
A program that records images from the OV7670 camera and displays them on a SPI TFT display. Working at 15fps
Fork of OV7670_Testing by
main.cpp
00001 // 00002 // OV7670 + FIFO AL422B camera board test 00003 // 00004 #include "mbed.h" 00005 #include "ov7670.h" 00006 #include "stdio.h" 00007 #include "SPI_TFT.h" 00008 #include "string" 00009 #include "Arial12x12.h" 00010 #include "Arial24x23.h" 00011 #include "Arial28x28.h" 00012 #include "font_big.h" 00013 00014 OV7670 camera( 00015 p28,p27, // SDA,SCL(I2C / SCCB) 00016 p21,p22,p20, // VSYNC,HREF,WEN(FIFO) 00017 Port0,0x07800033, 00018 p23,p24,p25) ; // RRST,OE,RCLK 00019 00020 // the TFT is connected to SPI pin 5-7 00021 SPI_TFT TFT(p5, p6, p7, p8, p14,"TFT"); // mosi, miso, sclk, cs, reset 00022 00023 Serial pc(USBTX,USBRX); 00024 00025 #define SIZEX (160) 00026 #define SIZEY (120) 00027 #define SIZE 19200 00028 00029 unsigned char bank0 [SIZE]; 00030 unsigned char *bank1 = (unsigned char *)(0x2007C000); 00031 00032 int main() { 00033 00034 // Set up the TFT 00035 TFT.claim(stdout); // Send stdout to the TFT display 00036 TFT.background(Black); // Set background to black 00037 TFT.foreground(White); // Set chars to white 00038 TFT.cls(); // Clear the screen 00039 TFT.set_font((unsigned char*) Arial12x12); // Select the font 00040 TFT.set_orientation(3); // Select orientation 00041 00042 // Reset camera on power up 00043 camera.Reset() ; 00044 00045 // Set up for 160*120 pixels RGB565 00046 camera.InitQQVGA() ; 00047 00048 // Print message to screen 00049 pc.printf("Hit Any Key to stop RGBx160x120 Capture Data.\r\n"); 00050 00051 // Kick things off by capturing an image 00052 camera.CaptureNext() ; 00053 while (camera.CaptureDone() == false) ; 00054 00055 // Now enter the main loop 00056 while (!pc.readable()) { 00057 00058 // Start reading in the image data from the camera hardware buffer 00059 camera.ReadStart(); 00060 00061 // Read in the first half of the image 00062 for (int i = 0; i < SIZE; i++) { 00063 bank0[i] = camera.ReadOneByte(); 00064 } 00065 00066 // Read in the second half of the image 00067 for (int i = 0; i < SIZE ; i++) { 00068 bank1[i] = camera.ReadOneByte(); 00069 } 00070 00071 // Stop reading the image 00072 camera.ReadStop() ; 00073 00074 // Immediately request the next image to be captured (takes around 45ms) 00075 camera.CaptureNext() ; 00076 00077 // Use this time to display the image on the screen 00078 // Display the top half 00079 TFT.Bitmap(0,0,160,60,bank1); 00080 // Display the bottom half 00081 TFT.Bitmap(0,60,160,60,bank0); 00082 00083 /* Note: we still have around 15ms left here to do other stuff */ 00084 00085 // Now wait for the image to finish being captured 00086 while (camera.CaptureDone() == false) ; 00087 } 00088 }
Generated on Fri Jul 15 2022 02:45:33 by
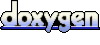