
tes
Dependencies: ASyncTicker EthernetInterface WebSocketClient mbed-rtos mbed MbedJSONValue xbee_lib
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include <stdio.h> 00004 #include "Websocket.h" 00005 #include "xbee.h" 00006 #include "xbeeFrame.h" 00007 #include "MbedJSONValue.h" 00008 #include <ctype.h> 00009 #include <string> 00010 #include "btNode.h" 00011 #include <map> 00012 #include <utility> 00013 #define PORT 80 00014 const std::string HUBID = "1"; 00015 00016 // status leds 00017 // led 1 00018 // lit - successful ethernet connection 00019 DigitalOut led_ethernet(LED1); 00020 00021 // led 2 00022 // lit - successful socket connection 00023 DigitalOut led_socket(LED2); 00024 00025 map<int, btNode> BTnodes; 00026 00027 EthernetInterface ethernet; 00028 Websocket ws("ws://192.168.0.4:8080/"); 00029 00030 bool sync_devices() 00031 { 00032 MbedJSONValue sync_data; 00033 00034 std::string sync_string; 00035 00036 // fill the object 00037 sync_data["TYPE"] = "SYNC"; 00038 sync_data["ORIGIN"] = "HUB"; 00039 sync_data["ID"] = "N/A"; 00040 00041 //sync_data["DATA"] = "N/A"; 00042 //iterate over local nodes and send node list to svr over socket 00043 00044 // map<char, int>::iterator p; 00045 00046 int index = 0; 00047 00048 for (std::map<int, btNode>::iterator it = BTnodes.begin(); it != BTnodes.end(); ++it) 00049 { 00050 MbedJSONValue device = it->second.GetAsJSON(); 00051 printf("dev : %s\n\r", device.serialize()); 00052 sync_data["DEVICES"][index] = device.serialize(); 00053 index++; 00054 } 00055 sync_data["COUNT"] = index; 00056 sync_data["STATUS"] = "0"; 00057 00058 sync_string = sync_data.serialize(); 00059 00060 ws.send((char *)sync_string.c_str()); 00061 00062 // serialize it into a JSON string 00063 00064 00065 return true; 00066 } 00067 00068 void pull_updates() 00069 { 00070 // build json request string 00071 MbedJSONValue request; 00072 00073 std::string s; 00074 00075 // fill the object 00076 request["TYPE"] = "UPDATE"; 00077 request["ORIGIN"] = "HUB"; 00078 request["ID"] = "N/A"; 00079 request["DATA"] = "N/A"; 00080 request["STATUS"] = "N/A"; 00081 00082 // serialize it into a JSON string 00083 s = request.serialize(); 00084 00085 // printf("json: %s\r\n", s.c_str()); 00086 00087 char str[100]; 00088 char id[30]; 00089 char new_msg[50]; 00090 00091 // string with a message 00092 // sprintf(str, "PU"); 00093 ws.send((char *) s.c_str()); 00094 00095 // clear the buffer and wait a sec... 00096 memset(str, 0, 100); 00097 wait(0.5f); 00098 00099 // websocket server should echo whatever we sent it 00100 if (ws.read(str)) 00101 { 00102 memset(id, 0, 30); 00103 memset(new_msg, 0, 50); 00104 00105 // response from server is JSON 00106 // parse back to a traversable object 00107 MbedJSONValue updt_res; 00108 00109 parse(updt_res, str); 00110 printf("> %s from svr with data %s\n\r", updt_res["TYPE"].get<std::string>().c_str(), updt_res["DATA"].get<std::string>().c_str()); 00111 sscanf((char *) updt_res["DATA"].get<std::string>().c_str(), "%s %[^\t]", id, new_msg); 00112 00113 // send string to xbee HERE 00114 if (strlen(new_msg) == 0) 00115 { 00116 printf("--> Nothing to update.\n\r\n\r"); 00117 return; 00118 }else{ 00119 printf("--> id : %s string: %s \n\r", id, new_msg); 00120 } 00121 00122 std::string real_msg(new_msg); 00123 00124 btNode bt = BTnodes.find(atoi(id))->second; 00125 00126 printf("> Found device with ID : %d\n\r\n\r", bt.getID()); 00127 00128 std::string result = bt.SendMessage(real_msg); 00129 00130 printf("--> xbee response : %s\n\r\n\r", result.c_str()); 00131 00132 } 00133 } 00134 00135 int main() 00136 { 00137 //add a registered device to the device array 00138 btNode a(1, "msp430-homer", HUBID); 00139 btNode b(30, "mp430-adam", HUBID); 00140 BTnodes.insert(pair<int, btNode>(1,a)); 00141 BTnodes.insert(pair<int, btNode>(30,b)); 00142 00143 led_ethernet = 0; 00144 led_socket = 0; 00145 00146 ethernet.init(); // connect with DHCP 00147 00148 int ret_val = ethernet.connect(); 00149 00150 if (0 == ret_val) 00151 { 00152 printf("\n\rIP Address: %s\n\r", ethernet.getIPAddress()); 00153 00154 led_ethernet = 1; 00155 } 00156 else 00157 { 00158 error("ethernet failed to connect: %d.\n\r", ret_val); 00159 } 00160 00161 int interval = 5; 00162 00163 if (ws.connect()) 00164 { 00165 led_socket = 1; 00166 } 00167 00168 Timer timer; 00169 00170 timer.start(); 00171 00172 sync_devices(); 00173 00174 // every <interval> seconds call to the server to pull updates 00175 while (true) 00176 { 00177 if (timer.read() >= interval) 00178 { 00179 // perform checks 00180 pull_updates(); 00181 timer.reset(); 00182 timer.start(); 00183 } 00184 } 00185 //ws.close(); 00186 } 00187 00188 00189
Generated on Sat Jul 16 2022 01:27:24 by
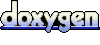