) 00107 * @return 0 on success, or error code on failure 00108 */ 00109 virtual int set_credentials(const char *ssid, const char *pass, nsapi_security_t security = NSAPI_SECURITY_NONE); 00110 00111 /** Set the WiFi network channel - NOT SUPPORTED 00112 * 00113 * This function is not supported and will return NSAPI_ERROR_UNSUPPORTED 00114 * 00115 * @param channel Channel on which the connection is to be made, or 0 for any (Default: 0) 00116 * @return Not supported, returns NSAPI_ERROR_UNSUPPORTED 00117 */ 00118 virtual int set_channel(uint8_t channel); 00119 00120 /** Stop the interface 00121 * @return 0 on success, negative on failure 00122 */ 00123 virtual int disconnect(); 00124 00125 /** Get the internally stored IP address 00126 * @return IP address of the interface or null if not yet connected 00127 */ 00128 virtual const char *get_ip_address(); 00129 00130 /** Get the internally stored MAC address 00131 * @return MAC address of the interface 00132 */ 00133 virtual const char *get_mac_address(); 00134 00135 /** Get the local gateway 00136 * 00137 * @return Null-terminated representation of the local gateway 00138 * or null if no network mask has been recieved 00139 */ 00140 virtual const char *get_gateway(); 00141 00142 /** Get the local network mask 00143 * 00144 * @return Null-terminated representation of the local network mask 00145 * or null if no network mask has been recieved 00146 */ 00147 virtual const char *get_netmask(); 00148 00149 /** Gets the current radio signal strength for active connection 00150 * 00151 * @return Connection strength in dBm (negative value) 00152 */ 00153 virtual int8_t get_rssi(); 00154 00155 /** Scan for available networks 00156 * 00157 * This function will block. 00158 * 00159 * @param ap Pointer to allocated array to store discovered AP 00160 * @param count Size of allocated @a res array, or 0 to only count available AP 00161 * @param timeout Timeout in milliseconds; 0 for no timeout (Default: 0) 00162 * @return Number of entries in @a, or if @a count was 0 number of available networks, negative on error 00163 * see @a nsapi_error 00164 */ 00165 virtual int scan(WiFiAccessPoint *res, unsigned count); 00166 00167 /** Translates a hostname to an IP address with specific version 00168 * 00169 * The hostname may be either a domain name or an IP address. If the 00170 * hostname is an IP address, no network transactions will be performed. 00171 * 00172 * If no stack-specific DNS resolution is provided, the hostname 00173 * will be resolve using a UDP socket on the stack. 00174 * 00175 * @param address Destination for the host SocketAddress 00176 * @param host Hostname to resolve 00177 * @param version IP version of address to resolve, NSAPI_UNSPEC indicates 00178 * version is chosen by the stack (defaults to NSAPI_UNSPEC) 00179 * @return 0 on success, negative error code on failure 00180 */ 00181 using NetworkInterface::gethostbyname; 00182 00183 /** Add a domain name server to list of servers to query 00184 * 00185 * @param addr Destination for the host address 00186 * @return 0 on success, negative error code on failure 00187 */ 00188 using NetworkInterface::add_dns_server; 00189 00190 /** Register callback for status reporting 00191 * 00192 * The specified status callback function will be called on status changes 00193 * on the network. The parameters on the callback are the event type and 00194 * event-type dependent reason parameter. 00195 * 00196 * In ESP32 the callback will be called when processing OOB-messages via 00197 * AT-parser. Do NOT call any ESP8266Interface -functions or do extensive 00198 * processing in the callback. 00199 * 00200 * @param status_cb The callback for status changes 00201 */ 00202 virtual void attach(mbed::Callback<void(nsapi_event_t, intptr_t)> status_cb); 00203 00204 /** Get the connection status 00205 * 00206 * @return The connection status according to ConnectionStatusType 00207 */ 00208 virtual nsapi_connection_status_t get_connection_status() const; 00209 00210 /** Provide access to the NetworkStack object 00211 * 00212 * @return The underlying NetworkStack object 00213 */ 00214 virtual NetworkStack *get_stack() 00215 { 00216 return this; 00217 } 00218 00219 private: 00220 bool _dhcp; 00221 char _ap_ssid[33]; /* 32 is what 802.11 defines as longest possible name; +1 for the \0 */ 00222 char _ap_pass[64]; /* The longest allowed passphrase */ 00223 nsapi_security_t _ap_sec; 00224 char _ip_address[NSAPI_IPv6_SIZE]; 00225 char _netmask[NSAPI_IPv4_SIZE]; 00226 char _gateway[NSAPI_IPv4_SIZE]; 00227 nsapi_connection_status_t _connection_status; 00228 Callback<void(nsapi_event_t, intptr_t)> _connection_status_cb; 00229 00230 void set_connection_status(nsapi_connection_status_t connection_status); 00231 void wifi_status_cb(int8_t wifi_status); 00232 }; 00233 00234 #endif
Generated on Wed Jul 13 2022 05:33:36 by
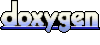