
football_project_wo_output
Fork of football_project by
Embed:
(wiki syntax)
Show/hide line numbers
configs.h
00001 #ifndef CONFIGS_h 00002 #define CONFIGS_h 00003 /////////////////////////////////// HARDWARE SELECTION /////////////////////////////////// 00004 00005 // Board select, if none selected - LPC1768 assumed 00006 #define NORDIC // nRF51822 (highest priority) 00007 #define NUCLEO // Nucleo-F411RE (lesser priority) 00008 #define HARD_V2 // version 2 hardware for nRF51822 00009 00010 // Frequency select 00011 //#define FREQUENCY RF69_868MHZ 00012 #define FREQUENCY RF69_915MHZ 00013 00014 #define HIGH_POWER // High power radio 00015 00016 // Output select 00017 #define NO_DISP // No display? 00018 #define NO_SERIAL // Send info over serial? 00019 #define TEXT_LCD // Text LCD display 00020 #define _I2C_DISP // I2C display (SPI assumed) 00021 #define ENABLE_SOUND // Turns on sound in the beep() function 00022 #define ENABLE_PIN // is there a pin to enable radio via MOSFET? 00023 #define BLE_ENABLE // enable BlueTooth FOTA 00024 00025 /////////////////////////////////// CONSTANTS /////////////////////////////////// 00026 00027 #define EVERY_NODE 255 // Abstract address we are sending the packets to (doesn't matter) 00028 #define NETWORKID 102 // The same on all nodes that talk to each other 00029 00030 #define CYCLE_MS 2400 00031 #define SEND_RATE_MS 400L // 0.33 s 00032 //#define SEND_DESYNC 0.20 // 0.20 -> send rate +/-10% 00033 #define MAX_RECORDS 6 00034 #define NAME_LEN 5 00035 #define SIGNALS_VALID_MS 4000 00036 #define MAX_HISTORY_MS 30000 // 30 seconds 00037 00038 #define NOTE_A3 0.004545454 00039 #define NOTE_A4 0.002272727 00040 #define NOTE_A5 0.001136363 00041 00042 const uint8_t SPACE[] = {1, 50, 60, 100}; // 5, 10, 15, 20 meters 00043 00044 // P-MOSFET 00045 #define LOW_ON 0 00046 #define LOW_OFF 1 00047 00048 #ifdef HARD_V2 00049 00050 // N-MOSFETS 00051 #define MED_ON 1 00052 #define MED_OFF 0 00053 #define HIGH_ON 1 00054 #define HIGH_OFF 0 00055 00056 #else // HARD_V2 00057 00058 // P-MOSFETS 00059 #define MED_ON 0 00060 #define MED_OFF 1 00061 #define HIGH_ON 0 00062 #define HIGH_OFF 1 00063 00064 #endif // HARD_V2 00065 00066 /////////////////////////////////// LOGIC /////////////////////////////////// 00067 #ifdef NORDIC 00068 00069 #ifndef NO_DISP 00070 #define NO_DISP // NORDIC --> no display (use Serial) 00071 #endif 00072 00073 #ifdef NUCLEO 00074 #undef NUCLEO // NORDIC --> not NUCLEO 00075 #endif 00076 00077 00078 #define PRESSED 1 00079 #define RELEASED 0 00080 00081 #else // not NORDIC 00082 00083 #ifdef BLE_ENABLE 00084 #undef BLE_ENABLE // not NORDIC -> no BLE 00085 #endif 00086 00087 #define PRESSED 0 00088 #define RELEASED 1 00089 00090 #endif // NORDIC 00091 00092 #ifdef ENABLE_SOUND 00093 #ifdef NORDIC 00094 #ifdef HARD_V2 00095 #define _TEST_LED_SOUND 00096 #endif 00097 #endif 00098 #endif 00099 00100 /////////////////////// PINS /////////////////////// 00101 #ifdef NORDIC 00102 00103 #ifdef HARD_V2 00104 00105 #define LED_SPACE5 P0_21 00106 #define LED_SPACE10 P0_22 00107 #define LED_SPACE15 