The PCAL6416A is a low-voltage 16-bit general purpose I/O (GPIO) expander with interrupt. This component library is compatible to basic operation as GPIO expanders: PCAL6416A, PCAL9555, PCA9555, PCA9535, PCA9539, PCAL9554, PCA9554 and PCA9538. On addition to this, this library is including mbed-SDK-style APIs. APIs that similar to DigitaiInOut, DigitalOut, DigitalIn, BusInOUt, BusOut and BusIn are available.
GpioDigitalInOut.h
00001 /** GpioDigitalInOut API for GPIO-expander component class 00002 * 00003 * @author Akifumi (Tedd) OKANO, NXP Semiconductors 00004 * @version 0.6 00005 * @date 19-Mar-2015 00006 * 00007 * Released under the Apache 2 license 00008 */ 00009 00010 #ifndef MBED_GpioDigitalInOut 00011 #define MBED_GpioDigitalInOut 00012 00013 #include "mbed.h" 00014 #include "CompGpioExp.h" 00015 #include "GpioDigitalInOut.h" 00016 00017 /** GpioDigitalInOut class 00018 * 00019 * @class GpioDigitalInOut 00020 * 00021 * "GpioDigitalInOut" class works like "DigitalInOut" class of mbed-SDK. 00022 * This class provides pin oriented API, abstracting the GPIO-expander chip. 00023 * 00024 * Example: 00025 * @code 00026 * #include "mbed.h" 00027 * #include "PCAL9555.h" 00028 * 00029 * PCAL9555 gpio_exp( p28, p27, 0x40 ); // SDA, SCL, Slave_address(option) 00030 * GpioDigitalInOut pin( gpio_exp, X0_0 ); 00031 * 00032 * int main() 00033 * { 00034 * pin.output(); 00035 * pin = 0; 00036 * wait_us( 500 ); 00037 * pin.input(); 00038 * wait_us( 500 ); 00039 * } 00040 * @endcode 00041 */ 00042 class GpioDigitalInOut 00043 { 00044 public: 00045 00046 #if DOXYGEN_ONLY 00047 /** GPIO-Expander pin names */ 00048 typedef enum { 00049 X0_0, /**< P0_0 pin */ 00050 X0_1, /**< P0_1 pin */ 00051 X0_2, /**< P0_2 pin */ 00052 X0_3, /**< P0_3 pin */ 00053 X0_4, /**< P0_4 pin */ 00054 X0_5, /**< P0_5 pin */ 00055 X0_6, /**< P0_6 pin */ 00056 X0_7, /**< P0_7 pin */ 00057 X1_0, /**< P1_0 pin (for 16-bit GPIO device only) */ 00058 X1_1, /**< P1_1 pin (for 16-bit GPIO device only) */ 00059 X1_2, /**< P1_2 pin (for 16-bit GPIO device only) */ 00060 X1_3, /**< P1_3 pin (for 16-bit GPIO device only) */ 00061 X1_4, /**< P1_4 pin (for 16-bit GPIO device only) */ 00062 X1_5, /**< P1_5 pin (for 16-bit GPIO device only) */ 00063 X1_6, /**< P1_6 pin (for 16-bit GPIO device only) */ 00064 X1_7, /**< P1_7 pin (for 16-bit GPIO device only) */ 00065 X0 = X0_0, /**< P0_0 pin */ 00066 X1 = X0_1, /**< P0_1 pin */ 00067 X2 = X0_2, /**< P0_2 pin */ 00068 X3 = X0_3, /**< P0_3 pin */ 00069 X4 = X0_4, /**< P0_4 pin */ 00070 X5 = X0_5, /**< P0_5 pin */ 00071 X6 = X0_6, /**< P0_6 pin */ 00072 X7 = X0_7, /**< P0_7 pin */ 00073 X8 = X1_0, /**< P1_0 pin (for 16-bit GPIO device only) */ 00074 X9 = X1_1, /**< P1_1 pin (for 16-bit GPIO device only) */ 00075 X10 = X1_2, /**< P1_2 pin (for 16-bit GPIO device only) */ 00076 X11 = X1_3, /**< P1_3 pin (for 16-bit GPIO device only) */ 00077 X12 = X1_4, /**< P1_4 pin (for 16-bit GPIO device only) */ 00078 X13 = X1_5, /**< P1_5 pin (for 16-bit GPIO device only) */ 00079 X14 = X1_6, /**< P1_6 pin (for 16-bit GPIO device only) */ 00080 X15 = X1_7, /**< P1_7 pin (for 16-bit GPIO device only) */ 00081 00082 X_NC = ~0x0L /**< for when the pin is left no-connection */ 00083 } GpioPinName; 00084 #endif 00085 00086 /** Create a GpioDigitalInOut connected to the specified pin 00087 * 00088 * @param gpiop Instance of GPIO expander device 00089 * @param pin_name DigitalInOut pin to connect to 00090 */ 00091 GpioDigitalInOut( CompGpioExp &gpiop, GpioPinName pin_name ); 00092 00093 /** 00094 * Destractor 00095 */ 00096 virtual ~GpioDigitalInOut(); 00097 00098 /** Set the output, specified as 0 or 1 (int) 00099 * 00100 * @param v An integer specifying the pin output value, 00101 * 0 for logical 0, 1 (or any other non-zero value) for logical 1 00102 */ 00103 virtual void write( int v ); 00104 00105 /** Return the output setting, represented as 0 or 1 (int) 00106 * 00107 * @returns 00108 * an integer representing the output setting of the pin if it is an output, 00109 * or read the input if set as an input 00110 */ 00111 virtual int read( void ); 00112 00113 /** Set as an output 00114 */ 00115 void output( void ); 00116 00117 /** Set as an input 00118 */ 00119 void input( void ); 00120 00121 /** A shorthand for write() 00122 */ 00123 GpioDigitalInOut& operator= ( int rhs ); 00124 GpioDigitalInOut& operator= ( GpioDigitalInOut& rhs ); 00125 00126 /** A shorthand for read() 00127 */ 00128 virtual operator int( void ); 00129 00130 private: 00131 CompGpioExp *gpio_p; 00132 GpioPinName pin; 00133 int output_state; 00134 int config_state; 00135 00136 void write( int pin, int value ); 00137 void configure( int pin, int value ); 00138 } 00139 ; 00140 00141 #endif // MBED_GpioDigitalInOut
Generated on Tue Jul 12 2022 14:15:36 by
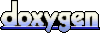