
a
Embed:
(wiki syntax)
Show/hide line numbers
vnh.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file VNH.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-2017 00007 * @brief This file contains the class declaration for the VNH Bridge Driver 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 /* Inclusion guard */ 00013 #ifndef VNH_HPP 00014 #define VNH_HPP 00015 00016 /* The mbed library */ 00017 #include <mbed.h> 00018 /* Functions to compute common mathematical operations and transformations */ 00019 #include <cmath> 00020 00021 namespace drivers{ 00022 // ! Current getter interface 00023 class ICurrentGetter{ 00024 public: 00025 virtual float GetCurrent(void)=0; 00026 }; 00027 00028 /** 00029 * @brief Command setter interface. 00030 * 00031 */ 00032 class ICommandSetter{ 00033 public: 00034 virtual void Run(float pwm); 00035 }; 00036 00037 //! VNH class. 00038 /*! 00039 * It is used for implementing motor bridge control. 00040 * */ 00041 class VNH:public ICurrentGetter, public ICommandSetter 00042 { 00043 public: 00044 /* Constructor */ 00045 VNH(PinName, PinName, PinName, PinName); 00046 /* Destructor */ 00047 ~VNH(); 00048 /* Start */ 00049 void Start(void); 00050 /* Stop */ 00051 void Stop(void); 00052 /* Run */ 00053 void Run(float speed); 00054 /* Brake */ 00055 void Brake(); 00056 /* Inverse */ 00057 void Inverse(float f_speed); 00058 /* Get current */ 00059 float GetCurrent(void); 00060 00061 private: 00062 /* Go current */ 00063 void Go(float speed); 00064 /* increment member */ 00065 float increment; 00066 /* current speed member */ 00067 float current_speed; 00068 /* PWM */ 00069 PwmOut pwm; 00070 /* pin A */ 00071 DigitalOut ina; 00072 /* pin B */ 00073 DigitalOut inb; 00074 /* current value */ 00075 AnalogIn current; 00076 }; 00077 00078 00079 }; // namespace drivers 00080 00081 00082 #endif
Generated on Tue Jul 12 2022 22:40:51 by
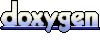