
test public
Dependencies: HttpServer_snapshot_mbed-os
AUDIO_GRBoard.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2017 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #ifndef MBED_AUDIO_GRBOARD_H 00025 #define MBED_AUDIO_GRBOARD_H 00026 00027 #if defined(TARGET_RZ_A1H) 00028 00029 #include "mbed.h" 00030 #include "TLV320_RBSP.h" 00031 00032 /** AUDIO_GRBoard class 00033 * 00034 */ 00035 class AUDIO_GRBoard : public TLV320_RBSP { 00036 public: 00037 00038 /** Create a audio codec class 00039 * 00040 * @param int_level Interupt priority (SSIF) 00041 * @param max_write_num The upper limit of write buffer (SSIF) 00042 * @param max_read_num The upper limit of read buffer (SSIF) 00043 */ 00044 AUDIO_GRBoard(uint8_t int_level = 0x80, int32_t max_write_num = 16, int32_t max_read_num = 16) : 00045 TLV320_RBSP(P10_13, I2C_SDA, I2C_SCL, P4_4, P4_5, P4_7, P4_6, int_level, max_write_num, max_read_num) { 00046 TLV320_RBSP::mic(true); 00047 TLV320_RBSP::micVolume(true, false); 00048 } 00049 }; 00050 00051 #elif defined(TARGET_GR_LYCHEE) 00052 00053 #include "mbed.h" 00054 #include "MAX9867_RBSP.h" 00055 00056 /** AUDIO_GRBoard class 00057 * 00058 */ 00059 class AUDIO_GRBoard : public MAX9867_RBSP { 00060 public: 00061 00062 /** Create a audio codec class 00063 * 00064 * @param int_level Interupt priority (SSIF) 00065 * @param max_write_num The upper limit of write buffer (SSIF) 00066 * @param max_read_num The upper limit of read buffer (SSIF) 00067 */ 00068 AUDIO_GRBoard(uint8_t int_level = 0x80, int32_t max_write_num = 16, int32_t max_read_num = 16) : 00069 MAX9867_RBSP(P1_7, P1_6, P2_7, P2_9, P2_8, P2_6, int_level, max_write_num, max_read_num) { 00070 } 00071 }; 00072 00073 #elif defined(TARGET_RZ_A2M_EVB) || defined(TARGET_RZ_A2M_EVB_HF) 00074 00075 #include "mbed.h" 00076 #include "WM8978_RBSP.h" 00077 00078 /** AUDIO_GRBoard class 00079 * 00080 */ 00081 class AUDIO_GRBoard : public WM8978_RBSP { 00082 public: 00083 00084 /** Create a audio codec class 00085 * 00086 * @param int_level Interupt priority (SSIF) 00087 * @param max_write_num The upper limit of write buffer (SSIF) 00088 * @param max_read_num The upper limit of read buffer (SSIF) 00089 */ 00090 AUDIO_GRBoard(uint8_t int_level = 0x80, int32_t max_write_num = 16, int32_t max_read_num = 16) : 00091 WM8978_RBSP(P8_6, NC, P8_7, P8_4, P9_6, P9_5, P9_4, P9_3, P6_4, int_level, max_write_num, max_read_num) { 00092 } 00093 }; 00094 00095 #else 00096 00097 #include "mbed.h" 00098 #include "AUDIO_RBSP.h" 00099 00100 class AUDIO_GRBoard : public AUDIO_RBSP { 00101 public: 00102 AUDIO_GRBoard(uint8_t int_level = 0x80, int32_t max_write_num = 16, int32_t max_read_num = 16){} 00103 00104 virtual void power(bool type = true) {} 00105 virtual bool format(char length) { return false; } 00106 virtual bool frequency(int hz) { return false; } 00107 virtual int write(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf = NULL) { return -1; } 00108 virtual int read(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf = NULL) { return -1; } 00109 virtual bool outputVolume(float leftVolumeOut, float rightVolumeOut) { return false; } 00110 virtual bool micVolume(float VolumeIn) { return false; } 00111 }; 00112 00113 #endif 00114 00115 #endif
Generated on Wed Jul 13 2022 05:33:36 by
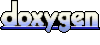