
nearly working
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include <string> 00003 #include <iostream> 00004 using namespace std; 00005 00006 BusOut LED_Disp(p7,p11,p9,p8,p5,p6,p10,p12); 00007 00008 InterruptIn button1(p14); 00009 InterruptIn button2(p15); 00010 volatile bool button1Up = true; 00011 volatile bool button2Up = true; 00012 volatile unsigned int counter = 0; 00013 00014 void DisplayNumber(int num, BusOut &Bus) 00015 { 00016 num = num % 10; 00017 00018 switch(num) 00019 { 00020 case 0: 00021 LED_Disp = ~0x3F; 00022 break; 00023 case 1: 00024 LED_Disp = ~0x06; 00025 break; 00026 case 2: 00027 LED_Disp = ~0x5B; 00028 break; 00029 case 3: 00030 LED_Disp = ~0x4F; 00031 break; 00032 case 4: 00033 LED_Disp = ~0x66; 00034 break; 00035 case 5: 00036 LED_Disp = ~0x6D; 00037 break; 00038 case 6: 00039 LED_Disp = ~0x7D; 00040 break; 00041 case 7: 00042 LED_Disp = ~0x07; 00043 break; 00044 case 8: 00045 LED_Disp = ~0x7F; 00046 break; 00047 case 9: 00048 LED_Disp = ~0x67; 00049 break; 00050 } 00051 } 00052 00053 void up1(){ 00054 button1Up = true; 00055 } 00056 00057 void down1(){ 00058 button1Up = false; 00059 } 00060 00061 void up2(){ 00062 button2Up = true; 00063 } 00064 00065 void down2(){ 00066 button2Up = false; 00067 } 00068 00069 int main() 00070 { 00071 button1.rise(&down1); 00072 button1.fall(&up1); 00073 button2.rise(&down2); 00074 button2.fall(&up2); 00075 00076 while(1){ 00077 LED_Disp = -1; 00078 while(!button1Up) 00079 { 00080 DisplayNumber(counter, LED_Disp); 00081 counter++; 00082 00083 } 00084 while(!button2Up) 00085 { 00086 DisplayNumber(counter, LED_Disp); 00087 counter--; 00088 00089 } 00090 } 00091 00092 00093 } 00094 00095 00096 00097 00098 00099 00100 00101 00102 00103 00104
Generated on Mon Jul 25 2022 08:51:07 by
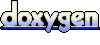