
E-Paper Device (EPD) based MiniNote module, powered by mbed on LPC1114FBD48. Shared in public domain with enclosure in 3D step format, hardware interface compatible with microBUS interface. Anyone can contribute on this project.
ssd1606.h
00001 #ifndef __SSD1606_H__ 00002 #define __SSD1606_H__ 00003 00004 #include "mbed.h" 00005 #define MODE1 00006 00007 /* 00008 The width/height parameters depend on different vendor. The max is 180x128 00009 http://www.solomon-systech.com.cn/cn/product/advanced-display/bistable-display-driver-controller/ 00010 */ 00011 #define SSD1606_WIDTH 172 00012 #define SSD1606_HEIGHT 72 00013 00014 #define GREY_BPP 2 // [1:2], 1bpp or 2bpp 00015 00016 #define COLBUF_SIZE (SSD1606_HEIGHT / 8 * GREY_BPP) // 72/8*2=18 Byte for 2bpp 00017 #define FRAMEBUF_SIZE (SSD1606_WIDTH * COLBUF_SIZE) // 172 * 18 = 3096B 00018 00019 #define IRAM_8KB 00020 00021 #define ROM2BPP_SIZE 3096 00022 #define ROM1BPP_SIZE 1548 00023 00024 //ROM IMAGE INDEX 00025 #define PIC_ONE 1 00026 #define PIC_NOKIA 2 00027 #define PIC_SUNING 3 00028 #define PIC_SUSHI 4 00029 #define PIC_20OFF 5 00030 #define PIC_CHESSBOARD 6 00031 #define PIC_ROYALTY 7 00032 #define PIC_FU 8 00033 #define PIC_GC4 9 00034 #define PIC_SAVE 10 00035 #define PIC_RUSSIAN 11 00036 #define PIC_COMPANY 12 00037 #define PIC_KOREAN 13 00038 #define PIC_OED 14 00039 #define PIC_OEDBOOK 15 00040 #define PIC_JAPANESE 16 00041 #define PIC_RECT 17 00042 00043 //FUNCTION IMAGE INDEX 00044 #define TEST_GREY1 1 00045 #define TEST_GREY2 2 00046 #define TEST_BLK 3 00047 #define TEST_WHT 4 00048 #define TEST_HALF 5 00049 #define TEST_STRIP1 6 00050 #define TEST_STRIP2 7 00051 00052 #define SPI_BUS_DELAY 5 00053 00054 class SSD1606 00055 { 00056 public: 00057 SSD1606(PinName cs, PinName rst, PinName dc, PinName busy, PinName data, PinName clk); 00058 void reset(); 00059 void initialize(); 00060 void update(); 00061 void off(); 00062 void on(); 00063 void deepsleep(); 00064 void sleep(); 00065 void wake(); 00066 void set_inverse(); 00067 void set_lut(); 00068 void set_command_between_images(); 00069 void set_display_start_line(unsigned char val); 00070 void nop(); 00071 void set_charge_pump_enable(unsigned char enable); 00072 void clear(); 00073 void set_pixel(int x, int y); 00074 void clear_pixel(int x, int y); 00075 void set_font(unsigned char *font, unsigned char width); 00076 void set_double_height_text(unsigned int double_height); 00077 void putc(unsigned char c); 00078 void printf(const char *format,...); 00079 void read_busy(void); 00080 void rom_image(const unsigned char *bitmap); 00081 void test_image(unsigned char idx); 00082 00083 private: 00084 //SPI &_mspi; //Software SPI used 00085 DigitalOut _cs, _reset, _dc; 00086 DigitalIn _busy; 00087 DigitalOut _data, _clk; 00088 //unsigned char _screen[3096]; 00089 unsigned char _screen[1548]; 00090 //unsigned char _buf[128]; 00091 int _cursor_x, _cursor_y; 00092 00093 void _send_command(unsigned char code); 00094 void _send_data(unsigned char value); 00095 void _send_data_1bpp(unsigned char value); 00096 void _vspi_write(unsigned char value); 00097 void _draw_pattern(unsigned char value, unsigned int pix); 00098 00099 unsigned char *_console_font_data; 00100 unsigned int _console_font_width; 00101 unsigned int _double_height_text; 00102 }; 00103 00104 00105 #endif
Generated on Mon Jul 18 2022 01:51:50 by
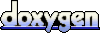