P0_0 00108 #define LED_SPACE20 P0_29 00109 #define LED_TEAM_A P0_11 00110 #define LED_TEAM_B P0_9 00111 #define LED_BUZZER_ON P0_8 00112 00113 #define BUZZER P0_5 00114 #define BUZZ_HIGH P0_4 00115 #define BUZZ_MED P0_1 00116 00117 #define BUT_VOL_MORE P0_28 00118 #define BUT_VOL_LESS P0_10 00119 #define BUT_SPACE P0_15 00120 #define BUT_TEAM P0_13 00121 00122 #else // HARD_V2 00123 00124 #define LED_SPACE5 P0_7 00125 #define LED_SPACE10 P0_8 00126 #define LED_SPACE15 P0_9 00127 #define LED_SPACE20 P0_11 00128 #define LED_TEAM_A P0_22 00129 #define LED_TEAM_B P0_0 00130 #define LED_BUZZER_ON P0_3 00131 00132 #define BUZZER P0_5 00133 #define BUZZ_HIGH P0_4 00134 #define BUZZ_MED P0_1 00135 #define BUZZ_LOW P0_2 00136 00137 #define BUT_VOL_MORE P0_28 00138 #define BUT_VOL_LESS P0_10 00139 #define BUT_SPACE P0_21 00140 #define BUT_TEAM P0_15 00141 00142 #endif // HARD_V2 00143 00144 #define RFM_MOSI P0_19 00145 #define RFM_MISO P0_18 00146 #define RFM_SCK P0_17 00147 #define RFM_SS P0_20 00148 #define RFM_IRQ P0_12 00149 #ifdef ENABLE_PIN 00150 #define RFM_ISM_EN P0_16 00151 #endif // ENABLE_PIN 00152 00153 #define CHARGE P0_6 00154 #define V_MEAS 00155 #define VBAT 00156 #define PWR_BTN 00157 00158 #define ANALOG_IN CHARGE 00159 00160 #else 00161 #ifdef NUCLEO 00162 00163 #define LED_SPACE5 PC_3 00164 #define LED_SPACE10 D1 00165 #define LED_SPACE15 PA_14 00166 #define LED_SPACE20 PA_15 00167 #define LED_TEAM_A PC_11 00168 #define LED_TEAM_B PB_2 00169 #define LED_BUZZER_ON PD_2 00170 00171 #define BUZZER PB_8 00172 #define BUZZ_HIGH 00173 #define BUZZ_MED 00174 #define BUZZ_LOW 00175 00176 #define BUT_VOL_MORE PC_12 00177 #define BUT_VOL_LESS PC_10 00178 #define BUT_SPACE PH_1 00179 #define BUT_TEAM USER_BUTTON 00180 00181 #define RFM_MOSI D11 00182 #define RFM_MISO D12 00183 #define RFM_SCK D13 00184 #define RFM_SS D10 00185 #define RFM_IRQ D9 00186 #ifdef ENABLE_PIN 00187 #define RFM_ISM_EN PA_13 00188 #endif // ENABLE_PIN 00189 #define CHARGE 00190 #define V_MEAS 00191 #define VBAT 00192 #define PWR_BTN 00193 00194 #define ANALOG_IN A5 00195 00196 00197 #else // it's LPC 00198 00199 #define LED_SPACE5 00200 #define LED_SPACE10 00201 #define LED_SPACE15 00202 #define LED_SPACE20 00203 #define LED_TEAM_A 00204 #define LED_TEAM_B 00205 #define LED_BUZZER_ON 00206 00207 #define BUZZER p21 00208 #define BUZZ_HIGH 00209 #define BUZZ_MED 00210 #define BUZZ_LOW 00211 00212 #define BUT_VOL_MORE 00213 #define BUT_VOL_LESS 00214 #define BUT_SPACE 00215 #define BUT_TEAM p5 00216 00217 #define RFM_MOSI p11 00218 #define RFM_MISO p12 00219 #define RFM_SCK p13 00220 #define RFM_SS p10 00221 #define RFM_IRQ p9 00222 #ifdef ENABLE_PIN 00223 #define RFM_ISM_EN 00224 #endif // ENABLE_PIN 00225 00226 #define CHARGE 00227 #define V_MEAS 00228 #define VBAT 00229 #define PWR_BTN 00230 00231 #define ANALOG_IN p15 00232 00233 #endif // NUCLEO 00234 #endif // NORDIC 00235 00236 00237 //////////////////////////////////////////////////////////// 00238 #endif // CONFIGS_h
Generated on Mon Jul 18 2022 02:20:10 by
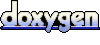