
Water Level Monitoring over IoT for ECE 4180 in Spring 2020 at Gatech
Dependencies: mbed Servo 4DGL-uLCD-SE Ultrasonic HCSR04 Seed_Ultrasonic_Range
main.cpp
00001 /* 00002 ECE 4180 Final Project: Rainwater Harvesting System with IoT 00003 00004 SAMPLE DATA: There's a lot of noise specially in the beginning when water just bounces around the empty tank 00005 reading the sensor at longer intervals of time might help reduce the noise but the data in general looks like 00006 this with bounds (~empty : ~full) => (~192mm : ~ 34mm) 00007 00008 */ 00009 00010 //adding the libraries 00011 #include "mbed.h" 00012 #include "ultrasonic.h" 00013 #include "uLCD_4DGL.h" 00014 #include "Servo.h" 00015 00016 00017 //defining the pinouts 00018 uLCD_4DGL uLCD(p28,p27,p30); // serial tx, serial rx, reset pin; 00019 //ultrasonic mu(p6, p7, .1, 1, &dist); 00020 //I2C rangefinder(p9, p10); //sda, sc1 00021 Serial pc(USBTX, USBRX); //tx, rx 00022 //Servo myservo(p21); 00023 00024 //defining arbitrary variables 00025 int height; 00026 //bool LidClosed = false; 00027 //bool empty; 00028 //float p = 0; 00029 00030 00031 //sensor function for height 00032 void dist(int distance) 00033 { 00034 height = distance; 00035 //height = mu.getCurrentDistance(void); 00036 printf("DISTANC %d mm\r\n", distance); 00037 00038 } 00039 00040 ultrasonic mu(p6, p7, .5, 1, &dist); 00041 00042 //pass on the height return volume 00043 int Volume(int h) 00044 { 00045 double pi = 3.14; 00046 double radius = 6.5; 00047 return(pi*radius*radius*h); 00048 } 00049 00050 00051 00052 //Displays the water level and volume on LCD 00053 void Display() 00054 { 00055 int V = height/3; 00056 volatile int level = 27 + V; 00057 uLCD.baudrate(BAUD_3000000); 00058 uLCD.background_color(BLACK); 00059 uLCD.cls(); //replace with a black retangle instead oc clear screen 00060 uLCD.locate(3,12); 00061 uLCD.printf("VOLUME= %d",Volume(height)); 00062 //uLCD.printf("Volume = "); 00063 //void rectangle(int, int, int, int, int); 00064 //uLCD.locate(1,1); 00065 uLCD.rectangle(50, 25, 102, 92, 0x0FFFFF); 00066 // dynamic rectangle size -> second argument of rectangle should be based on the sonar reading level. 00067 uLCD.filled_rectangle(52, level, 100, 90, 0x00FF00); // fill rectangle based on current volume levels 00068 wait(0.5); 00069 } 00070 00071 00072 00073 //void tankLid() 00074 //{ 00075 // //check is tank is empty and lid is closed then opens 00076 // if(( empty == 1) && (LidClosed == true)) 00077 // { 00078 // for(p=0; p<=10; p++) 00079 // { 00080 // myservo = p/10.0; 00081 // 00082 // } 00083 // LidClosed = false; 00084 // 00085 // } 00086 // 00087 // //checks if tank is full and lid is open then closes 00088 // else if((empty == 0) &&(LidClosed == false)) 00089 // { 00090 // for(p=10; p>=0; p--) 00091 // { 00092 // myservo = p/10.0; 00093 // 00094 // } 00095 // LidClosed = true; 00096 // } 00097 // } 00098 00099 int main() 00100 { 00101 mu.startUpdates(); 00102 00103 while (1) 00104 { 00105 00106 //tank empty == 1 open lid 00107 mu.checkDistance(); //checks height value fro sonar 00108 //height = mu.getCurrentDistance(); 00109 wait(0.5); 00110 Display(); //computes volume and display volume level 00111 wait(0.5); 00112 //stream current level value to SDcard repeat 00113 //pc.printf("DISTANCE %d mm\r\n", height);// 00114 //pc.printf("TEST\n"); 00115 00116 } 00117 00118 } 00119
Generated on Sat Jul 23 2022 17:29:07 by
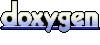