
tes
Dependencies: ASyncTicker EthernetInterface WebSocketClient mbed-rtos mbed MbedJSONValue xbee_lib
btNode.cpp
00001 #include "btNode.h" 00002 #include "mbed.h" 00003 #include "xbee.h" 00004 #include "xbeeFrame.h" 00005 #include "MbedJSONValue.h" 00006 00007 const char btNode::ADDRESS[8] = {0x00, 0x13, 0xA2, 0x00, 0x40, 0x9B, 0x6D, 0xB0}; 00008 00009 btNode::btNode(int D_ID, std::string D_NAME, std::string H_ID){ 00010 ID = D_ID; 00011 DeviceName = D_NAME; 00012 DisplayString = "{Apple:$1;}"; 00013 HubID = H_ID; 00014 00015 00016 } 00017 00018 MbedJSONValue btNode::GetAsJSON() 00019 { 00020 MbedJSONValue thisnode; 00021 00022 //std::string s; 00023 00024 // fill the object 00025 thisnode["device_id"] = ID; 00026 thisnode["device_name"] = DeviceName; 00027 thisnode["device_dataset"] = DisplayString; 00028 thisnode["device_hub_id"] = HubID; 00029 00030 // serialize it into a JSON string 00031 //s = request.serialize(); 00032 00033 return thisnode; 00034 } 00035 00036 std::string btNode::getName() 00037 { 00038 return DeviceName; 00039 } 00040 00041 std::string btNode::getDisplayString() 00042 { 00043 return DisplayString; 00044 } 00045 00046 void btNode::setName(std::string newname) 00047 { 00048 DeviceName = newname; 00049 } 00050 00051 std::string btNode::SendMessage(std::string msg) { 00052 00053 xbeeFrame xbee(p9, 00054 p10, 00055 p11); 00056 00057 std::string full_msg = ""; 00058 00059 char send_data[50] = "xbee string"; 00060 00061 char to_send[100]; 00062 char * p = to_send; 00063 char * r = send_data; 00064 00065 while (*r) 00066 { 00067 *p++ = *r++; 00068 } 00069 00070 *p++ = ' '; 00071 r = (char *)msg.c_str(); 00072 00073 while (*r) 00074 { 00075 *p++ = *r++; 00076 } 00077 00078 *p++ = '\r'; 00079 *p = '\0'; 00080 00081 //printf("the data to send from class is %s\n\r\n\r", to_send); 00082 00083 char data_buf[50]; 00084 00085 xbee.InitFrame(); 00086 xbee.SetDestination((unsigned char *) ADDRESS); 00087 xbee.SetPayload(to_send); 00088 printf("--> Sending payload: %s\n\r\n\r", to_send); 00089 xbee.AssembleFrame(); 00090 xbee.SendFrame(); 00091 00092 00093 00094 for (int i = 0; i < 2; i++) 00095 { 00096 xbee.ReceiveFrame(data_buf, 500); 00097 00098 if (xbee.frameReceived) 00099 { 00100 xbee.frameReceived = 0; 00101 00102 if (xbee.GetType() == TX_STATUS) 00103 { 00104 if (xbee.GetStatus() == 0) 00105 { 00106 printf("--> Send success!\n\r"); 00107 } 00108 else 00109 { 00110 printf("--> Send failed :(\n\r"); 00111 //return "sending failed...."; 00112 } 00113 } 00114 else if (xbee.GetType() == RX_PACKET_64) 00115 { 00116 printf("--> Received data: %s\n\r", data_buf); 00117 } 00118 } 00119 } 00120 00121 00122 00123 std::string response(data_buf); 00124 return response ; 00125 00126 }
Generated on Sat Jul 16 2022 01:27:24 by
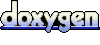