
Code for an ethernet-enabled mbed that calls to a web api, and reasons with JSON resonses
Dependencies: NetServices RPCInterface mbed picojson xbee_api
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "HTTPClient.h" 00004 #include "NTPClient.h" 00005 #include "HTTPServer.h" 00006 #include "RPCFunction.h" 00007 #include <sstream> 00008 #include "picojson.h" 00009 #include "xbee.h" 00010 #include "xbeeFrame.h" 00011 00012 00013 00014 #define HOSTNAME "HUB-01" 00015 #define DEVICENAME "ADAM" 00016 00017 00018 00019 EthernetNetIf eth(HOSTNAME); 00020 HTTPClient http; 00021 NTPClient ntp; 00022 Ticker pull_ticker; 00023 xbeeFrame xbee(p9,p10,p11); 00024 00025 const char dest_address[8] = {0x00, 0x13, 0xA2, 0x00, 0x40, 0x9B, 0x6D, 0xB0}; 00026 const char payload[] = "connection_from_host"; 00027 00028 char send_data[50] = "xbee string"; 00029 00030 00031 using namespace std; 00032 00033 00034 void pull_updates(){ 00035 00036 HTTPText a_txt; 00037 00038 HTTPResult rs = http.get("192.168.0.4:3000/pull_updates", &a_txt); 00039 if (rs==HTTP_OK) { 00040 printf("Result ok : %s (code : %d )\n\r", a_txt.gets(), rs); 00041 } else { 00042 printf("Error %s\n\r", a_txt.gets()); 00043 } 00044 00045 char id[30] = ""; 00046 char new_msg[50] = ""; 00047 00048 sscanf((char *)a_txt.gets(), "%s %s", id, new_msg); 00049 if(strlen(new_msg) == 0){ 00050 printf("no data\n\r"); 00051 return; 00052 }else{ 00053 printf("update device %s with string %s\n\r", id, new_msg); 00054 } 00055 00056 char to_send[100]; 00057 char* p = to_send; 00058 char* r = send_data; 00059 while(*r) 00060 *p++ = *r++; 00061 *p++ = ' '; 00062 r = new_msg; 00063 while(*r) 00064 *p++ = *r++; 00065 *p = '\0'; 00066 00067 00068 00069 char data_buf[50]; 00070 xbee.InitFrame(); 00071 xbee.SetDestination((unsigned char *)dest_address); 00072 xbee.SetPayload(to_send); 00073 xbee.AssembleFrame(); 00074 xbee.SendFrame(); 00075 00076 00077 for(int i = 0; i<2; i++) 00078 { 00079 xbee.ReceiveFrame(data_buf, 500); 00080 if(xbee.frameReceived) 00081 { 00082 xbee.frameReceived = 0; 00083 if(xbee.GetType() == TX_STATUS) 00084 { 00085 if(xbee.GetStatus() == 0) 00086 printf("Send success!\n\r"); 00087 else 00088 printf("Send failed :(\n\r"); 00089 } 00090 else if (xbee.GetType() == RX_PACKET_64) 00091 printf("Received data: %s\n\r", data_buf); 00092 } 00093 } 00094 00095 00096 00097 00098 00099 } 00100 00101 int main() { 00102 00103 00104 printf("Try starting the program with the network disconnected and then connect after a few timeouts reported\n\n\r"); 00105 EthernetErr ethErr; 00106 int count = 0; 00107 do { 00108 printf("Setting up %d...\n\r", ++count); 00109 ethErr = eth.setup(); 00110 if (ethErr) printf("Timeout\n\r", ethErr); 00111 } while (ethErr != ETH_OK); 00112 00113 printf("Connected OK\n\r"); 00114 const char* hwAddr = eth.getHwAddr(); 00115 printf("HW address : %02x:%02x:%02x:%02x:%02x:%02x\n\r", 00116 hwAddr[0], hwAddr[1], hwAddr[2], 00117 hwAddr[3], hwAddr[4], hwAddr[5]); 00118 00119 IpAddr ethIp = eth.getIp(); 00120 printf("IP address : %d.%d.%d.%d\n\r", ethIp[0], ethIp[1], ethIp[2], ethIp[3]); 00121 00122 char ip_buffer[20]; 00123 sprintf(ip_buffer, "%d.%d.%d.%d", ethIp[0], ethIp[1], ethIp[2], ethIp[3]); 00124 00125 //the hub will register itself with the server 00126 HTTPMap msg; 00127 00128 stringstream url; 00129 url << "192.168.0.4:3000/hubconnect?h_id=" << HOSTNAME << "&h_name=" << DEVICENAME << "&h_ip=" << ip_buffer; 00130 const std::string uri = url.str(); 00131 00132 printf("query server : %s \n\r", uri); 00133 00134 HTTPResult r1 = http.post(uri.c_str(),msg,NULL); 00135 if( r1 == HTTP_OK ) 00136 { 00137 printf("Hub %s registered with server (code : %d )\n\r", HOSTNAME, r1); 00138 } 00139 else 00140 { 00141 printf("Could not register, error : %d\n\r", r1); 00142 } 00143 00144 00145 00146 00147 printf("\nHTTPClient get...\n"); 00148 HTTPText txt; 00149 HTTPResult r = http.get("192.168.0.4:3000/devices", &txt); 00150 if (r==HTTP_OK) { 00151 printf("Result ok : %s (code : %d )\n\r", txt.gets(), r); 00152 } else { 00153 printf("Error %s\n", txt.gets()); 00154 } 00155 00156 //begin polling the server for updates to the devices 00157 00158 pull_ticker.attach(&pull_updates, 5.0); 00159 00160 00161 }
Generated on Sat Jul 16 2022 20:24:24 by
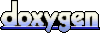