
a
Embed:
(wiki syntax)
Show/hide line numbers
sensorpublisher.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file SensorPublisher.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-year 00007 * @brief This file contains the class declaration for the sensor publisher 00008 * functionality. Because template classes are used, the code was 00009 * placed in a corresponding .inl file and not in a .cpp one. 00010 ****************************************************************************** 00011 */ 00012 00013 /* Include guard */ 00014 #ifndef SENSOR_PUBLISHER_HPP 00015 #define SENSOR_PUBLISHER_HPP 00016 00017 /* The mbed library */ 00018 #include <mbed.h> 00019 /* Container that encapsulates fixed size arrays */ 00020 #include <array> 00021 /* String types, character traits and a set of converting functions */ 00022 #include <string> 00023 #include<TaskManager/taskmanager.hpp> 00024 00025 00026 namespace examples{ 00027 namespace sensors 00028 { 00029 //! ProximityPublisher class. 00030 /*! 00031 * It inherits class task::CTask. 00032 * It is used for publishing proximity sensor values. 00033 */ 00034 template <class C_Sensor,uint Nr_Senrsor> 00035 class ProximityPublisher:public task::CTask 00036 { 00037 public: 00038 /* Sensor array */ 00039 using SensorArrayT = std::array<C_Sensor*,Nr_Senrsor>; 00040 /* Constructor */ 00041 ProximityPublisher(uint32_t,SensorArrayT,Serial&); 00042 /* Serial callback attached to object */ 00043 static void staticSerialCallback(void* obj,char const * a, char * b); 00044 protected: 00045 /* Run method */ 00046 void _run(); 00047 /* Serial callback */ 00048 void serialCallback(char const * a, char * b); 00049 private: 00050 /* Sensor array */ 00051 SensorArrayT m_sensors; 00052 /* Reference to serial object */ 00053 Serial& m_serial; 00054 /* Active flag */ 00055 bool m_isActivate; 00056 }; 00057 }; // namespace sensors 00058 }; // namespace examples 00059 00060 #include "sensorpublisher.inl" 00061 #endif
Generated on Tue Jul 12 2022 22:40:51 by
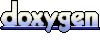