
test public
Dependencies: HttpServer_snapshot_mbed-os
AsciiFont.h
00001 /* Copyright (c) 2016 dkato 00002 * SPDX-License-Identifier: Apache-2.0 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 /**************************************************************************//** 00017 * @file AsciiFont.h 00018 * @brief AsciiFont API 00019 ******************************************************************************/ 00020 00021 #ifndef ASCII_FONT_H 00022 #define ASCII_FONT_H 00023 00024 #include "mbed.h" 00025 00026 /** Draw the character of the ASCII code. 00027 * 00028 * Example 00029 * @code 00030 * #include "mbed.h" 00031 * #include "AsciiFont.h" 00032 * 00033 * #define WIDTH (12) 00034 * #define HEIGHT (16) 00035 * #define BYTE_PER_PIXEL (1u) 00036 * #define STRIDE (((WIDTH * BYTE_PER_PIXEL) + 7u) & ~7u) //multiple of 8 00037 * 00038 * uint8_t text_field[STRIDE * HEIGHT]; 00039 * 00040 * //for debug 00041 * void print_text_field() { 00042 * int idx = 0; 00043 * 00044 * for (int i = 0; i < HEIGHT; i++) { 00045 * for (int j = 0; j < STRIDE; j++) { 00046 * printf("%02x", text_field[idx++]); 00047 * } 00048 * printf("\r\n"); 00049 * } 00050 * printf("\r\n"); 00051 * } 00052 * 00053 * int main() { 00054 * AsciiFont ascii_font(text_field, WIDTH, HEIGHT, STRIDE, BYTE_PER_PIXEL); 00055 * 00056 * ascii_font.Erase(0xcc); 00057 * ascii_font.DrawStr("AB", 0, 0, 0x11, 1); 00058 * ascii_font.DrawChar('C', AsciiFont::CHAR_PIX_WIDTH, 00059 * AsciiFont::CHAR_PIX_HEIGHT, 0x22, 1); 00060 * print_text_field(); //debug print 00061 * 00062 * ascii_font.Erase(); 00063 * ascii_font.DrawStr("D", 0, 0, 0xef, 2); 00064 * print_text_field(); //debug print 00065 * 00066 * ascii_font.Erase(0x11, 6, 0, 6, 8); 00067 * print_text_field(); //debug print 00068 * } 00069 * @endcode 00070 */ 00071 00072 class AsciiFont { 00073 public: 00074 00075 /** Constructor: Initializes AsciiFont. 00076 * 00077 * @param p_buf Text field address 00078 * @param width Text field width 00079 * @param height Text field height 00080 * @param stride Buffer stride 00081 * @param colour Background color 00082 * @param byte_per_pixel Byte per pixel 00083 */ 00084 AsciiFont(uint8_t * p_buf, int width, int height, int stride, int byte_per_pixel, uint32_t const colour = 0); 00085 00086 /** Erase text field 00087 * 00088 */ 00089 void Erase(); 00090 00091 /** Erase text field 00092 * 00093 * @param colour Background color 00094 */ 00095 void Erase(uint32_t const colour); 00096 00097 /** Erase text field 00098 * 00099 * @param colour Background color 00100 * @param x Erase start position of x coordinate 00101 * @param y Erase start position of y coordinate 00102 * @param width Erase field width 00103 * @param height Erase field height 00104 */ 00105 void Erase(uint32_t const colour, int x, int y, int width, int height); 00106 00107 /** Draw a string 00108 * 00109 * @param str String 00110 * @param x Drawing start position of x coordinate 00111 * @param y Drawing start position of y coordinate 00112 * @param color Font color 00113 * @param font_size Font size (>=1) 00114 * @param max_char_num The maximum number of characters 00115 * @return The drawn number of characters 00116 */ 00117 int DrawStr(const char * str, int x, int y, uint32_t const colour, int font_size = 1, uint16_t const max_char_num = 0xffff); 00118 00119 /** Draw a character 00120 * 00121 * @param x Drawing start position of x coordinate 00122 * @param y Drawing start position of y coordinate 00123 * @param color Font color 00124 * @param font_size Font size (>=1) 00125 * @return true if successfull 00126 */ 00127 bool DrawChar(char c, int x, int y, uint32_t const colour, int font_size = 1); 00128 00129 /** The pixel width of a character. (font_size=1) 00130 * 00131 */ 00132 static const int CHAR_PIX_WIDTH = 6; 00133 00134 /** The pixel height of a character. (font_size=1) 00135 * 00136 */ 00137 static const int CHAR_PIX_HEIGHT = 8; 00138 00139 private: 00140 uint8_t * p_text_field; 00141 int max_width; 00142 int max_height; 00143 int buf_stride; 00144 int pixel_num; 00145 uint32_t background_colour; 00146 }; 00147 #endif
Generated on Wed Jul 13 2022 05:33:36 by
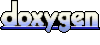