
a
Embed:
(wiki syntax)
Show/hide line numbers
vnh.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file VNH.cpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-2017 00007 * @brief This file contains the class defoinition for the VNH Bridge Driver 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 #include <Drivers/vnh.hpp> 00013 00014 00015 namespace drivers{ 00016 00017 00018 00019 /** @brief VNH class constructor 00020 * 00021 * Constructor method 00022 * 00023 * @param _pwm PWM pin 00024 * @param _ina pin A 00025 * @param _inb pin B 00026 * @param _current current analog pin 00027 */ 00028 VNH::VNH(PinName _pwm, PinName _ina, PinName _inb, PinName _current) 00029 :pwm(_pwm) 00030 ,ina(_ina) 00031 ,inb(_inb) 00032 ,current(_current) 00033 { 00034 pwm.period_us(200); 00035 increment = 0.01; 00036 current_speed = 0; 00037 } 00038 00039 /** @brief VNH class destructor 00040 * 00041 * Destructor method 00042 * 00043 * 00044 */ 00045 VNH::~VNH() 00046 { 00047 } 00048 00049 /** @brief Start method 00050 * 00051 * 00052 * 00053 */ 00054 void VNH::Start(void) 00055 { 00056 ina = !inb; 00057 pwm.write(0); 00058 } 00059 00060 /** @brief Stop method 00061 * 00062 * 00063 * 00064 */ 00065 void VNH::Stop(void) 00066 { 00067 ina = inb; 00068 } 00069 00070 /** @brief Run method 00071 * 00072 * @param speed speed value 00073 * 00074 */ 00075 void VNH::Run(float speed) 00076 { 00077 Go(speed); 00078 } 00079 00080 /** @brief Get current method 00081 * 00082 * 00083 * \return Current value 00084 */ 00085 float VNH::GetCurrent(void) 00086 { 00087 return (current.read()) * 5 / 0.14; 00088 } 00089 00090 /** @brief Go method 00091 * 00092 * @param speed speed value 00093 * 00094 */ 00095 void VNH::Go(float speed) 00096 { 00097 //pwm.write(abs(speed)); 00098 if (speed < 0) 00099 { 00100 ina = 0; 00101 inb = 1; 00102 } 00103 else 00104 { 00105 ina = 1; 00106 inb = 0; 00107 } 00108 pwm =std::abs(speed); 00109 } 00110 00111 /** @brief Inverse method 00112 * 00113 * @param f_speed speed value 00114 * 00115 */ 00116 void VNH::Inverse(float f_speed) 00117 { 00118 ina=!ina; 00119 inb=!inb; 00120 pwm =std::abs(f_speed); 00121 } 00122 00123 /** @brief Brake method 00124 * 00125 * 00126 * 00127 */ 00128 void VNH::Brake() 00129 { 00130 ina.write(0); 00131 inb.write(0); 00132 pwm.write(100.0); 00133 } 00134 00135 }; // namespace drivers
Generated on Tue Jul 12 2022 22:40:51 by
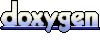