
working multi line scoller message on lcd
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "C12832_lcd.h" 00003 00004 C12832_LCD lcd; // create lcd object 00005 00006 char welcome_str1[] ="**********"; 00007 char welcome_str2[] =" Welcome "; 00008 char welcome_str3[] ="**********"; 00009 00010 int vert_line_hight = 9; 00011 00012 void scroll_msg_right(void); // fuction prototypes 00013 void scroll_msg_left(void); 00014 00015 00016 int main() 00017 { 00018 scroll_msg_right(); 00019 wait(0.1); 00020 scroll_msg_left(); 00021 00022 while(1) 00023 {} 00024 } 00025 00026 00027 00028 // scroll message right 00029 void scroll_msg_right(void) 00030 { 00031 for(int i=10; i>0; i--) // scroll one character on at a time 00032 { 00033 lcd.cls(); 00034 lcd.locate(0,0); 00035 lcd.printf("%s", (welcome_str1+i)); 00036 00037 lcd.locate(0, vert_line_hight); 00038 lcd.printf("%s", (welcome_str2+i)); 00039 00040 lcd.locate(0, vert_line_hight*2); 00041 lcd.printf("%s", (welcome_str3+i)); 00042 00043 wait(0.2); 00044 } 00045 00046 for(int i=0; i<45; i++) // scroll message 00047 { 00048 lcd.cls(); 00049 lcd.locate(i,0); 00050 lcd.printf("%s", welcome_str1); 00051 00052 lcd.locate(i, vert_line_hight); 00053 lcd.printf("%s", welcome_str2); 00054 00055 lcd.locate(i, vert_line_hight*2); 00056 lcd.printf("%s", welcome_str3); 00057 00058 wait(0.075); 00059 } 00060 } 00061 00062 00063 void scroll_msg_left(void) 00064 { 00065 for(int i=45; i>0; i--) 00066 { 00067 lcd.cls(); 00068 lcd.locate(i,0); 00069 lcd.printf("%s", welcome_str1); 00070 00071 lcd.locate(i, vert_line_hight); 00072 lcd.printf("%s", welcome_str2); 00073 00074 lcd.locate(i, vert_line_hight*2); 00075 lcd.printf("%s", welcome_str3); 00076 00077 wait(0.075); 00078 } 00079 00080 for(int i=0; i<11; i++) // scroll one character on at a time 00081 { 00082 lcd.cls(); 00083 lcd.locate(0,0); 00084 lcd.printf("%s", (welcome_str1+i)); 00085 00086 lcd.locate(0, vert_line_hight); 00087 lcd.printf("%s", (welcome_str2+i)); 00088 00089 lcd.locate(0, vert_line_hight*2); 00090 lcd.printf("%s", (welcome_str3+i)); 00091 00092 wait(0.2); 00093 } 00094 }
Generated on Wed Jul 13 2022 21:04:38 by
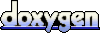